The `sscanf_s` function in C++ is a safer version of `sscanf`, used to read formatted data from a string into specified variables while ensuring buffer overflows are prevented.
#include <cstdio>
int main() {
const char* input = "42 3.14 Hello";
int intValue;
float floatValue;
char stringValue[10];
sscanf_s(input, "%d %f %s", &intValue, &floatValue, stringValue, (unsigned)_countof(stringValue));
return 0;
}
What is sscanf_s?
`sscanf_s` is a safer variant of the classic `sscanf` function used in C and C++ for performing formatted input. It is particularly significant when dealing with strings and user input, as it helps mitigate common security issues, especially buffer overflows. By requiring the size of the buffers as an additional argument, `sscanf_s` ensures that the program can avoid writing beyond allocated memory.

Why Use sscanf_s?
The primary reasons to choose `sscanf_s` over the traditional `sscanf` are:
- Security: `sscanf_s` provides enhanced input validation features, which help prevent vulnerabilities related to buffer overflow attacks.
- Robustness: It reduces the risk of undefined behavior that occurs when input exceeds expected buffer sizes, which is a common pitfall when handling user input.

Syntax of sscanf_s
Basic Syntax
The basic syntax of the `sscanf_s` function is as follows:
int sscanf_s(const char *buffer, const char *format, ...);
Parameters Explained
- buffer: This is the source string that contains the data you want to parse. It must be a null-terminated string.
- format: A string that specifies how to interpret the data in `buffer`. This includes format specifiers (e.g., `%d`, `%f`, `%s`).
- ...: Additional arguments that provide pointers to where the parsed data should be stored. For strings, you also need to specify the size of the buffer.
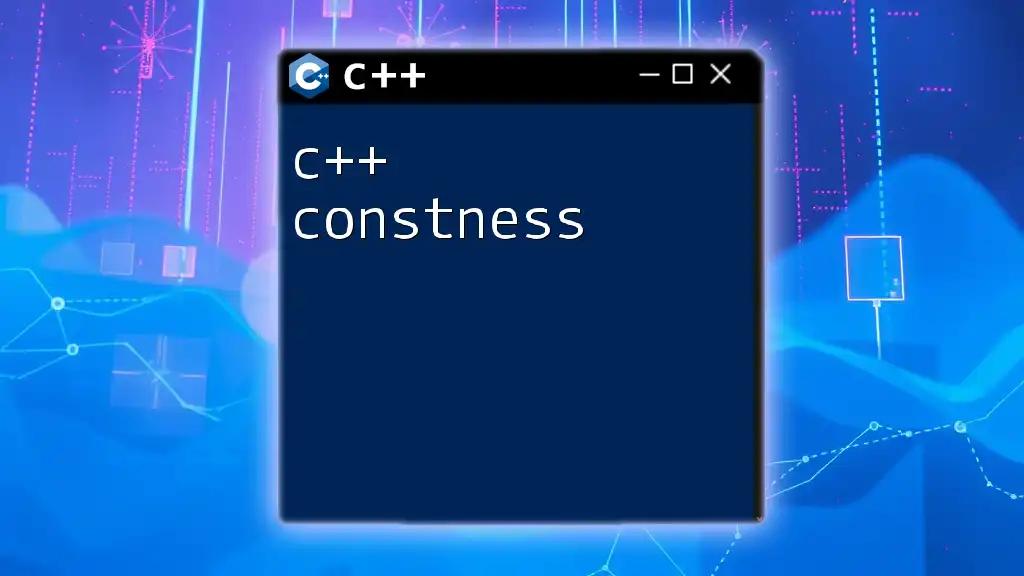
Implementing sscanf_s in C++
Setting Up Your Environment
Before using `sscanf_s`, you'll need to include the appropriate header:
#include <cstdio> // for sscanf_s
Basic Example
Here’s a simple example to illustrate how to use `sscanf_s` effectively:
const char *input = "Name: John Age: 30";
char name[50];
int age;
sscanf_s(input, "Name: %s Age: %d", name, sizeof(name), &age);
Explanation of Example
In this example, the input string contains both a name and an age. The `sscanf_s` function extracts the values based on the provided format string:
- `"Name: %s Age: %d"` indicates that you're looking for a string (`%s`) followed by an integer (`%d`).
