In C++, the symbol for logical OR is `||`, which is used to combine two boolean expressions, returning true if at least one of the expressions evaluates to true.
Here’s an example:
#include <iostream>
int main() {
bool a = true;
bool b = false;
if (a || b) {
std::cout << "At least one condition is true." << std::endl;
}
return 0;
}
Understanding the C++ OR Symbol
Definition of the OR Symbol in C++
In C++, the OR symbol is represented by two distinct operators: the logical OR operator (`||`) and the bitwise OR operator (`|`). Understanding the difference between these two symbols is crucial for effective programming.
- Logical OR (`||`): This operator is used to combine two boolean expressions. The result is `true` if at least one of the expressions evaluates to true.
- Bitwise OR (`|`): This operator performs a bitwise comparison of two integers. It compares corresponding bits of the operands and returns a new integer where each bit is set to `1` if at least one of the original bits is `1`.
Syntax of the OR Symbol
The syntax of the OR operators is straightforward, but understanding where and how to use them is essential for logical reasoning in your code.
With logical OR (`||`), the syntax is typically found in conditional statements, such as in `if` statements:
if (condition1 || condition2) {
// Execute code if either condition is true
}
For the bitwise OR (`|`), you would see it used in expressions involving integers:
int result = a | b; // Combines bits of a and b
Knowing how to effectively use these operators requires an understanding of their precedence and associativity. Logical OR has lower precedence than logical AND (`&&`), meaning that AND operations are evaluated first unless parentheses are used to specify order.
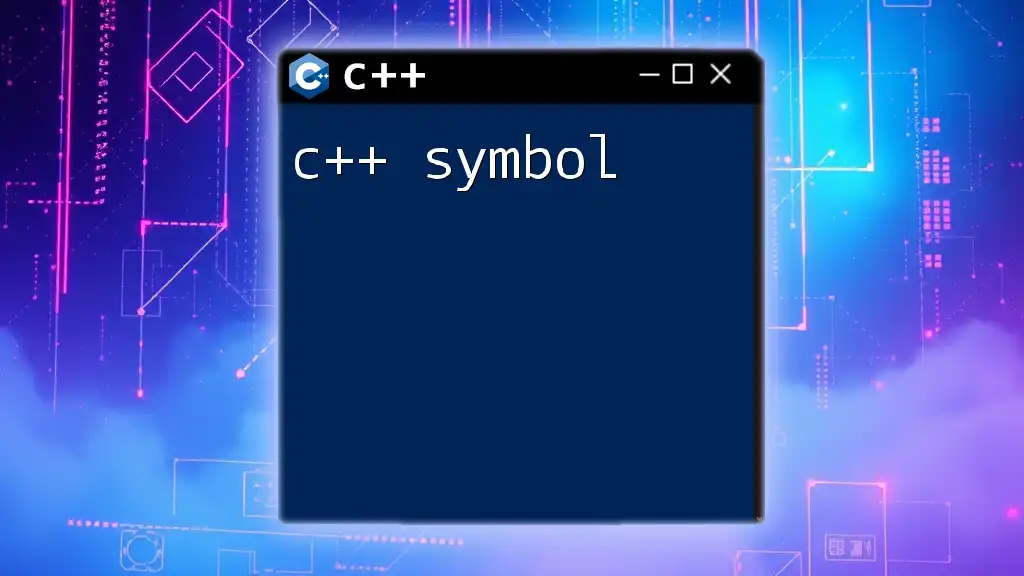
Practical Applications of the OR Symbol
Using the Logical OR Operator (`||`)
The logical OR operator (`||`) is prevalent in conditional logic. It enables the combination of multiple conditions, allowing developers to execute code based on various scenarios.
For instance, consider the code snippet below, where we want to decide whether to go outside based on the weather and the day of the week:
bool isSunny = true;
bool isWeekend = false;
if (isSunny || isWeekend) {
std::cout << "Let's go outside!" << std::endl;
}
In the above example, since one of the conditions (`isSunny`) is `true`, the message is printed. This use of `||` allows for flexible conditions, helping to avoid long and convoluted `if` statements.
Using the Bitwise OR Operator (`|`)
The bitwise OR operator (`|`) plays a crucial role in bit manipulation. This is particularly useful in systems programming, graphics processing, and scenarios where performance optimization is key.
