C++ is a powerful programming language that allows you to write fast and efficient applications, and getting started with it involves writing a simple Hello World program.
Here’s a basic example of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful programming language known for its efficiency and versatility. Developed by Bjarne Stroustrup starting in the late 1970s, C++ builds upon the foundation of the C programming language, introducing features that support object-oriented programming (OOP) as well as generic programming. Today, C++ is widely used in system/software development, game development, and performance-critical applications.
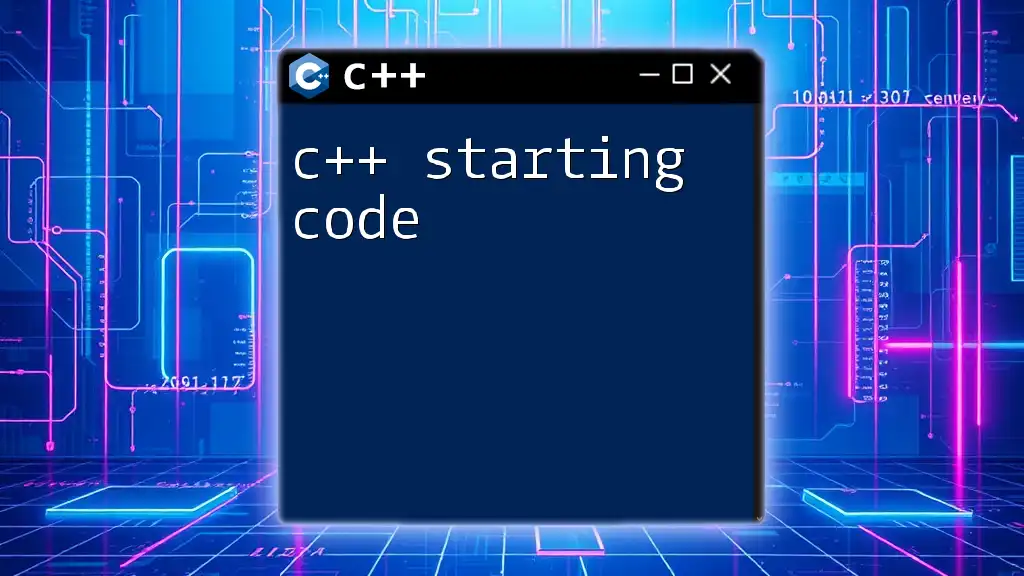
Why Learn C++?
Learning C++ offers several advantages:
-
Performance: C++ is known for its high-performance capabilities. It allows developers to write programs that are very close to the hardware, making it a preferred choice for resource-intensive applications.
-
Control: C++ gives developers fine-grained control over system resources and memory management, which is critical in high-performance applications.
-
Multi-paradigm support: C++ supports various programming paradigms, including imperative, object-oriented, and generic programming, making it a flexible choice for various projects.
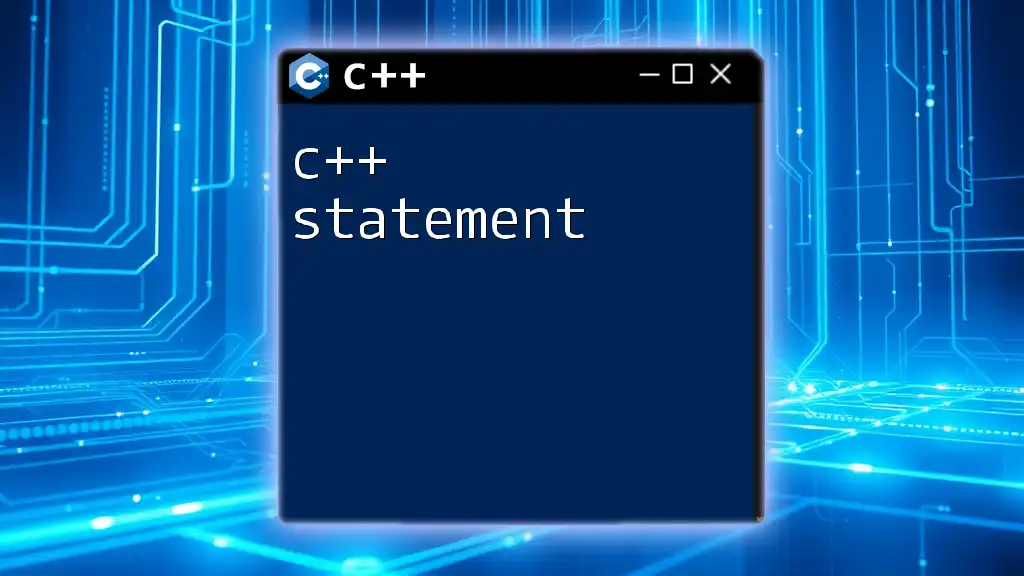
Setting Up Your C++ Environment
Before writing C++ code, it's essential to have a suitable development environment.
