The `sprintf` function in C++ is used to format and store a string in a character array, allowing for formatted output without printing to the console.
#include <cstdio>
int main() {
char buffer[50];
int age = 25;
sprintf(buffer, "I am %d years old.", age);
// buffer now contains "I am 25 years old."
return 0;
}
What is `sprintf`?
`C++ sprintf` is a powerful function used in C and C++ for formatted output to a string. It allows developers to compose strings by embedding variable data within fixed format multiple types of values into one larger string, making it an essential tool in a programmer's toolkit.
This function is particularly useful when you need to create strings that include numeric values, characters, or formatted data for display purposes in a console application or logging.
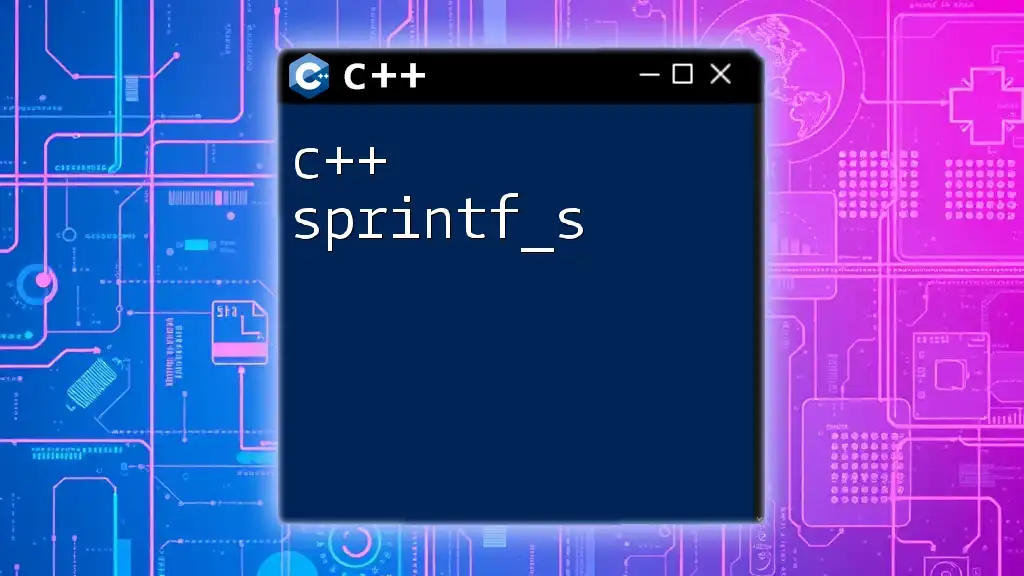
When to Use `sprintf`
You should consider using `sprintf` in situations where:
- You need to combine multiple data types into a single string.
- The data to output is known and will remain within the bounds of your string buffer.
- Precision in formatting and placement is required; for instance, when generating concise reports or logs.
It’s crucial to compare `sprintf` to other options such as `cout` or more modern C++ formatting options like `std::ostringstream`. While `cout` offers type safety, `sprintf` provides a legacy way to control string formatting directly.