- The size of the `name` buffer is explicitly passed to ensure safe operation. This extra parameter is essential since it helps `sscanf_s` limit how much data is read into the buffer.
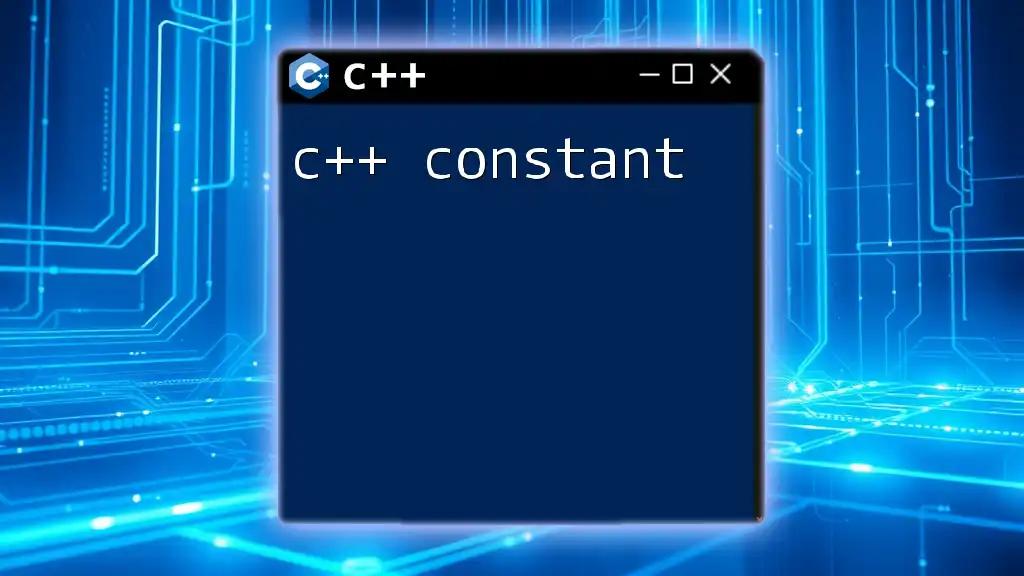
Advanced Use Cases of sscanf_s
Using Multiple Format Specifiers
You can extend the usage of `sscanf_s` to handle multiple data types. Here’s an example:
const char *input = "Product: Widget Price: 19.99 Quantity: 10";
char product[50];
float price;
int quantity;
sscanf_s(input, "Product: %s Price: %f Quantity: %d", product, sizeof(product), &price, &quantity);
Handling Strings Safely
Using `sscanf_s` requires careful handling of strings. Always ensure you define the correct buffer sizes to avoid memory issues. Always think about the potential for input that may exceed anticipated lengths, and make buffer sizes generous where necessary to accommodate unexpected input.
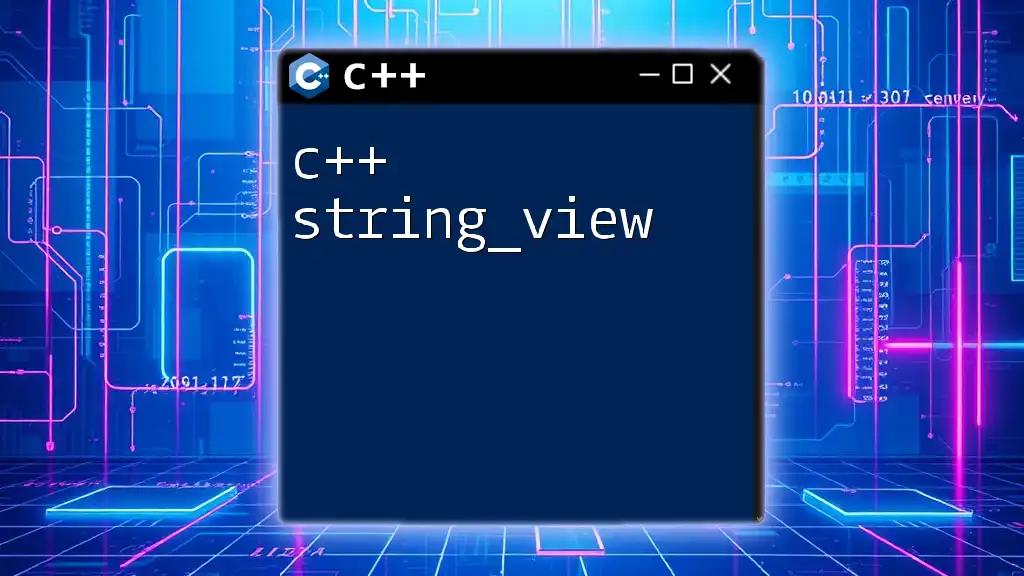
Error Handling with sscanf_s
Return Value Explained
The return value of `sscanf_s` is essential for error handling. It indicates how many input items were successfully assigned. For example:
int result = sscanf_s(input, "Name: %s Age: %d", name, sizeof(name), &age);
if (result < 2) {
// Handle error
}
If `result` is less than the expected number of assignments (2 in this case), your program should implement error recovery processes to address the incomplete parsing.
