In C++, options for manipulating command-line arguments are facilitated through the `argc` and `argv` parameters in the `main()` function, allowing programs to receive input when executed.
Here's a code snippet demonstrating how to handle command-line options:
#include <iostream>
int main(int argc, char* argv[]) {
for (int i = 1; i < argc; ++i) {
std::cout << "Option " << i << ": " << argv[i] << std::endl;
}
return 0;
}
Understanding Command-Line Options
What are Command-Line Options?
C++ options refer to specific command-line arguments that allow users to modify the behavior or output of a program without changing its source code. These options enable the implementation of flexible functionality and provide a user-friendly interface for programs.
Types of Command-Line Options
C++ options typically fall into two categories:
- Short Options: These are single-letter flags preceded by a single hyphen, such as `-h` for help or `-v` for version.
- Long Options: These are more descriptive and are preceded by two hyphens, like `--help` or `--version`. They enhance readability and make the command-line interface more intuitive.
Implementing Command-Line Options in C++
To handle command-line options in C++, programmers frequently utilize the `argc` and `argv` parameters passed to the `main()` function.
- `argc` is the argument count, indicating how many command-line arguments were provided.
- `argv` is an array of C-style strings (character pointers) representing each argument.
Example: Basic Command-Line Option Parsing
Here is a simple program that prints the command-line arguments it receives:
int main(int argc, char* argv[]) {
for (int i = 0; i < argc; ++i) {
std::cout << argv[i] << std::endl;
}
return 0;
}
In this example, the program loops through `argv`, outputting each argument to the console. This basic structure provides a foundation for option parsing.
Example of Recognizing Basic Options
Let's create a simple C++ program that recognizes `-h` (help option) and `--version`:
#include <iostream>
#include <cstring>
int main(int argc, char* argv[]) {
for (int i = 1; i < argc; ++i) {
if (strcmp(argv[i], "-h") == 0 || strcmp(argv[i], "--help") == 0) {
std::cout << "Usage: my_program [options]" << std::endl;
return 0;
} else if (strcmp(argv[i], "--version") == 0) {
std::cout << "My Program Version 1.0" << std::endl;
return 0;
}
}
std::cout << "No options provided." << std::endl;
return 0;
}
In this code, the program checks the provided options and responds appropriately, demonstrating how to handle user input effectively.
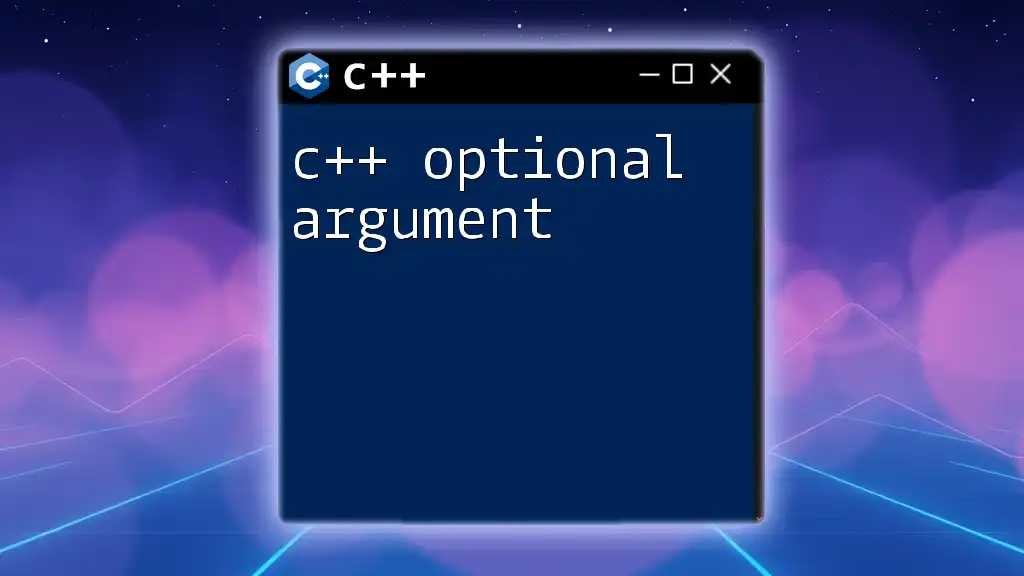
Using C++ Libraries for Option Parsing
Why Use Libraries?
