The `sprintf_s` function in C++ is a safer version of `sprintf`, designed to format a string and prevent buffer overflows by requiring the size of the destination buffer.
#include <cstdio>
int main() {
char buffer[50];
sprintf_s(buffer, sizeof(buffer), "The value of pi is approximately %.2f", 3.14);
printf("%s\n", buffer);
return 0;
}
Overview of sprintf_s
`sprintf_s` is a function in C++ used for formatting strings. It serves a similar purpose to the traditional `sprintf` function but offers enhanced security by preventing buffer overflows, a common vulnerability in C and C++ programming. This makes `sprintf_s` a preferred choice in scenarios where input safety is paramount.
The importance of secure string manipulation cannot be overstated, especially in an era where data breaches can cause significant damage. By using `sprintf_s`, developers can reduce the risk associated with improperly sized buffers—a frequent source of security flaws in applications.
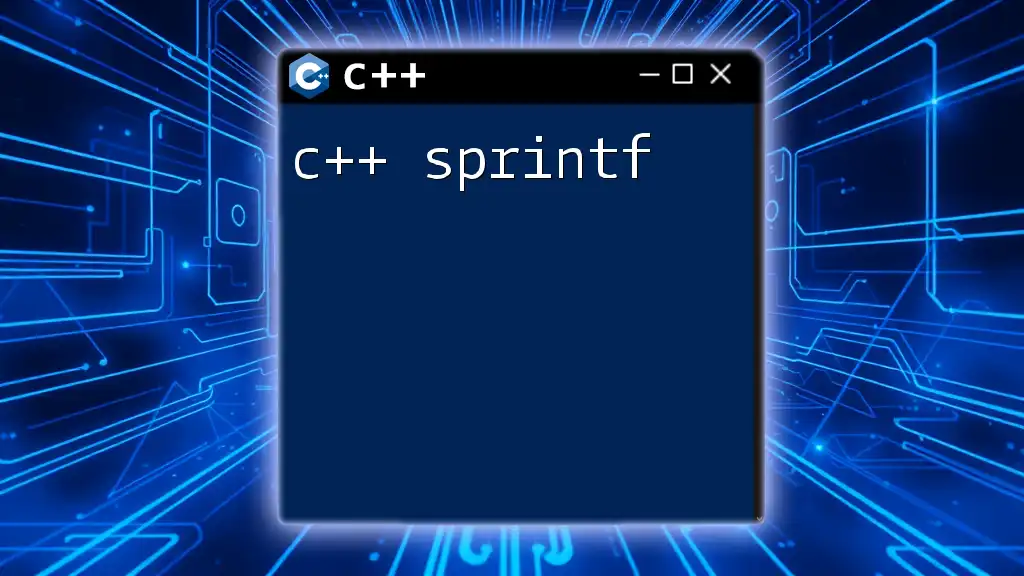
Understanding the Syntax of sprintf_s
Basic Syntax of sprintf_s
The syntax of the `sprintf_s` function can be expressed as follows:
int sprintf_s(char *buffer, size_t sizeOfBuffer, const char *format, ...);
Let's delve into each component of this syntax:
- buffer: This is the destination where the formatted string will be stored. It must be a valid character array with sufficient size to hold the resulting string.
- sizeOfBuffer: This parameter indicates the total size of the destination buffer in bytes. It is crucial for ensuring that `sprintf_s` does not write beyond the bounds of the array.
- format: The format specifier string that guides how the subsequent arguments (if any) are formatted and inserted into the buffer. This can include various placeholders for different data types.