Choosing a Compiler
To run and compile C++ code, you need a compiler. Some of the popular compilers include:
- GCC (GNU Compiler Collection): Widely used across different platforms; great for beginners due to its extensive documentation.
- Clang: Known for its performance and error messaging.
- MSVC (Microsoft Visual C++): Specifically designed for Windows environments, offering robust features for professionals.
Installation steps for each compiler vary, but are generally straightforward. Follow the official installation guides for your preferred compiler to get started.
Installing an IDE
An Integrated Development Environment (IDE) can streamline your programming experience. Popular C++ IDEs include:
- Visual Studio: Rich features and an extensive toolset, great for Windows development.
- Code::Blocks: Lightweight and easy to set up, suitable for beginners.
- Eclipse: Offers plugins for C++ development and supports multiple languages.
Follow the specific instructions provided by the IDE of your choice to install and set it up on your system.
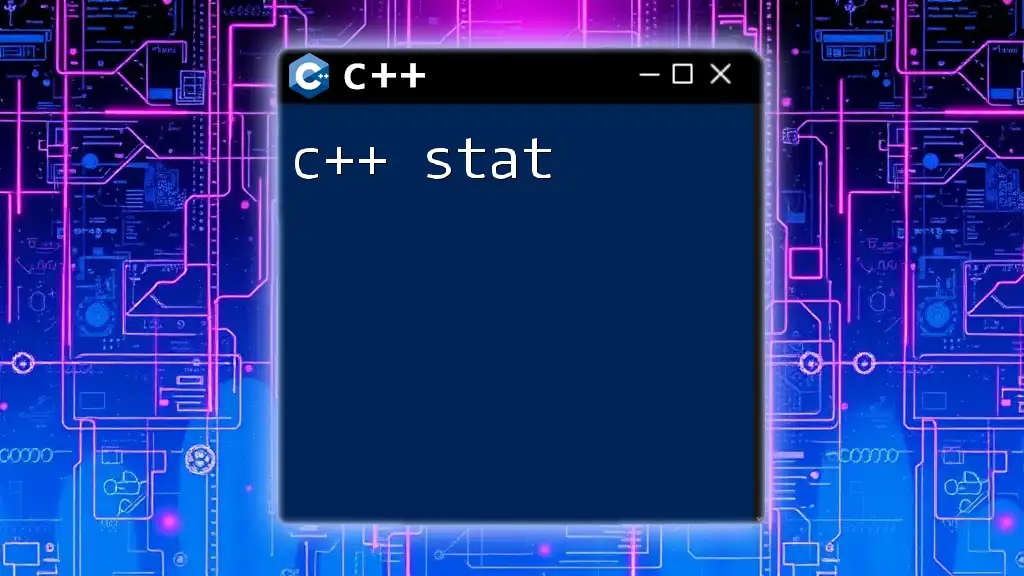
Writing Your First C++ Program
Understanding the Structure of a C++ Program
A basic C++ program typically includes key components:
- Headers: Libraries that provide useful functions and classes.
- Main Function: Entry point of every C++ program.
Here’s an example of a very simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation:
- `#include <iostream>` tells the compiler to include the Input/Output stream library, which allows us to use `std::cout` for console output.
