A free C++ compiler allows developers to write, compile, and execute C++ code without any cost, making it accessible for learning and development.
Here's a simple example of a C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Compilers
What is a Compiler?
A compiler is a specialized software program designed to translate high-level programming languages, such as C++, into machine code that a computer's processor can execute. This translation allows developers to write code in a more human-readable form while enabling the computer to understand and execute it efficiently.
How Compilers Work
The functioning of a compiler can be broken down into several key steps, each of which plays a crucial role in the final output of the program.
-
Preprocessing: In this initial stage, the compiler prepares the source code for compilation. This includes handling directives, such as `#include`, and macros. The end result is a modified version of the source code, with all of these directives resolved.
-
Compilation: The core of the process, where the preprocessed code is converted into assembly language, a low-level language that is closer to machine code.
-
Linking: This final stage combines different object files and libraries into a single executable program. It resolves external references, ensuring that all functions and variables are correctly linked.
For example, consider the following simple C++ code snippet:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
During preprocessing, the `#include <iostream>` directive tells the compiler to include the appropriate header for handling output. The actual translation of this code happens in the compilation stage, and during linking, the compiler ensures that the `std::cout` function works correctly.
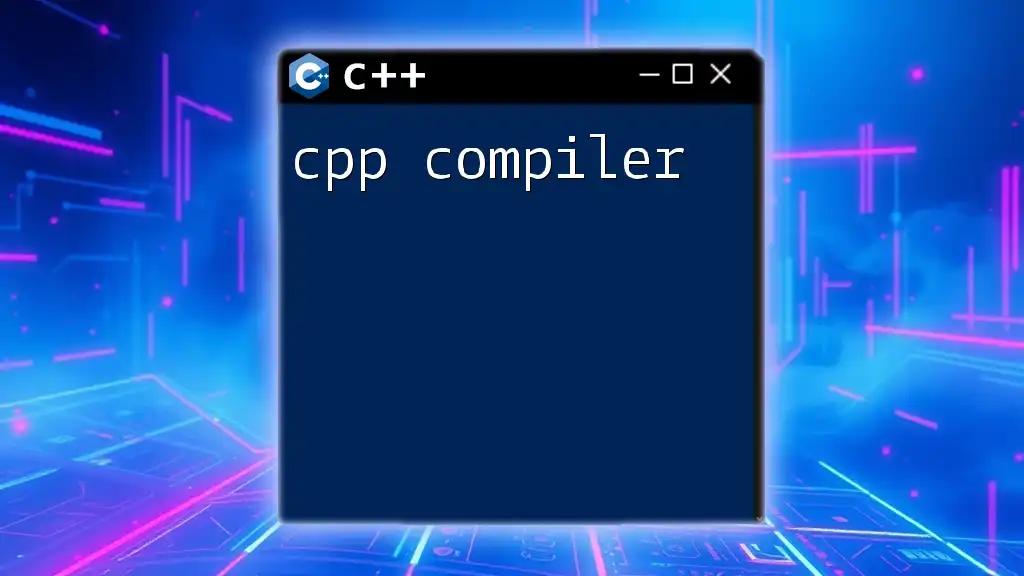
Benefits of Using Free C++ Compilers
Using a free C++ compiler offers numerous advantages, especially for beginners yet also for experienced programmers. Here are some notable benefits:
-
Accessibility for Beginners: Free compilers allow anyone to start learning and practicing C++ without the barrier of software costs, enabling widespread access to programming education.
-
Cost-Effectiveness: Particularly for hobbyists, students, or those working on small projects, free compilers provide a no-cost solution for development, making it sensible for limited budgets.
-
Community Support: Popular free compilers often boast extensive community support, including forums, documentation, and tutorials, which can be invaluable when encountering challenges or complexities in coding.

Popular Free C++ Compilers
GCC (GNU Compiler Collection)
GCC is one of the most widely used compilers for C and C++ programming languages, known for its robustness and cross-platform compatibility.
-
Key Features: It provides excellent support for various languages, a mature debugging interface, and optimization capabilities that improve the performance of the compiled programs.
-
Installation Guide:
- Windows: You can install GCC via MinGW. Download MinGW from [minGW.org](http://www.mingw.org/) and follow the installation instructions.
- macOS: Install GCC using Homebrew. Simply run `brew install gcc` in the terminal.
- Linux: Generally, GCC is pre-installed. You can check by typing `g++ --version` in your terminal. If not, use your package manager (e.g., `sudo apt install g++` for Ubuntu).
-
Example Usage: To compile and run a simple program, use the following commands in your terminal:
g++ hello.cpp -o hello
./hello
Clang
Clang is known for its excellent performance and modular architecture, making it a preferred choice for many C++ developers.
-
Key Features: It offers fast compilation times, a well-designed error message system, and advanced code analysis capabilities.
-
Installation Guide:
- Windows: Download a precompiled binary from [llvm.org](http://llvm.org/), or use a package manager like Chocolatey: `choco install llvm`.
