The `calloc` function in C++, which allocates memory for an array of elements and initializes them to zero, is typically used in C programming and can be called from C++ as well.
Here’s a simple example of using `calloc` in C++:
#include <cstdlib> // Include this header for calloc
#include <iostream>
int main() {
int* array = (int*)calloc(5, sizeof(int)); // Allocates memory for an array of 5 integers
if (array != nullptr) {
for (int i = 0; i < 5; i++) {
std::cout << array[i] << " "; // Should print 0 for each element
}
free(array); // Don't forget to free the allocated memory
}
return 0;
}
What is `calloc`?
Definition and Purpose
`calloc`, which stands for "contiguous allocation," is a C-style memory allocation function utilized in C++ for dynamic memory management. Unlike its cousin `malloc`, `calloc` also initializes the allocated memory to zero. This makes it particularly useful when you need a block of memory and want to ensure that all bytes are initialized.
Syntax of `calloc`
The syntax of `calloc` is as follows:
void* calloc(size_t num, size_t size);
- Parameters:
- `num`: The number of elements you want to allocate.
- `size`: The size of each element in bytes.
- Return Value: If the allocation is successful, `calloc` returns a pointer to the allocated memory. If it fails, it returns `nullptr`.
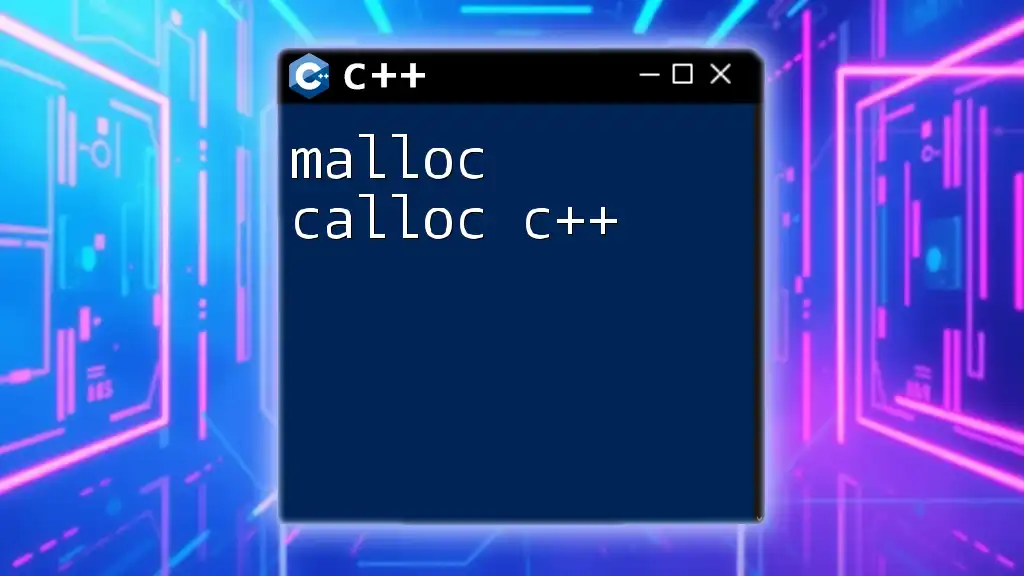
How `calloc` Works
Memory Allocation
Dynamic memory is essential for programs that require memory management at runtime, especially for data structures like arrays, linked lists, etc. When you invoke `calloc`, it allocates a contiguous block of memory that can accommodate an array of elements, ensuring that all bits are set to zero.
Structure of Allocated Memory
Visualizing how `calloc` allocates memory can aid in understanding its benefits. When you allocate an array of `N` integers with `calloc`, the memory address points to a block sufficient to hold `N * sizeof(int)` bytes. The key benefit here is that every element of this array starts off at zero, mitigating risks of garbage values.
