In C++, symbols typically refer to operators, keywords, or identifiers used in the language's syntax, which are essential for performing operations and defining program structure.
Here's a code snippet demonstrating the use of a common symbol in C++:
#include <iostream>
int main() {
int a = 5; // Declaration with the '=' symbol
int b = 10;
int sum = a + b; // '+' symbol is used for addition
std::cout << "The sum is: " << sum << std::endl; // '<<' is the stream insertion symbol
return 0;
}
What Are Symbols in C++?
Symbols in C++ represent the fundamental building blocks of the language, allowing programmers to communicate instructions to the compiler. They act as identifiers for variables, functions, and data types, serving as a bridge between human language and machine understanding. Understanding the different types of symbols and their uses is crucial for writing effective and maintainable C++ code.
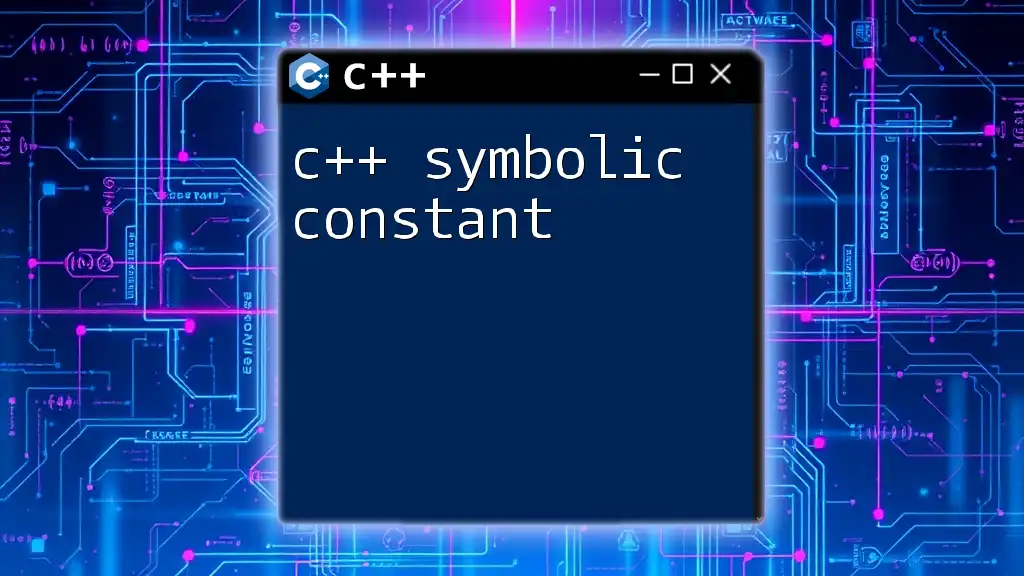
Types of Symbols in C++
Identifiers
Identifiers are names given to entities in a program such as variables, functions, arrays, etc. They enable programmers to effectively refer to these entities throughout their code.
Rules for Naming Identifiers
When declaring identifiers, specific rules must be followed:
- Case Sensitivity: C++ recognizes identifiers as case-sensitive, meaning `Variable` and `variable` are treated as distinct identifiers.
- Character Restrictions: Identifiers can include letters, digits, and underscores, but cannot begin with a digit.
Examples of valid and invalid identifiers:
int validIdentifier; // valid
int _anotherValid; // valid
int 1invalidIdentifier; // invalid (starts with a digit)
int invalid-variable; // invalid (contains a hyphen)
Operators
Operators in C++ are symbols that perform operations on one or more operands. A diverse set of operators exists, making C++ a powerful language for mathematical and logical computations.
Arithmetic Operators
These symbols perform basic math calculations. Common arithmetic operators include:
- Addition (+): Adds two operands.
- Subtraction (-): Subtracts the second operand from the first.
- Multiplication (*): Multiplies two operands.
- Division (/): Divides the first operand by the second.
Example:
int a = 5;
int b = 10;
int sum = a + b; // Using the + operator
Relational Operators
Relational operators are used to compare two values, returning a boolean result. Key relational operators include:
- Equal to (==): Checks if two operands are equal.
- Not equal to (!=): Checks if two operands are not equal.
- Greater than (>): Checks if the left operand is greater than the right.
Example:
if (a > b) { // Using the > operator
// Code to execute if a is greater than b
}
Logical Operators
Logical operators are used to combine multiple boolean expressions. Common logical operators include:
- AND (&&): Returns true if both operands are true.
- OR (||): Returns true if at least one operand is true.
