In C++, the `throw` keyword is used to signal an exception that can be caught and handled by a try-catch block, allowing for error management in programs.
#include <iostream>
void exampleFunction(int value) {
if (value < 0) {
throw std::invalid_argument("Negative value error");
}
std::cout << "Value is: " << value << std::endl;
}
int main() {
try {
exampleFunction(-5);
} catch (const std::invalid_argument& e) {
std::cerr << "Caught exception: " << e.what() << std::endl;
}
return 0;
}
What is `throw` in C++?
The `throw` keyword in C++ is a critical component of the language's exception handling mechanism. It is used to indicate a problem during the execution of a program, allowing the program to respond to errors in a flexible manner without crashing outright. By utilizing `throw`, developers can signal that a particular operation has encountered a condition that it cannot handle.
The role of `throw` is to "throw" an exception from one part of a code base to another, allowing it to be caught and handled elsewhere. It’s essential for robust error handling, offering a clear separation between normal and exceptional flows of control in your programs.
The `throw` Keyword
Using `throw` is integral for effective error reporting in C++. Unlike traditional methods such as returning error codes, which can be easily ignored, `throw` creates an explicit signal that something went wrong. By using exceptions, developers can manage errors with greater context and fewer chances of oversight, as they force the program to handle or propagate these errors.
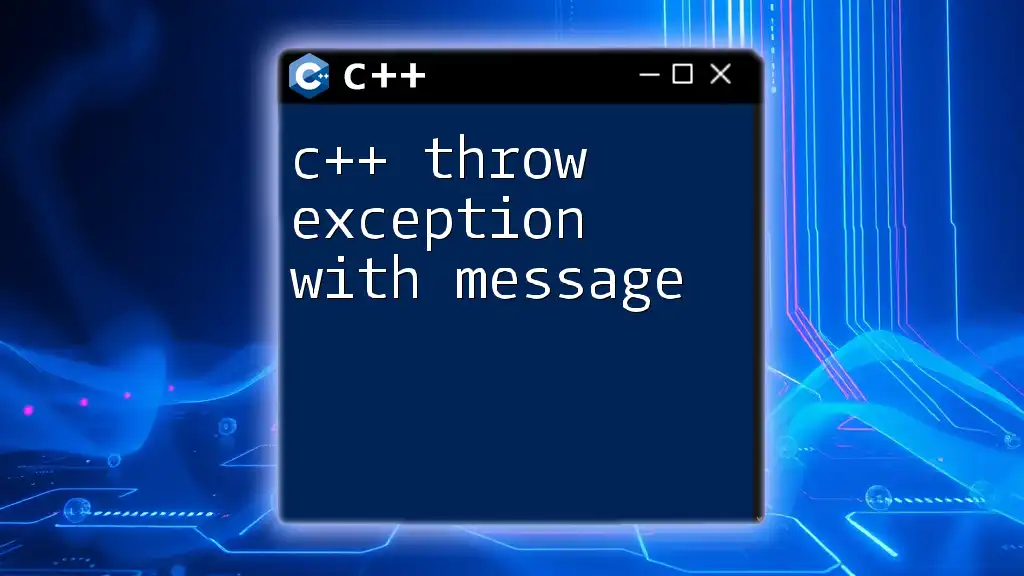
Basic Syntax of `throw`
To use `throw`, you need a simple yet effective syntax. The core structure of a `throw` statement is:
throw expression;
The `expression` can be any valid expression, including an object of an exception class.
Example: Throwing a Simple Exception
Here’s a foundational example of using `throw`:
#include <iostream>
#include <stdexcept>
void riskyFunction() {
throw std::runtime_error("Risky operation failed!");
}
int main() {
try {
riskyFunction();
} catch (const std::exception &e) {
std::cout << "Caught exception: " << e.what() << std::endl;
}
return 0;
}
In this example, `riskyFunction` throws a `std::runtime_error` if it encounters an issue. The `main` function wraps the call in a `try` block, which effectively catches the exception if it occurs. The `catch` block then provides feedback, indicating exactly what went wrong through the `what()` method.
