The `strtok_s` function in C++ is a safer version of `strtok` that allows for tokenizing a string into smaller substrings, taking a context pointer to maintain state across successive calls.
Here's a code snippet to illustrate its usage:
#include <iostream>
#include <cstring>
int main() {
char str[] = "Hello,World,Example";
char* context = nullptr;
char* token = strtok_s(str, ",", &context);
while (token != nullptr) {
std::cout << token << std::endl;
token = strtok_s(nullptr, ",", &context);
}
return 0;
}
What is `strtok_s`?
`strtok_s` is a function introduced in the C11 standard that provides a safer alternative for tokenizing strings in C and C++. Unlike its predecessor `strtok`, `strtok_s` is designed to handle strings in a way that avoids potential security vulnerabilities, such as buffer overflows, by requiring an additional context parameter. This makes it a preferred choice when working with string tokenization in modern C++ programming.

The Need for Safe String Manipulation
String manipulation is a crucial aspect of programming, but it can also lead to significant security risks if not handled properly. Traditional functions like `strtok` manipulate strings without any safety checks, exposing developers to common issues such as buffer overflows. Such vulnerabilities could potentially lead to data corruption, crashes, or even exploitation of the program by malicious actors. `strtok_s` addresses these shortcomings by enforcing safer practices, ensuring developers can tokenize strings without the risk of unexpected behavior.
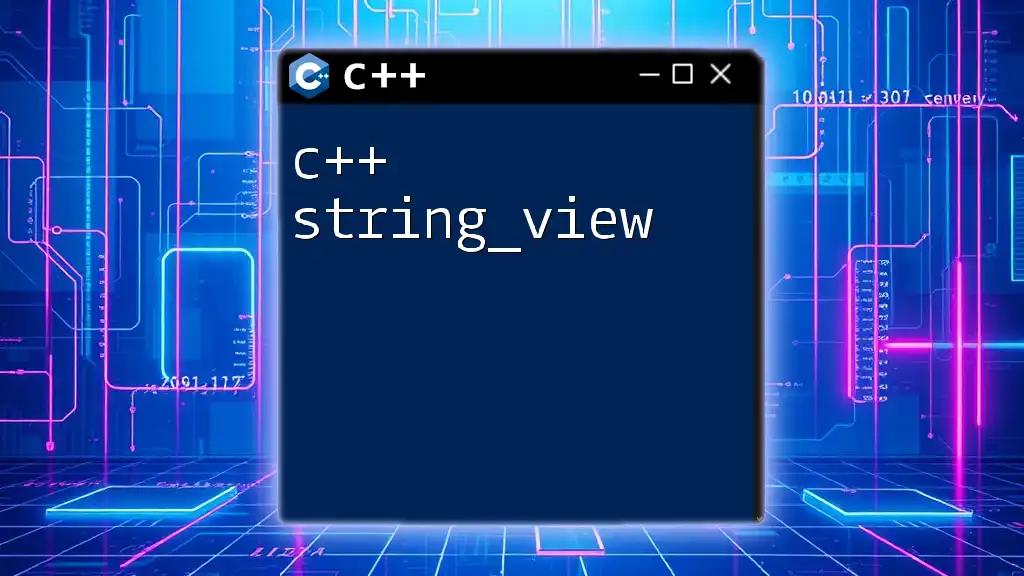
Understanding Tokenization
What is Tokenization?
Tokenization is the process of splitting a string into smaller components called tokens. This is often crucial for parsing data, reading configuration files, or processing user input. For example, when reading commands from a user, you might want to split the input based on spaces or other delimiters. By tokenizing a string, you can extract meaningful information in a structured way.
How Tokenization Works in C++
In C++, tokenization typically involves identifying a set of delimiters—characters or sequences that define boundaries between tokens. For instance, in the sentence "apple,banana;cherry", both commas and semicolons can serve as delimiters. A tokenization function analyzes the string and extracts substrings between these delimiters, allowing developers to work with discrete pieces of text.