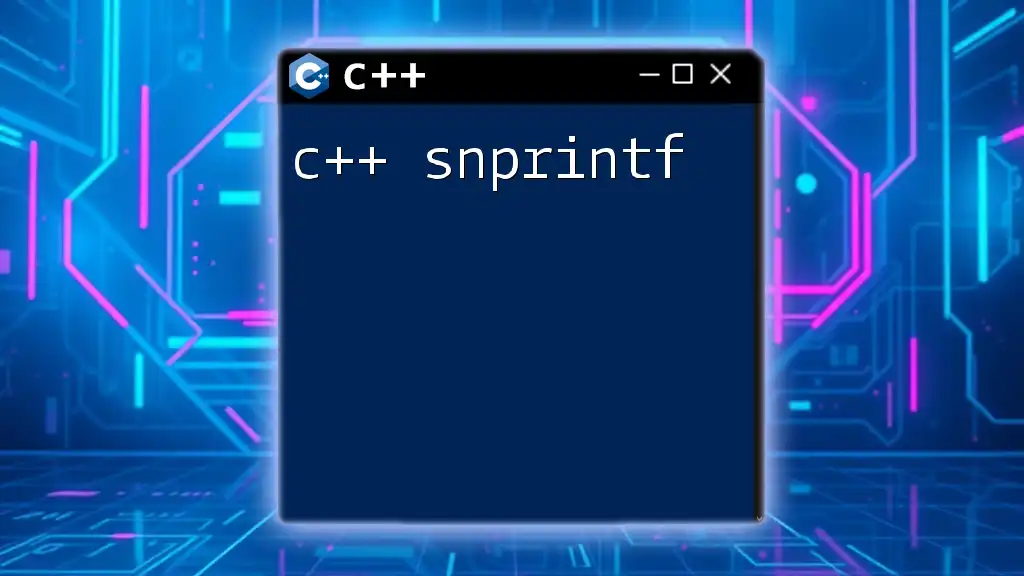
Understanding the Syntax of `sprintf`
Basic Syntax Breakdown
The basic syntax of `sprintf` is:
int sprintf(char *str, const char *format, ...);
- `char *str`: This parameter points to a buffer where the generated string will be stored. It is essential to ensure that this buffer is large enough to accommodate the formatted string to avoid overflow.
- `const char *format`: This string specifies how subsequent arguments (if any) are converted for output.
- `...`: This is a variable list of arguments that are formatted according to the format specifiers in the format string.
Detailed Explanation of Parameters
-
Destination Buffer: The buffer variable is where `sprintf` writes the final formatted string. Care must be taken to allocate sufficient memory for the buffer to store the result effectively. A common mistake is to underestimate the size needed, leading to buffer overflows, which can cause program crashes or unexpected behaviors.
-
Format String: A format string consists of plain text and format specifiers. Specifiers dictate how the corresponding arguments are formatted. For instance, `%d` will format an integer, `%f` for a float, while `%s` is used for strings.
-
Variable Arguments: The use of ellipsis (`...`) allows you to pass multiple arguments which are processed by the function based on the format string. This flexibility is potent but requires careful alignment of types to specifiers to avoid runtime errors.
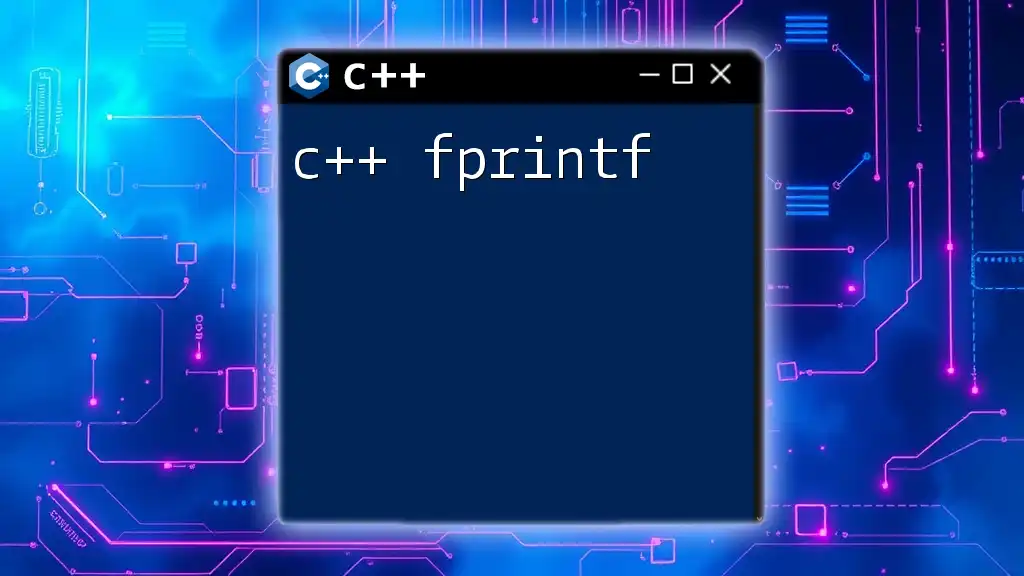
Format Specifiers in `sprintf`
Common Format Specifiers
-
Integer Formats: Use `%d` for signed integers and `%u` for unsigned integers. For example:
int age = 25; sprintf(buffer, "Age: %d", age); // Outputs: Age: 25
-
Floating Point Formats: `%f` is for normal floats, `%e` for scientific notation, and `%g` combines both formats intelligently to save space. Example:
double pi = 3.14159; sprintf(buffer, "Value of Pi: %.2f", pi); // Outputs: Value of Pi: 3.14
-
Character and String Formats: You can use `%c` for a single character and `%s` for strings:
char initial = 'A'; sprintf(buffer, "Initial: %c", initial); // Outputs: Initial: A
-
Pointer Format: The `%p` specifier is used to print pointer values, showing the memory address:
int *ptr = &age; sprintf(buffer, "Address of age: %p", (void*)ptr); // Outputs: Address of age: 0x7ffd5b351c4c
Width and Precision Modifiers
Width and precision in formatting allow for sophisticated control over the output. Width specifies the minimum number of characters to be printed; if the value is shorter, it pads with spaces:
sprintf(buffer, "Number: %5d", 10); // Outputs: Number: 10
Precision can be applied to floating point numbers:
sprintf(buffer, "Float: %.3f", 3.1); // Outputs: Float: 3.100
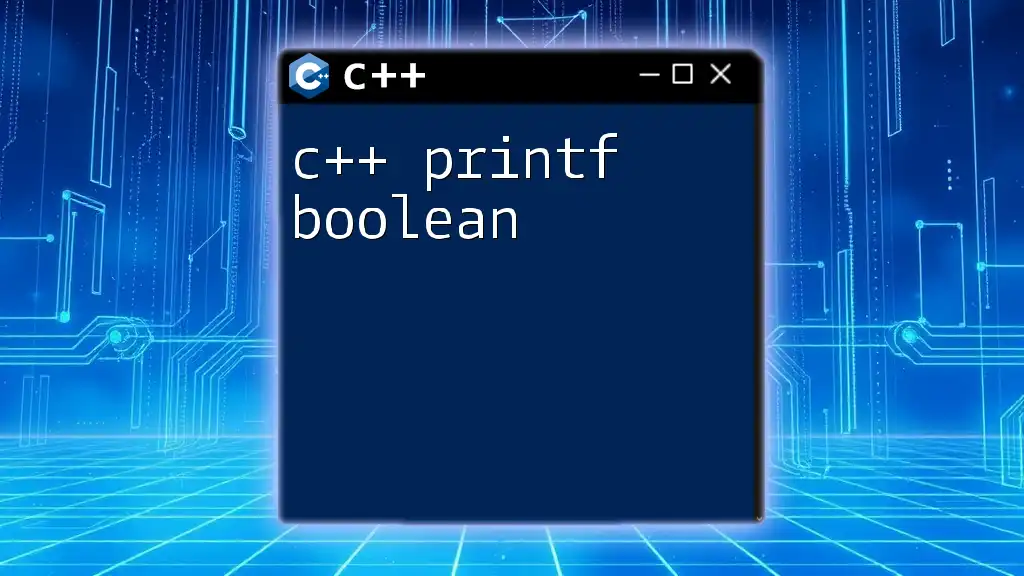
Implementing `sprintf` in C++
Basic Example
Here’s a simple implementation of `sprintf`:
#include <cstdio> // Include cstdio for sprintf
#include <iostream>
int main() {
char buffer[100];
int age = 25;
sprintf(buffer, "I am %d years old", age);
std::cout << buffer << std::endl; // Outputs: I am 25 years old
return 0;
}
In this example, `sprintf` formats a string containing the age variable and stores it in the buffer, which is then printed to the console.