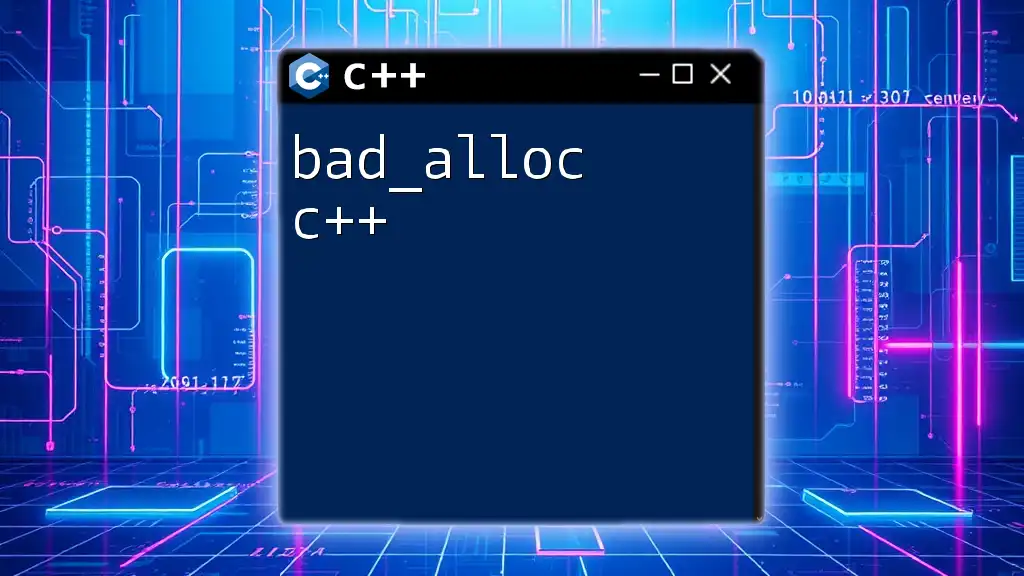
When to Use `calloc`
Use Cases
`calloc` is particularly advantageous in scenarios where:
- You are allocating memory for data structures that represent collections. For instance, if you need to create an array of structs and initialize each field.
- You require predictable default values, as all allocated memory is initialized to zero. This is notably useful in algorithms where an initial value plays a crucial role.
In comparison with other memory allocation functions, `calloc` provides the unique benefit of zero-initialization. While `malloc` merely allocates memory (and leaves it uninitialized), `new` in C++ initializes objects but does not have a direct equivalent to the `num` and `size` parameters.
Performance Considerations
While `calloc` is invaluable for memory initialization, it's essential to consider potential performance implications. The zero-initialization process may add overhead compared to `malloc`, depending on your specific use case. If initialization is unnecessary, using `malloc` can be more efficient. Always weigh the benefits of memory safety against performance needs in critical applications.
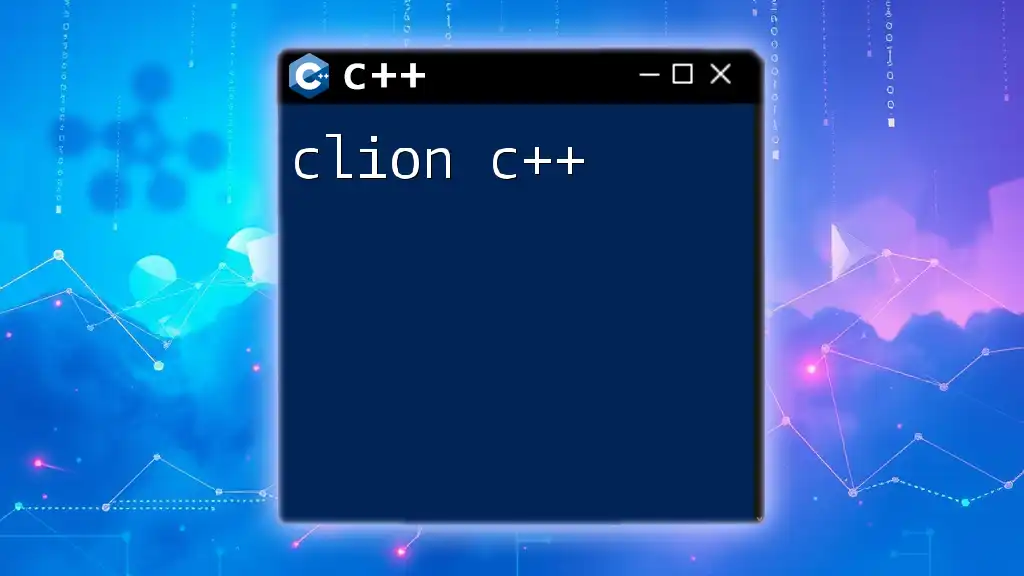
Example Usage of `calloc`
Basic Example
Here’s a straightforward example demonstrating the usage of `calloc` in C++:
#include <cstdlib>
#include <iostream>
int main() {
int *arr = static_cast<int*>(calloc(5, sizeof(int)));
if (arr == nullptr) {
std::cerr << "Memory allocation failed!" << std::endl;
return 1;
}
// Using allocated memory
for (int i = 0; i < 5; i++) {