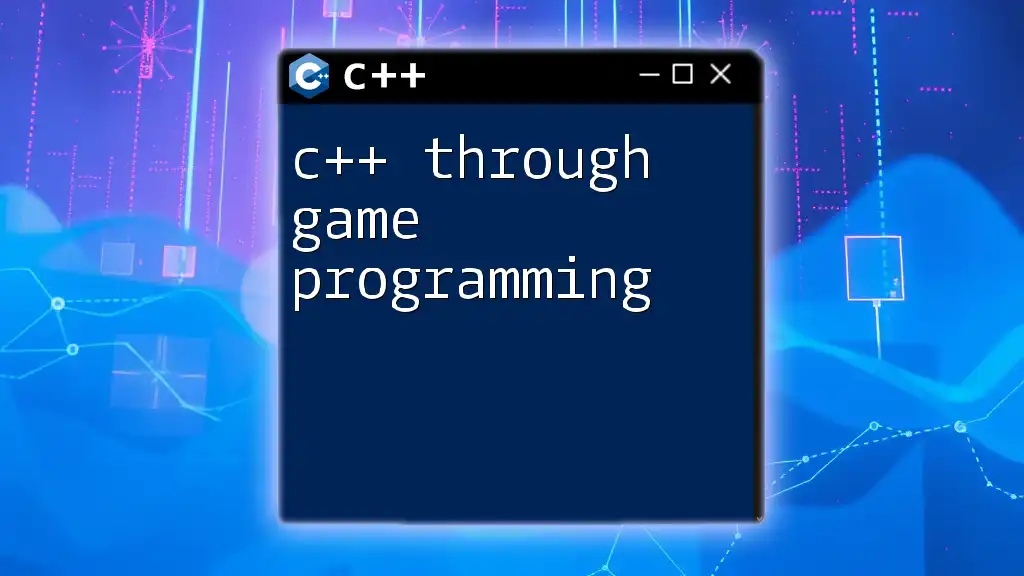
How `throw` Works with Exception Handling
The Relationship between `throw`, `try`, and `catch`
In C++, exception handling revolves around three keywords: `try`, `catch`, and `throw`. When you anticipate that a certain piece of code might throw an exception, it is enclosed in a `try` block. If an exception occurs, control transfers from the point of the exception to a corresponding `catch` block that can handle that exception.
Example: Complete Try-Catch Block
Let’s consider another example that shows this relationship more clearly:
#include <iostream>
#include <stdexcept>
void divide(int a, int b) {
if (b == 0) {
throw std::invalid_argument("Division by zero is not allowed");
}
std::cout << "Result: " << a / b << std::endl;
}
int main() {
try {
divide(10, 0);
} catch (const std::invalid_argument &e) {
std::cerr << "Caught: " << e.what() << std::endl;
}
return 0;
}
In this code, the `divide` function checks for a division by zero. When `b` is zero, a `std::invalid_argument` exception is thrown. The `main` function’s `try` block calls `divide`, and upon encountering the exception, control passes to the corresponding `catch` block to handle the error. This structure encourages a clean separation of normal code from error handling code, enhancing readability and maintainability.