Example:
if (a > b && a < 20) { // Using the && operator
// Code to execute if both conditions are true
}
Punctuation Symbols
Punctuation symbols are essential for structuring C++ code correctly. They include braces, semicolons, and commas.
Braces, Semicolons, and Commas
- Braces (`{}`): Define the beginning and end of a block of code.
- Semicolon (`;`): Indicates the end of a statement.
- Comma (`,`): Used to separate multiple variables or function arguments.
Example:
if (condition) { // Using braces
// Code block
} // End of block
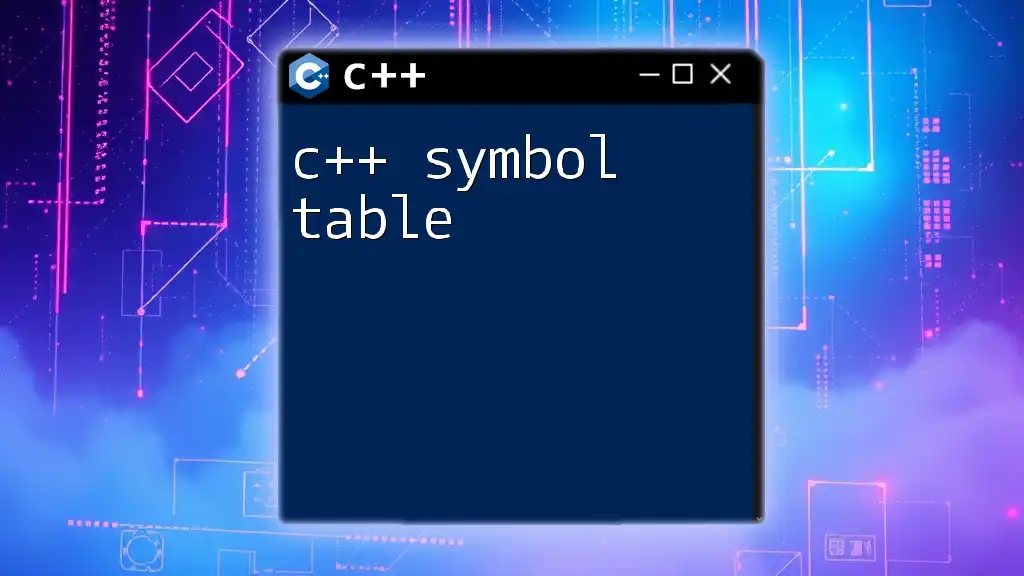
The Role of Symbols in the C++ Language
How Symbols Facilitate Communication between Compiler and Programmer
Symbols in C++ provide a structured format through which instructions and logical constructs can be represented. Every time a programmer uses a symbol, they create a need for the compiler to interpret and translate that symbol into machine code, ultimately affecting how the program executes. By mastering symbols, programmers can enhance their effectiveness and efficiency in writing C++ code.
Common Symbol Errors and How to Avoid Them
Common Pitfalls: Misusing symbols can lead to syntax errors or logic errors within the code. For example, unmatched braces can confuse the compiler, leading to compilation failures or unexpected behavior.
To avoid such errors, always ensure that every opening brace `{` has a corresponding closing brace `}`, and utilize consistent formatting to enhance code readability.
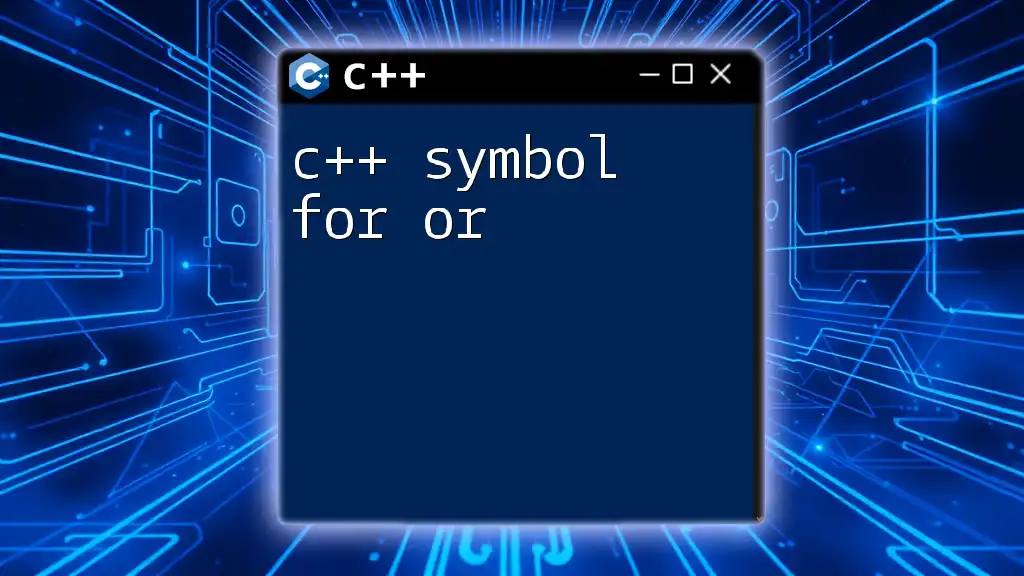
Symbol Tables in C++ Compiling Process
What Are Symbol Tables?