- `int main()` is the starting point of our program. The code inside the braces `{}` defines the instructions that the program will execute.
- `std::cout << "Hello, World!" << std::endl;` sends the text “Hello, World!” to the console, followed by an end-of-line command.
- `return 0;` indicates that the program has finished successfully.
How to Compile and Run C++ Code
Compiling involves translating your C++ code into machine code that the computer can execute. If you’re using the command line, a general command structure for GCC would be:
g++ hello.cpp -o hello
To run the compiled program, you would execute:
./hello
(This command applies to Unix-like systems; on Windows, you would use `hello.exe`.)
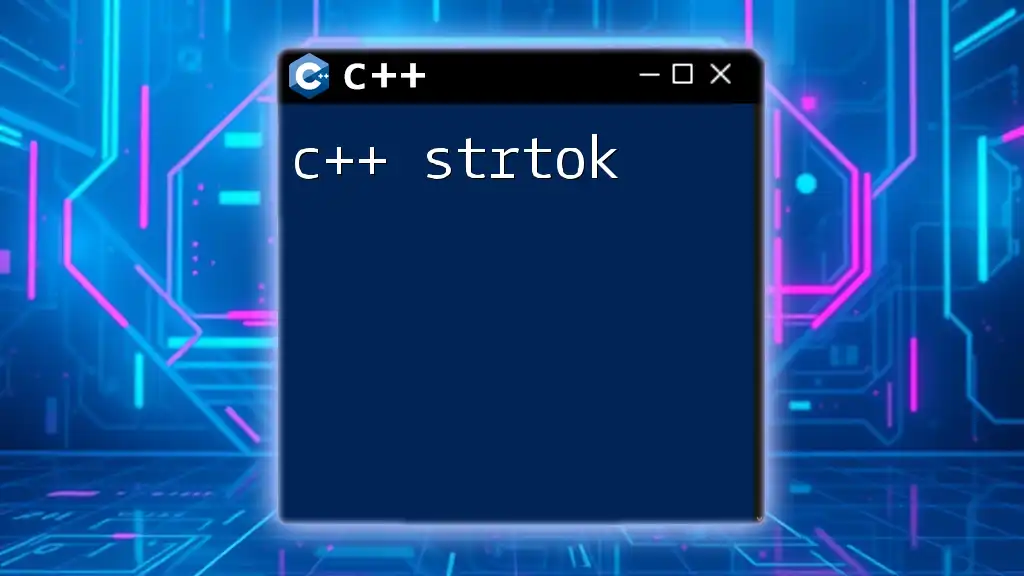
Basic Syntax and Concepts
As you embark on your C++ start, understanding the core syntax and concepts is crucial.
Variables and Data Types
In C++, variables are reserved spaces in memory to hold values. The language supports several primitive data types:
- `int` for integers
- `float` for floating-point numbers
- `char` for characters
- `string` for text data (using the `std::string` library)
Here’s an example demonstrating variable declaration and initialization:
int age = 25;
float salary = 60000.50;
char grade = 'A';
Each variable is defined with a specific data type, allowing the compiler to allocate the appropriate amount of memory.
Control Structures
Control structures allow you to dictate the flow of your program. Two primary control structures include conditional statements and loops.
Conditional Statements
Conditional statements like `if`, `else`, and `switch` are essential to execute different code blocks based on certain conditions. Here’s a simple example:
if (age >= 18) {
std::cout << "You are an adult." << std::endl;
}
This snippet checks the age variable and prints a message based on the condition.
Loops
Loops enable repetitive execution of code segments. Common loop constructs include `for`, `while`, and `do-while`.
