Symbolic C++ refers to the use of C++ in contexts where symbolic computation is applied, enabling the manipulation of mathematical expressions in a flexible and expressive manner.
Here's a simple code snippet demonstrating symbolic computation using the SymEngine library in C++:
#include <symengine/symengine.h>
#include <symengine/real_double.h>
int main() {
using namespace SymEngine;
RCP<const Basic> x = symbol("x");
RCP<const Basic> expr = add(x, integer(2)); // Creates the expression x + 2
std::cout << "Expression: " << *expr << std::endl;
return 0;
}
What is Symbolic C++?
Symbolic C++ is a powerful library that enables programmers to perform symbolic computation using the C++ programming language. Symbolic computation differs fundamentally from numeric computation in that it operates on mathematical expressions and symbols rather than specific numeric values.
Importance of Symbolic Computation in C++
By integrating symbolic computation capabilities, C++ developers can evaluate, manipulate, and simplify mathematical expressions and equations directly within their code. This opens up possibilities in various fields such as engineering, scientific research, and education.
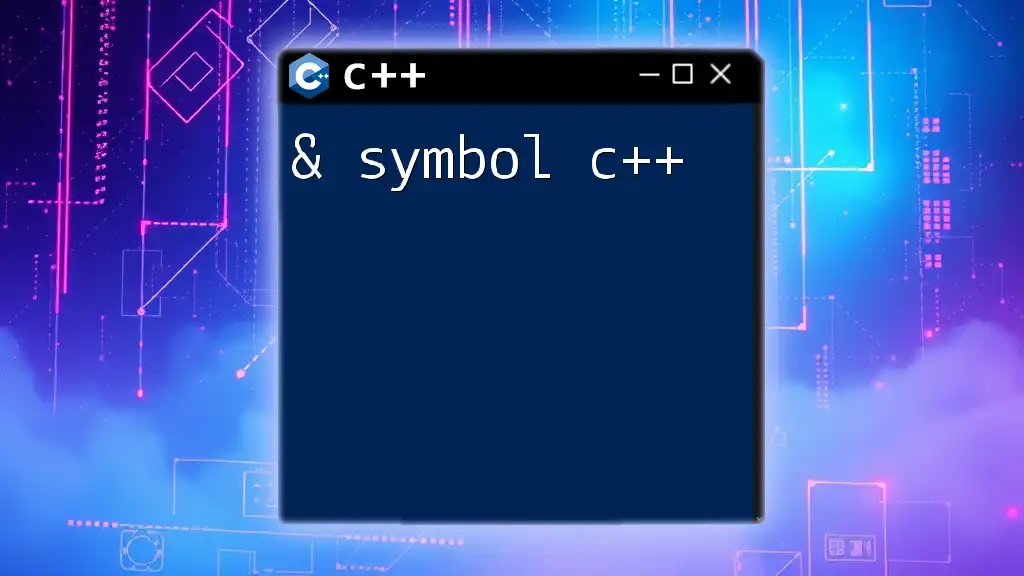
Historical Context
To understand symbolic C++, it is essential to consider its evolution within the programming community. Symbolic computation has a rich history, with significant contributions from figures and projects that paved the way for modern libraries. Initially, symbolic computation became prominent through languages like LISP and Mathematica, which were specifically designed for manipulating mathematical symbols and operations. The development of symbolic computing in C++ incorporates efficiency and object-oriented programming paradigms, allowing for more robust applications.