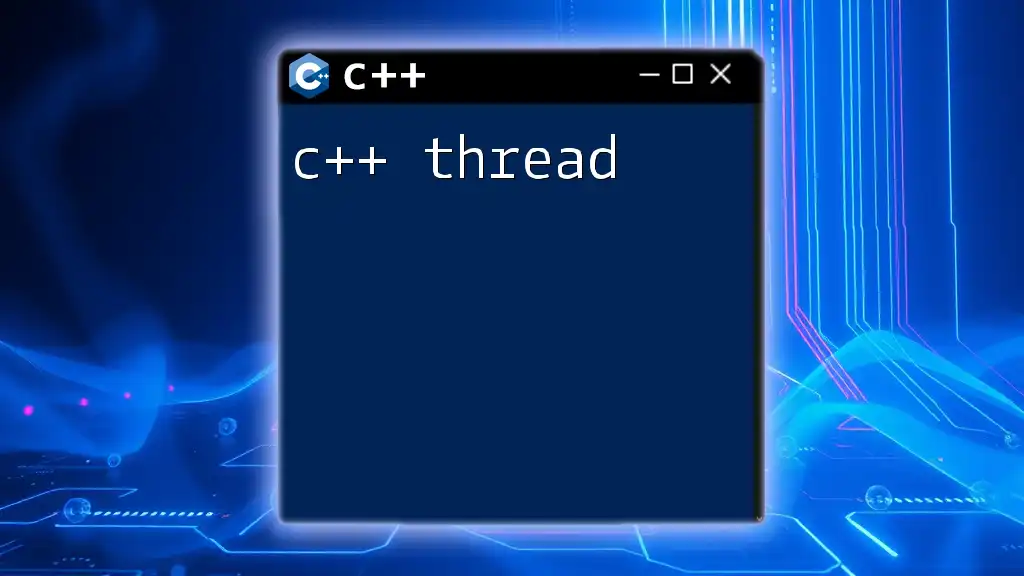
Types of Exceptions and `throw`
Standard Exceptions in C++
C++ comes with a rich library of standard exceptions defined in the `<stdexcept>` header. These standard exceptions categorize errors into logical groups, making it intuitive for developers to handle various error scenarios.
Common exception types include:
- `std::runtime_error`: Indicates errors that occur during runtime which are not strictly predictable.
- `std::logic_error`: Represents errors in logic that can be detected at compile time.
Creating Custom Exceptions
Sometimes, standard exceptions aren’t enough. C++ allows developers to define custom exception classes by inheriting from `std::exception`. This gives developers the flexibility to create error types specific to their applications.
Here's an example of a custom exception:
#include <iostream>
#include <exception>
class CustomException : public std::exception {
public:
const char* what() const noexcept override {
return "This is a custom exception";
}
};
void triggerCustomException() {
throw CustomException();
}
int main() {
try {
triggerCustomException();
} catch (const CustomException &e) {
std::cout << "Caught: " << e.what() << std::endl;
}
return 0;
}
In this example, the `CustomException` class overrides the `what()` method to provide a specific error message. When `triggerCustomException` is called, it throws an instance of `CustomException`, which is then caught and handled in the `main` function.
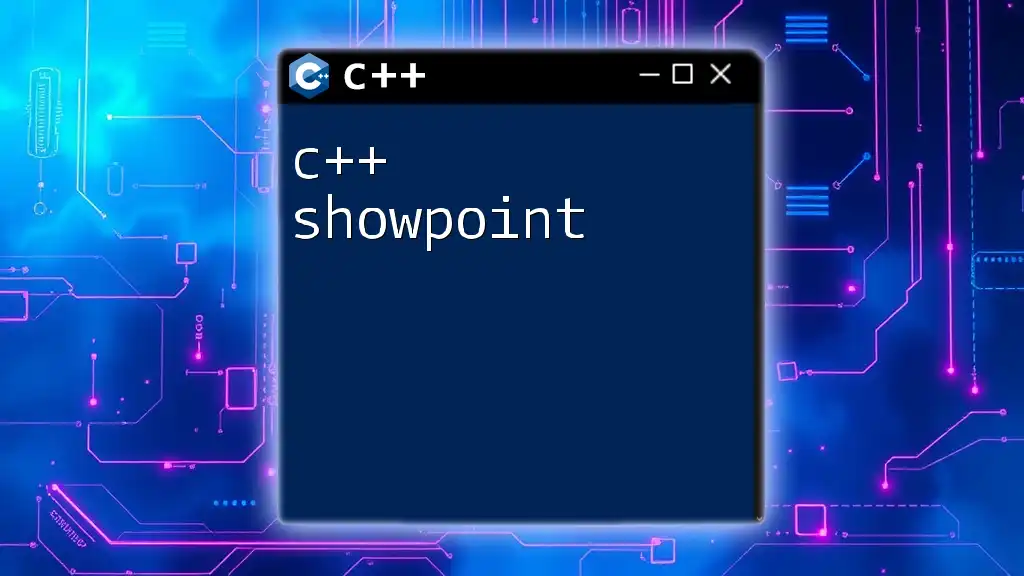
Best Practices for Using `throw` in C++
When to Use `throw`
Using `throw` effectively requires understanding when exceptions are appropriate:
- When an operation cannot complete as intended: For instance, reading from a file that does not exist should throw an error.
