When converting C++ code to C#, the syntax and features of both languages can lead to variations in implementation, as demonstrated in the following example that illustrates a simple function to calculate the square of a number.
// C++ example
int square(int x) {
return x * x;
}
// C# equivalent
int Square(int x) {
return x * x;
}
What is C++?
C++ is a powerful programming language that combines high-level and low-level features, making it suitable for system programming, game development, and performance-critical applications. Developed in the early 1980s, C++ has remained popular due to its versatility and the ability to integrate both procedural and object-oriented programming paradigms.
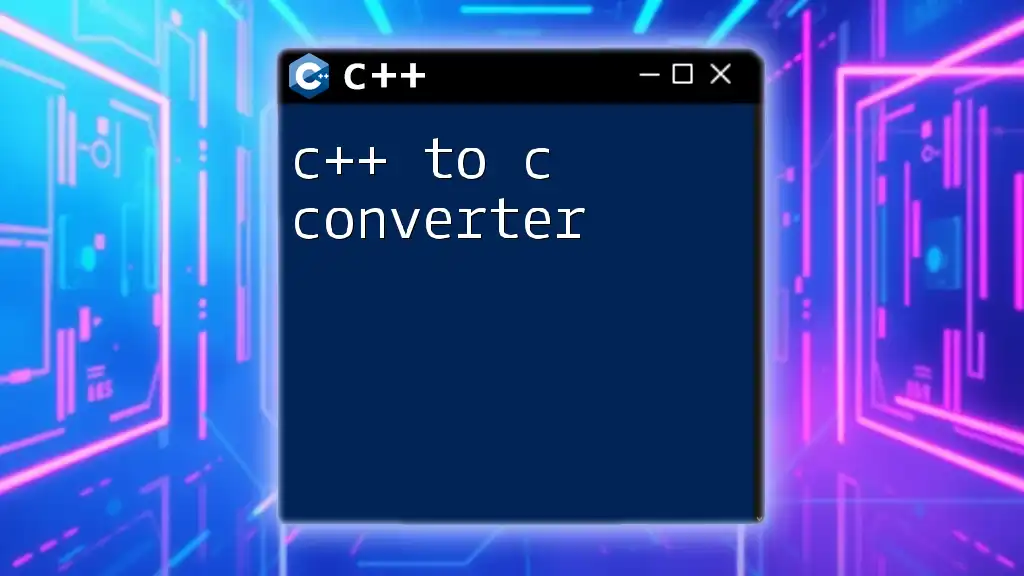
What is C#?
C#, pronounced "C-sharp," is a modern, object-oriented programming language developed by Microsoft as part of its .NET initiative. It is designed to provide a balance between simplicity and power. With strong support for data types, ease of use, and extensive libraries, C# has become a favorite choice for Windows applications, web development, and game development using Unity.
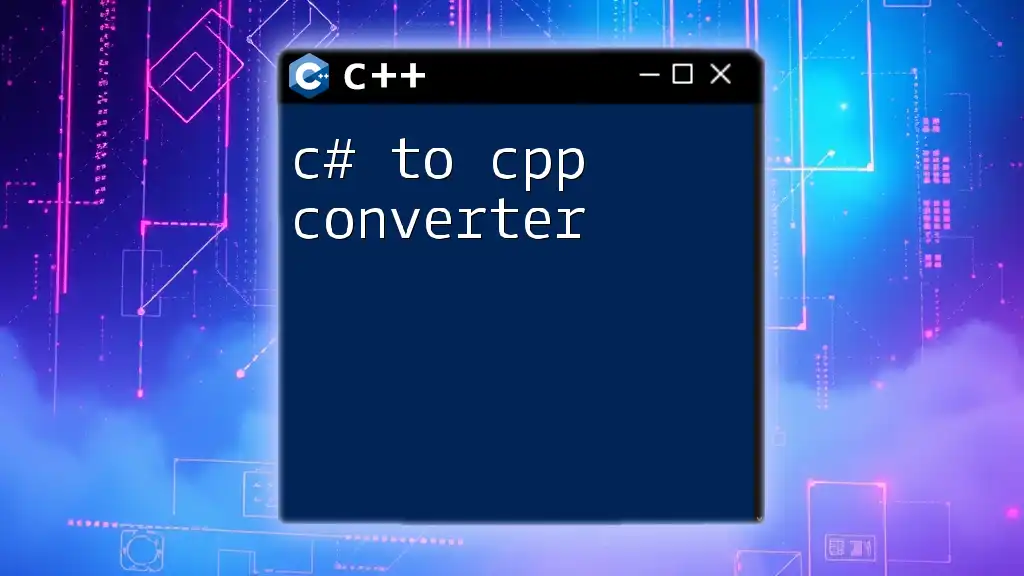
Understanding the Need for Conversion
The need to convert C++ code to C# can arise for several reasons:
- Platform Shift: Moving applications from a desktop to a web-based or cloud environment often necessitates a shift to C# due to its seamless integration with the .NET framework.