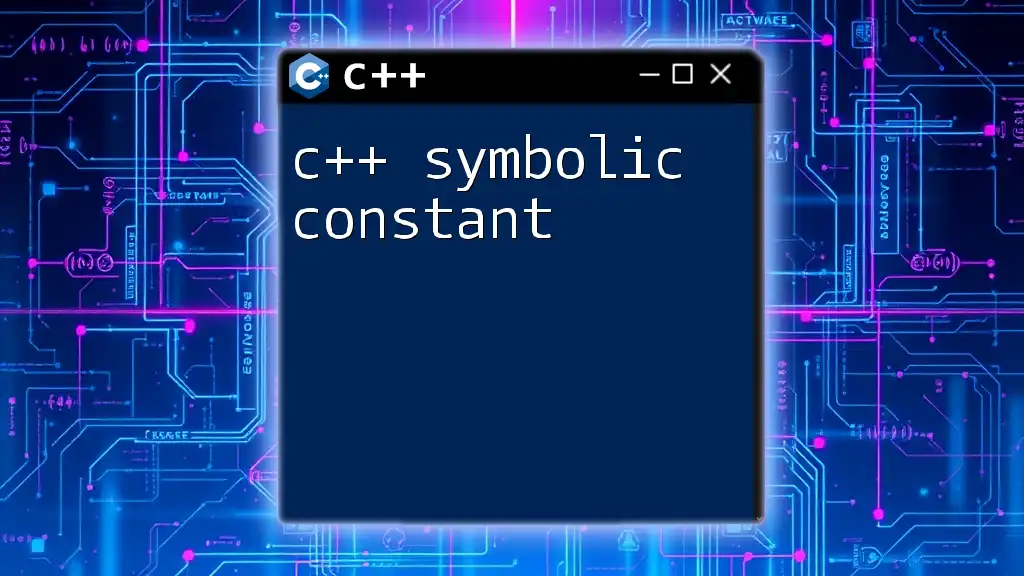
Understanding The Foundation of Symbolic Computation
What is Symbolic Computation?
At its core, symbolic computation refers to the manipulation of mathematical expressions in their algebraic form, as opposed to working solely with numerical approximations. For instance, in symbolic computation, \(x^2 + y^2\) remains in its algebraic form, while numeric computation would involve calculating specific values for \(x\) and \(y\).
Key Concepts in Symbolic C++
A few foundational concepts are vital:
- Variables represent symbols in expressions that can change.
- Expressions can include operations such as addition, subtraction, multiplication, and more.
- Abstract Syntax Tree (AST) is a representation of the structure of expressions, allowing for easier manipulation and evaluation of formulas.
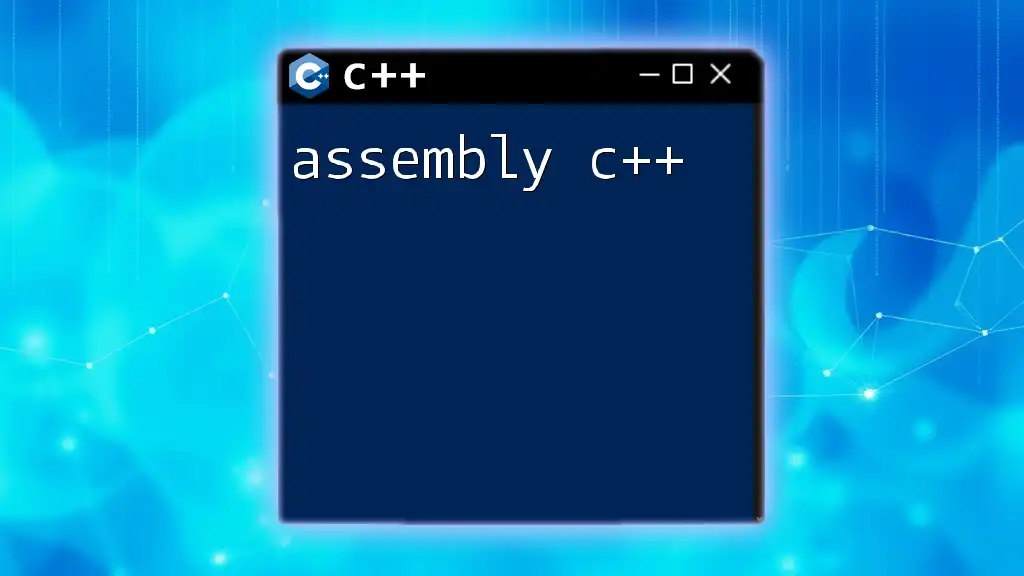
Setting Up Symbolic C++ Environment
System Requirements and Tools
Before diving into symbolic computation, ensure your development environment is equipped with necessary tools and libraries:
- A C++ compiler (e.g., GCC, Clang)
- CMake for building projects
- The Symbolic C++ library itself, which can be found on repositories like GitHub.
