A C# to C++ converter is a tool that translates C# programming constructs into equivalent C++ syntax, allowing developers to seamlessly transition between the two languages.
Here’s a simple code snippet illustrating a basic translation of a C# class to C++:
// C# code
// public class MyClass {
// public void MyMethod() {
// Console.WriteLine("Hello, World!");
// }
// }
// Equivalent C++ code
#include <iostream>
class MyClass {
public:
void MyMethod() {
std::cout << "Hello, World!" << std::endl;
}
};
Understanding the Fundamentals of C# and C++
What is C#?
C# is a high-level programming language developed by Microsoft. It's widely used for developing Windows applications, web services, and game development using the Unity game engine. C# is known for its ease of use, type safety, and a rich set of libraries.
C# supports modern programming paradigms like object-oriented programming and functional programming, making it versatile and robust for varied applications. Its syntax resembles that of other C-derived languages, which allows for seamless transitions for developers familiar with C, C++, or Java.
What is C++?
C++ is a powerful, high-performance programming language that is widely used for system/software development, game programming, and real-time simulations. Recognized for its efficiency and control over system resources, C++ provides low-level manipulation and supports both procedural and object-oriented programming.
C++ introduces features such as templates, operator overloading, and manual memory management — aspects often streamlined or abstracted in higher-level languages like C#. This offers immense flexibility for developers, allowing them to optimize their code at a granular level.
Key Differences Between C# and C++
Understanding the contrast between C# and C++ is essential when considering a C# to CPP converter. Below are critical differences:
-
Memory Management: C# incorporates automatic garbage collection, freeing developers from manual memory handling. In contrast, C++ requires explicit memory allocation (`new`) and deallocation (`delete`), which demands a deeper understanding of memory management.
-
Syntax Differences: While both languages share similar keywords, their syntax can vary significantly. For example, in C#, properties are used for encapsulation, whereas C++ relies on accessor methods.
-
Object-Oriented Programming Concepts: C# supports single inheritance with interfaces, while C++ allows multiple inheritance, making it crucial to understand how these constructs can translate between languages.

Why Would You Need a C# to C++ Converter?
Benefits of Converting C# Code to C++
Converting C# code to C++ can lead to various advantages, including but not limited to:
-
Performance Enhancements: C++ generally provides better performance and control over system resources, making it ideal for applications that require high efficiency, such as game development and real-time systems.
-
Cross-Platform Compatibility: C++ code can be compiled and run on multiple platforms, unlike C#, which is primarily Windows-centric unless using frameworks like .NET Core.
-
Legacy System Integration: Many existing systems and applications rely on C++. Converting code allows for better integration and maintenance of legacy systems while building upon modern architectures.
Scenarios for Conversion
You might require a converter in scenarios like:
- Migrating applications that need better performance.
- Porting game engines or applications into environments where C++ is more suitable.

Tools for C# to C++ Conversion
Overview of Converter Tools
Using converter tools can greatly simplify the process of translating code, allowing developers to streamline their workflow and minimize errors. The right C# to C++ converter can automate much of the tedious coding tasks involved in conversion.
Recommended C# to C++ Converters
Tool: CSharp2CPP
CSharp2CPP is a popular tool that automates the conversion process, providing an interface where you can input your C# code and receive C++ code in return.
How to use it: Simply copy your C# code, paste it into CSharp2CPP’s converter interface, and click the convert button. The tool will generate C++ code, which you can further refine before deployment.
Tool: SharpDevelop
SharpDevelop is an open-source IDE that includes conversion capabilities through plugins. By installing the appropriate plugins, you can export your C# project into C++.
Step-by-step usage: Open your project in SharpDevelop, navigate to the conversion plugin, and follow the prompts to export the C++ version of your code.
Tool: Manual Conversion Approach
Manual conversion should not be overlooked, especially when dealing with complex applications. In certain cases, this approach allows for tailored optimization.
Pros and cons: While manual conversion offers flexibility, it also demands more effort and understanding of the two languages’ intricacies. Errors stemming from incorrect translations can lead to software bugs.

Step-by-Step Guide on Converting C# to C++
Preparing Your C# Code
Before diving into conversion, clean up your C# code. Ensure that it follows best practices, is well-commented, and free of unnecessary complexities. An organized project structure eases the transition to C++.