Consider the following example:
int a = 5; // 0101 in binary
int b = 3; // 0011 in binary
int result = a | b; // results in 0111, which is 7
std::cout << "Bitwise OR result: " << result << std::endl;
Here, each bit is evaluated and combined: if either bit is `1` in `a` or `b`, the corresponding bit in the result is set to `1`. This allows developers to manipulate individual bits effectively.
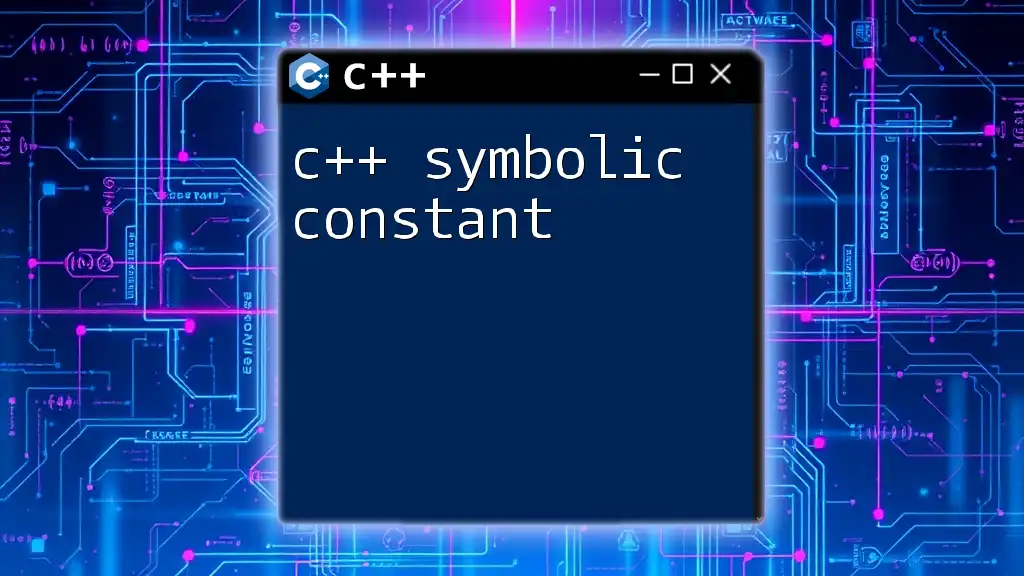
Common Mistakes with the OR Symbol
Logical vs. Bitwise Confusion
A common error for many developers is conflating the logical and bitwise OR operators. While both are used predominantly in conditional statements, their outcomes differ significantly.
Using `|` in a conditional expression without realizing it may lead to unintended consequences. Ensure to choose `||` when you are working with boolean conditions and `|` when performing bitwise operations.
Short-Circuiting Behavior
One of the fundamental characteristics of the logical OR operator (`||`) is its short-circuit evaluation. This means if the first condition evaluates to `true`, the second condition will not be checked. This can prevent unnecessary computations and potential errors.
For instance:
int x = 0;
if (x != 0 || (10 / x > 1)) {
// This will not throw a division by zero error
}
In this example, the second condition is skipped because the first condition is `false`. Short-circuiting can optimize performance and reduce the risk of runtime errors in your code.