Using Format Specifiers
The format specifier plays a vital role in determining how the output will be structured within the buffer. Below are some of the most common specifiers:
- `%d`: Integer format
- `%f`: Floating-point format
- `%s`: String format
For instance, using `%d` allows you to represent integers in your output:
int a = 42;
sprintf_s(buffer, sizeof(buffer), "The answer is: %d", a);
Likewise, the `%f` specifier is used for floating-point numbers:
float pi = 3.14159;
sprintf_s(buffer, sizeof(buffer), "Value of Pi: %f", pi);
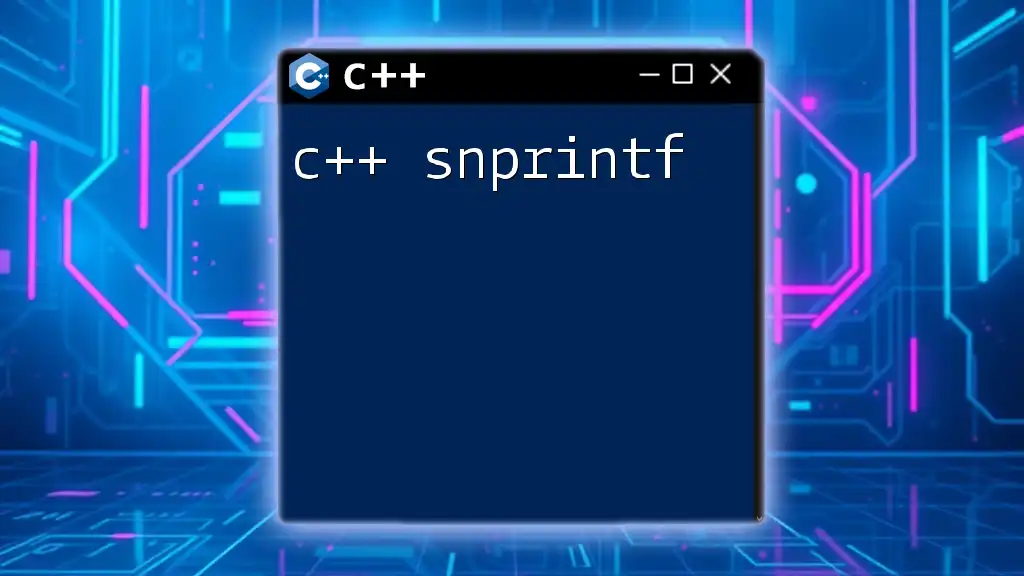
Practical Examples of Using sprintf_s
Basic Example of sprintf_s
One of the simplest applications of `sprintf_s` demonstrates its use in formatting a basic string:
#include <cstdio>
int main() {
char buffer[50];
int value = 100;
sprintf_s(buffer, sizeof(buffer), "Value: %d", value);
printf("%s\n", buffer);
return 0;
}
In this example, `sprintf_s` safely formats the integer value into the provided buffer, ensuring that it does not exceed its limit and is properly formatted as a string.
Advanced Example with Multiple Variables
Using multiple variables in `sprintf_s` is straightforward and enhances flexibility:
#include <cstdio>
int main() {
char buffer[100];
const char* name = "Alice";
int age = 30;
sprintf_s(buffer, sizeof(buffer), "%s is %d years old.", name, age);
printf("%s\n", buffer);
return 0;
}
This snippet showcases how the function can format both strings and integers into a coherent output. Formatting multiple variables in a single call is a hallmark of `sprintf_s`, making it exceptionally versatile for diverse applications.
Example Handling Edge Cases
One of the main benefits of using `sprintf_s` is its built-in protection against buffer overflows:
#include <cstdio>
#include <iostream>
int main() {
char buffer[10];
const char* longString = "This is too long";
sprintf_s(buffer, sizeof(buffer), "%s", longString); // Will truncate
printf("%s\n", buffer); // Only prints "This is "
return 0;
}
In this case, the `longString` exceeds the size of the `buffer`. Instead of causing a buffer overflow, `sprintf_s` truncates the output, ensuring that only the allowed amount is written. This safety feature is crucial in application development.