Here’s an example of a `for` loop that prints numbers from 0 to 9:
for (int i = 0; i < 10; ++i) {
std::cout << i << std::endl;
}
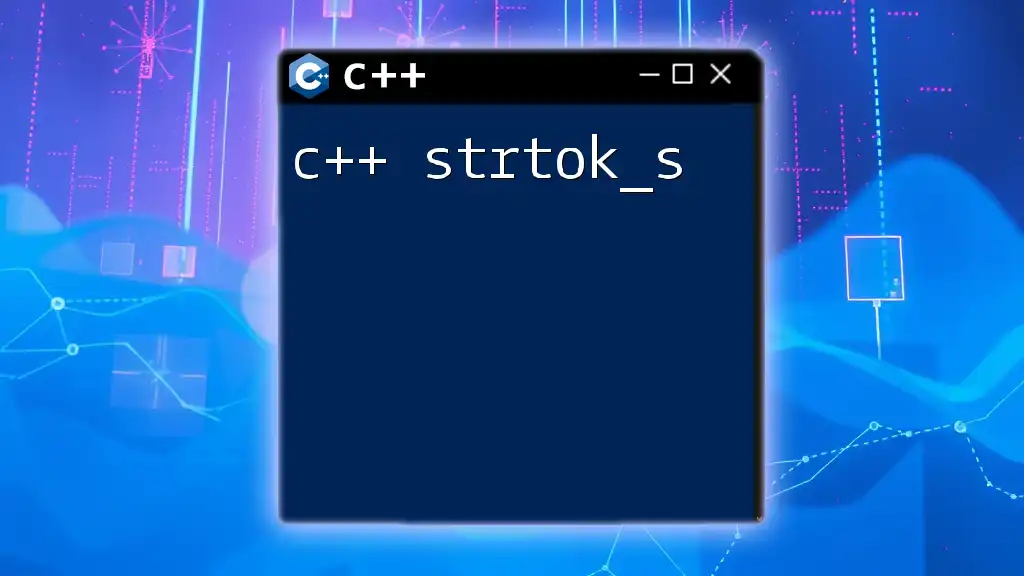
Functions in C++
Functions are blocks of code that perform specific tasks and can be reused throughout your program.
Defining Functions
To define a function, you specify a return type, function name, and parameters. Here’s an example of a simple function that adds two integers:
int add(int a, int b) {
return a + b;
}
You can call this function in your `main()` method:
int result = add(5, 10);
std::cout << "The sum is: " << result << std::endl;
Function Overloading
C++ allows multiple functions to have the same name as long as their parameter lists differ (overloading). This allows for greater flexibility and usability. For example, you can have one `add` function for integers and another for doubles:
double add(double a, double b) {
return a + b;
}
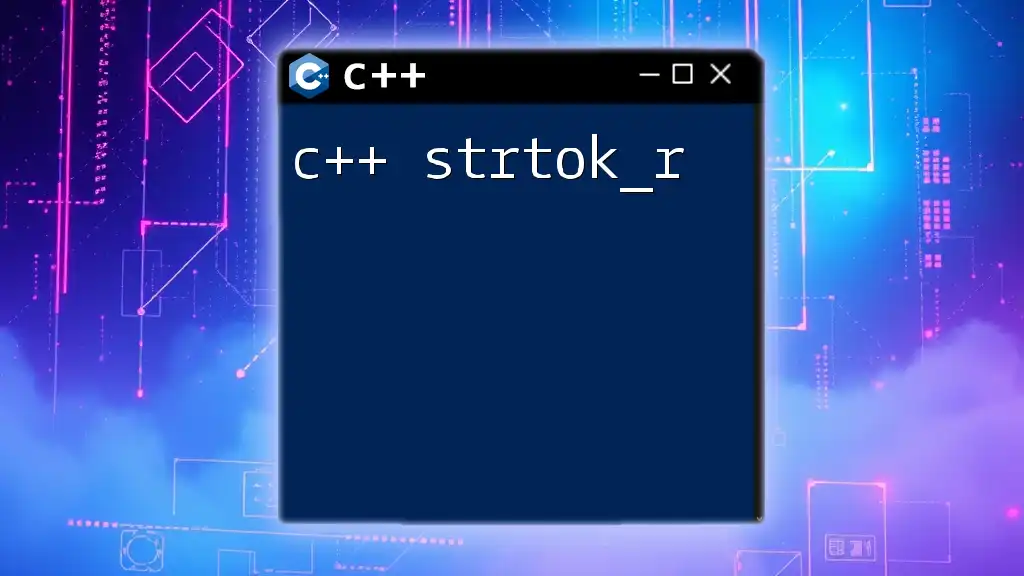
Object-Oriented Programming in C++
C++ is renowned for its object-oriented programming capabilities, which are centered around concepts such as classes and objects.