std::cout << arr[i] << " "; // Should print zeros
}
free(arr); // Freeing the allocated memory
return 0;
}
In this example, `calloc` allocates memory for 5 integers, initializing all to zero. The safety check following the memory allocation ensures that you handle any potential errors gracefully. It highlights critical skills in C++ memory management.
Advanced Example
Let's explore how `calloc` can be effectively used with structures:
struct Employee {
char name[50];
int id;
};
int main() {
Employee *emp = static_cast<Employee*>(calloc(3, sizeof(Employee)));
if (emp == nullptr) {
std::cerr << "Memory allocation failed!" << std::endl;
return 1;
}
// Populate the struct array
emp[0].id = 101;
strcpy(emp[0].name, "Alice");
// Display employee information
for (int i = 0; i < 3; i++) {
std::cout << "Employee ID: " << emp[i].id << ", Name: " << emp[i].name << std::endl;
}
free(emp); // Freeing the allocated memory
return 0;
}
In this case, we allocate memory for an array of `Employee` structures. Each element is zero-initialized, ensuring predictable behavior. This example demonstrates how `calloc` can effectively manage complex data types, highlighting the need for careful memory management in C++.
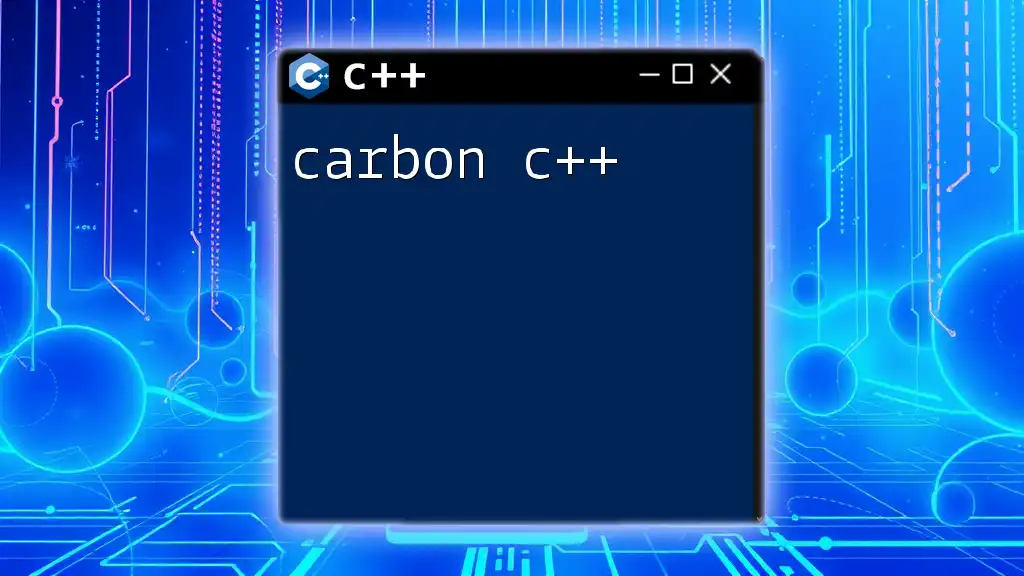
Common Mistakes and Pitfalls
Memory Leaks
One of the most common pitfalls when working with dynamic memory allocation is memory leaks—failing to free allocated memory can lead to wasted resources and degraded performance over time. Always pair your `calloc` calls with a corresponding `free()` to ensure that you correctly release allocated memory when it is no longer needed.
