A C++ symbolic constant is a variable whose value remains unchanged throughout the program, typically defined using the `const` keyword or the `#define` preprocessor directive.
const double PI = 3.14159;
Introduction to Symbolic Constants
Symbolic constants are a vital concept in C++ that enhance both the clarity and maintainability of code. By representing fixed values with meaningful names rather than raw literals, programmers can make their code significantly more understandable. Imagine reading a piece of code filled with numbers; it can be hard to decipher what those numbers actually represent. However, if you use symbolic constants, your logic becomes transparent at a glance.
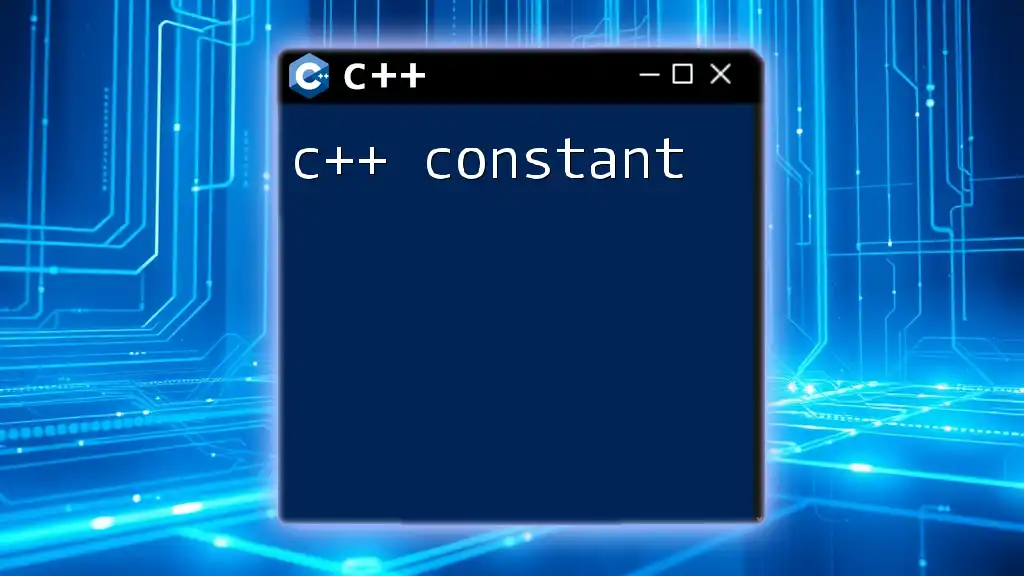
Understanding C++ and Constants
In C++, variables are placeholders for data that can change during program execution. Constants, on the other hand, are fixed values that remain unchanged. Understanding the distinction between these two is crucial for effective programming.
What is a Constant?
Constants in C++ can be categorized into various types:
- Numeric Constants: Such as integers, floating-point numbers, etc. For example, `3.14` is a numeric constant representing the value of Pi.
- Character Constants: Represent single character values, e.g., `'A'`, `'\n'`.
- String Constants: Represent sequences of characters, such as `"Hello, World!"`.
The key difference between constants and variables is immutability. As a programmer, when you declare a constant, you are asserting that its value will never change, which can help prevent unforeseen bugs.