- macOS: Clang comes pre-installed on macOS. You can verify with `clang++ --version`.
- Linux: Use your package manager, i.e., `sudo apt install clang`.
-
Example Usage: Here's how to compile a C++ program:
clang++ hello.cpp -o hello
./hello
Code::Blocks
Code::Blocks is an integrated development environment (IDE) that allows users to develop and compile C++ code seamlessly.
-
Key Features: Offers multiple compiler support, customizability through plugins, and a straightforward user interface.
-
Installation Guide: Download Code::Blocks from [codeblocks.org](http://www.codeblocks.org/) and follow the installation prompts.
-
Creating a New Project with Code::Blocks: Simply select File > New > Project, choose “Console Application,” and follow the wizard to set it up.
-
Compiler Setup in Code::Blocks: Go to Settings > Compiler to choose the installed compiler and tweak the settings accordingly.
-
Example Usage: Write your C++ code as usual, and then build and run it within the IDE via the menu options.
Visual Studio Community
Visual Studio Community is a powerful IDE from Microsoft that includes a free version specifically catered to individual developers and small teams.
-
Key Features: Advanced debugging tools, integrated Git support, and a vast library of extensions.
-
Installation Guide: Download it from the [Visual Studio website](https://visualstudio.microsoft.com/) and select the C++ workload during installation.
-
Writing Your First C++ Program: Create a new project, choose “Console Application”, and write your C++ code in the `main` file.
-
Debugging Features: Utilize breakpoints, step through code, and view variable states to troubleshoot efficiently.
Online Compilers
Online compilers offer a convenient way to write and test C++ code directly from a web browser.
- Overview: They eliminate the need for local installations and allow for quick experiments or collaborative coding.
Repl.it
Repl.it is a user-friendly online compiler that supports C++ among many other languages.
-
Features: Offers a built-in collaborative editor, live sharing feature, and an easy-to-use interface.
-
How to Use Repl.it: Directly open the Repl.it website, select C++, start coding in the provided editor, and run your program with a single click.
Ideone
Ideone is another excellent online compiler primarily for quick code testing.
-
Features: Allows for sharing code snippets and executing them in multiple programming languages.
-
Creating and Running Code Snippets: Go to the Ideone website, select C++, paste your code in the editor, and click “Run” to see the output instantly.
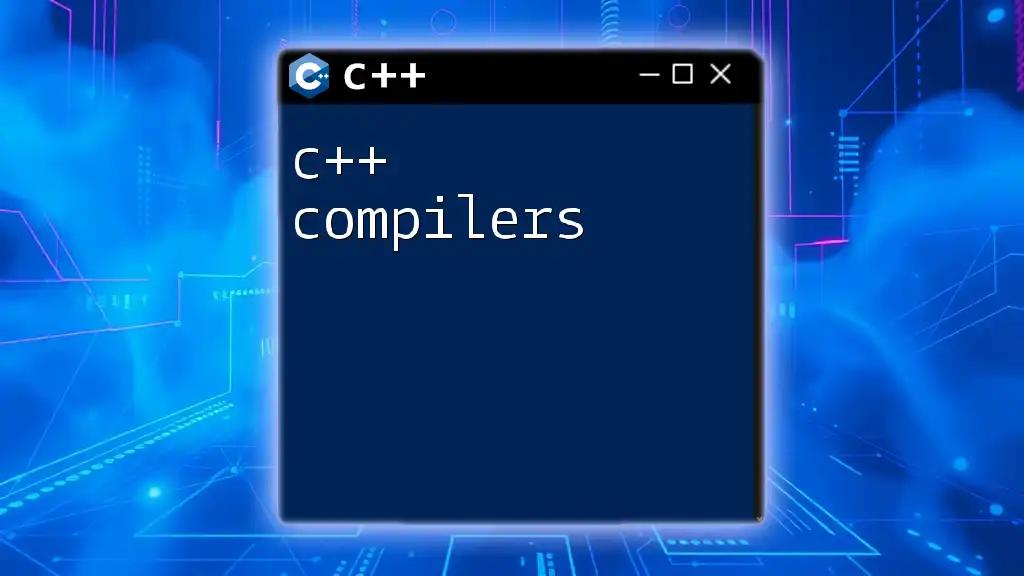
Choosing the Right Compiler for Your Needs
When deciding on a free C++ compiler, consider several factors:
-
Operating System Compatibility: Ensure the compiler you choose works efficiently on your system—Windows, macOS, or Linux.
-
Project Requirements: If you need advanced features like debugging, select a more robust IDE, such as Visual Studio Community or Code::Blocks.
-
Ease of Use and Community Support: Some compilers come with excellent documentation and community forums that can greatly enhance your learning experience.