Common Input Errors
Debugging issues with `sscanf_s` can include:
- Incorrect format strings
- Input strings not corresponding to expected formats
- Insufficient buffer sizes leading to partial reads
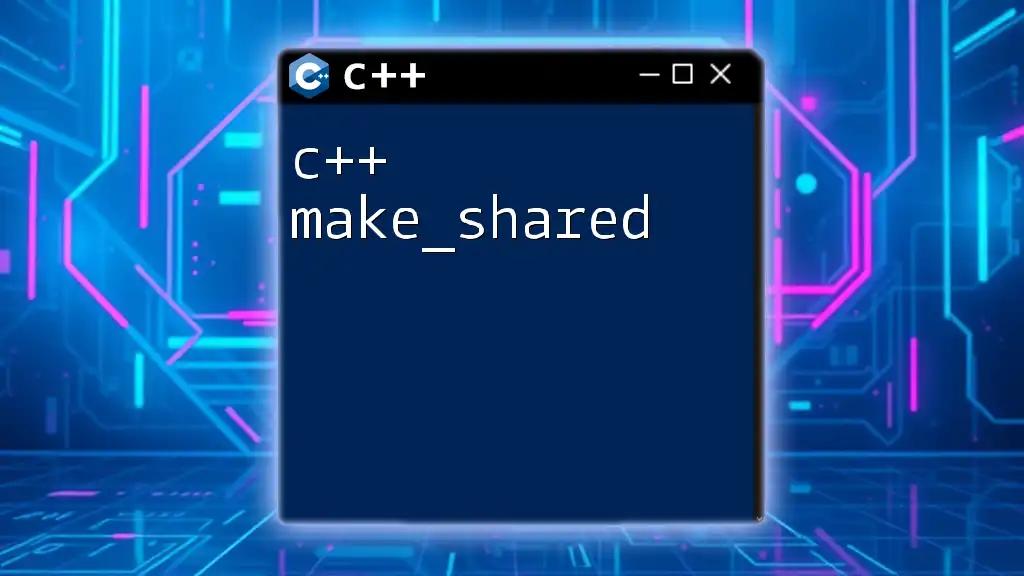
Best Practices for Using sscanf_s
Security Considerations
While `sscanf_s` already improves security, follow these practices to enhance your input handling:
- Always specify buffer sizes for strings to prevent overflows.
- Validate inputs after reading to ensure they conform to expected formats.
Performance Considerations
`sscanf_s` may introduce slight performance overhead compared to the standard `sscanf`. However, the trade-off is generally worth it when considering safety and robustness in your applications. Use profiling to ensure performance fits your application's needs.

Common Mistakes When Using sscanf_s
Incorrect Buffer Sizes
One common mistake is neglecting the size argument for strings. If you provide a buffer size that is shorter than the input, it can lead to undefined behavior. Be diligent in checking and setting proper sizes.
Using the Wrong Format Specifiers
Format specifier mismatches can lead to erroneous behavior, such as trying to store data in the wrong variable type or causing segmentation faults. Always ensure that format specifiers match the data types of the variables you're providing.