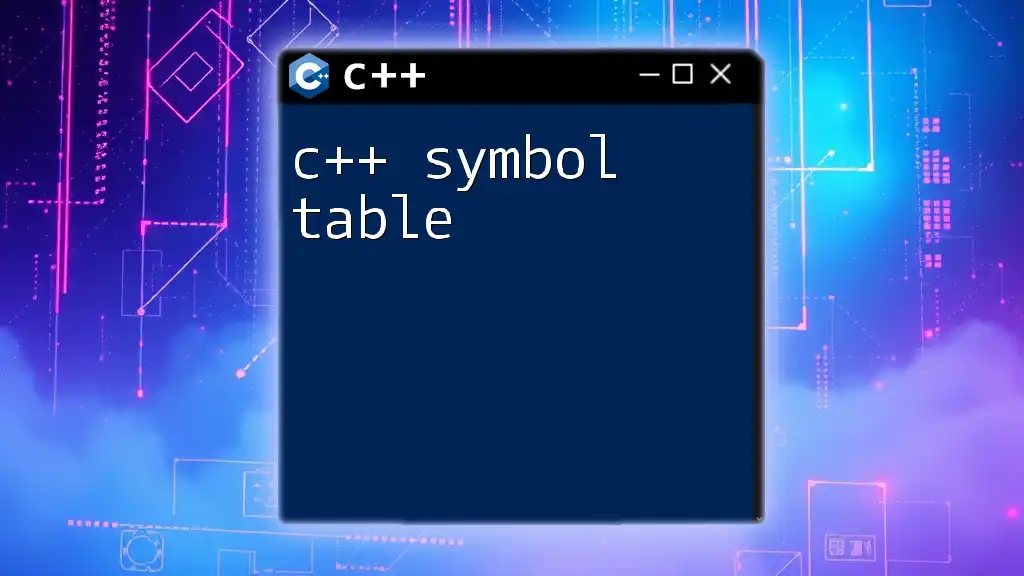
Symbolic Constants in C++
Definition and Importance of Symbolic Constants
A symbolic constant is essentially a name that represents a constant value. Its main purpose is to give a specific meaning to values, thus providing context and reducing the risk of errors caused by using "magic numbers."
How to Create Symbolic Constants in C++
In C++, symbolic constants can be created using:
-
`#define` Directive: This preprocessor directive defines a symbolic constant that can be used throughout the program.
Example:
#define PI 3.14
-
Enum as Symbolic Constants: Enumerations provide a type-safe way to group related constants, making your code easier to manage.
Example:
enum Color { RED, GREEN, BLUE };
When defining symbolic constants, choose clear, descriptive names to enhance readability.
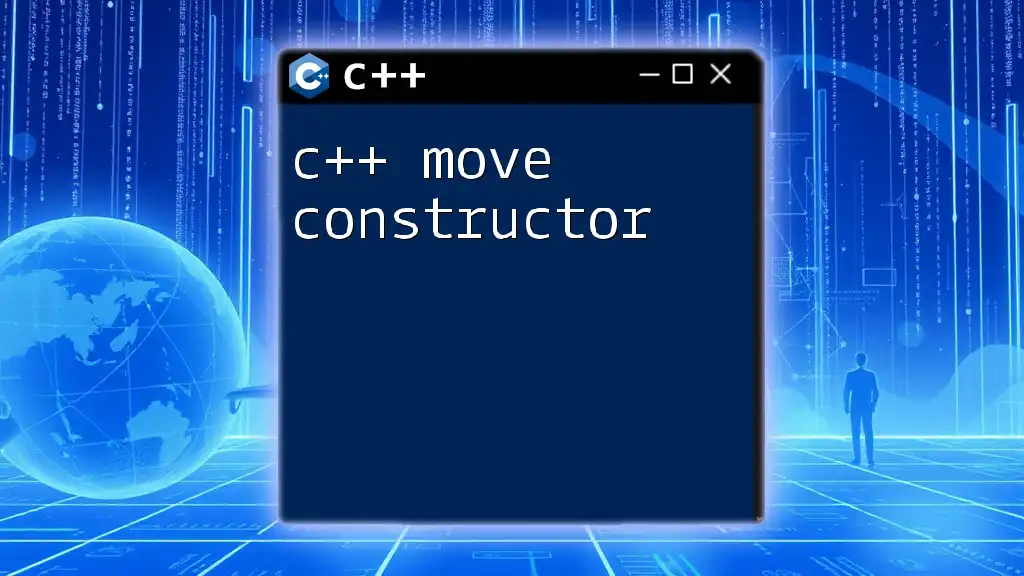
Best Practices for Using Symbolic Constants
When to Use Symbolic Constants
Symbolic constants are especially useful in scenarios that involve fixed values appearing in multiple places in your code. By using symbolic constants, you improve maintainability; if you ever need to change the value, you can do so in a single location.
