In C++, `bad_alloc` is an exception thrown by the memory allocation operator (`new`) when it fails to allocate the requested memory. Here's a simple code snippet demonstrating this:
#include <iostream>
#include <new> // for std::bad_alloc
int main() {
try {
int* arr = new int[100000000000]; // Attempt to allocate a large array
} catch (const std::bad_alloc& e) {
std::cerr << "Memory allocation failed: " << e.what() << std::endl;
}
return 0;
}
Understanding `bad_alloc`
What is `bad_alloc`?
`bad_alloc` is an exception in C++ that is thrown when a dynamic memory allocation request using the `new` keyword fails. This exception is part of the C++ Standard Library and serves as a signal that your program has encountered insufficient memory to fulfill a request. Understanding `bad_alloc` is critical for robust memory management in C++, as it allows developers to anticipate and gracefully handle memory allocation failures.
When Does `bad_alloc` Occur?
`bad_alloc` can occur in various scenarios. Typically, it is triggered when:
- The system runs low on memory.
- The requested memory size exceeds available memory.
- Memory fragmentation prevents large contiguous memory allocations.
For example, in a case where you attempt to allocate a large array and the memory requirements cannot be met, the `new` operation will raise a `bad_alloc` exception.
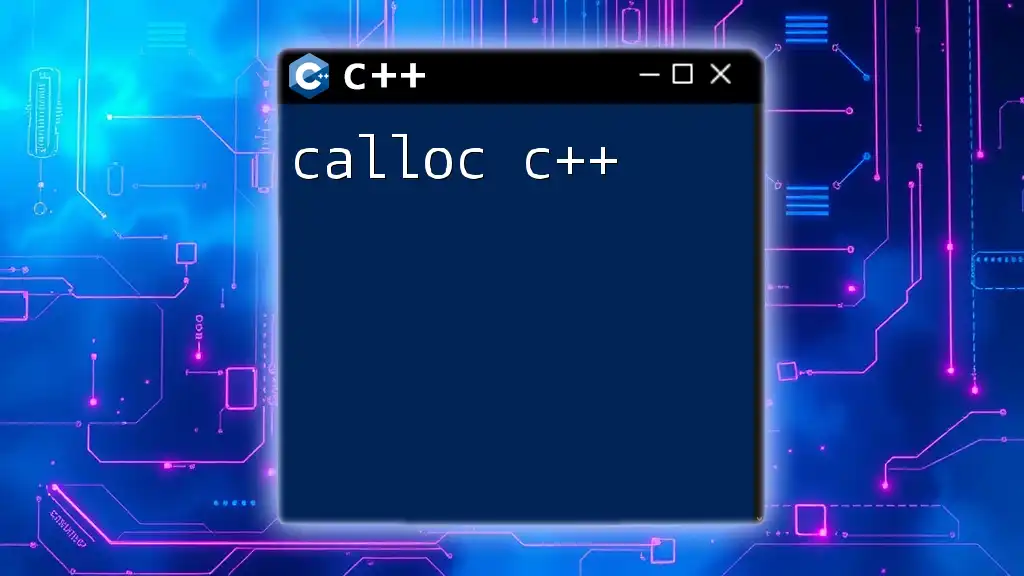
The Role of `std::bad_alloc`
Overview of the Exception Class
As part of the C++ Standard Library, `std::bad_alloc` inherits from the base class `std::exception`. This structure allows it to provide contextual information about the failure. When an allocation fails, `std::bad_alloc` captures the exception and can be caught using standard exception handling mechanisms, thereby enabling developers to take appropriate action.
How It Fits into Memory Allocation
Memory allocation is a fundamental operation in C++. When you use the `new` keyword to allocate memory, `std::bad_alloc` acts as a safety net, raising an exception if the allocation process fails. This mechanism is crucial for preventing program crashes due to unhandled memory allocation errors.
