A C++ symbol table is a data structure used by a compiler to store information about identifiers, such as variables and functions, including their type and scope during the compilation process.
#include <iostream>
#include <unordered_map>
#include <string>
class SymbolTable {
public:
void insert(const std::string &name, const std::string &type) {
table[name] = type;
}
std::string lookup(const std::string &name) {
return table.count(name) ? table[name] : "Not Found";
}
private:
std::unordered_map<std::string, std::string> table;
};
int main() {
SymbolTable symTable;
symTable.insert("x", "int");
std::cout << "Type of x: " << symTable.lookup("x") << std::endl; // Output: Type of x: int
return 0;
}
What is a Symbol Table?
A C++ symbol table is a crucial data structure used by compilers to keep track of the identifiers (names) used in a program, along with their associated information such as type and scope. Essentially, it acts as a repository for all variable declarations, functions, and other identifiers present in the code. The importance of symbol tables cannot be overstated: they play a significant role in the compilation process, especially during semantic analysis when the compiler verifies the correctness of the program.
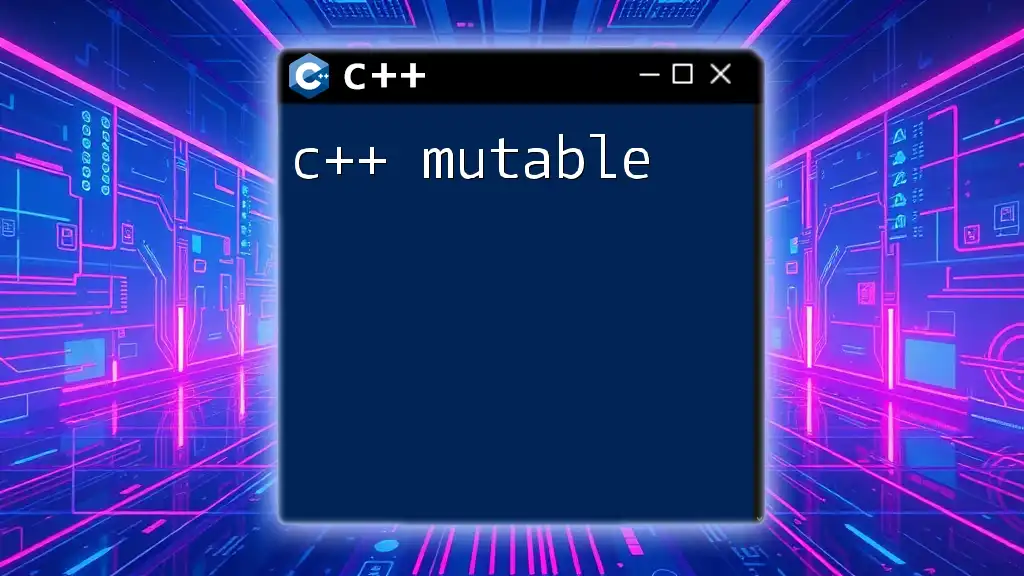
How Symbol Tables Work
Symbol tables store entries that represent each identifier in the form of a collection of attributes. These attributes typically include the name of the symbol, its type (such as int, float, or user-defined types), its value if it has been initialized, and the scope in which the identifier exists.
The scope defines where in the program the identifier is valid, while its lifetime dictates how long the identifier exists in memory. Typically, a symbol is created when a declaration is encountered and is destroyed when it goes out of scope.
