The `strtok_r` function in C++ is used for tokenizing strings in a thread-safe manner, allowing you to split a string into tokens based on specified delimiters.
#include <iostream>
#include <cstring>
int main() {
char str[] = "Hello,World,Example";
char *token;
char *rest = str;
while ((token = strtok_r(rest, ",", &rest))) {
std::cout << token << std::endl;
}
return 0;
}
What is strtok_r?
The `strtok_r` function is a part of the C standard library, and it is used for tokenizing strings. Unlike its predecessor, `strtok`, `strtok_r` is designed to be thread-safe, making it a preferred choice in multi-threaded applications. It effectively splits a string into smaller strings (tokens) based on specified delimiters, allowing developers to parse and manipulate text more effectively.

When to Use strtok_r?
Using `strtok_r` is beneficial when your program requires safe string tokenization in a concurrent context. If multiple threads need to perform tokenization on the same string or different strings without interfering with each other’s results, `strtok_r` provides the necessary mechanism to ensure safety.
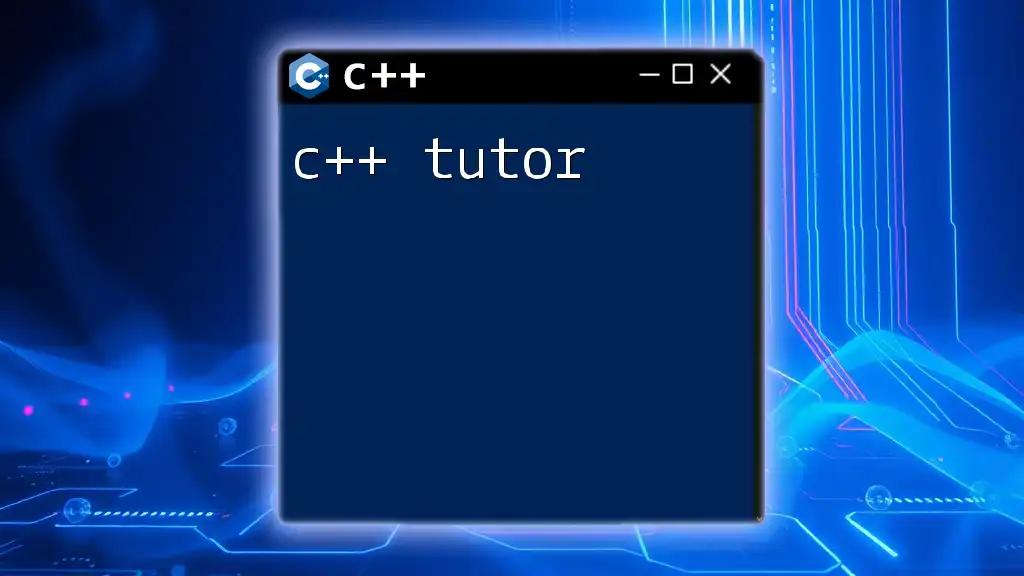
What is Tokenization?
Tokenization is the process of breaking down a string into smaller units or tokens. Each token can then be analyzed or processed separately. For example, consider a CSV (Comma-Separated Values) format: each value in a string like `"apple,banana,cherry"` would be individual tokens.