Basic Syntax Translation
Data Types Conversion
C# has predefined types that may not have direct equivalents in C++. Careful mapping is required to ensure correctness. For example, int in C# translates to int in C++, but decimal in C# may convert to double or a custom fixed-point type in C++.
Code Snippet Example:
// C# code
int number = 42;
decimal price = 19.99m;
// Equivalent C++ code
int number = 42;
double price = 19.99; // decimal in C# does not directly map to C++
Control Structures
Control statements, such as if-else or loops, have similar structures but varying syntax. Transitioning from C# to C++ may require minor adjustments.
Code Snippet Example:
// C# code
if (number > 0) {
Console.WriteLine("Positive Number");
}
// C++ equivalent
if (number > 0) {
std::cout << "Positive Number" << std::endl;
}
Object-Oriented Elements Conversion
Classes and Inheritance
Most object-oriented constructs will convert with little modification. However, C++ allows for multiple inheritance, while C# restricts to single inheritance with interfaces.
Code Snippet Example:
// C# code
public class Animal {
public virtual void Speak() { Console.WriteLine("Animal speaks"); }
}
public class Dog : Animal {
public override void Speak() { Console.WriteLine("Dog barks"); }
}
// Equivalent C++
class Animal {
public:
virtual void Speak() { std::cout << "Animal speaks" << std::endl; }
};
class Dog : public Animal {
public:
void Speak() override { std::cout << "Dog barks" << std::endl; }
};
Interfaces and Abstract Classes
C# interfaces transform into pure virtual classes in C++. It’s crucial to create the equivalent C++ structure properly.
Code Snippet Example:
// C# code
public interface IMovable {
void Move();
}
// C++ equivalent
class IMovable {
public:
virtual void Move() = 0; // Pure virtual function
};
Working with Libraries
.NET Libraries vs C++ Libraries
When converting, recognize that many .NET libraries lack direct C++ counterparts. Replace these with suitable C++ libraries. Familiarity with Boost or STL can be invaluable during this transition.
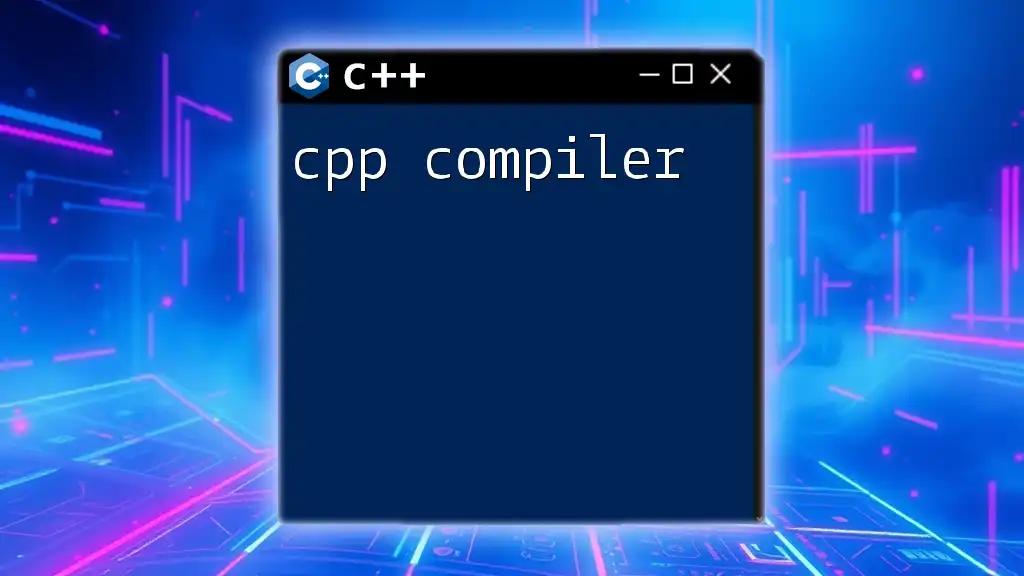
Handling Specific Scenarios in Conversion
Exception Handling
Exception handling differs significantly between C# and C++. C# utilizes a structured exception handling mechanism, while C++ requires careful management with the potential for resource leaks.