Using libraries for parsing command-line options is beneficial as it helps streamline the implementation, reduces boilerplate code, and enhances readability, making it easier for developers to focus on core functionalities.
Popular Libraries for Command-Line Options
Boost.ProgramOptions
One of the most popular libraries for handling command-line options in C++ is `Boost.ProgramOptions`. This library simplifies the process of defining and parsing options.
Basic Parsing Using Boost
Here’s how to implement a simple command-line option parser with `Boost.ProgramOptions`:
#include <boost/program_options.hpp>
#include <iostream>
namespace po = boost::program_options;
int main(int argc, char* argv[]) {
po::options_description desc("Allowed options");
desc.add_options()
("help,h", "produce help message")
("version,v", "show version information");
po::variables_map vm;
po::store(po::parse_command_line(argc, argv, desc), vm);
po::notify(vm);
if (vm.count("help")) {
std::cout << desc << std::endl;
}
return 0;
}
In this example, the program can parse both help and version options efficiently using Boost.
cxxopts
Another contemporary and lightweight library for command-line options is `cxxopts`. It is straightforward and user-friendly.
Simple Implementation Example
Here’s how to implement option handling in C++ using the `cxxopts` library:
#include <cxxopts.hpp>
#include <iostream>
int main(int argc, char* argv[]) {
cxxopts::Options options("MyProgram", "Description");
options.add_options()
("h,help", "Show help")
("v,version", "Show version");
auto result = options.parse(argc, argv);
if (result.count("help")) {
std::cout << options.help() << std::endl;
}
return 0;
}
This code illustrates the simplicity of defining options using `cxxopts`, making it a great choice for modern C++ applications.
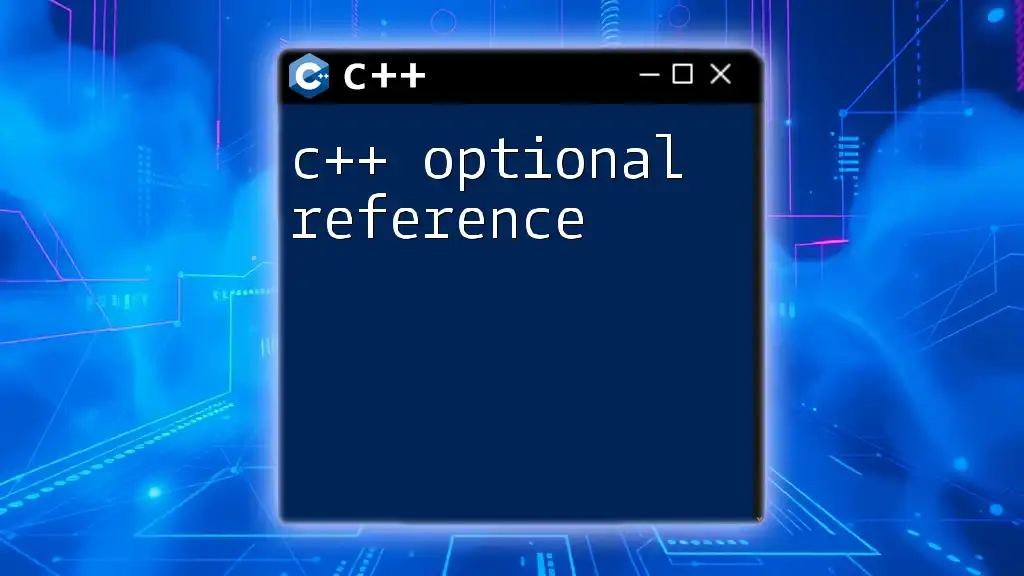
Advanced Option Handling
Custom Option Types
Defining custom option types allows developers to create more complex command-line interfaces. For example, if your program needs to accept a file path, you can define a custom type and validate it properly.