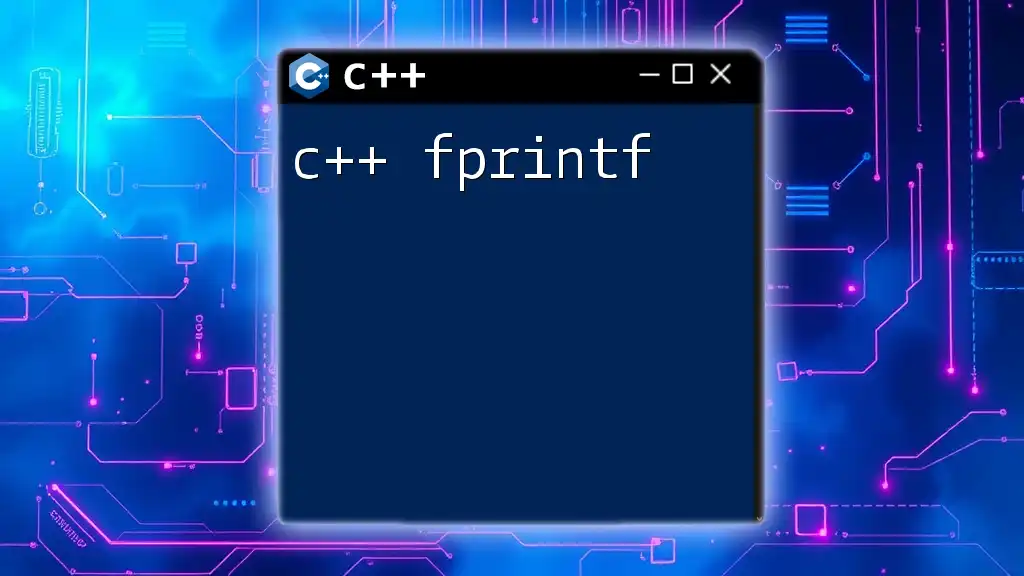
Error Handling with sprintf_s
Return Value of sprintf_s
When using `sprintf_s`, it is vital to check the return value. The function returns the number of characters printed excluding the null terminator. If it encounters an error, it usually returns a negative value indicating that something went wrong.
How to Handle Errors
Proper error handling is a best practice when using `sprintf_s`. You can implement an error check as follows:
#include <cstdio>
#include <cerrno>
int main() {
char buffer[10];
if (sprintf_s(buffer, sizeof(buffer), "Sample text") < 0) {
perror("Error occurred");
} else {
printf("%s\n", buffer);
}
return 0;
}
In this example, if an error occurs during formatting, the program reports the error using `perror()`. It is essential to handle errors gracefully in production code to maintain robustness and ensure user-friendliness.
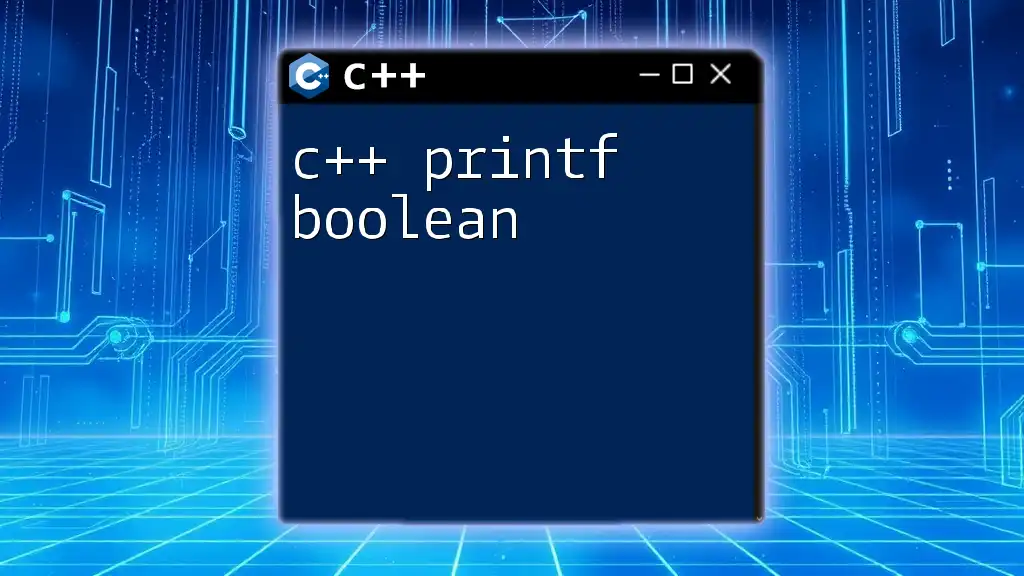
Performance Considerations
Performance of sprintf_s
When comparing `sprintf_s` to alternatives like `snprintf` or traditional `sprintf`, the focus should be on safety, not just performance. While `sprintf_s` may not always outperform its peers in terms of speed, its security features provide an essential benefit that should be prioritized, especially in safety-critical systems.