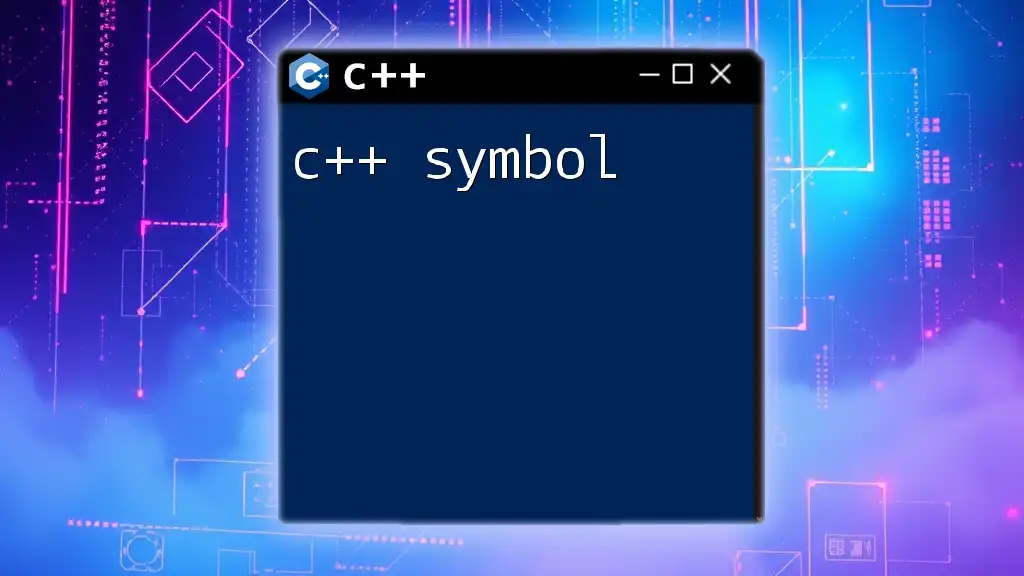
Types of Symbol Tables
Static vs Dynamic Symbol Tables
Static symbol tables are constructed at compile time, and they maintain identifiers in a fixed manner throughout the program. This can lead to faster lookups since the structure is predetermined.
Conversely, dynamic symbol tables are constructed during program execution, adapting to the variables that exist at runtime. This flexibility is useful in languages that allow dynamic typing or alter the scope and lifecycle of identifiers based on runtime conditions.
Flat vs Hierarchical Symbol Tables
In flat symbol tables, all identifiers exist at the same level, making it straightforward but potentially cumbersome in larger applications.
On the other hand, hierarchical symbol tables maintain nested scopes, allowing the same identifier name to exist in different blocks (e.g., a variable declared within a function can shadow a global variable of the same name). This structure is more suited for managing complex programs, as it respects the scope rules of the language.
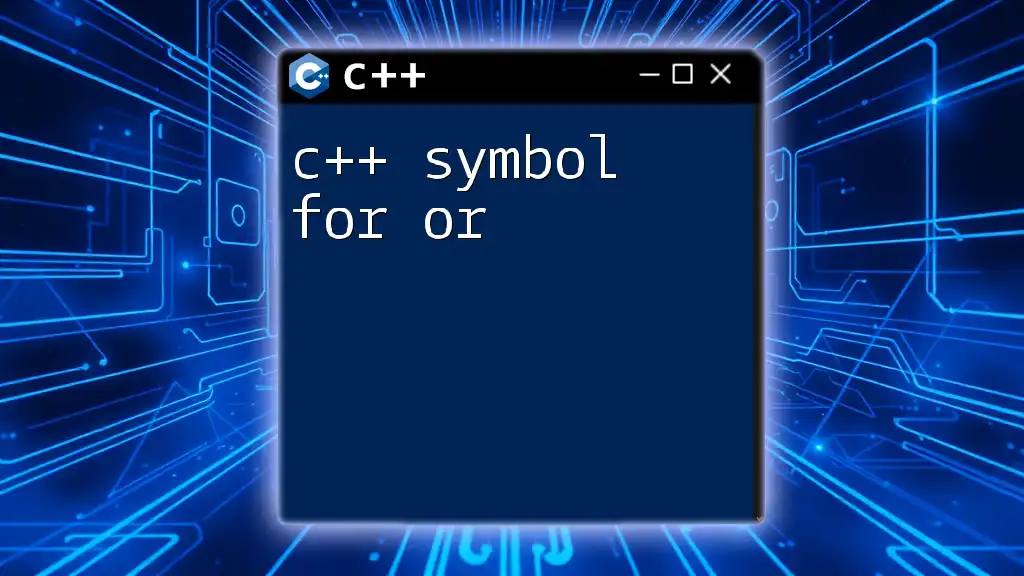
Components of a C++ Symbol Table
Key Elements
-
Names (Identifiers): These are the symbols used to refer to variables, functions, and other entities in C++. It’s essential to maintain consistency in naming conventions for better readability and maintainability.
-
Types: The type of an identifier denotes the kind of data it can hold (int, float, etc.). Understanding type definitions and conversions in C++ is crucial for ensuring type safety and efficient organization of data.
-
Values: These refer to the actual data stored in variables. C++ allows both initialized and uninitialized variables, and handling these correctly in the symbol table is fundamental.
Entry Structure
An entry in a symbol table might be represented structurally as follows:
struct SymbolEntry {
std::string name;
std::string type;
void* value; // Using void* to allow different data types
};
This structure allows the symbol table to encapsulate essential information about each identifier.