Advanced Example with Multiple Variables
For more complex scenarios, `sprintf` can handle multiple variables efficiently:
#include <cstdio>
#include <iostream>
int main() {
char buffer[200];
const char* name = "Alice";
double score = 95.6;
sprintf(buffer, "Student: %s, Score: %.2f", name, score);
std::cout << buffer << std::endl; // Outputs: Student: Alice, Score: 95.60
return 0;
}
In this case, multiple parameters are formatted into a single string, showcasing the ability of `sprintf` to manage various data types seamlessly.
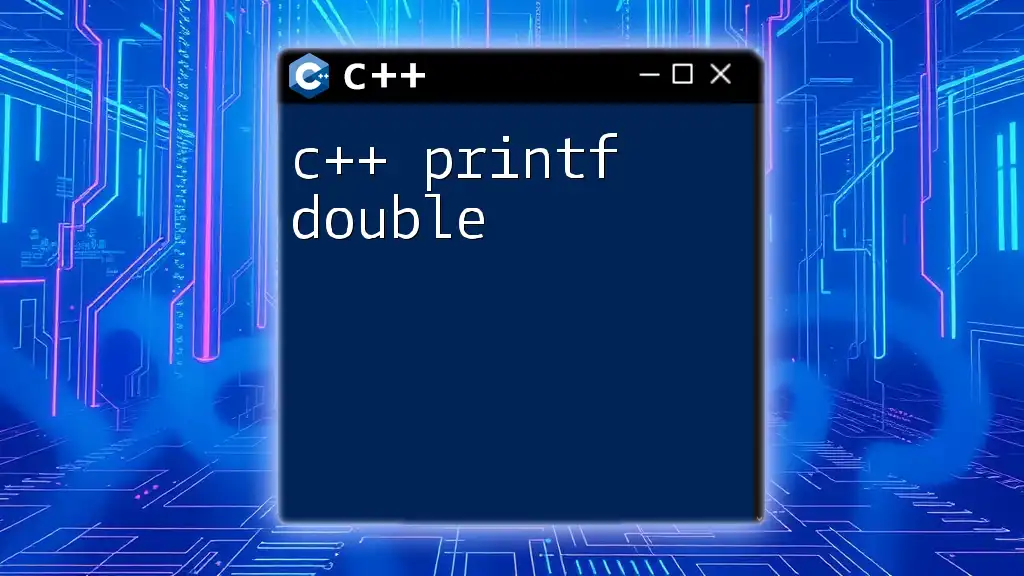
Common Mistakes When Using `sprintf`
Buffer Overflow Issues
One of the most significant risks when using `sprintf` is buffer overflow. Since `sprintf` does not check the size of the destination buffer, if the data exceeds the allocated space, it can lead to unpredictable results or program crashes. Proper buffer management is crucial.
To prevent buffer overflow, ensure that you always allocate enough space for the expected output. Utilizing functions like `snprintf`, which accept the buffer size, is also advisable.
Formatting Errors
Another common pitfall is formatting errors, which occur when the number of format specifiers does not match the number of supplied arguments, or when arguments are of the wrong type. This can lead to undefined behavior or wrong outputs. Always verify that the types of arguments passed correspond to the format strings.
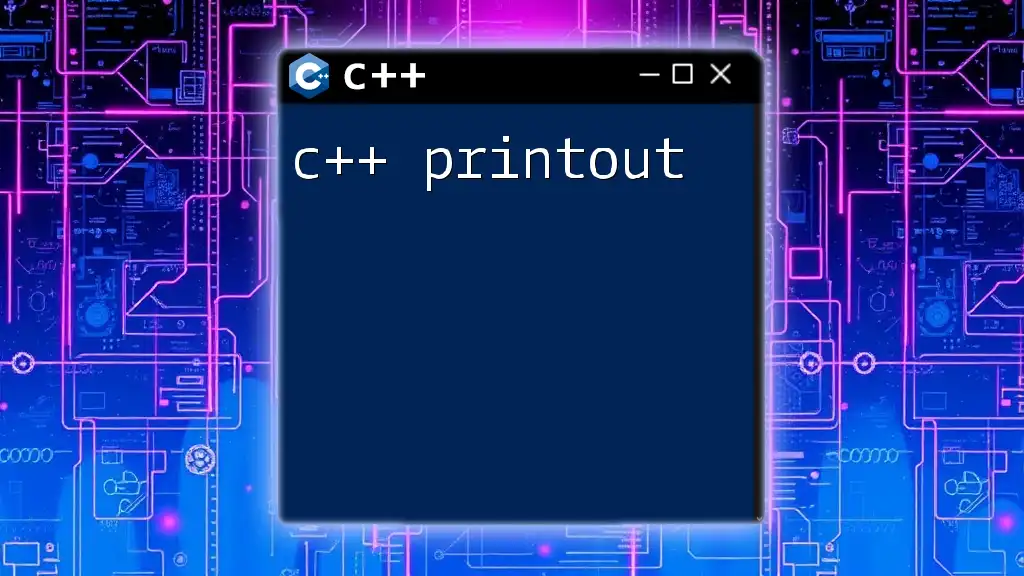
Alternative: `snprintf` as a Safeguard
Why Choose `snprintf`?