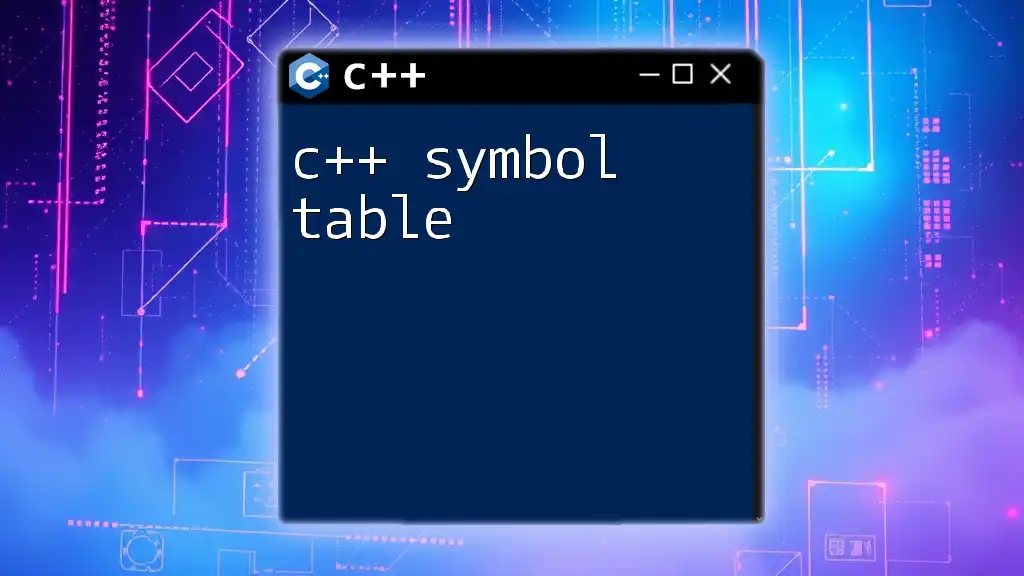
Combining with Other Logical Operators
The Complete Picture
In practice, conditions often involve more than just the OR operator. It’s essential to know how to combine different logical operators such as AND (`&&`) and NOT (`!`) for more robust conditions.
Consider the following complex statement that uses these operators:
bool condition1 = true;
bool condition2 = false;
if (condition1 || (!condition2 && condition1)) {
std::cout << "Complex condition is true!" << std::endl;
}
In this scenario, the code evaluates multiple conditions using both `||` and `&&`, demonstrating how to architect complex logical scenarios effectively.
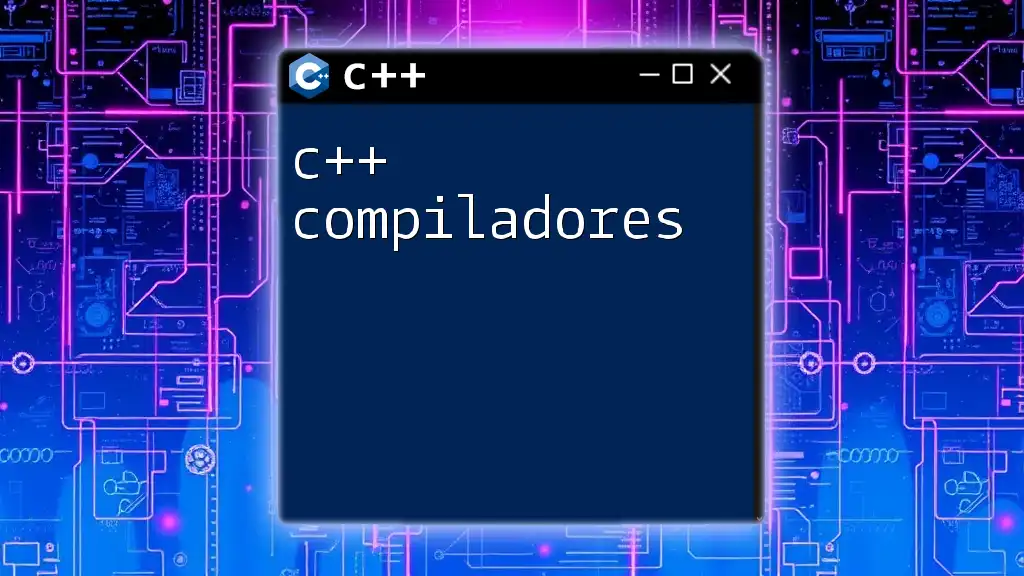
Best Practices for Using the OR Symbol in C++
Code Readability and Maintainability
When using the OR symbol in your code, prioritize readability. Using parentheses can improve clarity significantly:
if ((condition1 || condition2) && condition3) {
// This expression is clearer and less error-prone
}
Establishing consistent variable naming conventions also aids in maintaining clean code. Descriptive names make it easier to understand the conditions being evaluated.
Testing and Debugging Conditional Statements
Effective testing of conditional statements is vital. Consider writing unit tests that cover various combinations of boolean values. This will help identify edge cases and ensure all logical paths are functioning correctly.