Naming Conventions
Consistent naming conventions are essential. A widely adopted practice is to use uppercase letters for symbolic constants, with underscores separating words. For example, `GRAVITY_CONSTANT` is clearer than `gravityconstant`.
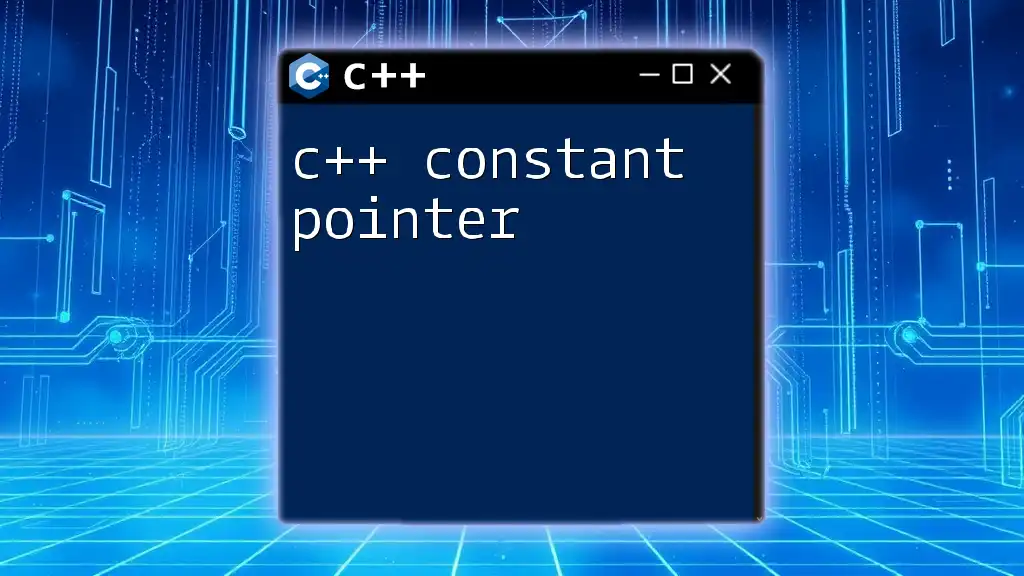
Benefits of Using Symbolic Constants
Enhancing Code Readability
Opting for symbolic constants significantly enhances code readability. When a developer encounters a symbolic constant like `MAX_CONNECTIONS`, they can immediately grasp its significance, as opposed to trying to figure out what the number `100` represents.
Preventing Magic Numbers
Magic numbers are arbitrary numeric literals that appear without context, making the code less readable and prone to errors. For instance, if you come across `if (x > 100)`, it's unclear why `100` is significant. Instead, using a symbolic constant like `if (x > MAX_CONNECTIONS)` clarifies the intention behind that value.
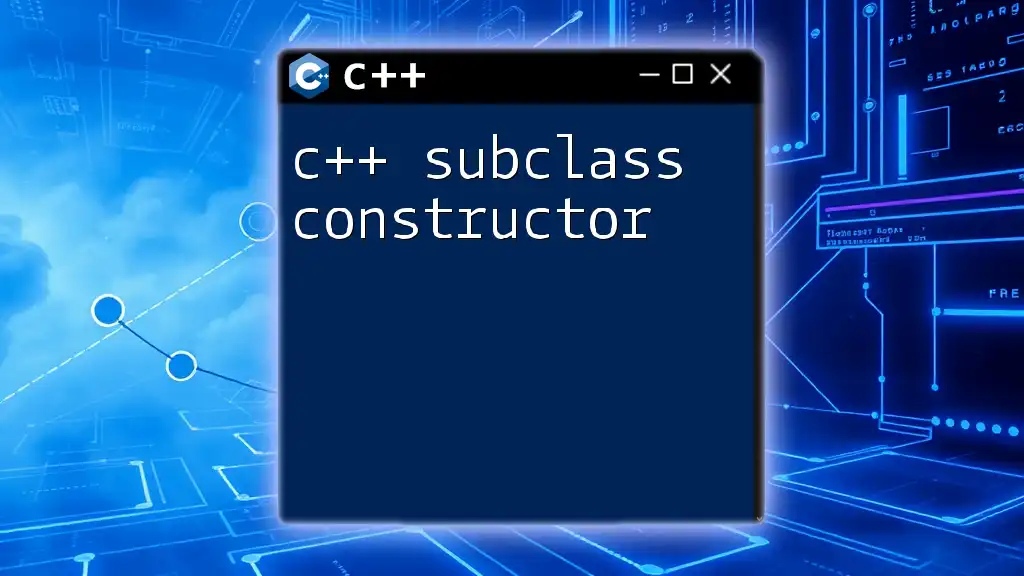
Examples of Symbolic Constants in Practice
Real-world Example
Let's look at a simple program that showcases the use of symbolic constants to make the code more understandable:
#include <iostream>
#define GRAVITY 9.81
int main() {
std::cout << "The acceleration from gravity is: " << GRAVITY << " m/s²" << std::endl;
return 0;
}
In this example, GRAVITY serves as a symbolic constant representing the acceleration due to gravity. It elevates the comprehensibility of the output.