- Developer Proficiency: Teams familiar with C# may prefer to work within this paradigm for faster development and maintenance.
- Access to Features: C# provides built-in features like garbage collection and a robust library of tools which can enhance productivity.
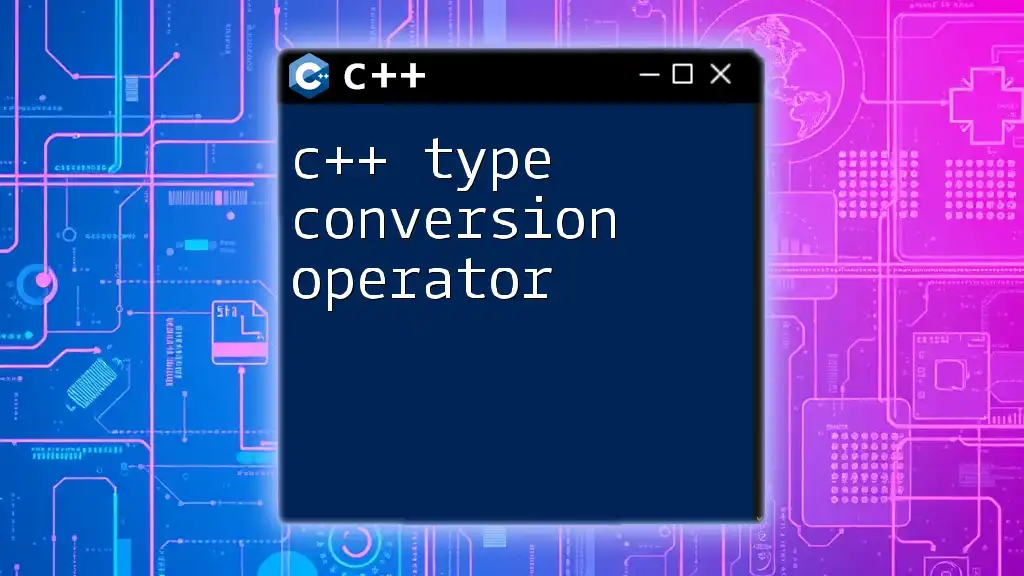
Key Differences Between C++ and C#
Syntax and Structure
The syntax in C++ and C# is similar, but some fundamental differences exist. For instance, while C++ uses pointers extensively, C# abstracts memory management by utilizing references.
Example:
C++ Code:
int main() {
int *ptr = new int(5);
delete ptr;
return 0;
}
C# Code:
class Program {
static void Main() {
int value = 5;
}
}
The C# code does not need to explicitly manage memory allocation and deallocation, simplifying code maintenance.
Memory Management
C++ requires the developer to manage memory manually, which can lead to errors such as memory leaks and segmentation faults. In contrast, C# employs a garbage collector that automatically reclaims memory, enhancing stability and reducing potential errors.
Error Handling
In C++, error handling is often done using return codes and exceptions. C# utilizes a more structured approach with its try-catch-finally blocks.
Example:
C++ Exception Handling:
try {
// Do something risky
}
catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
C# Exception Handling:
try {
// Do something risky
} catch (Exception e) {
Console.WriteLine($"Error: {e.Message}");
}
This structured approach in C# contributes to cleaner and more readable code.