Introduction to OOP Concepts
In C++, a class is a blueprint for creating objects.
Here’s an example class definition for a simple `Dog`:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
To create an object and use it:
Dog myDog;
myDog.bark();
In this example, `myDog` is an instance of the `Dog` class, and calling `bark()` outputs "Woof!" to the console.
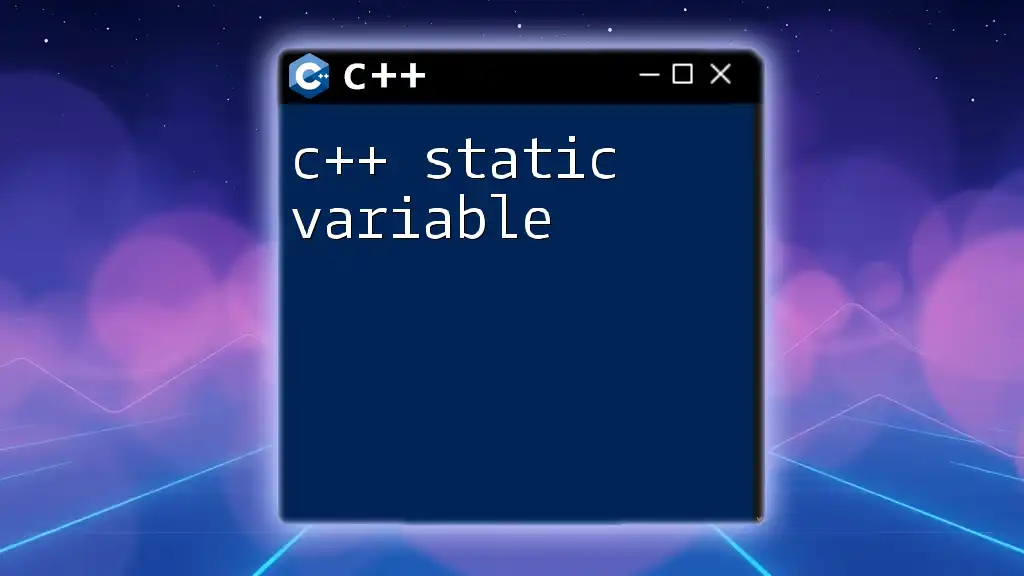
Common Mistakes in C++ Beginners Make
Embarking on your C++ start can be exhilarating, but it's essential to stay mindful of common pitfalls.
Avoiding Syntax Errors
New programmers often run into syntax errors due to small mistakes. Always keep an eye on:
- Semicolons: Each statement must end with a semicolon in C++.
- Brackets: Ensure that all opening brackets `{` have corresponding closing brackets `}`.
Proper indentation and structure not only help compile your code successfully but also enhance its readability.
Memory Management
C++ provides advanced memory management options that require you to allocate and deallocate memory manually using `new` and `delete`. Be cautious of common pitfalls:
- Memory Leaks: Failing to deallocate memory that is no longer needed.
- Dangling Pointers: Using pointers to memory that has already been freed.
Understanding memory management will significantly enhance your proficiency in C++.