- To enforce preconditions: If a function expects certain conditions to be true before it can execute, throwing exceptions when they are not met can be a good safety mechanism.
Avoiding Common Pitfalls
C++ developers should be cautious about several common pitfalls:
- Overusing exceptions: Exceptions can introduce complexity. Avoid throwing exceptions in critical loops or performance-sensitive areas unless absolutely necessary.
- Catching exceptions too broadly: Be specific in catching exceptions to avoid masking issues and introducing unintended behaviors.
- Not cleaning up resources: When exceptions are thrown, it is vital to ensure that any resources are cleaned properly to avoid resource leaks.
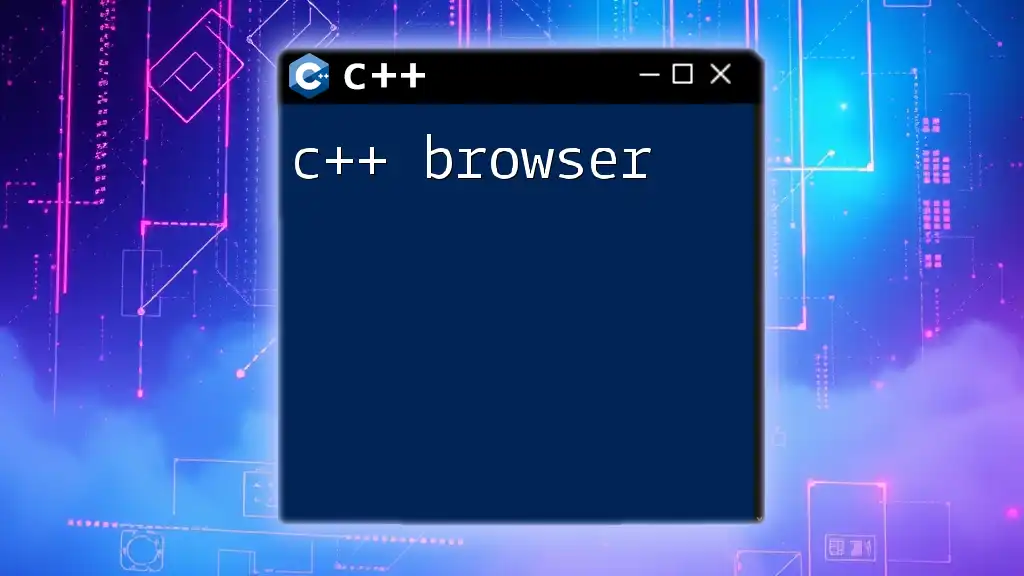
Performance Considerations
Performance Overheads of Exceptions
While exceptions provide powerful error handling, they do come with overheads. The process of handling an exception—throwing, catching, and unwinding the stack—can be more resource-intensive compared to simple return codes. In performance-critical applications, excessive use of exceptions can be detrimental.
Guidelines for Optimizing Exception Use
To mitigate the performance impacts:
- Limit exceptions to situations that truly represent exceptional conditions.
- Use exceptions for recoverable errors, while using alternative mechanisms for predictable errors.
- Use `noexcept` where applicable to optimize the performance when you are sure that a certain function will not throw exceptions.