Object-Oriented Programming Concepts
While both C++ and C# support object-oriented programming, their implementations differ significantly.
-
Inheritance: C++ uses multiple inheritance, allowing a class to inherit characteristics from more than one parent class. C# supports single inheritance but allows interfaces to implement multiple inheritance-like behavior.
-
Polymorphism: C++ requires the `virtual` keyword to allow overridden methods, while C# defaults to virtual methods and utilizes `override` to provide specific behavior.
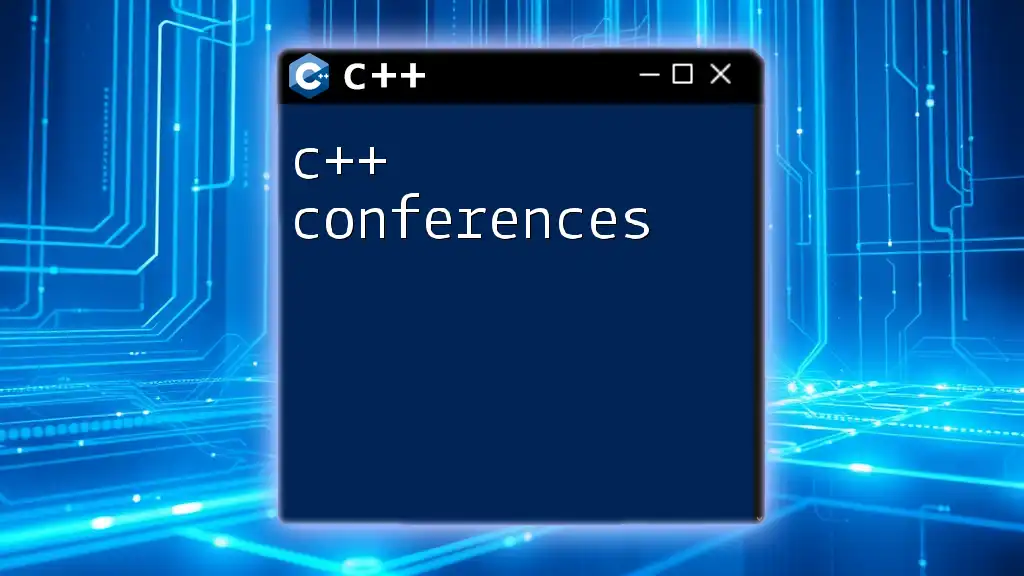
C++ to C# Conversion Strategies
Manual Conversion
Converting C++ code to C# manually can be a complex process, but it gives the developer complete control over the transition. This conversion involves:
- Analyzing the C++ Code: Understand the logic and flow of the original code.
- Mapping C++ Constructs to C#: Identify equivalent structures in C# for variables, control statements, and class definitions.
- Rewriting the Code: Implement the logic in C# while maintaining the original functionality.
Example of Manual Conversion:
class Calculator {
public:
int Add(int a, int b) {
return a + b;
}
};
Converted C# Code:
class Calculator {
public int Add(int a, int b) {
return a + b;
}
}
Using a C++ to C# Converter Tool
Numerous tools are available that automate the conversion process, providing a quicker solution than manual methods. Tools like C++ to C# converters analyze your C++ codebase and generate equivalent C# code, saving significant time.
However, developers should not solely rely on these tools. Always review the generated code for performance and security concerns, and adjust as necessary to fit the needs of the new environment.
Best Practices for Effective Conversion
To ensure a successful transition from C++ to C#, consider the following best practices:
- Test Before and After: Thoroughly test the original C++ code before converting it. After conversion, implement unit tests to ensure the C# code functions as intended.
- Redesign Where Needed: Use the conversion process as an opportunity to refactor and improve the structure and efficiency of your code.
- Document Changes: Maintain clear documentation on the differences encountered during the conversion to help future developers understand the context and decisions made.