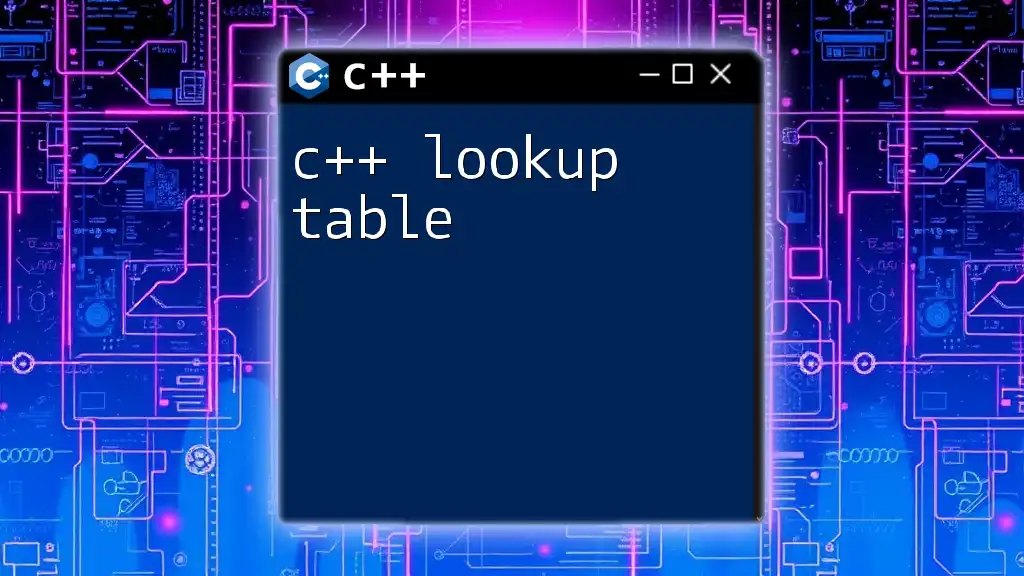
Creating a Simple Symbol Table in C++
Building Blocks of the Symbol Table
To create a C++ symbol table, it’s important to select suitable data structures. Common choices include arrays, linked lists, or hash maps. Hash maps are often preferred due to their efficiency in lookups.
Sample Implementation
Let’s walk through a simple implementation of a symbol table using a hash map.
#include <unordered_map>
#include <string>
class SymbolTable {
private:
std::unordered_map<std::string, SymbolEntry> table;
public:
void insert(const std::string& name, const std::string& type, void* value) {
SymbolEntry entry;
entry.name = name;
entry.type = type;
entry.value = value;
table[name] = entry; // Insert the entry into the table
}
SymbolEntry lookup(const std::string& name) {
if (table.find(name) != table.end()) {
return table[name]; // Return the found entry
}
throw std::runtime_error("Symbol not found!"); // Handle errors gracefully
}
};
Explanation of Methods
The `insert` method adds a new symbol to the table, while the `lookup` method retrieves a symbol by its name. If the symbol is not found during a lookup, an exception is thrown, providing clear feedback for debugging.
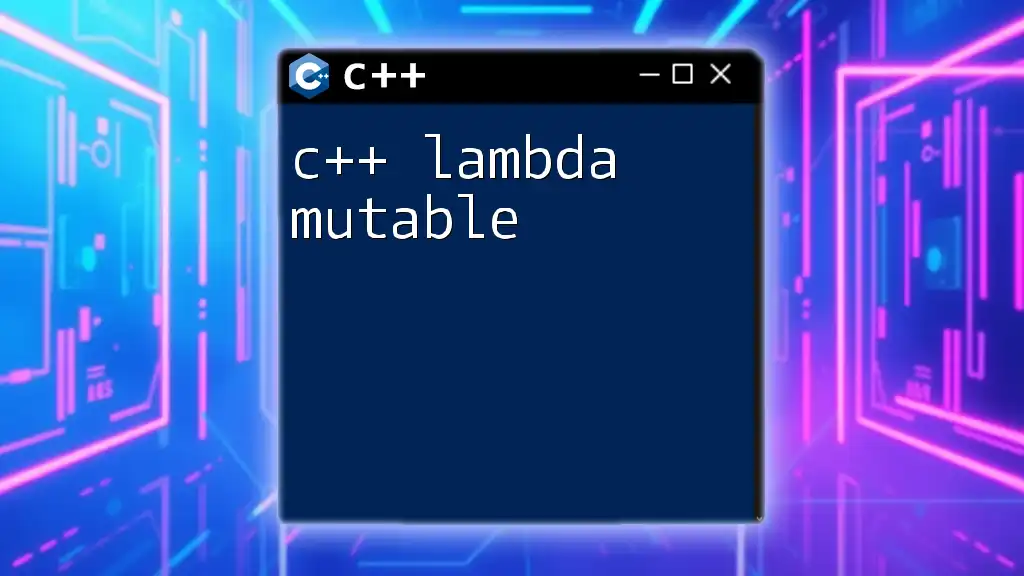
Scope and Lifetime in Symbol Tables
Understanding Scope
Scope in programming defines the region where identifiers are accessible. C++ has both local and global scopes. Local variables are only accessible within the block they are declared in, while global variables can be accessed from any part of the program.
