In C++, the `noexcept` keyword is used to indicate that a function is not expected to throw exceptions, allowing the compiler to optimize code and improve performance where exception handling is involved.
Here’s a simple code snippet demonstrating its use:
#include <iostream>
void myFunction() noexcept {
// Function implementation that won't throw exceptions
std::cout << "This function will not throw any exceptions." << std::endl;
}
Understanding Exceptions in C++
In C++, exceptions are anomalies that occur during the execution of a program, disrupting the normal flow of control. They can arise from various situations, including invalid user input, resource unavailability, and logical errors. The ability to handle these exceptions is crucial; this is facilitated by exception handling mechanisms in C++.
The Role of `noexcept`
The `noexcept` keyword is a powerful feature in C++. It explicitly informs the compiler and other developers that a function will not throw exceptions. This declaration can significantly optimize performance and enhance code maintainability, as it reduces the overhead associated with exception handling.
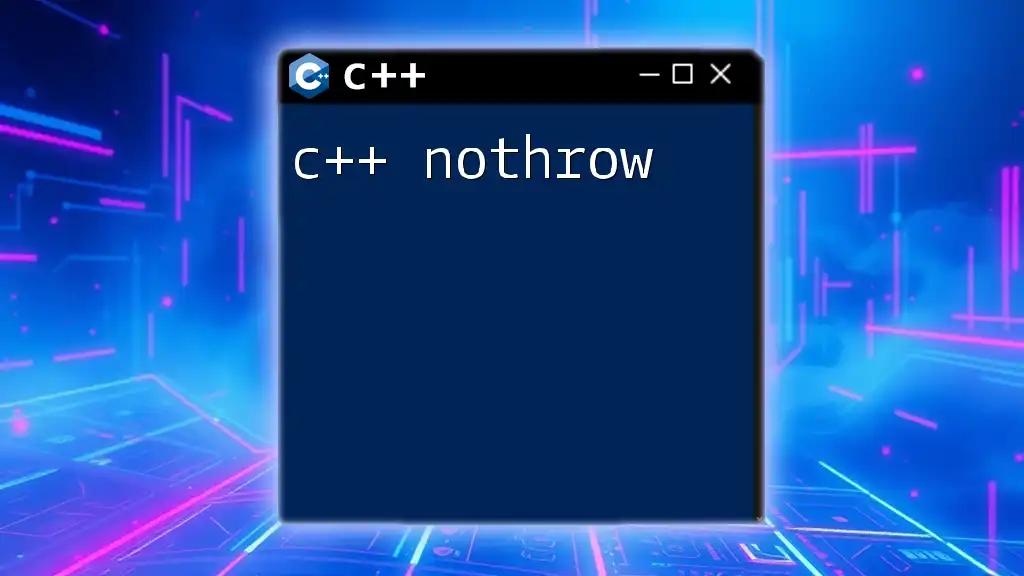
Importance of Using `noexcept`
Performance Benefits
Functions marked with `noexcept` can lead to improved performance. When a function guarantees that it will not throw exceptions, the compiler can make optimizations. For instance, it can omit certain checks and streamline the exception handling path, resulting in faster code execution.
Additionally, using `noexcept` avoids the runtime overhead tied to handling exceptions. When a function is marked as `noexcept`, the C++ standard library can perform certain operations with greater efficiency, knowing that exception-handling code will not need to be invoked.
Improved Code Clarity
The use of `noexcept` brings a level of clarity to code. When developers see the `noexcept` keyword, they immediately understand that the function is expected never to throw exceptions. This creates a promise to users of the function, enhancing the overall readability and maintainability of the codebase.