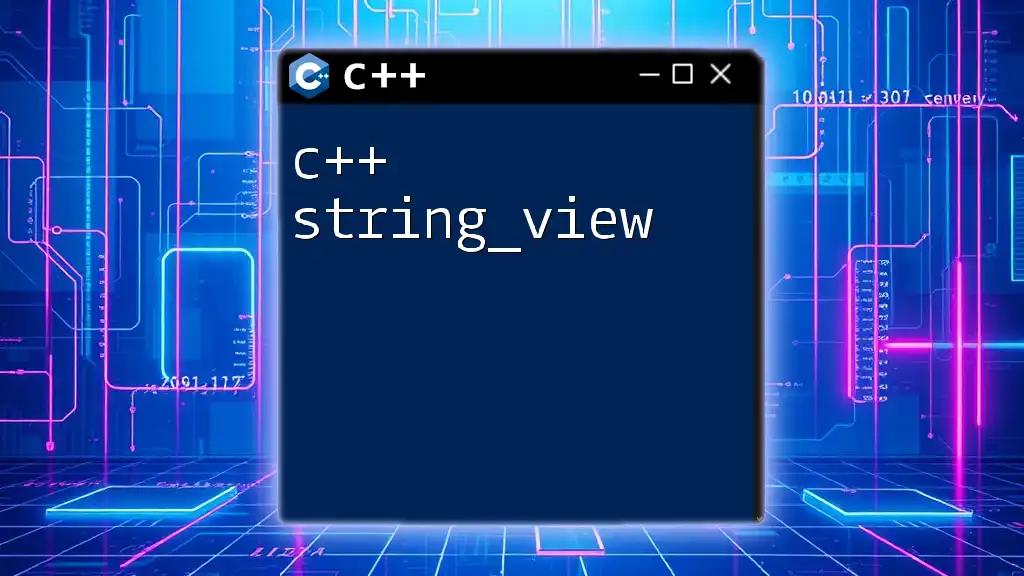
Why Tokenization Matters?
Tokenization plays a crucial role in various programming scenarios, such as:
- Data Parsing: Parsing input from files or user interfaces.
- Network Communication: Extracting meaningful information from data sent over the network, such as JSON or XML data.
- Query Parsing: Analyzing user-input queries for databases or search engines.

Syntax of strtok_r
To use `strtok_r`, it's essential to understand its syntax:
char* strtok_r(char* str, const char* delim, char** saveptr);
Parameters Breakdown
- str: This is the input string to be tokenized. On the first call to `strtok_r`, this should point to the string you want to process. On subsequent calls, this should be `NULL`, indicating you want to continue tokenizing the same string.
- delim: This is a string containing all delimiter characters. Each character in this string is treated as a possible delimiter separating tokens.
- saveptr: This is a pointer to a `char*` variable. It is used internally to store information between successive calls to keep track of the current position in the string.
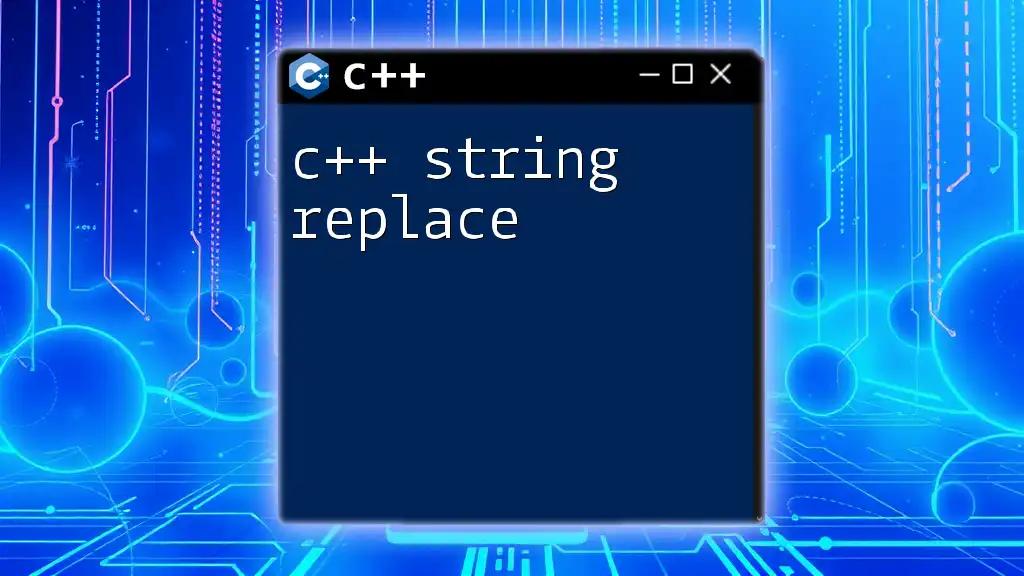
Step-by-Step Guide to Using strtok_r
Setting Up Your Environment
Make sure you include the necessary headers:
#include <iostream>
#include <cstring>
Basic Example of strtok_r
Here’s a straightforward example demonstrating how to tokenize a string using `strtok_r`:
int main() {
char str[] = "Hello,World,Part,2";
char* token;
char* saveptr;
token = strtok_r(str, ",", &saveptr);
while (token != NULL) {
std::cout << token << std::endl;
token = strtok_r(NULL, ",", &saveptr);
}
return 0;
}
Explanation of the Example
The program defines a string `str` that contains several words separated by commas.