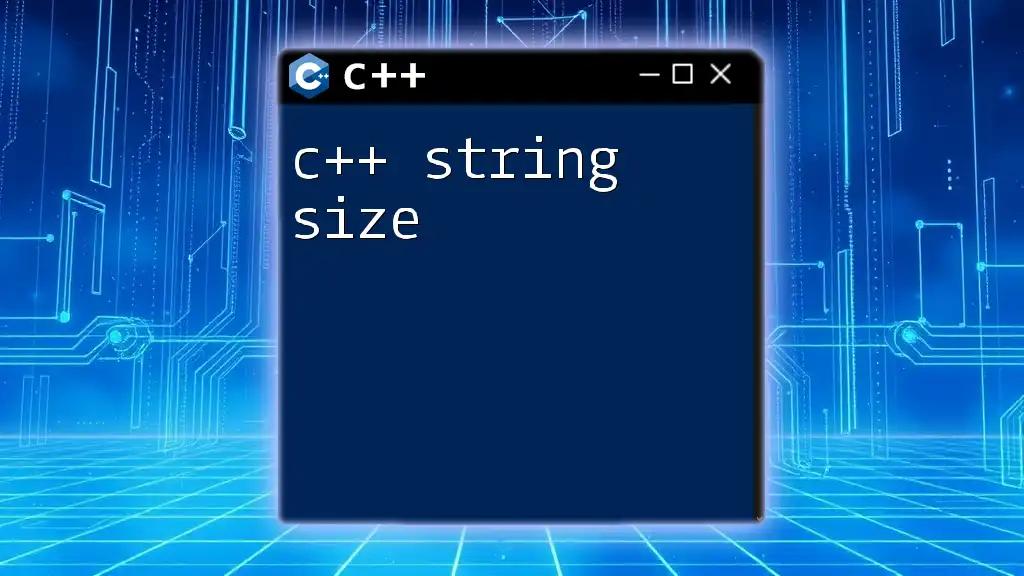
`strtok_s`: The Safe Alternative
Overview of `strtok_s` Function
The syntax of `strtok_s` can be summarized as follows:
char* strtok_s(char* str, const char* delimiters, char** context);
-
Parameters:
- `str`: The string to be tokenized. If it is NULL, `strtok_s` continues tokenization of the previous string.
- `delimiters`: A string containing all delimiter characters.
- `context`: A pointer to a `char*` that maintains context between successive calls.
-
Return Value: The function returns a pointer to the next token found in the string, or NULL if no further tokens exist.
Safety Features of `strtok_s`
The main advantage of `strtok_s` over `strtok` lies in its safety features. The context parameter not only allows for recursion without losing state but also prevents unintended overwrites by maintaining state information about which part of the string is being processed. This significantly reduces the risk of buffer overflow vulnerabilities and makes `strtok_s` a safer choice in real-world applications.

Using `strtok_s` in Your Code
Including Necessary Headers
To work with `strtok_s`, ensure that you include the necessary headers required for your string manipulation:
#include <iostream>
#include <cstdio>
Basic Example of Using `strtok_s`
Here’s a simple example demonstrating the usage of `strtok_s` to tokenize a string based on spaces:
#include <iostream>
#include <cstdio>
int main() {
char str[] = "Hello, World, Welcome";
char* context;
char* token = strtok_s(str, " ", &context);
while (token) {
std::cout << token << std::endl;
token = strtok_s(nullptr, " ", &context);
}
return 0;
}
In this code, we initialize a string containing multiple words separated by spaces. Using `strtok_s`, we extract individual tokens in a loop until no more tokens are found. This approach efficiently handles string delimiters and retrieves all portions of the string.
Advanced Example with Multiple Delimiters
Let's summarize how to use `strtok_s` with multiple delimiters effectively:
#include <iostream>
#include <cstdio>
int main() {
char str[] = "Apple;Banana,Cherry-Orange";
char* context;
char* token = strtok_s(str, ";,-", &context);
while (token) {
std::cout << token << std::endl;
token = strtok_s(nullptr, ";,-", &context);
}
return 0;
}
This advanced example uses various delimiters—semicolons, commas, and hyphens—to split the string into tokens. This versatility allows developers to specify multiple delimiters in a single function call, making it easier to extract tokens from strings that do not adhere to a strict delimiter convention.