Handling Errors and Exceptions
Robust error handling is crucial when working with command-line options. Implementing effective error handling lets users know when they make mistakes in their inputs.
Consider this error handling example that uses try-catch blocks to manage exceptions during option parsing:
try {
// Parse options here
} catch (const std::exception& e) {
std::cerr << "Error while parsing options: " << e.what() << std::endl;
}
Here, the program catches any exceptions thrown during the parsing process, providing feedback to the user regarding what went wrong.
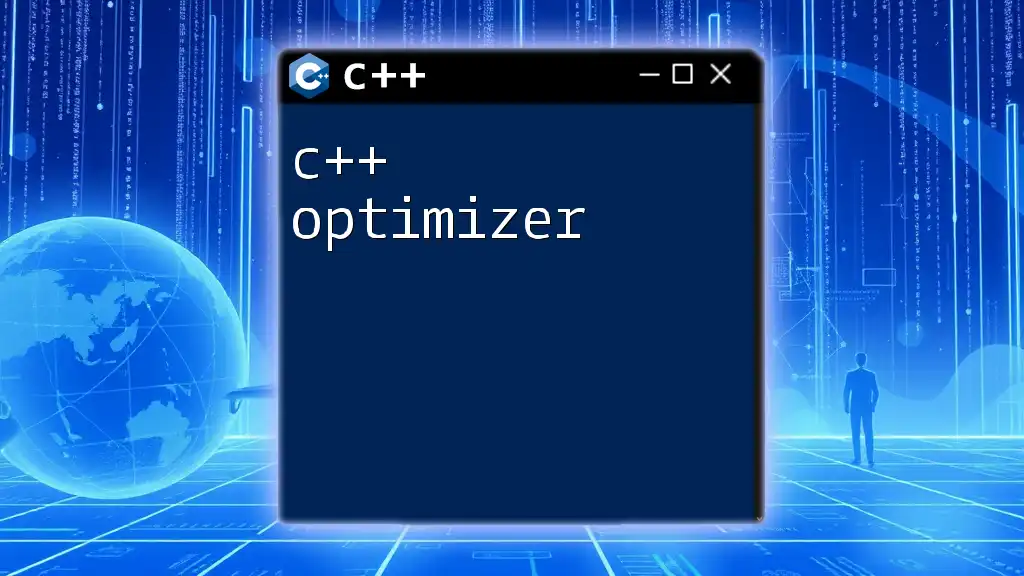
Real-World Applications of C++ Options
Use Cases in Industry
C++ options find widespread use in various industries. Many command-line tools, such as Git and Make, utilize options to enhance their usability. These tools allow users to execute complex operations with simple commands.
Examples of C++ Programs Using Options
Studying existing open-source projects can offer insights into how veteran developers structure their options in C++. This can inspire best practices when implementing your own options in projects.
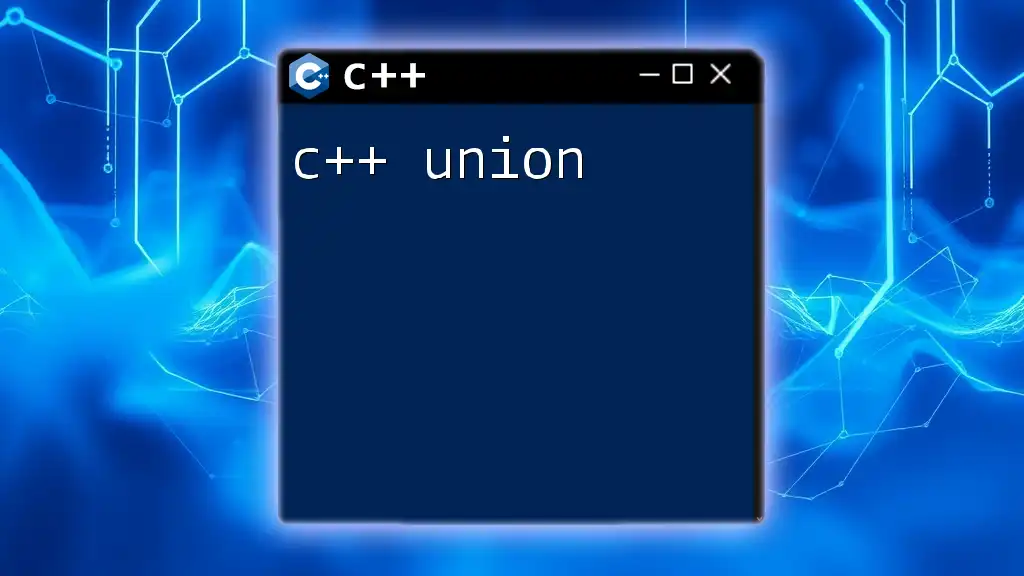
Best Practices for Working with Options in C++
Consistency in Option Naming
It is vital to maintain consistency in naming options across your command-line tools. Opt for either short flags or long options, but do so uniformly throughout your codebase.