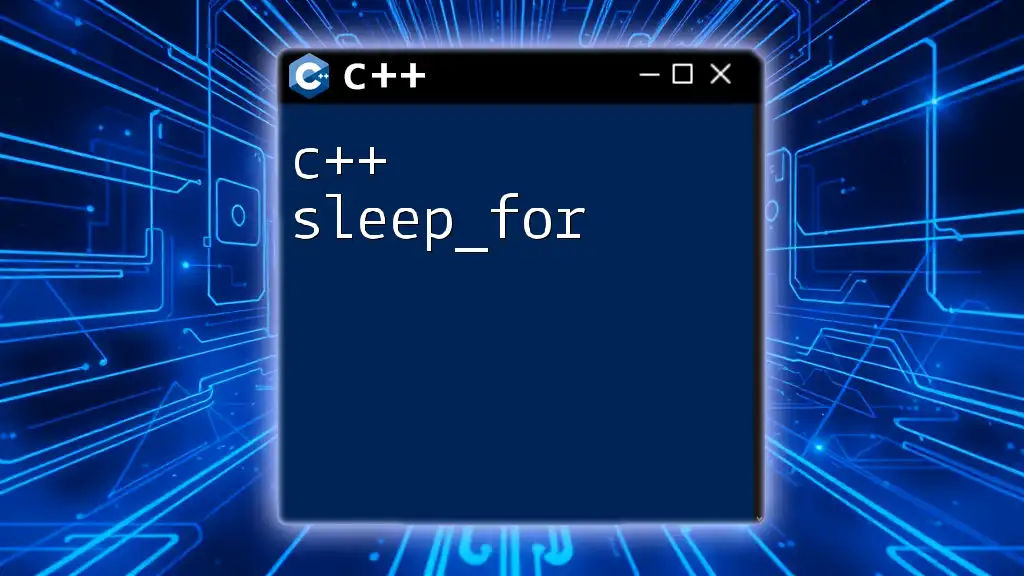
Conclusion
In summary, the C++ symbol for OR is a powerful component of the language that allows developers to construct intricate logical expressions efficiently. By mastering the distinctions between the logical OR (`||`) and bitwise OR (`|`), you can enhance your programming skills significantly. Practice regularly by implementing what you've learned, and soon you'll see improvement in the clarity and effectiveness of your C++ code.
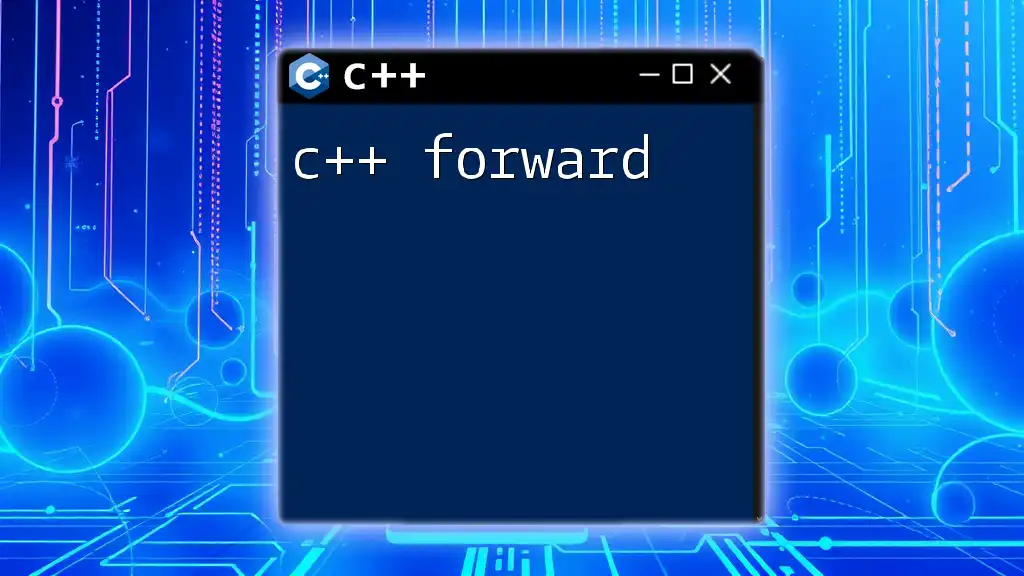
Additional Resources
For those looking to delve deeper, explore books and online platforms that offer exercises, challenges, and a community for discussing C++ programming. Engaging with these materials will solidify your understanding and application of the OR symbol in various contexts.