Code Snippet Example:
// C# code
try {
// Code that might throw
} catch (Exception ex) {
Console.WriteLine(ex.Message);
}
// C++ equivalent
try {
// Code that might throw
} catch (const std::exception& ex) {
std::cout << ex.what() << std::endl;
}
Memory Management
C# handles memory through garbage collection. In contrast, C++ requires manual memory management, which can lead to memory leaks if not done correctly.
Before converting, ensure that your original C# code does not rely on automatic memory management. As a C++ developer, you'll need to allocate and deallocate memory explicitly.
Code Snippet Example:
// C# code
var list = new List<int> {1, 2, 3};
// C++ equivalent
std::vector<int> list = {1, 2, 3};
// C++ automatically manages memory for STL containers, unlike raw pointers
Utilizing C# Features in C++
Using LINQ in C++
C#’s LINQ provides powerful querying capabilities that are not directly available in C++. To emulate this functionality, you may need to use a combination of algorithm libraries and custom logic.
Code Snippet Example:
// C# LINQ example
var filtered = myList.Where(x => x > 10);
// C++ using STL
std::vector<int> myList = {5, 12, 15, 7};
std::vector<int> filtered;
std::copy_if(myList.begin(), myList.end(), std::back_inserter(filtered), [](int x) { return x > 10; });
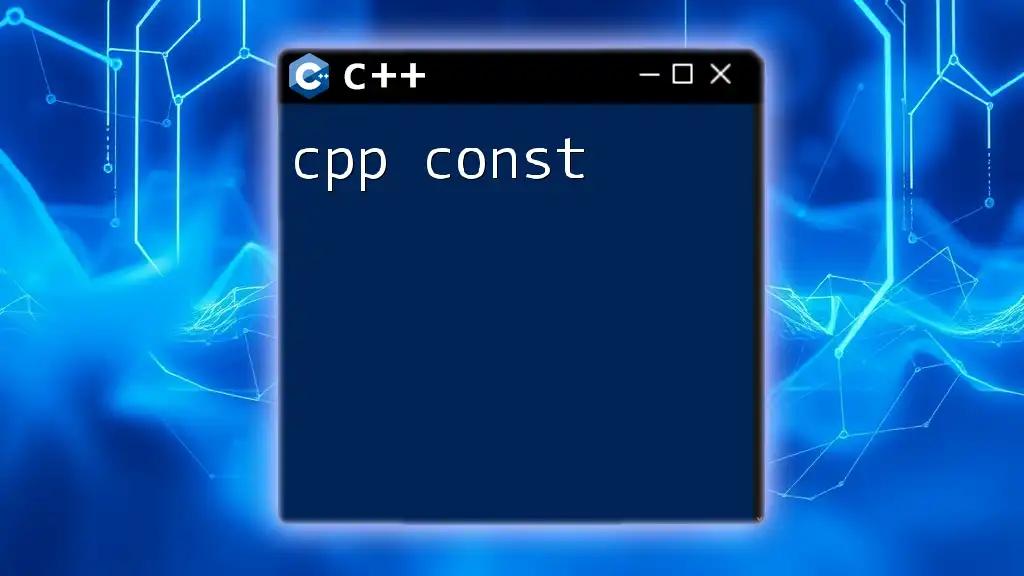
Testing and Debugging Converted Code
Best Practices for Testing
Once you have converted your code, it’s essential to establish testing protocols to ensure correctness. Use unit testing frameworks like Google Test or Catch2 in C++ to validate your logic.
Debugging Common Conversion Errors
Many developers face challenges during conversions due to language-specific errors. Familiarize yourself with common pitfalls such as mismatched data types, memory leaks, and unhandled exceptions that can arise during conversion.
Tools and Techniques for Debugging Converted C++ Code
Utilize debugging tools like gdb on Linux or Visual Studio’s debugging features on Windows to step through your code and find errors efficiently.