Null Pointer Dereferencing
Dereferencing a null pointer can lead to catastrophic application failures. Always verify that the pointer returned by `calloc` is not `nullptr` before attempting to use the allocated memory. Implementing robust error checking is essential for stable software.
Miscalculating Size
Misestimating the size required for the allocated memory can lead to severe issues such as buffer overruns. Double-check the values being passed to `calloc` to ensure that they accurately reflect the number and size of the elements you need.
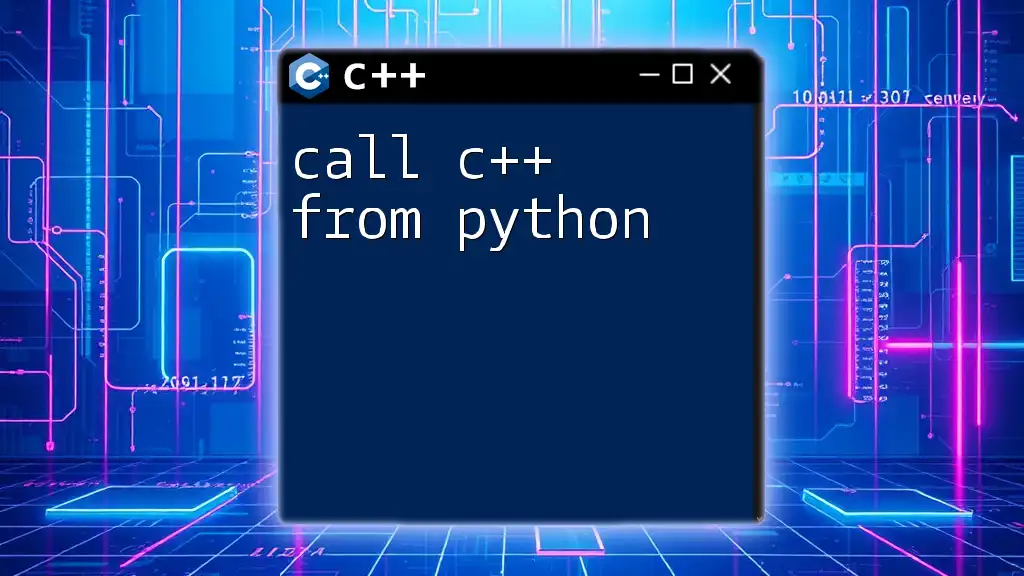
Alternatives to `calloc`
Using C++ New Operator
In C++, the `new` operator offers an alternative to `calloc`, providing automatic initialization of objects:
Employee *emp = new Employee[3];
With `new`, the default constructor for each element is called, which is generally preferable for complex types. However, remember to free the memory with `delete` instead of `free`.
Smart Pointers
For modern C++ applications, consider using smart pointers like `std::unique_ptr` or `std::shared_ptr`. These abstractions automatically manage memory, reducing the risks associated with manual memory management and improving safety:
#include <memory>
std::unique_ptr<Employee[]> emp(new Employee[3]);
This approach greatly decreases the likelihood of memory leaks or dangling pointers, effectively handling resource allocation and deallocation behind the scenes.