Lifetime of Symbols
The lifetime of a symbol dictates how long it exists in memory. C++ supports static and dynamic memory allocation:
-
Static memory allocation occurs at compile time, where the lifetime of variables persists throughout the program execution.
-
Dynamic memory allocation allows for creating variables during runtime. Understanding how memory is managed here is critical for proper identifier usage.
Consider this example demonstrating local variable lifetime:
void exampleFunction() {
int x = 10; // local variable x
} // x goes out of scope here
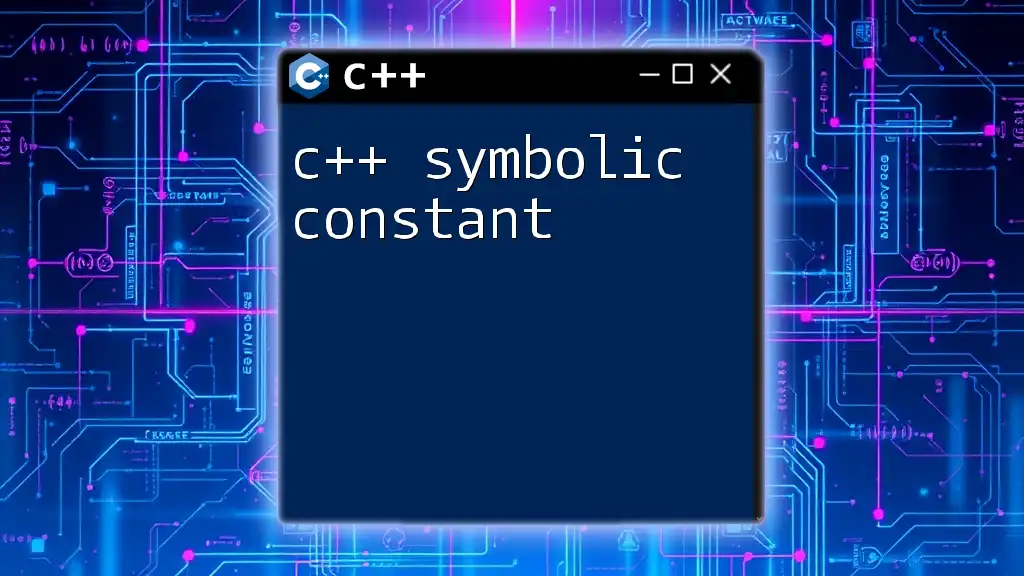
Symbol Table and Compiler Design
Role of Symbol Tables in Compilers
A C++ symbol table is integral to the compilation process, providing a reference point for the compiler to validate identifiers and their associated attributes. It supports the semantic analysis phase by ensuring that identifiers are declared before use and adhere to their assigned types.
Phases of Compilation Involving Symbol Tables
During the compilation process, the lexer generates tokens that often represent identifiers. The parser uses the symbol table to ensure that each identifier is valid according to the syntax and semantic rules defined for the program.
Throughout the code generation phase, the symbol table provides necessary details for generating machine code or intermediate code by mapping identifiers to memory addresses or registers.