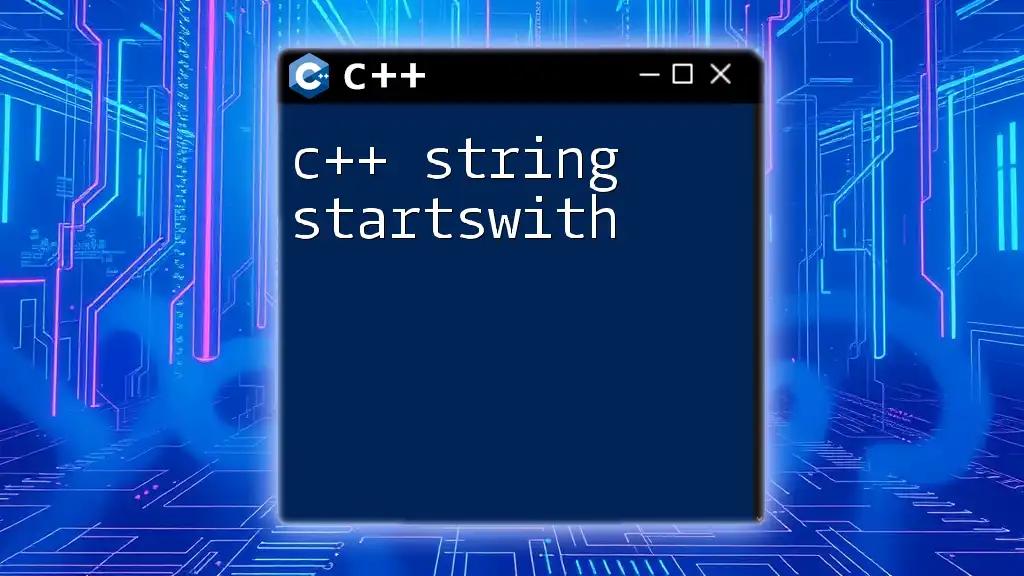
Common Pitfalls and Troubleshooting
Memory Management Concerns
While `strtok_s` helps prevent buffer overflows, it's still essential to manage memory properly. Ensure that strings passed to `strtok_s` are allocated correctly and that any references to those strings are handled safely. Avoid modifying the original string after calling `strtok_s`, as this could corrupt the tokenization process.
Handling NULL Returns
When using `strtok_s`, it’s essential to check for NULL returns appropriately. If `strtok_s` returns NULL, it indicates that there are no more tokens available in the string. Handling these scenarios gracefully prevents crashes and ensures robust code. Here’s how to manage this:
char* token;
while ((token = strtok_s(nullptr, " ", &context)) != NULL) {
std::cout << token << std::endl;
}
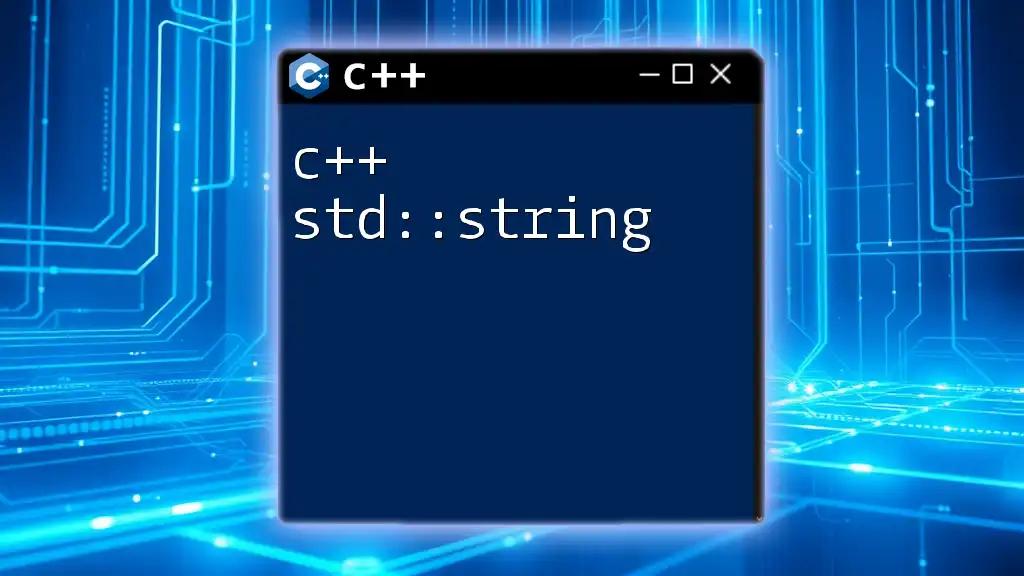
Best Practices for Using `strtok_s`
Consistency in Tokenization
When tokenizing strings, consistency in the delimiters used is critical. It’s important to choose the right set of delimiters for the specific data format you are working with. Analyze your input thoroughly to determine which characters should act as delimiters—this ensures you’re extracting exactly the tokens you need.