Installation Guide
To get started with Symbolic C++, follow these steps:
-
Clone the Repository
Clone the Symbolic C++ library from GitHub:git clone https://github.com/symbolic/symbolic.git
-
Build the Library
Use CMake to configure and build the library:cd symbolic mkdir build && cd build cmake .. make
-
Verify Installation Create a small test program to ensure the library is correctly installed:
#include <symbolic/symbolic.h> using namespace symbolic; int main() { Expression test = Symbol("x") + 1; std::cout << "Test expression: " << test << std::endl; // Outputs: x + 1 return 0; }
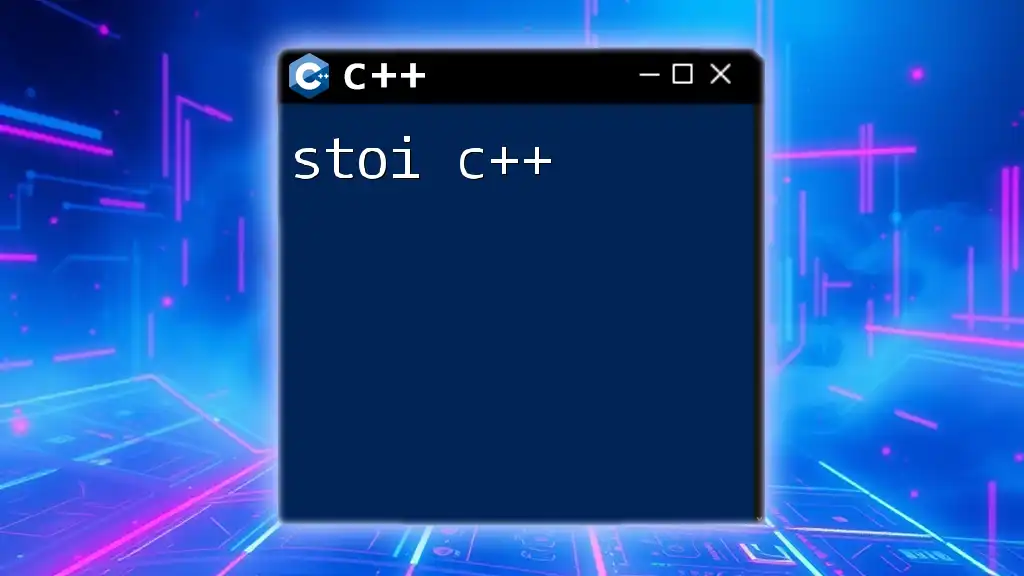
Core Features of Symbolic C++
Basic Syntax and Structure
Symbolic C++ utilizes a simple yet expressive syntax to represent mathematical symbols and expressions. Here's how to create basic expressions:
#include <symbolic/symbolic.h>
using namespace symbolic;
int main() {
Expression x = Symbol("x");
Expression y = Symbol("y");
Expression expression = x + y;
std::cout << expression << std::endl; // Outputs: x + y
return 0;
}
In this example, `Symbol("x")` creates a symbolic variable \(x\), which can be manipulated algebraically alongside other symbols and constants.
Manipulating Expressions
One of the significant advantages of symbolic computation is the ability to manipulate mathematical expressions effectively. For instance, you can easily simplify complex expressions:
Expression expr = (Symbol("x") * Symbol("y")) + (Symbol("y") * Symbol("x"));
Expression simplified = expr.simplify();
std::cout << simplified << std::endl; // Outputs: 2*x*y
This ability to simplify expressions enhances productivity and reduces errors by allowing developers to focus on the mathematical logic without getting bogged down by manual computations.
Solving Equations
Another vital feature of symbolic C++ is its capacity to solve equations symbolically. When given a symbolic expression, you can use the library to extract solutions directly:
Expression equation = Symbol("x")^2 - 4;
std::vector<Expression> solutions = solve(equation, Symbol("x"));
for (const auto& solution : solutions) {
std::cout << "Solution: " << solution << std::endl;
}
This snippet illustrates how to define a quadratic equation and retrieve its solutions symbolically. The results can be displayed in the console, making it easy to interpret without manual computation.