- In the first call to `strtok_r`, `str` is passed as the argument. The function looks for the first token, which is `"Hello"`, and updates `saveptr` to point to the next part of the string.
- On subsequent calls to `strtok_r`, passing `NULL` as the first parameter tells the function to continue from where it left off, returning each of the subsequent tokens until there are no more (`NULL` is returned).
- The output of this program will be:
Hello
World
Part
2
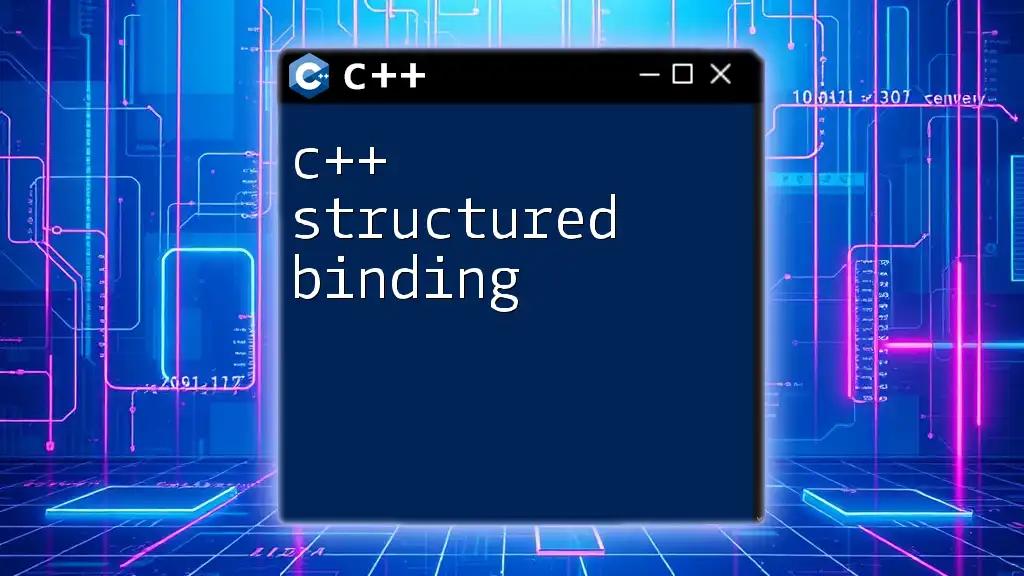
Handling Multiple Tokenization Scenarios
Tokenizing Multiple Strings
In some cases, you might need to tokenize multiple strings sequentially. Here’s how you can achieve that:
void tokenizeMultiple() {
const char* strings[] = {"Apple;Banana;Cherry", "Dog;Cat;Mouse"};
char* token;
char* saveptr;
for (const auto& str : strings) {
char* copy = strdup(str); // Duplicate to preserve original string
token = strtok_r(copy, ";", &saveptr);
while (token != NULL) {
std::cout << token << std::endl;
token = strtok_r(NULL, ";", &saveptr);
}
free(copy); // Free the duplicated string
}
}
Analysis of the Code
In this code, we duplicate the input strings using `strdup` to avoid modifying the original strings during tokenization.
- The loop goes through each string in the `strings` array and tokenizes each of them using `strtok_r`.
- After tokenization, it’s essential to free the duplicated string to prevent memory leaks.