Commenting and Documentation
Documenting the logic behind your tokenization is essential for maintaining code. Use comments to explain the purpose of each delimiter used and the expected structure of the input string. Clear documentation improves the readability of your code and assists future developers in understanding the choices made during development.
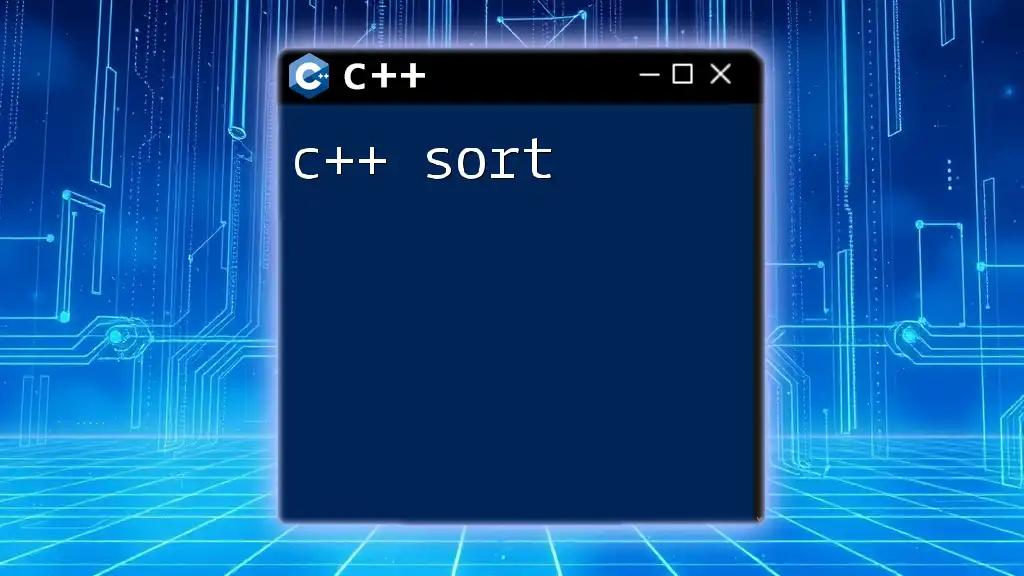
Conclusion
In summary, `strtok_s` is a safer and more efficient function for tokenizing strings in C++ that addresses the pitfalls of traditional methods. It combines ease of use with enhanced safety features, making it an invaluable tool for developers. By understanding and implementing `strtok_s`, you’ll significantly improve the reliability and security of your string manipulation logic in C++.

Additional Resources
To further enhance your understanding of C++ string manipulation, consider exploring the following resources:
- The official documentation for the C++ Standard Library functions.
- Tutorials and articles focused on string handling and processing in C++.
- Online forums and programming communities where you can ask questions and share your experiences with using `strtok_s`.