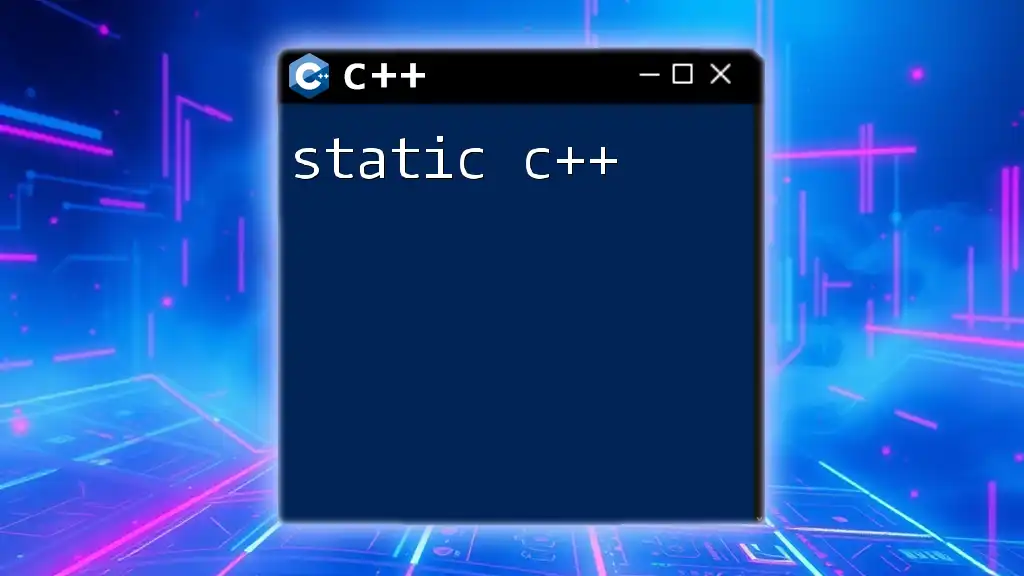
Advanced Symbolic Computation Techniques
Differentiation and Integration
Symbolic computation allows for advanced mathematical operations, such as differentiation and integration, directly within C++ code. Here’s how you can perform differentiation:
Expression func = sin(Symbol("x")) * exp(Symbol("x"));
Expression derivative = func.diff(Symbol("x"));
std::cout << derivative << std::endl; // Outputs: exp(x)*(sin(x) + cos(x))
In this example, the derivative of \(e^x \sin(x)\) is calculated symbolically, which can be invaluable in fields such as physics and engineering.
Numeric Evaluation of Symbolic Expressions
Symbolic expressions can be evaluated numerically, allowing developers to substitute numerical values for symbolic variables easily. Consider the following example:
Expression expression = Symbol("x") + 5;
Expression evaluated = expression.substitute(Symbol("x"), 2);
std::cout << evaluated.eval() << std::endl; // Outputs: 7
This functionality bridges the gap between symbolic and numeric computation, providing flexibility in application.
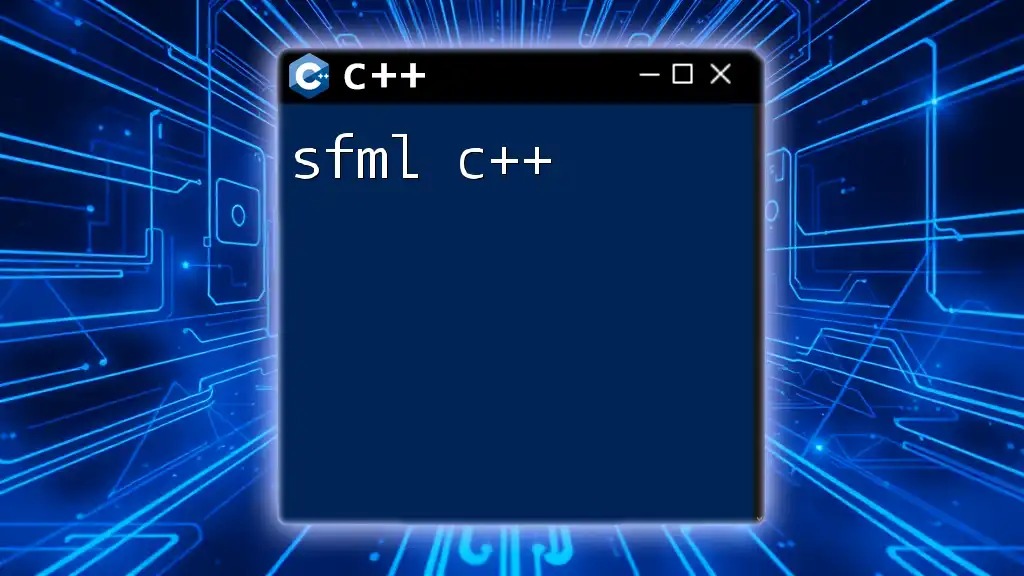
Use Cases of Symbolic C++
Applications in Engineering and Science
Symbolic C++ has numerous applications across various domains, notably in engineering and scientific research. Engineers can utilize symbolic computation for system modeling, optimization, and simulation, enhancing design processes and analysis.