Using Enum for Symbolic Constants
Consider using `enum` for creating symbolic constants:
#include <iostream>
enum Days { MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY };
int main() {
Days today = FRIDAY;
std::cout << "Today is " << today << std::endl; // Output: Today is 4
return 0;
}
Here, the `Days` enumeration provides clear and type-safe symbolic constants for days of the week.
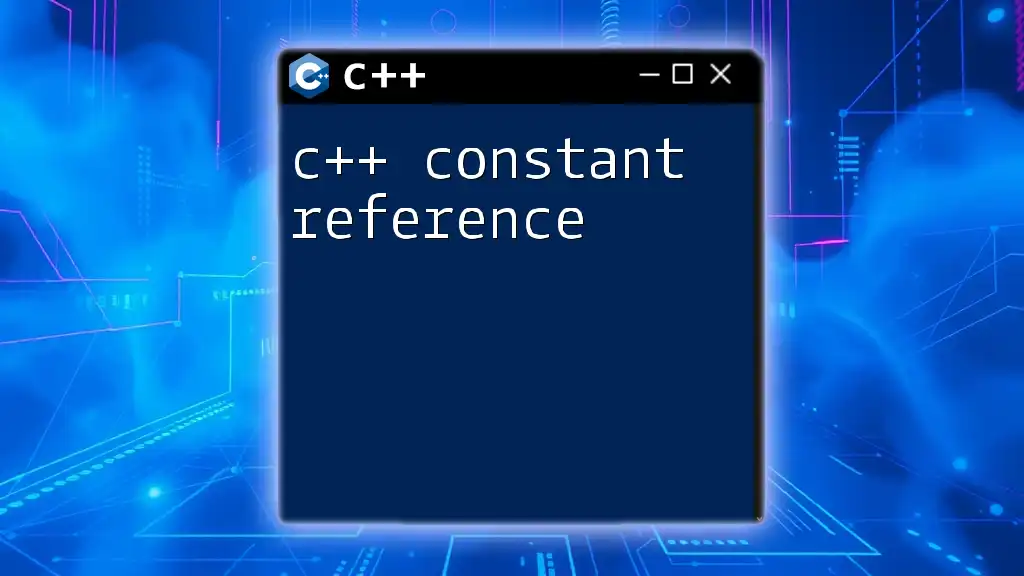
Common Pitfalls to Avoid
Misusing Symbolic Constants
One common mistake involving symbolic constants is inadvertently altering their values. Since symbolic constants are meant to be immutable, care must be taken not to redefine them unintentionally in different areas of your program.
Importance of Correct Scoping with Constants
Be aware of scoping issues. Global symbolic constants may lead to name clashes or bugs if different parts of your code redefine them. Using `const` variables within the appropriate scope is often a better practice.