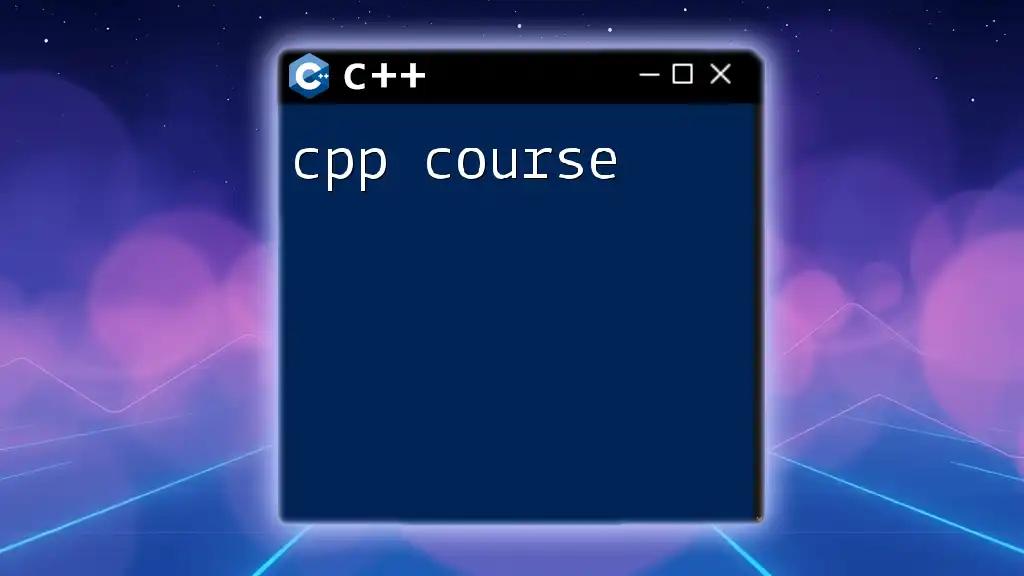
Conclusion
When to Choose Automatic vs Manual Conversion
While each approach has its merits, often a hybrid method can be most effective. Use automated tools to handle repetitive tasks but pay close attention to complex structures and patterns that require a manual touch.
Final Thoughts
The future of C# to C++ conversion is promising, especially as applications become more performance-oriented. Embracing both languages and their strengths can enhance your programming repertoire. With practice and a solid understanding of both paradigms, you can adeptly navigate the transition between C# and C++.
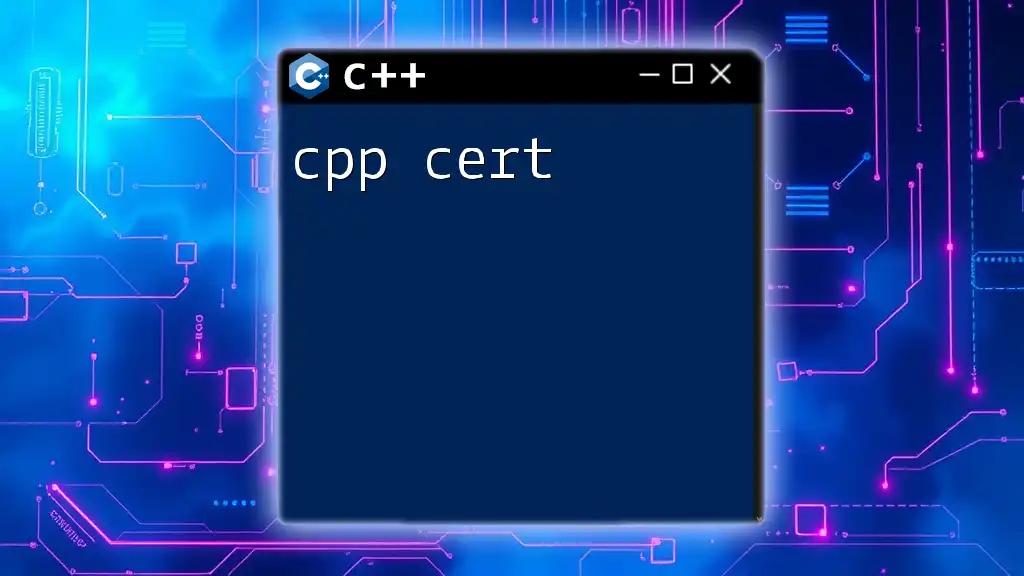
Additional Resources
Links to Further Reading
For detailed language documentation, check out Microsoft’s official C# documentation and C++ reference guides. Online communities like Stack Overflow and GitHub offer support and code sharing, which may assist you further in your conversion projects.
Tutorials & Courses
Consider enrolling in courses focusing on both languages, as well as understanding their nuances. This can significantly boost your ability to handle conversions effectively.