Symbolic C++ in Computer Algebra Systems
Compared to other systems, Symbolic C++ brings the added advantage of integration with C++'s performance and memory management. This feature is beneficial when developing specialized applications that require both speed and mathematical rigor.
Case Studies
Real-world applications of Symbolic C++ can demonstrate its strengths. For instance, in academic settings, it can aid in teaching complex mathematics by allowing students to visualize symbolic manipulation and its consequences in real time.
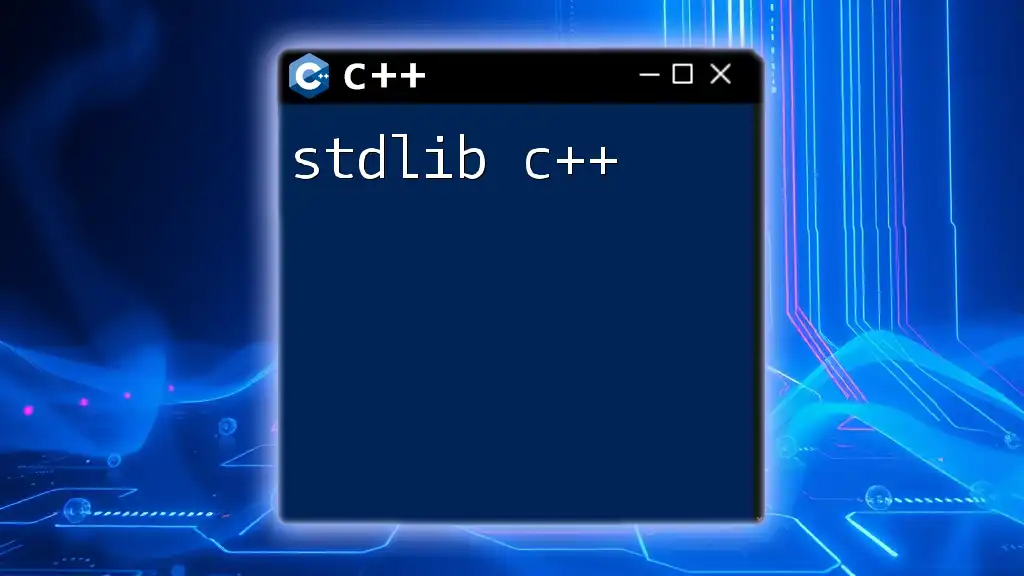
Challenges and Limitations
Computational Complexity
Despite its advantages, symbolic computation can suffer from high computational complexity. Operations on large symbolic expressions can become exponentially more time-consuming than their numeric counterparts. As a result, understanding the limitations of performance is crucial when deciding between symbolic and numeric methods.
Limitations of Symbolic Computation
While symbolic computation is a powerful tool, it isn't suitable for every application. For highly complex problems or cases where numerical results are sufficient, relying solely on symbolic computation may not be the best choice, as it can be inefficient.