User-Friendly Help Messages
Creating comprehensive help outputs is essential for user interaction. Providing a clear explanation of each option makes it easier for users to understand how to use your program effectively.
Testing and Debugging Command-Line Options
When working with options in C++, imagine how the end user will interact with your application. Testing various input scenarios and debugging potential issues is imperative to delivering a stable program. Ensure to test edge cases and invalid input formats as well.
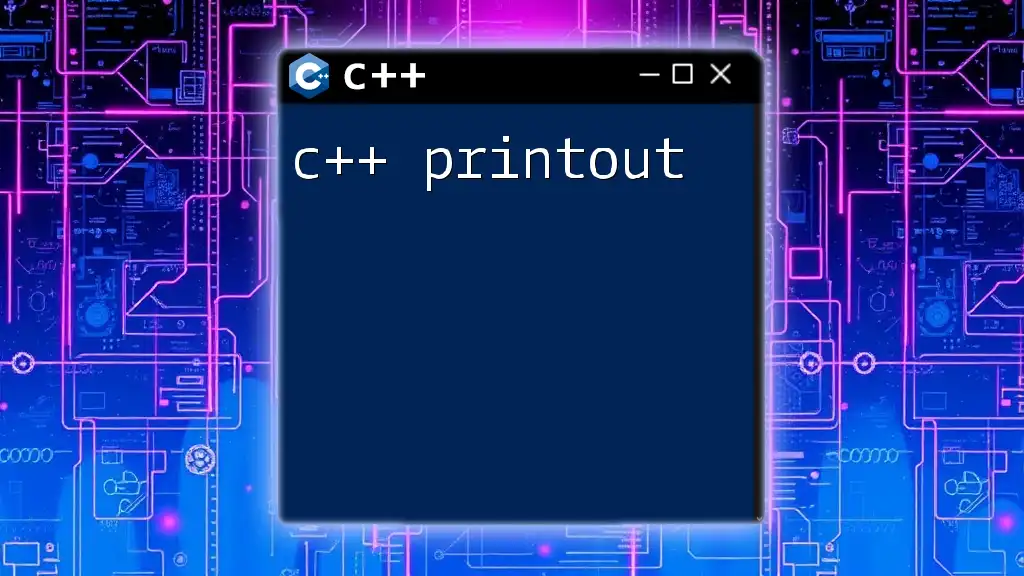
Conclusion
In summary, understanding and implementing C++ options can significantly enhance user experience and flexibility when developing command-line applications. With libraries like Boost.ProgramOptions and cxxopts, managing these options becomes straightforward and efficient.
As you implement C++ options in your own projects, remember to strive for readability, consistency, and user-friendliness. Don’t hesitate to experiment and expand your knowledge in this crucial area of C++ programming!