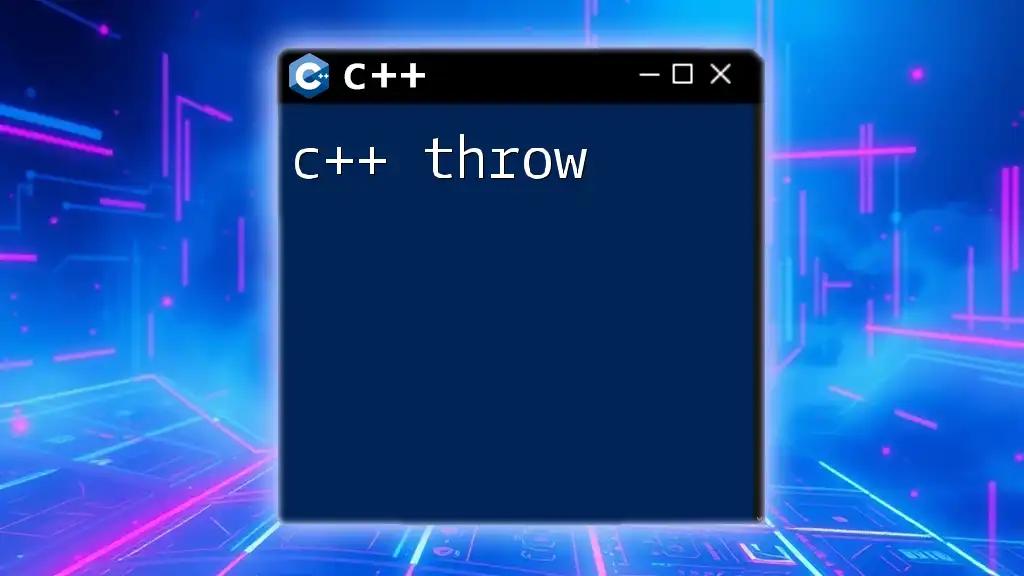
Using `noexcept` in Function Declarations
Basic Usage of `noexcept`
To declare a function as `noexcept`, you simply add the keyword to the function signature. For example:
void myFunction() noexcept {
// Function implementation
}
In this case, `myFunction()` is assured to not throw an exception. If an exception does occur within this function, the program will automatically call `std::terminate()`, leading to an abrupt end of the program.
When to Use `noexcept`
Identifying when to declare a function as `noexcept` is essential. Functions that perform non-throwing operations, such as those manipulating non-throwing objects or performing basic arithmetic, are excellent candidates for `noexcept`. However, it is crucial to avoid marking a function `noexcept` if an error might legitimately arise. This can lead to unintended program termination.
Consequences of Using `noexcept`
Using `noexcept` means understanding its implications. If a function marked `noexcept` does throw an exception, it results in a program termination, which is generally not desirable. As a result, caution is needed in asserting a function as `noexcept`.

`noexcept` with Lambdas and Functors
Declaring Lambdas as `noexcept`
Lambda expressions in C++ can also be declared with `noexcept`. This is a useful practice that ensures the lambda does not throw exceptions, providing the same benefits associated with regular function declarations. Here's how it looks:
auto myLambda = []() noexcept {
// Lambda implementation
};
Using `noexcept` in Functors
Similarly, functors – objects that can be called as if they were functions – can benefit from `noexcept`. Here’s an example:
struct MyFunctor {
void operator()() noexcept {
// Functor logic
}
};
By implementing `noexcept` in functors, you provide assurances of no exceptions being thrown, which can help maintain consistent behavior in complex systems.

The `noexcept` Operator
Understanding the `noexcept` Operator
C++ provides the `noexcept` operator, which allows the evaluation of whether a certain expression might throw an exception. This can be particularly useful for compile-time checks. For example:
void myFunction() {
static_assert(noexcept(myOtherFunction()), "myOtherFunction must not throw");
}
In this case, the static assertion will prevent compilation if `myOtherFunction` is capable of throwing exceptions, thereby promoting safer code practices.
Evaluation of Function Types
The `noexcept` operator helps in determining if a function type is `noexcept`. This is crucial for crafting interfaces that rely on exception safety guarantees, enabling developers to catch possible disallowed usages at compile time.

Exception-Safe Code Design
Writing Exception-Safe Code
Exception safety refers to the guarantees a program provides when exceptions occur. The levels of exception safety include:
- No-throw guarantee: A function will not throw exceptions.
- Strong guarantee: If a function throws, the program state remains unchanged.
- Basic guarantee: Some changes may occur, but no resources are leaked.
`noexcept` is fundamental to establishing a no-throw guarantee, ensuring that user expectations about program behavior are met.
Best Practices for Combining `noexcept` with RAII
When employing Resource Acquisition Is Initialization (RAII), it’s ideal to combine this strategy with `noexcept`. RAII ensures resources are tied to object lifetimes, alleviating concerns about resource leaks. Using `noexcept` within RAII structures further reinforces the design, as destructors for RAII classes must not throw exceptions.