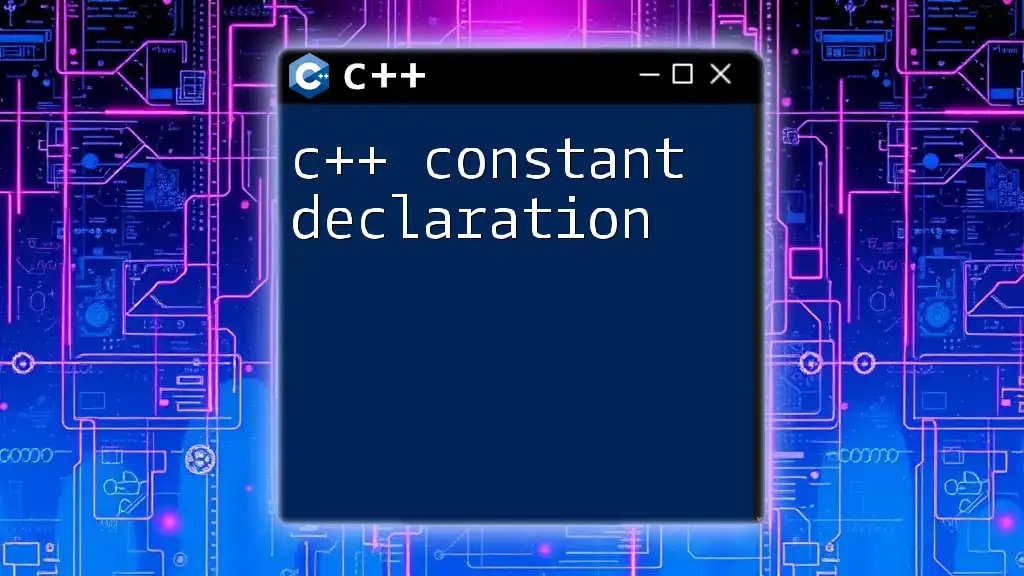
Conclusion
In summary, understanding and implementing symbolic constants in C++ can vastly improve the clarity and maintainability of your code. By using meaningful names instead of raw literals, you create self-documenting code that is easier for others—and future you—to understand. The journey towards cleaner, more efficient programming practices starts with the mindful application of symbolic constants.
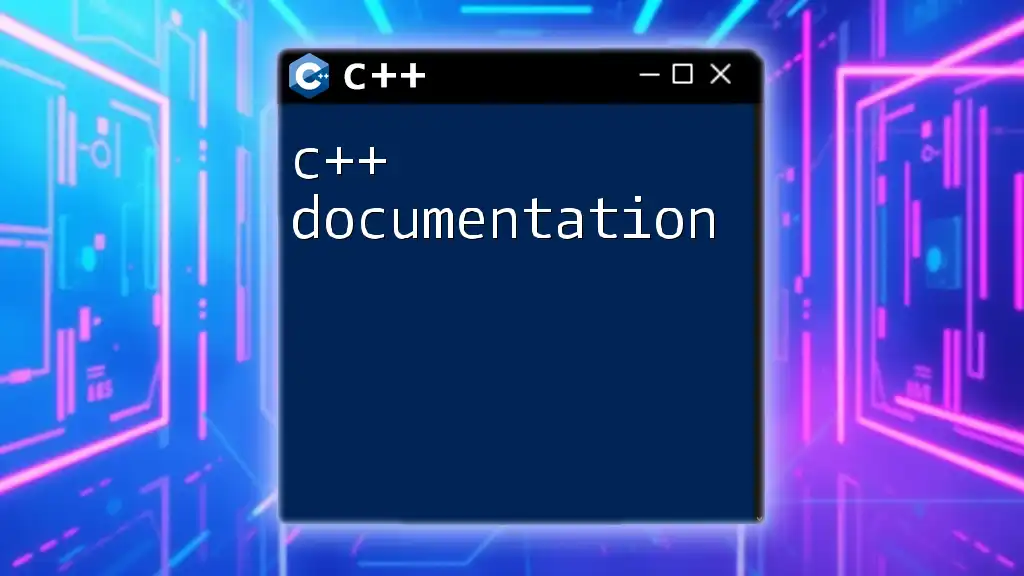
Additional Resources
For those keen on deepening their understanding of C++ and programming best practices, consider exploring books, articles, and online resources that delve into advanced programming techniques. Continuous learning is essential for growth in the ever-evolving landscape of technology.