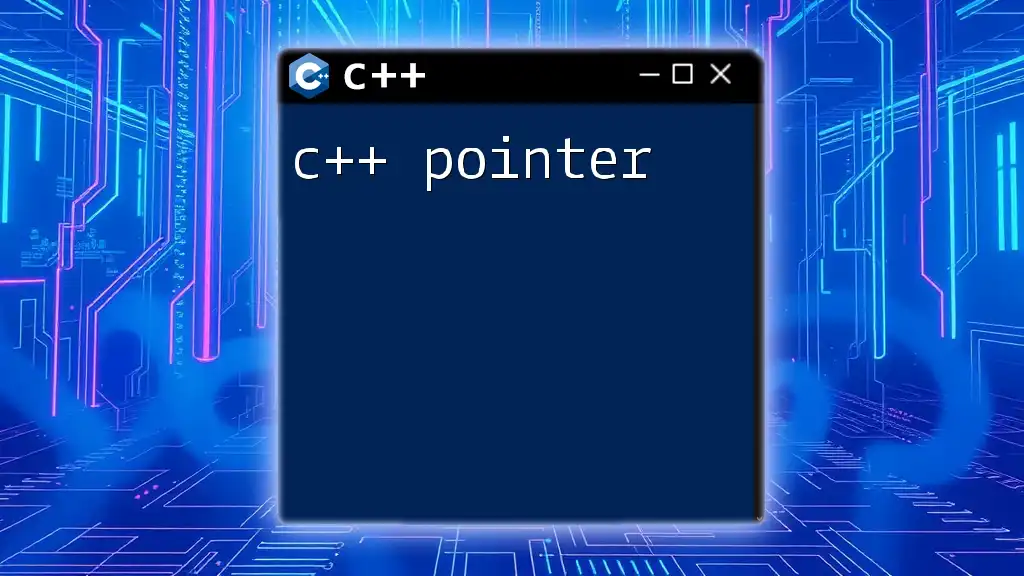
Further Reading and Resources
To deepen your understanding of C++ options and their implementations, refer to the official documentation for Boost.ProgramOptions and cxxopts. These resources provide in-depth information and examples to aid your learning journey. Additionally, exploring tutorials, forums, and courses can help solidify your command-line interface skills.
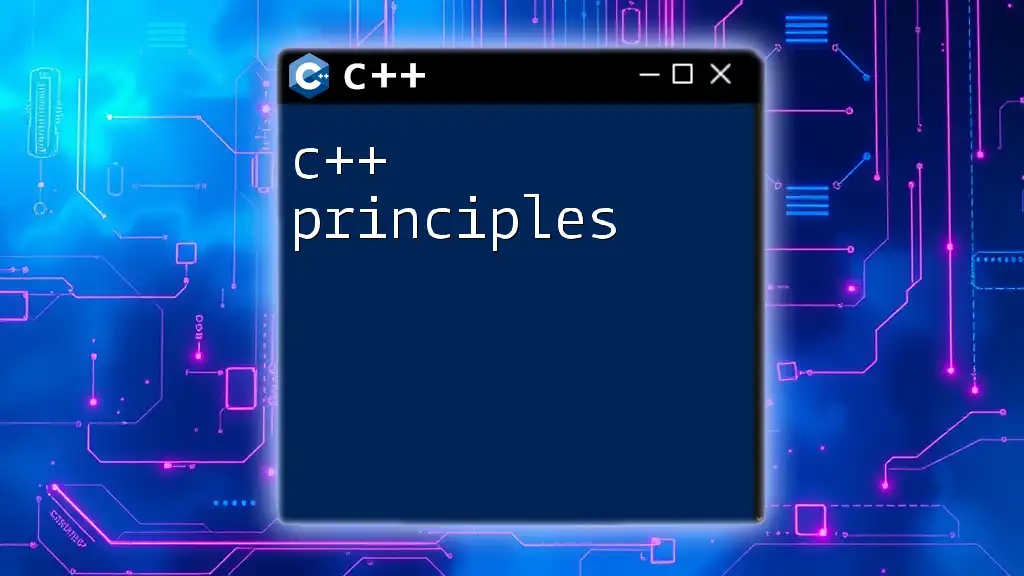
Call-to-Action
We encourage you to share your experiences with handling C++ options in the comments section. Ask questions or provide feedback based on your journey, and let’s enhance our understanding together!