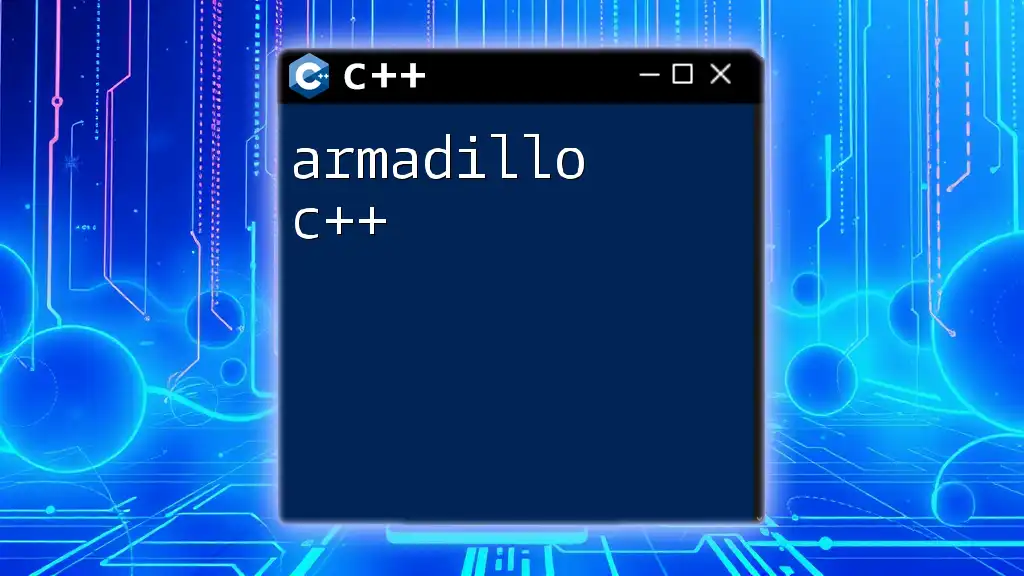
Common Causes of `bad_alloc`
Insufficient System Resources
One of the leading causes of `bad_alloc` is simply running out of memory. When a process requests more memory than what the operating system can provide, the C++ runtime will raise a `bad_alloc` exception. For example, trying to create a large data structure without considering available system resources will likely lead to this error.
Memory Fragmentation
Memory fragmentation occurs when free memory is split into small blocks that are not contiguous. This can make it impossible to allocate larger blocks of memory despite having enough total free space. In such a case, even if there is sufficient memory, fragmentation can result in a `bad_alloc` exception.
Excessive Memory Requests
Reaching out for large memory blocks can easily lead to `bad_alloc`, especially in situations where:
- The program requires an unusually large array or data structure.
- There are hard limits set by the operating system or environment.
If a programmer attempts to allocate too much memory, it can cause the allocation request to fail, raising the `bad_alloc` exception.
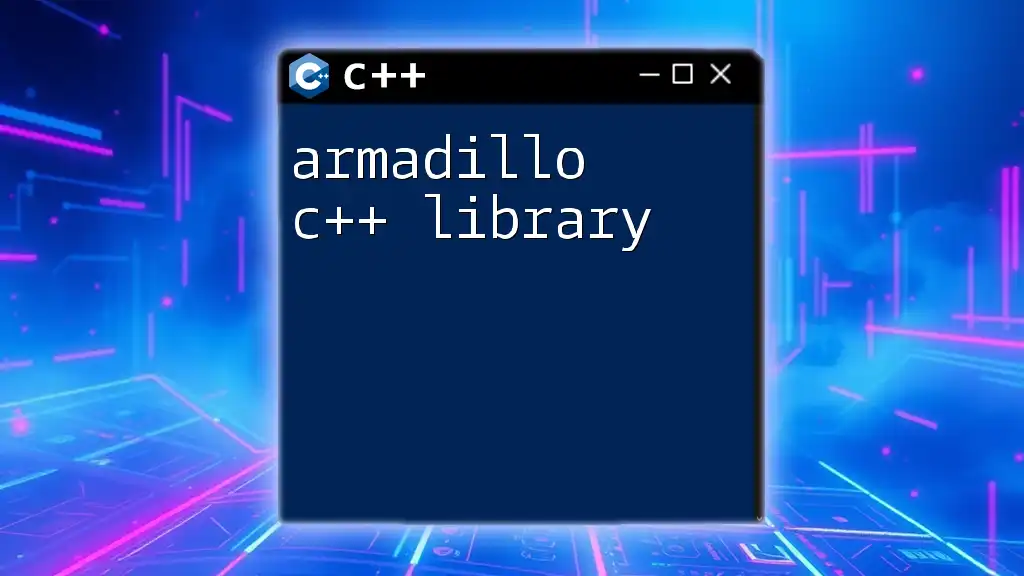
Handling `bad_alloc` in Code
Using Try-Catch Blocks
Handling `bad_alloc` effectively involves using a try-catch block. This allows your program to deal gracefully with memory allocation failures. Below is a code example demonstrating how to catch and handle a `bad_alloc` exception:
#include <iostream>
#include <new> // For std::bad_alloc
int main() {
try {
int* arr = new int[1000000000]; // Attempting massive allocation
} catch (const std::bad_alloc& e) {
std::cerr << "Memory allocation failed: " << e.what() << '\n';
return 1;
}
return 0;
}
In this example, you attempt to allocate a very large array. If the allocation fails, the program will catch the `bad_alloc` exception and print an error message, avoiding a crash.