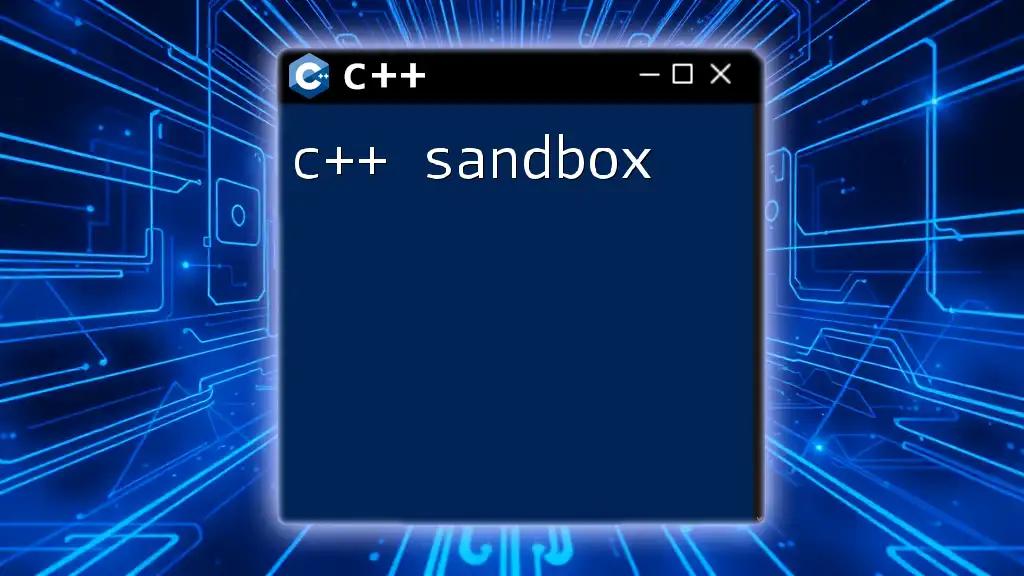
Conclusion
In summary, `sscanf_s` is a valuable function in C++ that ensures secure parsing of formatted input while preventing common pitfalls associated with buffer handling. By mastering its usage, you can significantly enhance the reliability and safety of your applications. We encourage you to put `sscanf_s` into practice in your coding projects and explore its capabilities in depth. Don't shy away from experimenting with different formats and inputs to fully appreciate its utility.
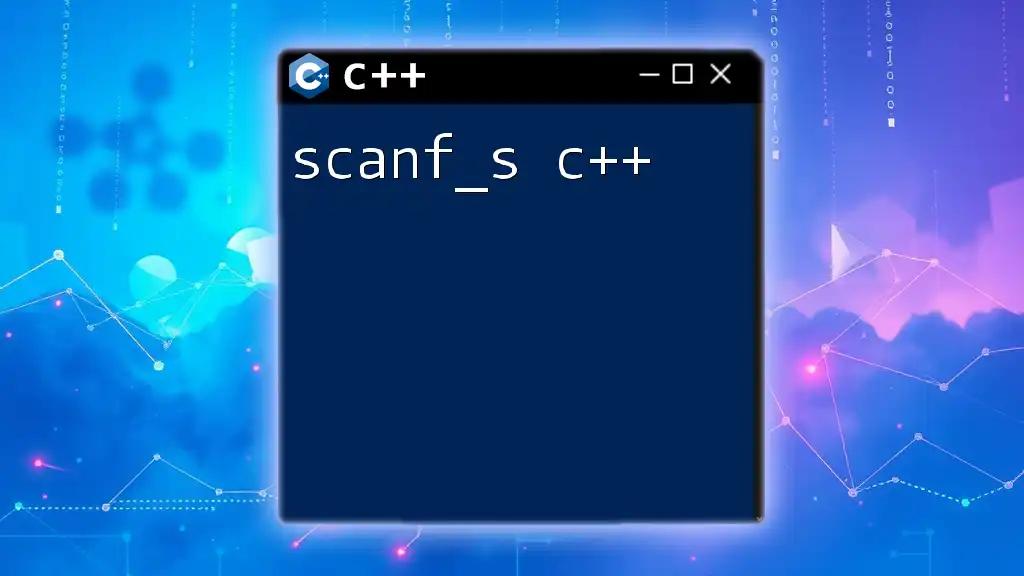
Additional Resources
For further reading, you can refer to the official documentation and additional tutorials available online. Engaging with community forums can also offer insights and help resolve any queries you might have as you delve deeper into using `sscanf_s` effectively.