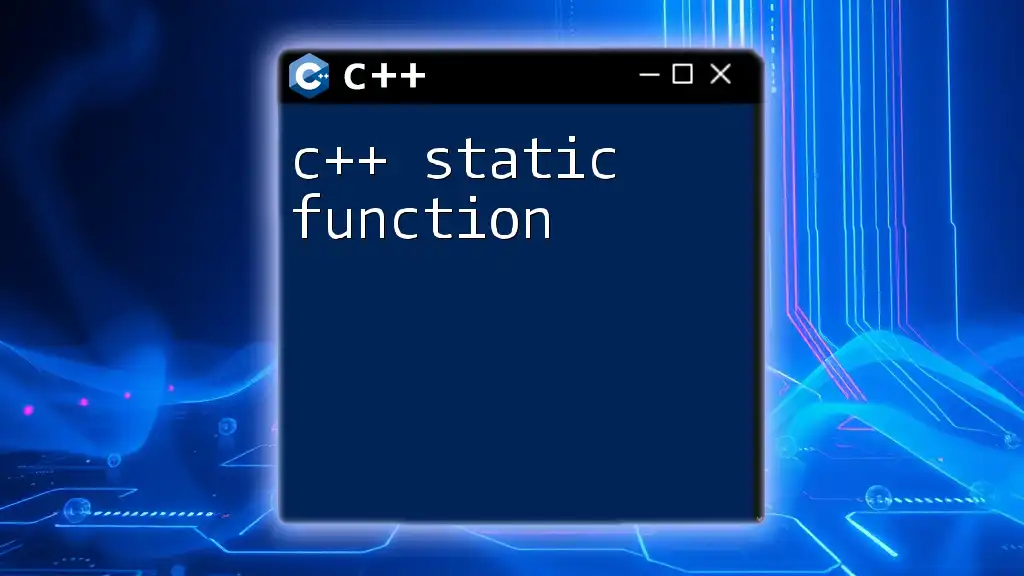
Resources for Learning C++
As you embark on your journey in C++, here are some resources that could enrich your learning experience:
Books and Online Courses
Consider reading foundational books such as "C++ Primer" by Stanley B. Lippman or "Effective Modern C++" by Scott Meyers. Online courses on platforms like Coursera, Udemy, and edX can also provide structured learning opportunities.
Community and Forums
Engaging with the C++ community can be invaluable. Websites like Stack Overflow, Reddit’s r/cpp, or specialized forums can provide support, answers, and collaborative opportunities.
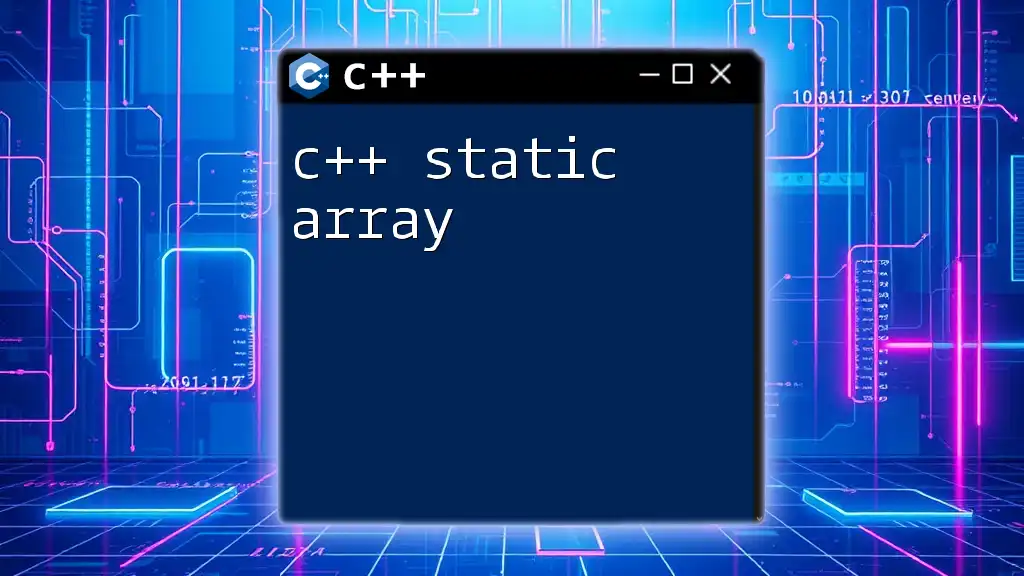
Conclusion
As you take your first steps into C++, remember that consistent practice is key to mastering this powerful language. By understanding the fundamentals, exploring advanced concepts, and engaging with community resources, you'll build a solid foundation for your programming journey. Start coding, experiment with examples, and, most importantly, enjoy the process of learning!