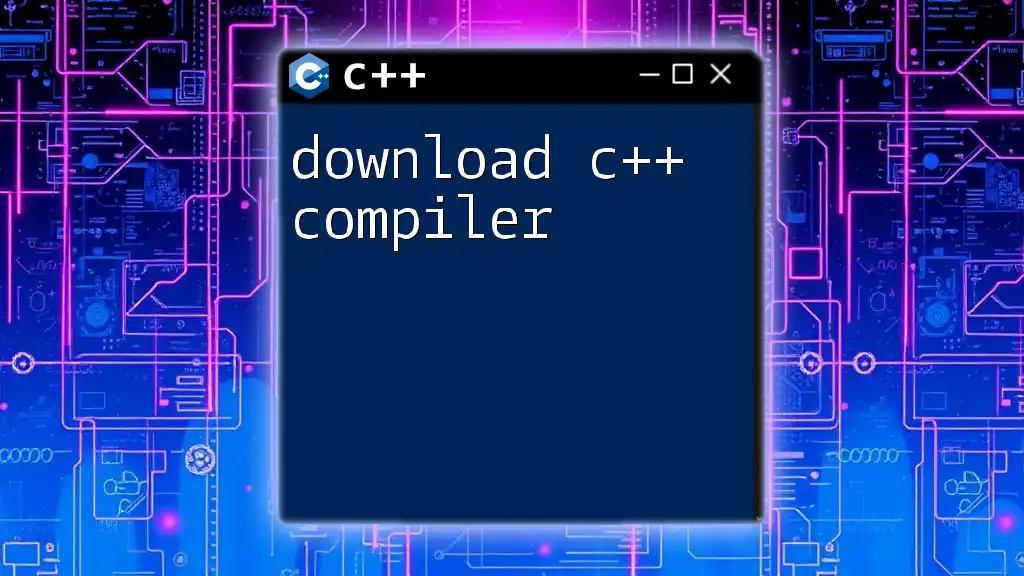
Conclusion
In summary, a free C++ compiler is an essential tool for anyone looking to delve into C++ programming. It not only makes the coding process accessible but also fosters an environment of learning and experimentation. With a plethora of options to choose from, including GCC, Clang, Code::Blocks, and online compilers, you have the flexibility to find the perfect fit for your needs.

Additional Resources
- Links to Official Compiler Documentation: Each compiler typically has its own dedicated documentation that offers detailed instructions and guides for use.
- Recommended C++ Learning Materials: Look for books, online courses, and tutorials that suit your learning style.
- Community Forums for Support: Websites like Stack Overflow and specific compiler forums can provide quick answers to your queries.
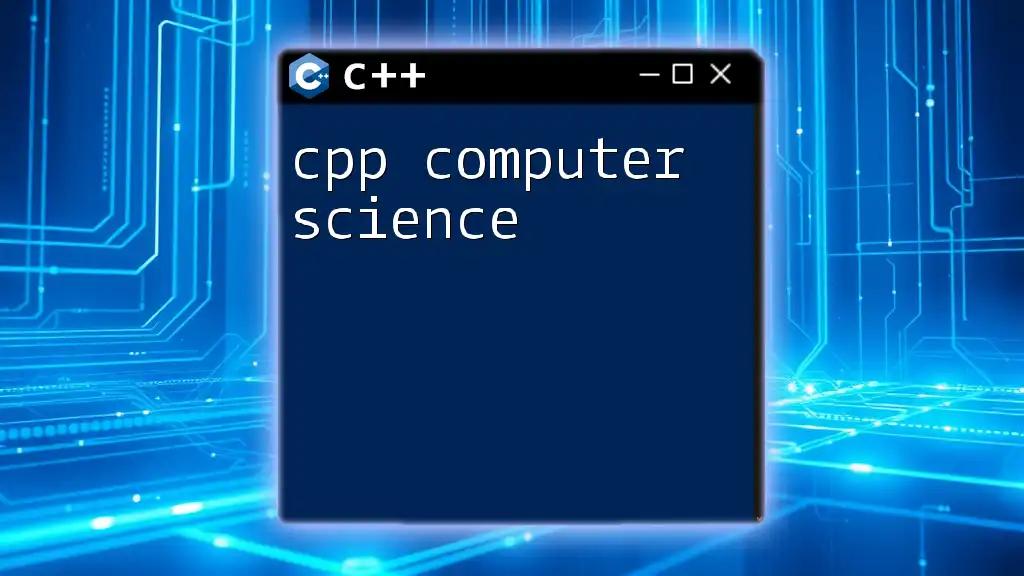
Frequently Asked Questions (FAQs)
What is the best free C++ compiler?
The best free compiler often depends on personal preference and project needs, but GCC and Clang are highly regarded for their features and performance.
Can I use multiple compilers on the same machine?
Yes, you can install and use multiple compilers. They're typically independent, allowing you to switch between them as needed.
How do I troubleshoot compilation errors?
Review error messages closely, check for syntax errors, and consult online resources or community forums to get help with specific issues.