`snprintf` is a safer alternative to `sprintf` because it includes a parameter for the maximum number of characters to write to the buffer. This helps prevent buffer overflow by ensuring that the function does not exceed the allocated space, adding a layer of safety in string handling.
Using `snprintf` with Examples
Below is an example that demonstrates how to use `snprintf` effectively:
#include <cstdio>
#include <iostream>
int main() {
char buffer[50];
int num = 100;
snprintf(buffer, sizeof(buffer), "Formatted number: %d", num);
std::cout << buffer << std::endl; // Outputs: Formatted number: 100
return 0;
}
In this snippet, `snprintf` formats the integer into the buffer and ensures it does not exceed the specified buffer size.
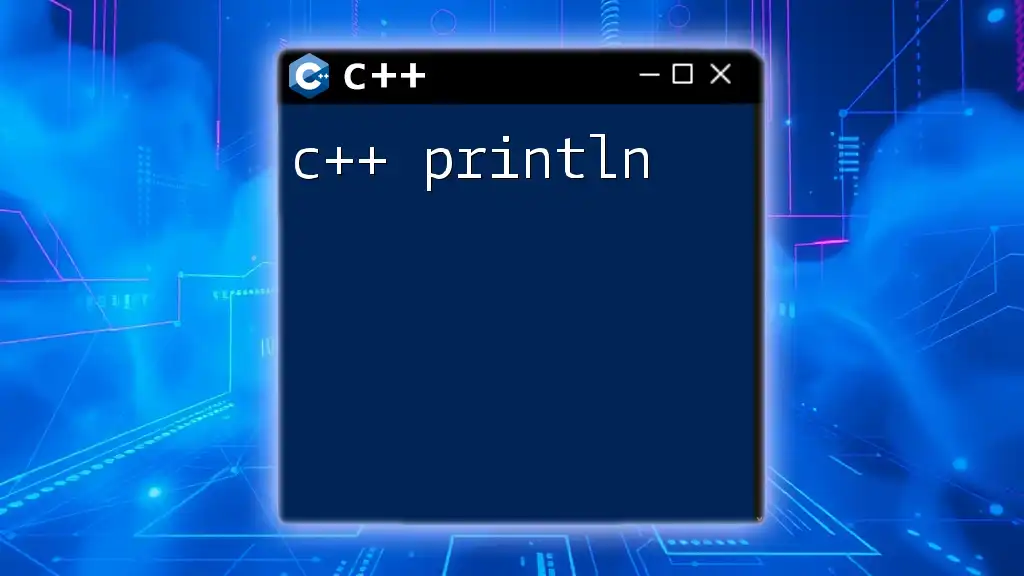
Conclusion
Summary of Key Points
In summary, `c++ sprintf` is a versatile function that allows you to format strings flexibly and concisely. Understanding its syntax, parameters, and format specifiers is key to effectively using this function. While it is powerful, it comes with risks that can be mitigated by turning to alternatives like `snprintf`.
Encourage Further Learning
To deepen your understanding of C++ and string formatting, consider exploring more resources and tutorials. Engaging with documentation and community forums can significantly enhance your grasp of various commands and their applications in C++.