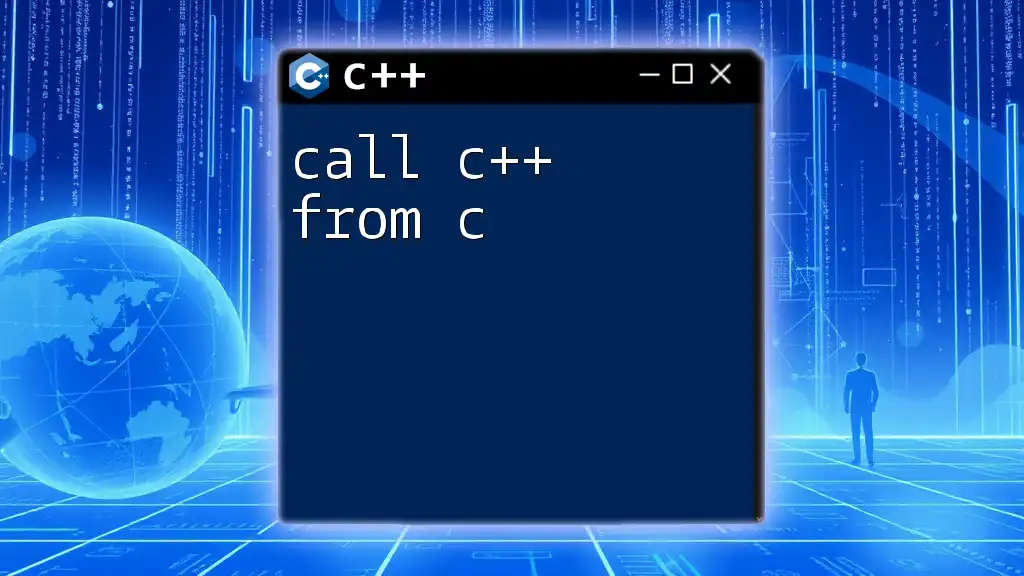
Conclusion
In this article, we explored `calloc` in C++, its syntax, advantages, and important considerations for effective usage. We delved into practical examples to illustrate how to use `calloc` for dynamic memory allocation with safe and predictable initialization. Understanding memory management is crucial for writing efficient and robust C++ applications.
As you continue your journey in mastering C++ memory management, we encourage you to experiment with different allocation techniques, including `calloc`, `malloc`, and the newer C++ constructs. Happy coding!
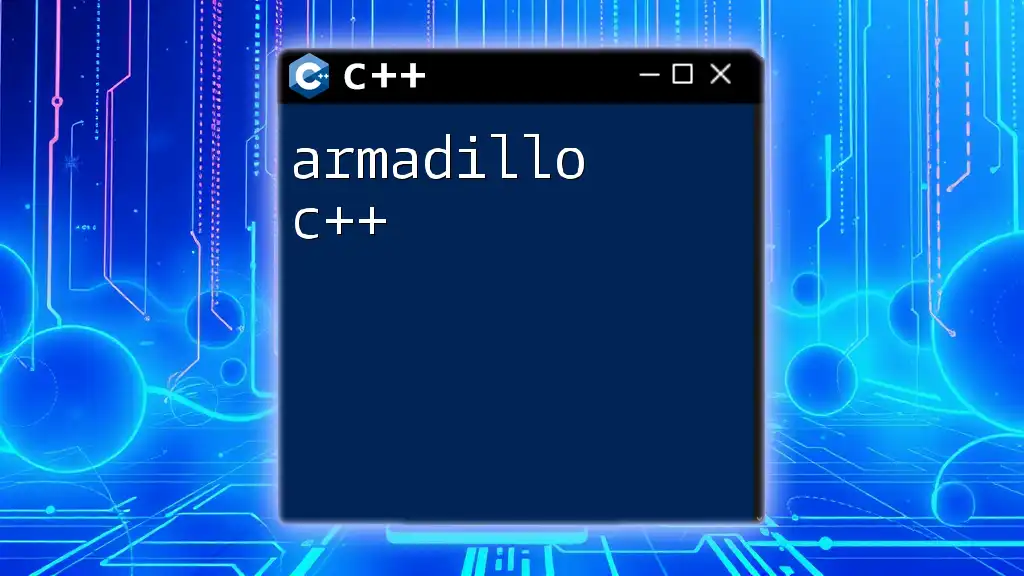
Additional Resources
For further exploration, consider checking out the official C++ documentation and recommended readings on memory management best practices. Each resource will enhance your understanding and proficiency in utilizing various memory allocation strategies in your C++ projects.