Symbol tables are data structures used by compilers to store information about identifiers (variables, functions, classes, etc.) and their associated attributes (data type, scope, and memory location). These tables are crucial during the compilation process as they help the compiler manage and resolve symbols effectively.
The Process of Symbol Resolution
Symbol resolution refers to the compilation stage where the compiler determines which variable or function is being referenced at any point in the code. It is essential for distinguishing between different scopes, such as global and local variables.
Example:
int globalVar; // Global identifier
void fun() {
int localVar; // Limited to function scope
// The compiler resolves both globalVar and localVar
}
In this scenario, when `globalVar` is used within `fun()`, the compiler recognizes it as the global variable, whereas `localVar` is limited to that function's scope.
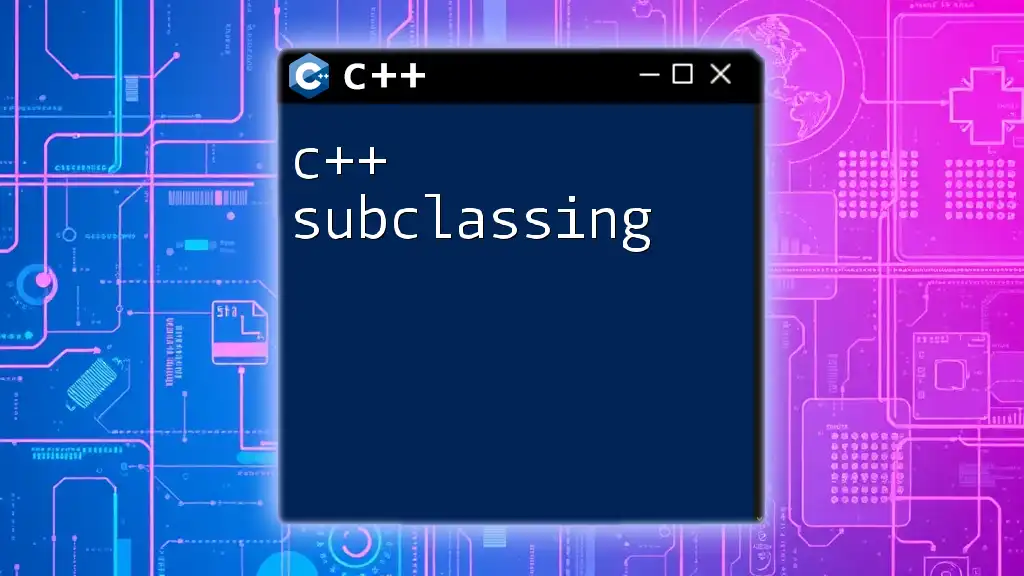
Best Practices for Using Symbols in C++
Consistent Naming Conventions
Adopting clear and consistent naming conventions for identifiers enhances code clarity and maintainability. Using meaningful names that reflect the purpose of the variable or function can significantly reduce ambiguity.
Avoiding Ambiguity
To ensure that symbols are easily understandable, avoid overloading identifiers in ways that can confuse the reader. Always prefer unique names unless necessary to overload.
Commenting on Symbol Usage
Effective commenting helps elucidate complex logic that involves multiple symbols. Regardless of how clear code may seem to the programmer, future readers or even the original programmer months down the line will appreciate contextual remarks.