The output will display all tokens from both strings, one per line.
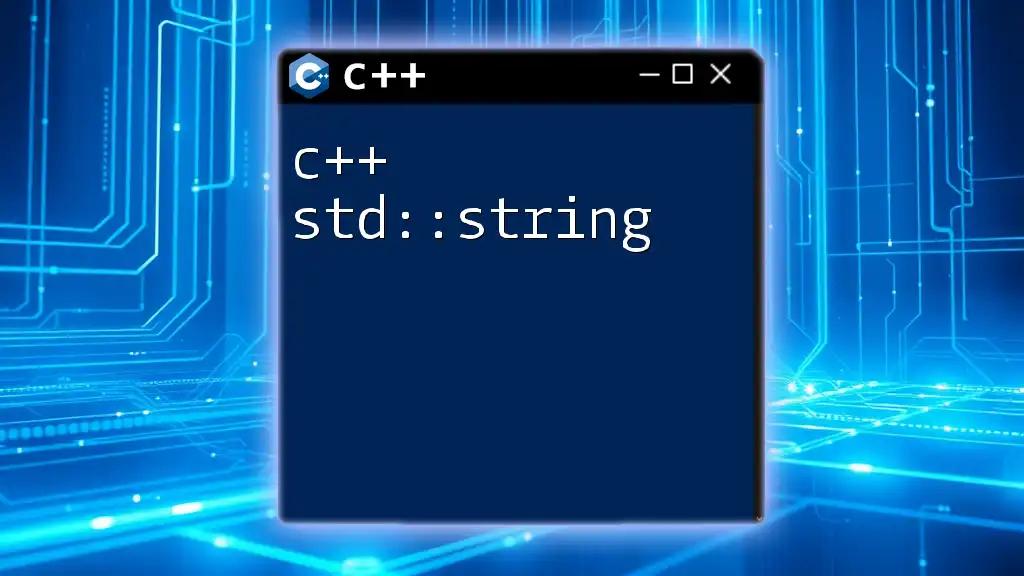
Common Pitfalls and Best Practices
Concurrency Issues and Thread-Safety
A significant advantage of using `strtok_r` is its thread-safety. Unlike `strtok`, which uses internal static storage for the last accessed token, `strtok_r` uses the passed `saveptr` to hold the context, meaning multiple threads can tokenize strings independently.
Using strtok_r Correctly
Ensure you handle cases where the input string is `NULL` or delimiters are absent or incorrect. These conditions can lead to unexpected results or crashes.
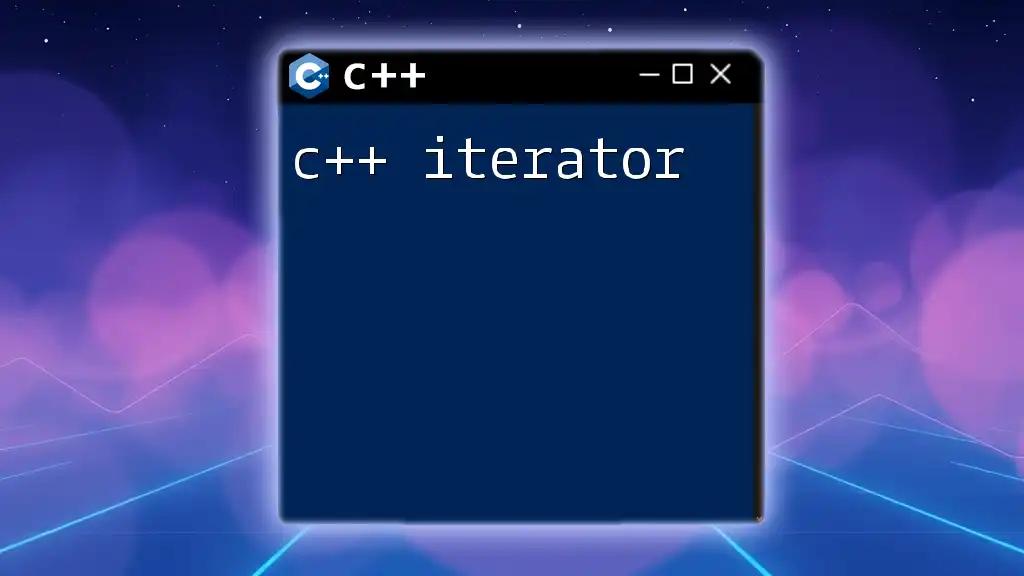
Debugging Issues with strtok_r
Common Errors
Some common mistakes include:
- Forgetting to initialize `saveptr`, leading to undefined behavior.