When to Use sprintf_s
Use `sprintf_s` in scenarios where:
- Input safety is a primary concern, such as handling user-provided data
- Maintaining readable and maintainable code is essential
- You require a function that automatically truncates output to fit buffer sizes
While using `sprintf_s`, be mindful of its limitations. For example, it is less flexible compared to some other string functions when dealing with dynamic or complex string operations.
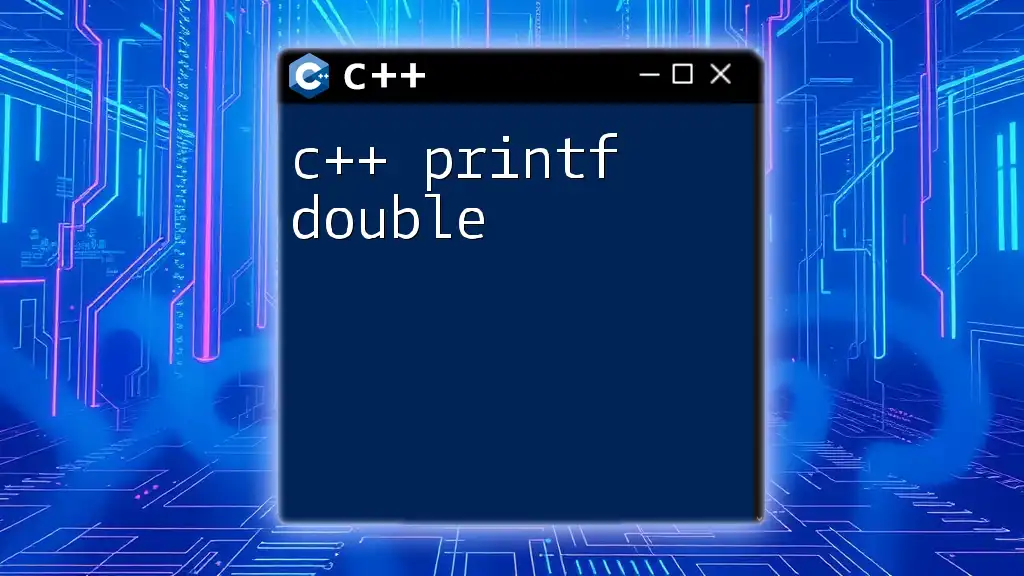
Conclusion
In summary, using `sprintf_s` in C++ is not just advantageous but essential for secure and efficient string manipulation. By employing `sprintf_s`, developers can significantly reduce the risk of buffer overflows while simplifying the process of formatting strings across various data types. Always ensure to check the return values and handle errors to maintain robust code. The adoption of safe programming practices like these ultimately leads to higher quality and more secure software.
For those looking to deepen their understanding of C++ and string handling, numerous resources are available online. Engaging with communities and documentation can provide further insights into best practices and advanced techniques in string management.