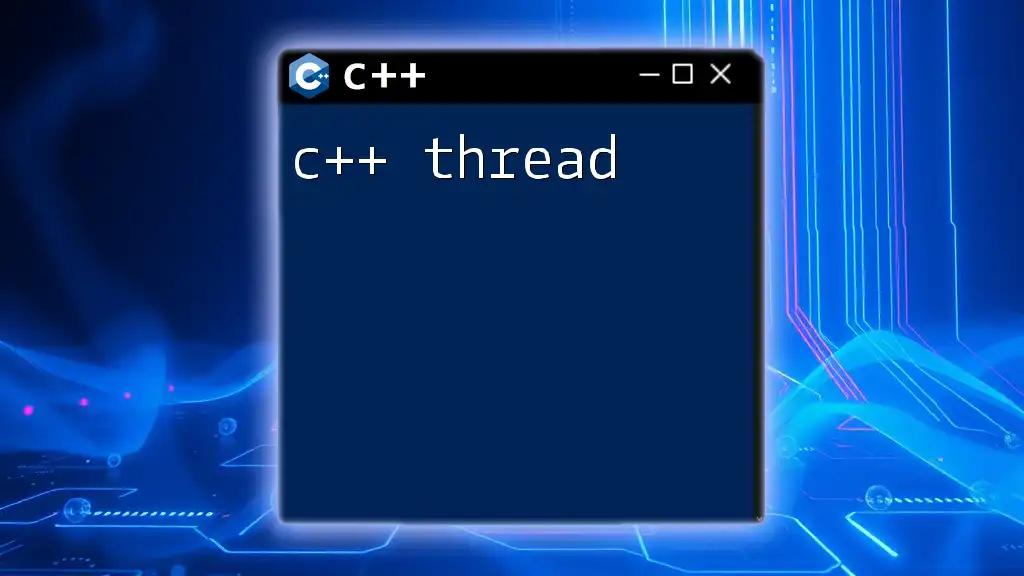
Trade-offs of Using `noexcept`
Potential Drawbacks
While `noexcept` has considerable advantages, there are potential drawbacks. For instance, using `noexcept` can inadvertently restrict the ability to utilize standard library functions that may throw exceptions, leading to design limitations.
Alternatives to `noexcept`
In certain situations, traditional exception handling might be safer or more appropriate. While `noexcept` promotes performance and clarity, being flexible and judicious about when to apply it is particularly vital in complex systems. Understanding when to employ traditional error handling can lead to more robust applications in scenarios where exceptions are expected and managed effectively.
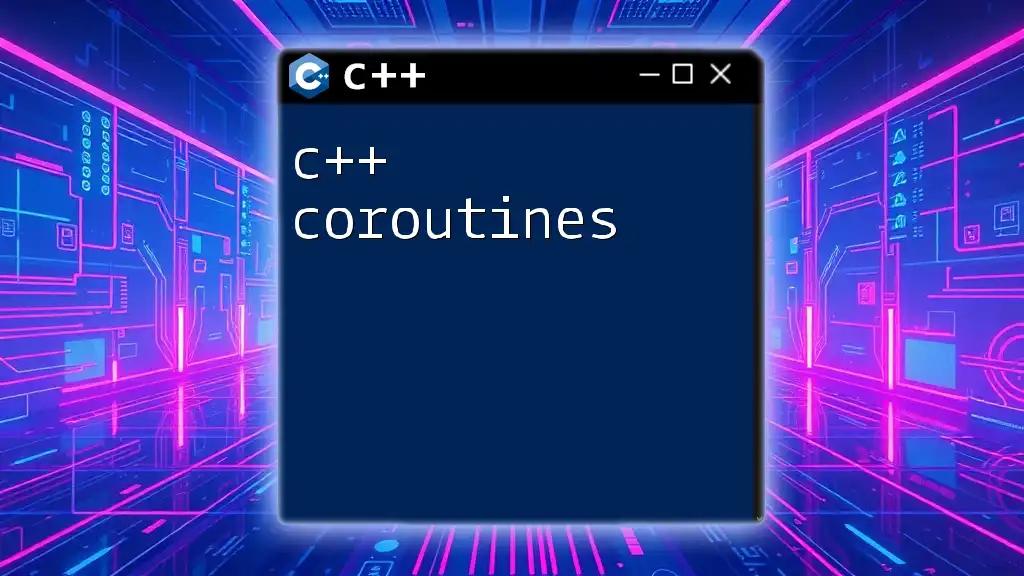
Conclusion
In conclusion, C++'s `noexcept` feature plays a significant role in modern C++ programming by offering enhanced performance and improved code clarity. Understanding how to effectively use the `noexcept` keyword in functions, lambdas, and functors is essential for crafting robust and efficient applications. As you explore more about `c++ no throw`, practicing its implementation will not only hone your skills but also improve the quality of your code. Remember, the balance between performance and safety is key in utilizing `noexcept` effectively, and careful consideration should be applied in its usage.

Additional Resources
To further enhance your understanding of `noexcept` in C++, consider diving into recommended books and online tutorials. Engaging with online communities dedicated to C++ development can also provide valuable insights and peer support. Consulting the official C++ documentation is essential for exploring the intricacies of `noexcept` and its impact on programming practices.