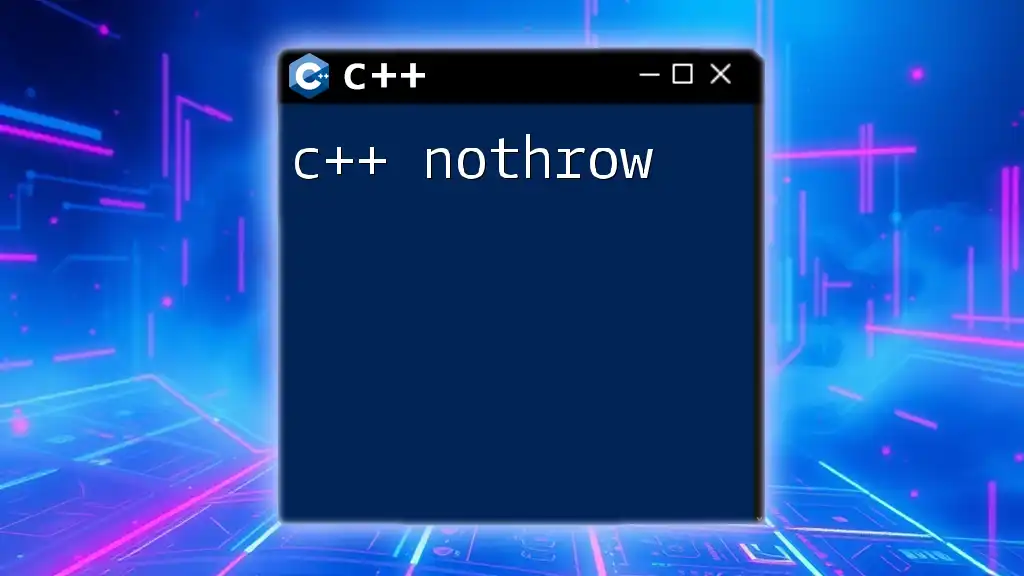
Conclusion
Understanding how to effectively use the `throw` keyword in C++ is essential for robust error management. By employing exceptions, developers can create more resilient applications that handle errors gracefully, enhance code clarity, and promote better separation between normal and exceptional code flows. As you practice with `throw`, embrace both the power and responsibility that come with it, ensuring your error handling practices lead to maintainable and efficient code.
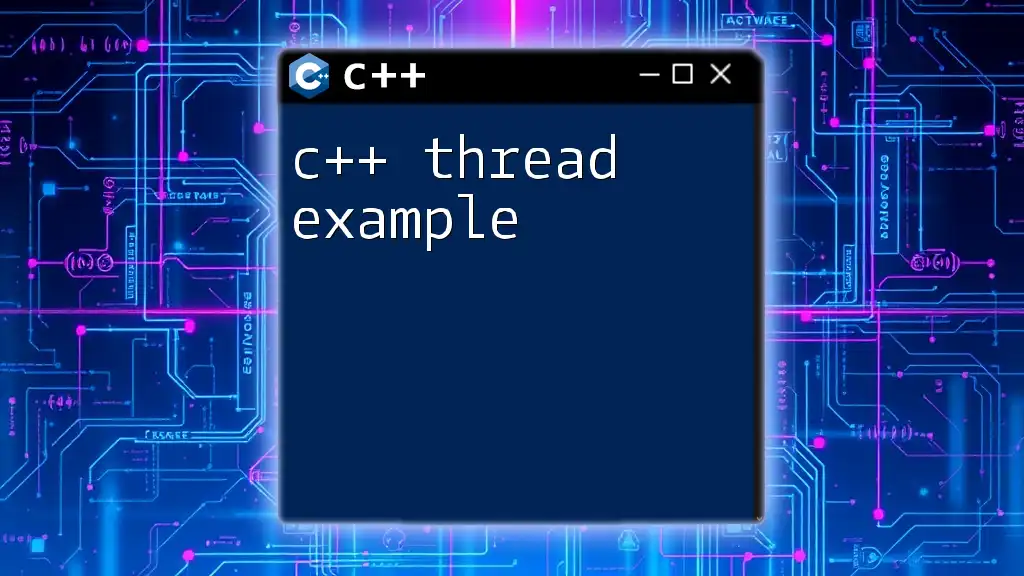
Additional Resources
For a deeper dive into C++ exception handling, consider exploring the following resources:
- C++ Standard Library Documentation
- Books focused on C++ programming and best practices
- Online tutorials showcasing real-world implementations of exception handling