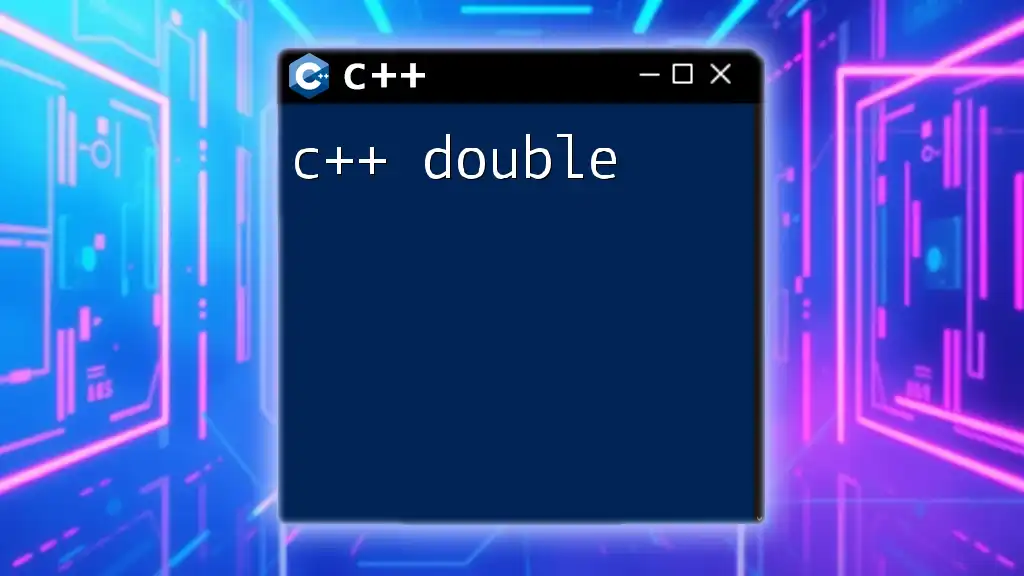
Error Handling and Debugging
Common Errors Related to Symbol Tables
When working with symbol tables, developers may encounter several common errors:
- Duplicate definitions: Attempting to declare the same identifier multiple times within the same scope can lead to compilation errors.
- Undeclared identifiers: Using a variable that has not been declared beforehand will generate linker errors.
Debugging Techniques
To effectively troubleshoot symbol table-related issues, consider using logging or debugging print statements. This can provide insight into the current state of the symbol table, helping identify where a lookup may have failed or whether an identifier has been correctly inserted.
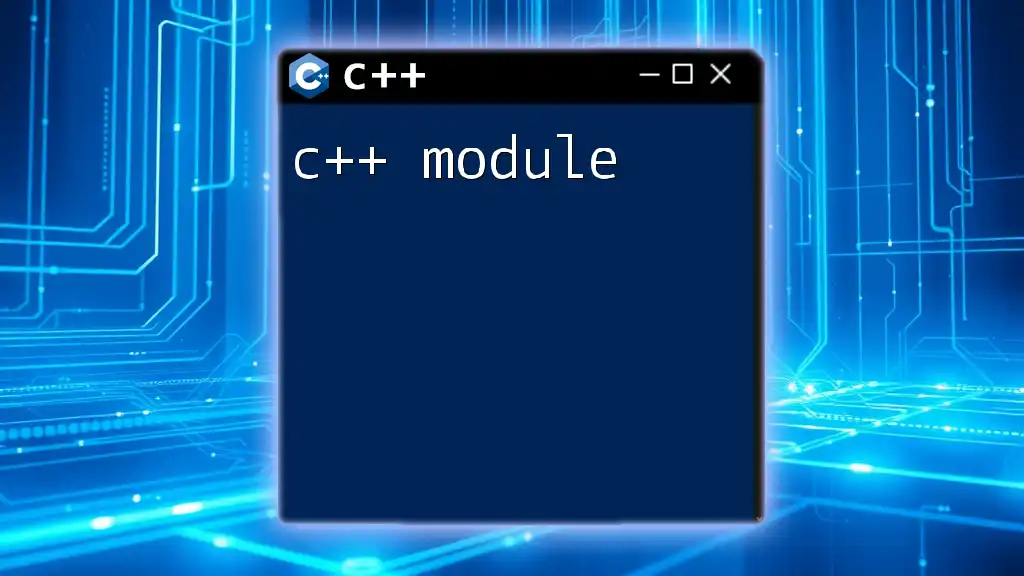
Best Practices for Managing Symbol Tables
Optimal Data Structures
Selecting the right data structure for your symbol table is crucial for performance. For example, while a list can work for small projects, hash maps generally offer superior efficiency for lookups in larger programs.
Code Organization
Keep your symbol table code organized and modular. Clearly define the interface for interacting with the symbol table and ensure that your implementation is easy to extend or modify. Comment heavily to describe the function and purpose of various components in your code.