Custom Error Messages
Improving user experience even further involves customizing the error messages provided when a `bad_alloc` exception occurs. This can aid developers in troubleshooting issues more efficiently. For instance, including specific details about the memory allocation attempt in your error message can provide greater context.
Graceful Degradation
An important aspect of handling `bad_alloc` is implementing graceful degradation. When memory allocation fails, applications should have fallback mechanisms to continue functioning, albeit with potentially reduced capabilities. For example, if a large data structure fails to allocate, you could instead load a smaller batch of data.
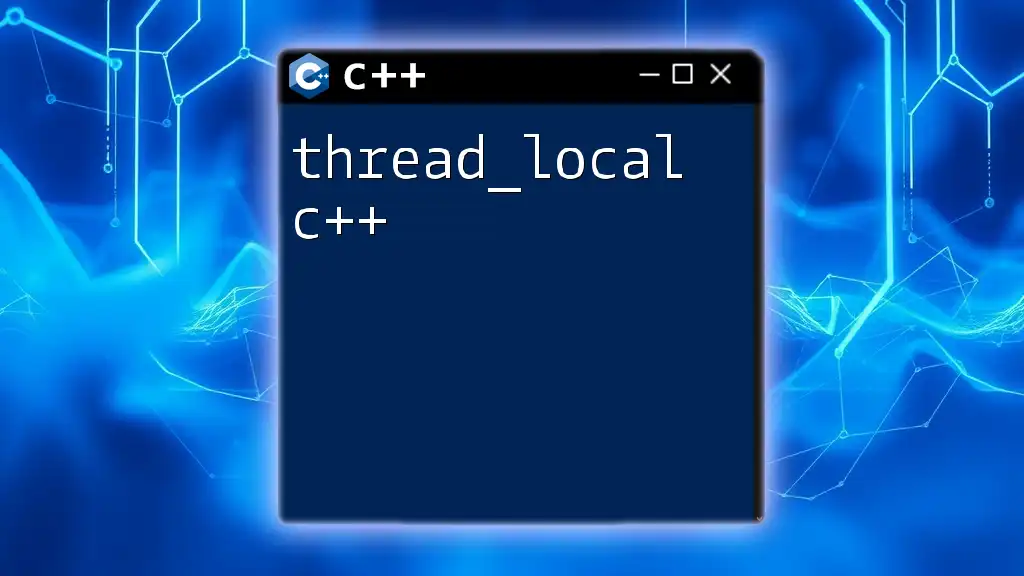
Best Practices to Avoid `bad_alloc`
Optimize Memory Usage
It is crucial to manage memory efficiently to prevent running into `bad_alloc` exceptions. This can be achieved through strategies such as:
- Minimizing the use of large, static arrays.
- Using data structures like `std::vector` which manage memory automatically, thus avoiding manual allocation pitfalls.
Example illustrating good memory practices:
std::vector<int> vec; // Automatically manages memory better
Using `std::vector` instead of raw pointers and arrays allows your program to resize dynamically and handle memory more efficiently.
Monitor Running Memory
Regularly monitoring your program’s memory usage can help you identify potential issues before they lead to a crash. Utilizing tools like Valgrind or AddressSanitizer can provide insights into memory allocation patterns, helping you optimize performance and reliability.
Implementing Smart Pointers
Smart pointers, such as `std::unique_ptr` and `std::shared_ptr`, are beneficial for memory management in C++. They automatically release memory when no longer in use, significantly reducing the likelihood of memory leaks and allocation failures.
Here's an example demonstrating smart pointer usage:
#include <memory>
std::unique_ptr<int[]> arr(new int[100]); // Automatic cleanup
In this case, the smart pointer ensures that the memory allocated for `arr` is properly released when it goes out of scope, reducing the risk of running into `bad_alloc`.