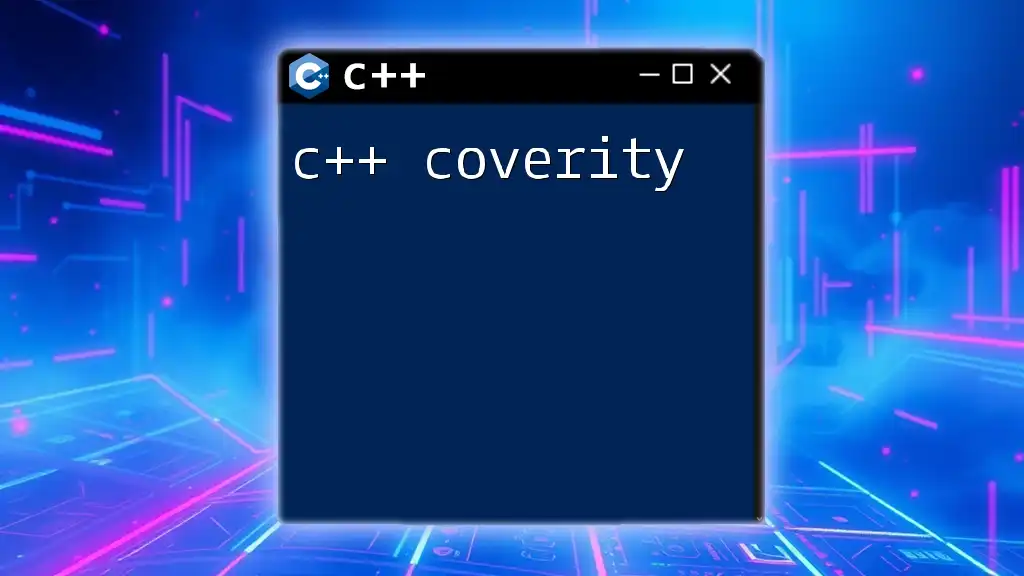
Code Snippet Examples
Simple C++ Program
Here’s a straightforward C++ program that calculates the sum of two numbers.
#include <iostream>
using namespace std;
int main() {
int number1, number2;
cout << "Enter two numbers: ";
cin >> number1 >> number2;
int sum = number1 + number2;
cout << "Sum: " << sum << endl;
return 0;
}
Converted C# Version
The equivalent program in C# would look like this:
using System;
class Program {
static void Main() {
Console.WriteLine("Enter two numbers: ");
int number1 = Convert.ToInt32(Console.ReadLine());
int number2 = Convert.ToInt32(Console.ReadLine());
int sum = number1 + number2;
Console.WriteLine($"Sum: {sum}");
}
}
Error Handling Example
C++ Exception Handling Snippet:
try {
throw runtime_error("Error occurred");
} catch (const runtime_error &e) {
cerr << "Caught Exception: " << e.what() << endl;
}
Equivalent C# Exception Handling:
try {
throw new Exception("Error occurred");
} catch (Exception e) {
Console.WriteLine($"Caught Exception: {e.Message}");
}
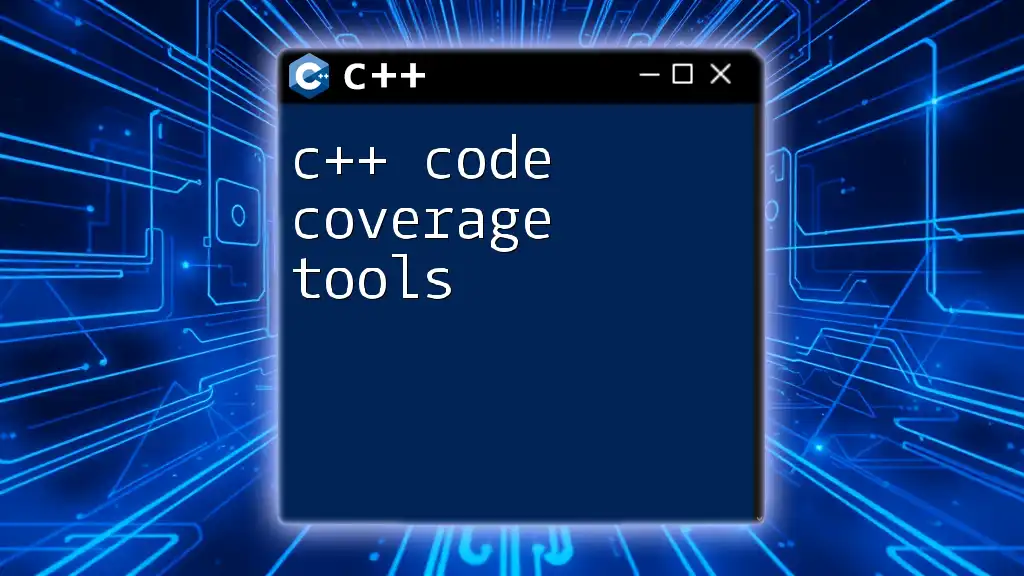
Common Challenges in C++ to C# Conversion
Complex Data Structures
C++ often uses pointers and references, which can complicate the translation to C#. For example, linked lists in C++ rely heavily on pointers, which do not exist in C# in the same manner.