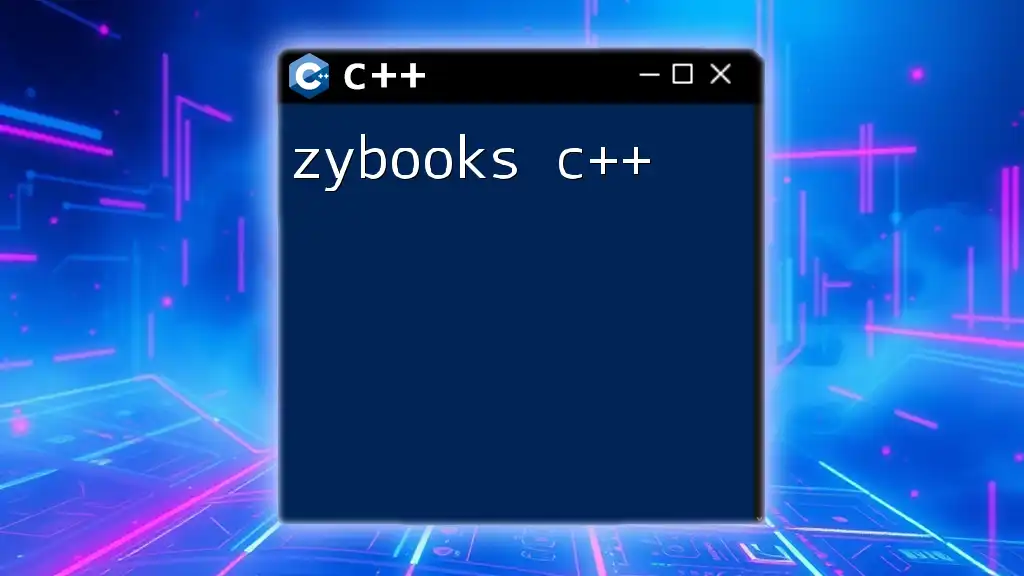
Conclusion
In summary, symbolic C++ offers a robust framework for integrating symbolic computation into C++ applications. It provides tools for manipulating mathematical symbols, solving equations, and conducting advanced algebraic operations. With its applications in diverse fields and a growing community, symbolic C++ is well-positioned to continue evolving and enhancing the way we interact with mathematics in programming.
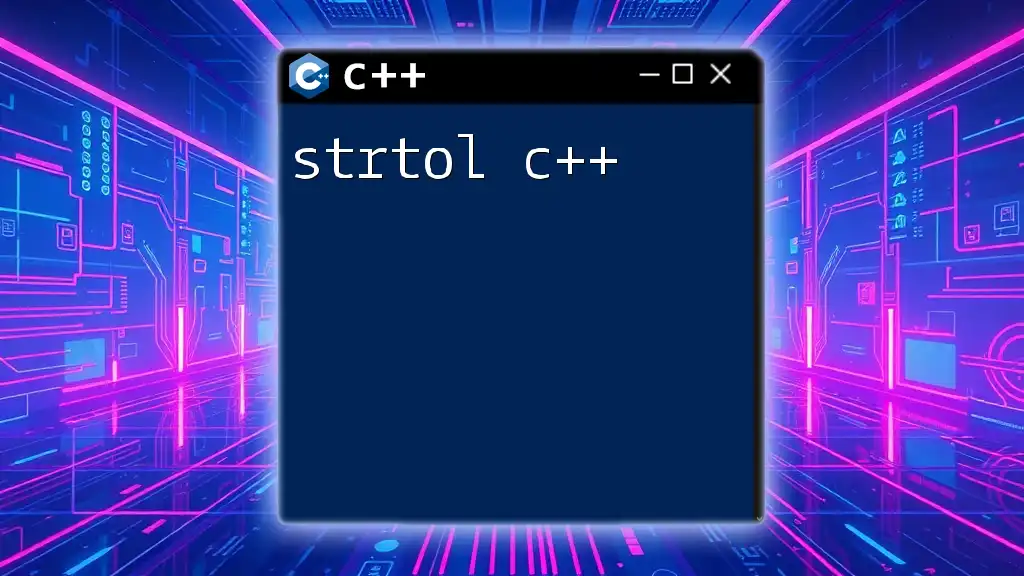
Additional Resources
To dive deeper into symbolic computation, consider the following resources:
- Books that cover symbolic computation and C++ programming.
- Online courses and communities that provide robust learning platforms.
- Open source projects that demonstrate the use of Symbolic C++ in practical applications.
By exploring these resources, you can broaden your understanding and expertise in the fascinating world of symbolic computation with C++.