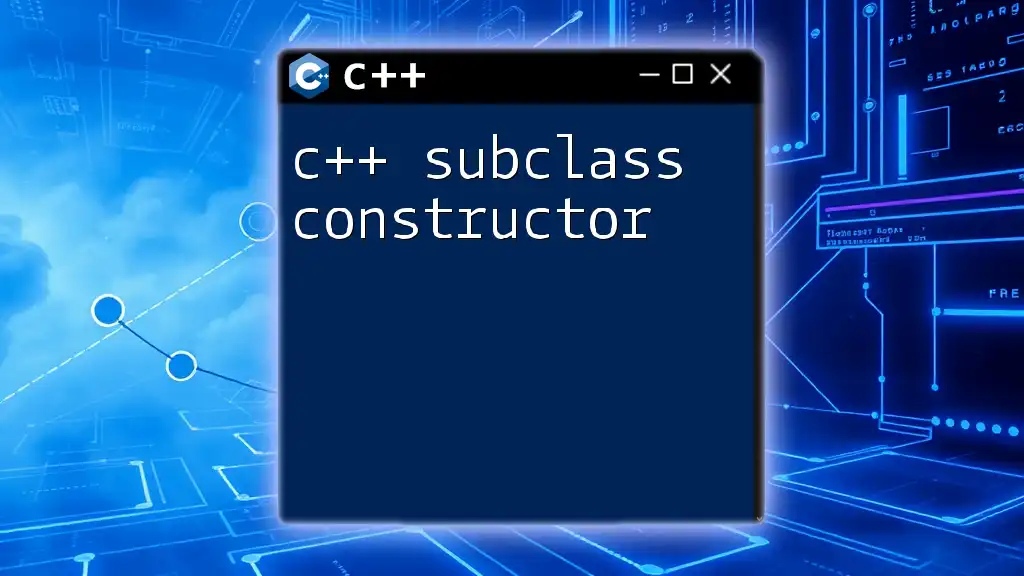
Conclusion
In summary, C++ symbols are fundamental to programming in the language, providing the tools necessary for effective communication between the programmer and the compiler. Understanding identifiers, operators, punctuation symbols, symbol tables, and best practices can enhance your coding proficiency. As you work on C++ projects, remember to apply these principles to improve clarity and functionality in your code.
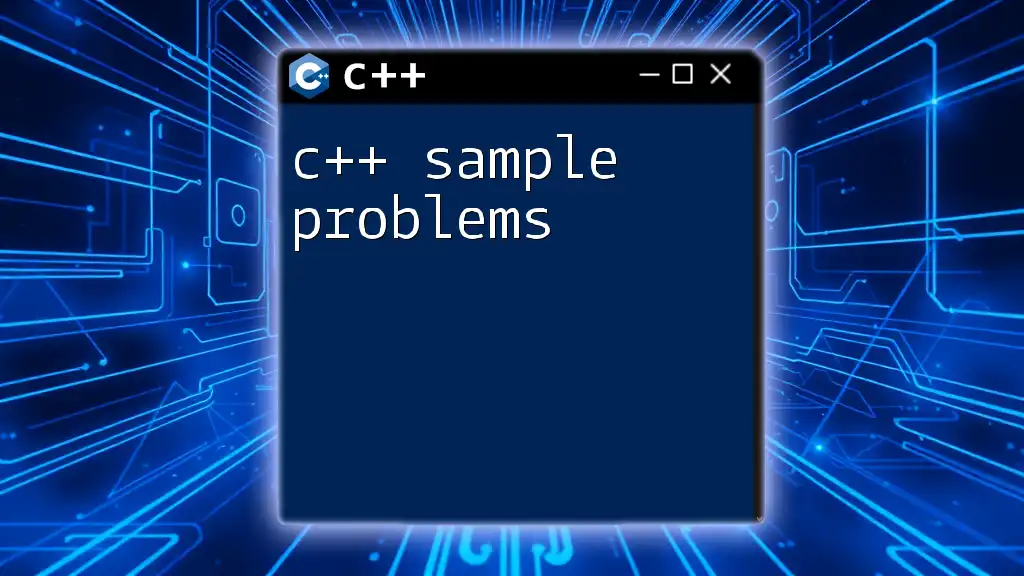
Additional Resources
To deepen your knowledge and enhance your understanding of C++ symbols:
- Seek out books focused on C++ programming and best practices.
- Engage with online courses that offer structured learning experiences.
- Utilize official C++ documentation and resources for reference on symbols and their uses.
FAQs about C++ Symbols
Have questions about the usage of symbols in C++? Here are some common queries:
- Q: Can symbols in C++ be overloaded?
A: Yes, operators can be overloaded for user-defined types, allowing for more intuitive operations on objects. - Q: What happens if I use a reserved keyword as a symbol?
A: Using reserved keywords as identifiers will lead to compilation errors, as they are defined by the language syntax.
By embracing the power of symbols in C++, you can enhance your programming skills and create robust applications with ease!