Example:
C++ Linked List Node:
struct Node {
int data;
Node* next;
};
Node* head = new Node();
head->data = 1;
head->next = nullptr;
C# Linked List Node:
class Node {
public int data;
public Node next;
}
Node head = new Node();
head.data = 1;
head.next = null;
Third-Party Libraries
If your C++ code relies on specific libraries, finding C# equivalents may pose a challenge. Develop a list of features dependent on these libraries and seek out the best C# alternatives.
Performance Considerations
While C# brings its own set of advantages, it may introduce performance overhead in certain situations due to garbage collection and the runtime environment. Profiling and optimizing converted code can help mitigate potential performance degradation.
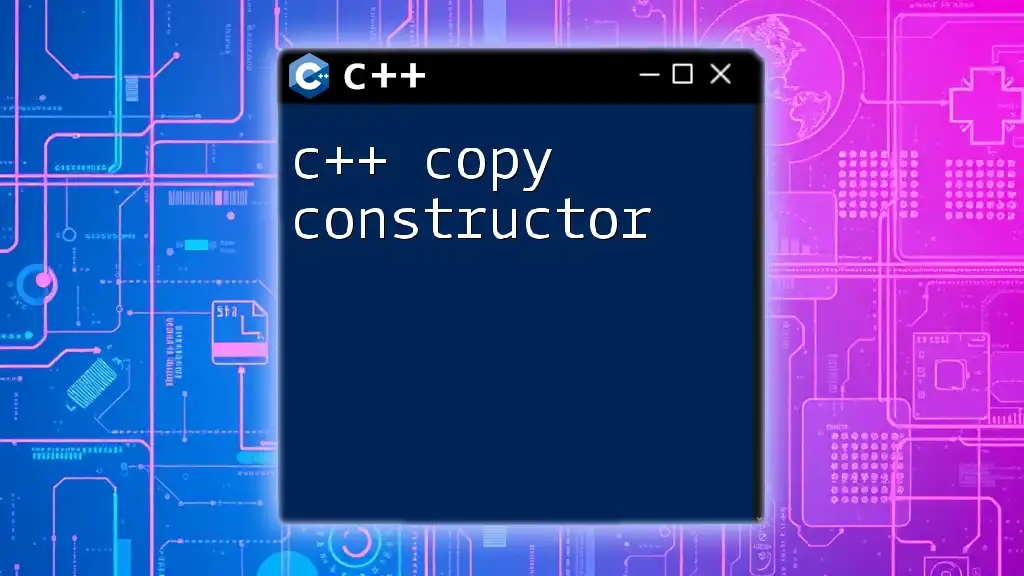
Conclusion
Successfully c++ to c# convert entails understanding key differences, utilizing conversion strategies, and addressing common challenges. By leveraging the tools and techniques discussed, developers can transform their C++ applications into robust C# solutions while maintaining functionality and performance.