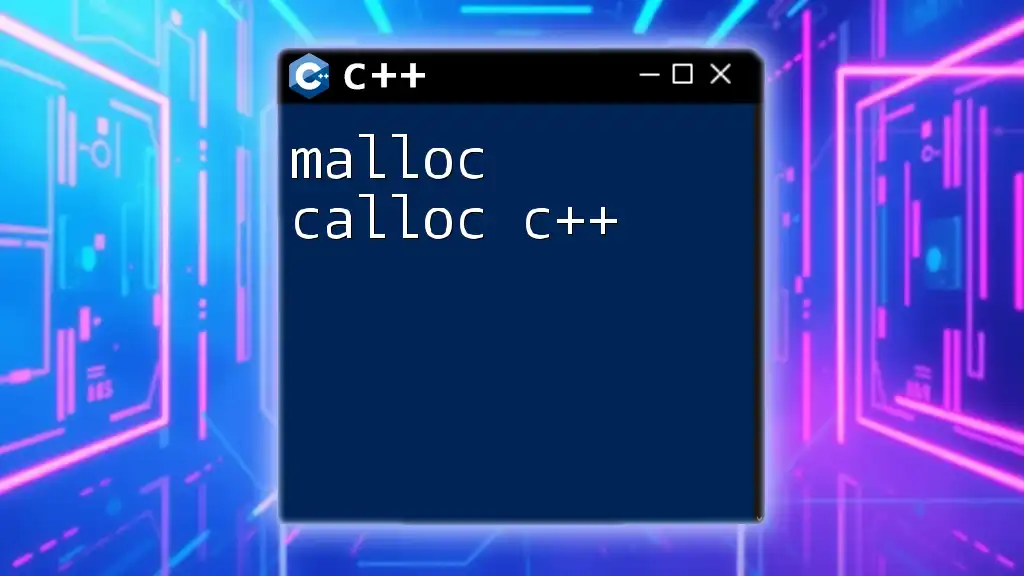
Conclusion
Recap of Key Points
The `bad_alloc` exception in C++ serves as a critical component for robust memory management. Understanding its underlying causes and how to handle them effectively is essential for any developer.
Encouragement to Embrace Robust Exception Handling
By anticipating and managing memory issues through proper exception handling, monitoring, and optimization techniques, developers can create more resilient applications in C++. Utilizing `std::bad_alloc` effectively will lead to safer and more reliable C++ programming.
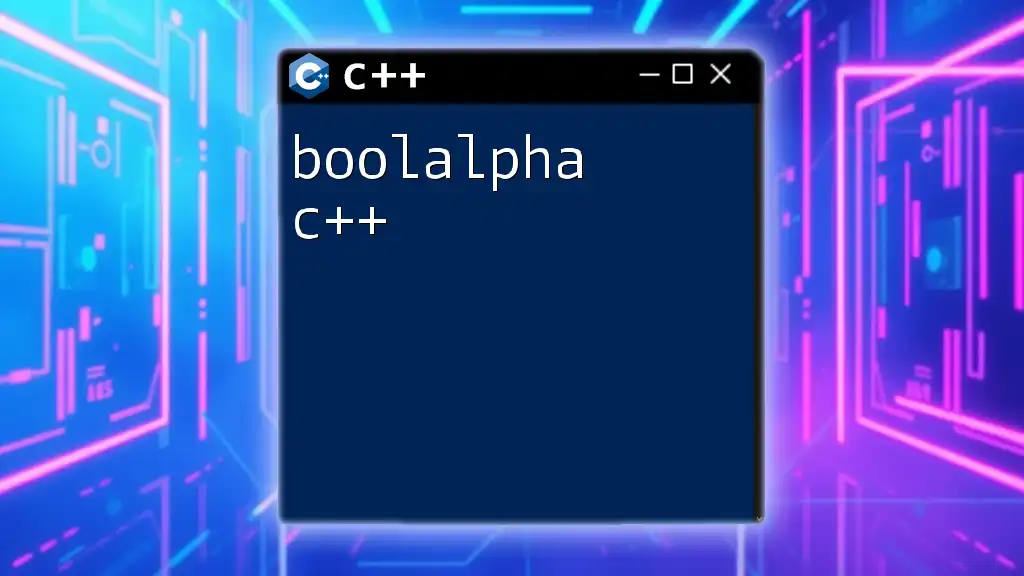
Additional Resources
Referencing C++ Documentation
For more detailed information on `bad_alloc` and exception handling, you can refer to the official C++ documentation.
Suggested Reading and References
A list of recommended books and tutorials on C++ exception handling and memory management can further enhance your understanding and skills in building robust applications.