- Attempting to tokenize const strings, which will cause runtime errors.
How to Debug
Make use of debugging tools or print statements to check the values of tokens and other variables at each step. Ensure to verify that each token is valid before proceeding to the next.
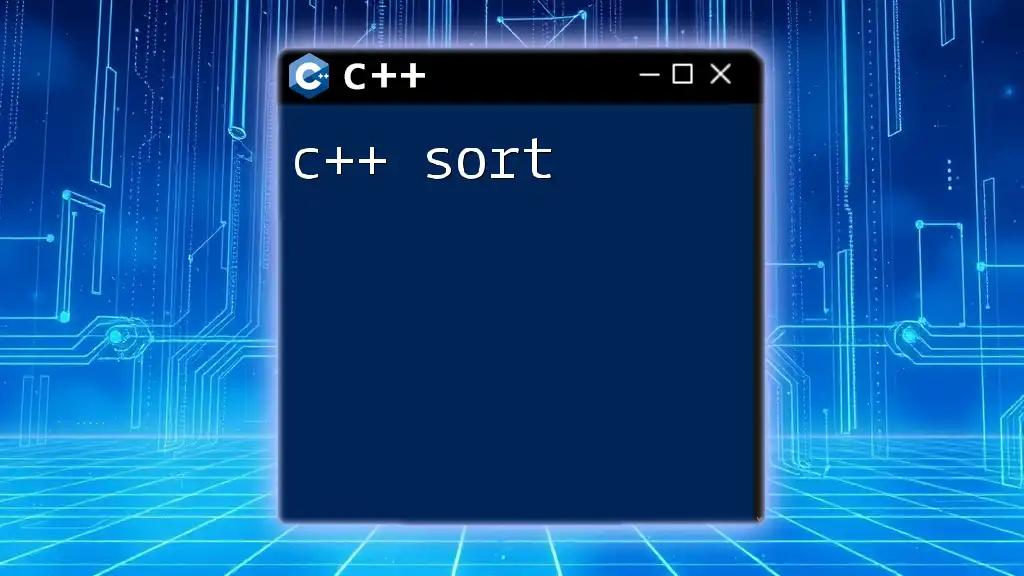
Alternatives to strtok_r
Other Tokenization Functions
If `strtok_r` does not suit your needs or if you're looking for more modern approaches, consider alternatives like:
- `std::string` with `std::find_first_of` for basic tokenization.
- `std::stringstream` for complex parsing tasks.
- Regular expressions can be quite powerful for more intricate string manipulations.
When to Choose Alternatives
Use alternatives when:
- You need more control over the tokenization process.
- Simplicity of implementation is required, wherein helper functions or classes are more manageable.

Conclusion
In summary, `c++ strtok_r` is an essential function for developers needing efficient and thread-safe string tokenization. Understanding its functionality, syntax, and usage can significantly enhance your string-handling capabilities in C++. Practice using `strtok_r` with various inputs to become proficient in string manipulation and parsing.

Further Reading and Resources
For those eager to dive deeper into C++ and string manipulation, consider checking out various programming books and online resources. Engaging with online communities dedicated to C++ can also be a great way to seek help and share knowledge with fellow developers.

Call to Action
Now that you have a comprehensive understanding of `c++ strtok_r`, it's time to practice! Start applying what you've learned by working on small projects or incorporating tokenization into your applications. Embrace the world of strings and elevate your programming skills!