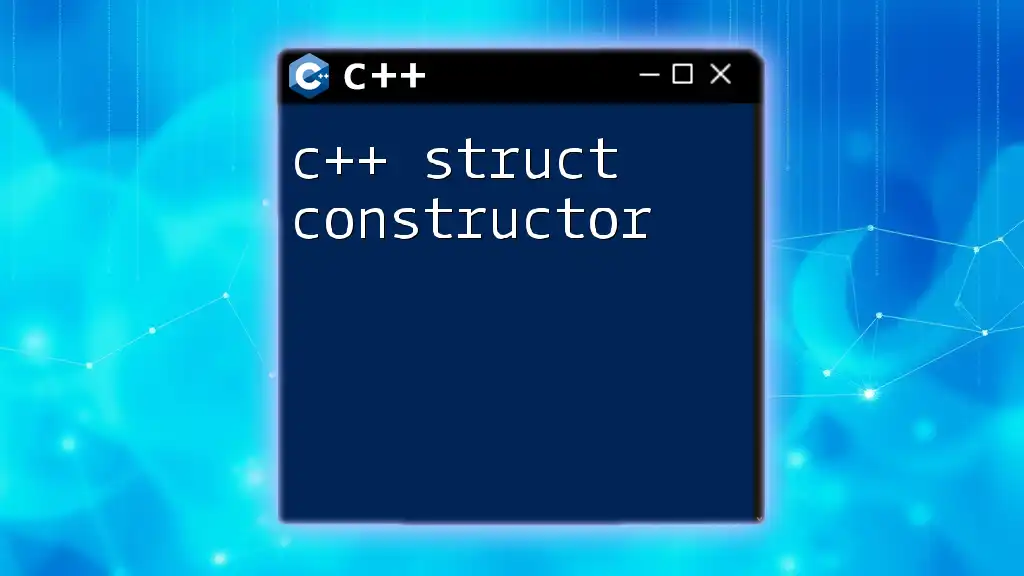
Additional Resources
To continue enhancing your skills in C++ to C# conversion, explore various online resources, tools, and forums that address specific concerns and share best practices within the development community.
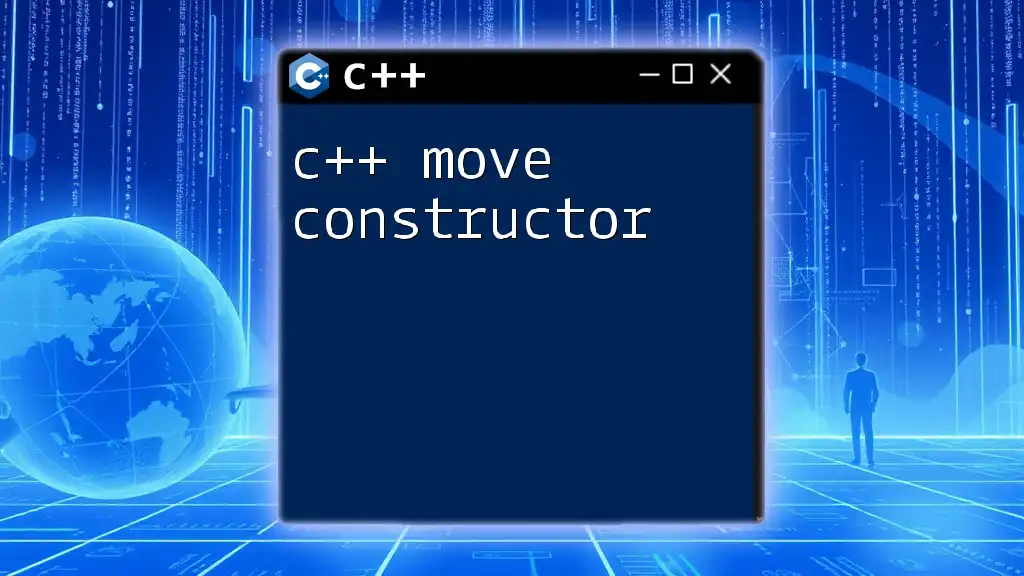
Call to Action
Join our community and share your experiences with C++ and C# conversion. Whether you have questions, insights, or challenges you’ve overcome, engaging with fellow developers can deepen your understanding and improve your skills. If you need personalized assistance or advice, don't hesitate to contact us!