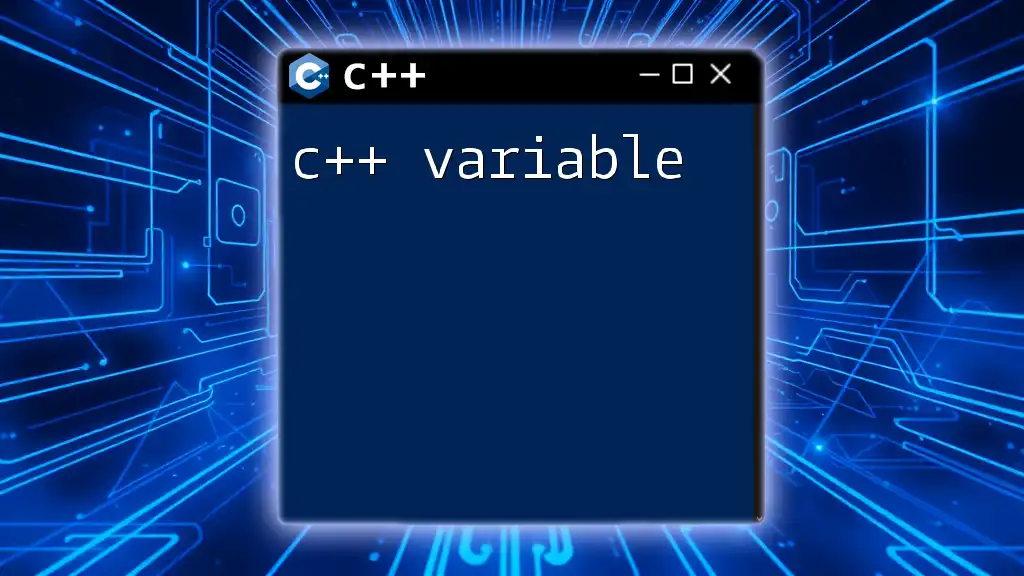
Conclusion
The C++ symbol table plays a pivotal role in the compilation process, facilitating the semantic understanding of identifiers in the program. Understanding how to effectively implement and manage a symbol table is a core skill for any C++ programmer aiming to develop robust, error-free code. For those interested in deepening their understanding of C++ and symbol tables, various resources are available, including textbooks, online courses, and coding communities.
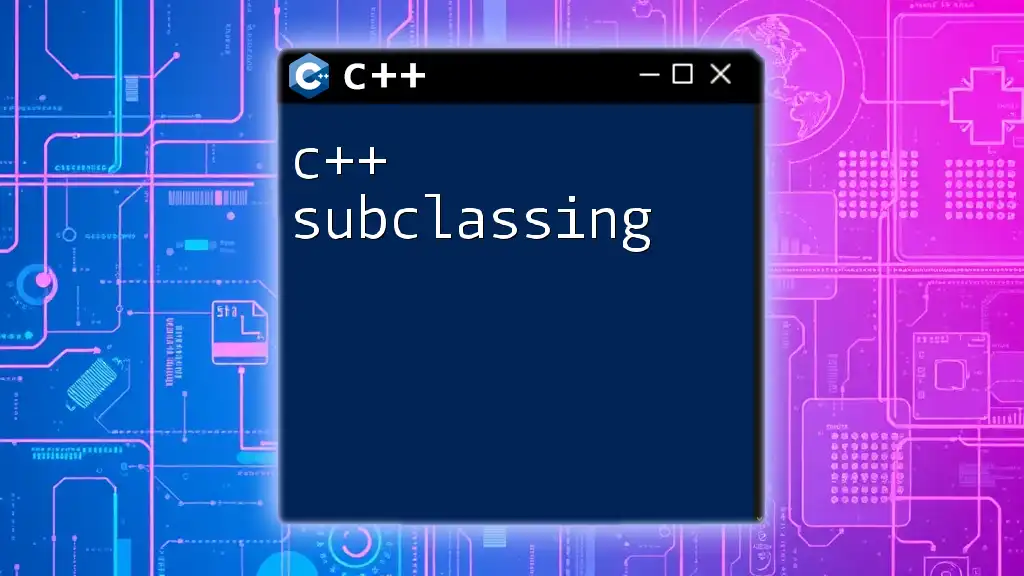
Call to Action
Join our C++ learning community today to explore further insights and discussions on effectively navigating the world of C++. Together, we can enhance our programming skills and tackle challenges with confidence!