A C++ nested for loop is a loop inside another loop, allowing you to iterate over multi-dimensional data structures or perform repetitive tasks in a compact manner.
Here’s an example of a nested for loop in C++:
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 2; j++) {
cout << "i: " << i << ", j: " << j << endl;
}
}
return 0;
}
Understanding the Structure of a Nested Loop
What is a Nested Loop?
A nested loop is simply a loop inside another loop. This structure allows for multiple levels of iteration, which is particularly useful when working with multidimensional data structures such as arrays or when performing repetitive tasks that require more than one iteration variable. The outer loop controls the iteration for the first element, while the inner loop executes all iterations for each outer loop iteration.
Visual Representation of Nested Loops
When visualizing how nested loops operate, it helps to think in terms of layers. Imagine the outer loop as a larger container that governs how many times a specific process (the inner loop) will be repeated. During each iteration of the outer loop, the inner loop executes fully.
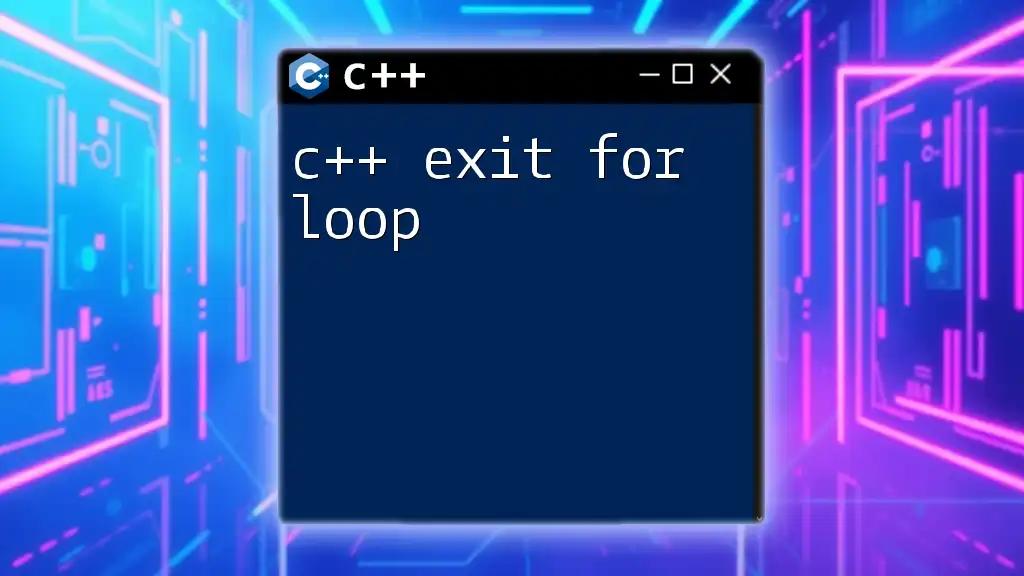
Syntax of a Nested For Loop
Basic Syntax
To construct a nested for loop, you begin with the outer loop followed by the inner loop. Here is how the syntax looks:
for (initialization; condition; increment) {
for (initialization; condition; increment) {
// Code block for inner loop
}
}
This basic structure establishes that for every complete iteration of the outer loop, the inner loop runs through all its iterations.
Example of a Simple Nested For Loop
Let’s look at a concrete example to clarify how this works:
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 2; j++) {
cout << "i: " << i << ", j: " << j << endl;
}
}
return 0;
}
In this example, the outer loop (controlled by `i`) iterates three times. Within each of these iterations, the inner loop (controlled by `j`) iterates twice. Thus, when this code runs, it will print out the various combinations:
i: 0, j: 0
i: 0, j: 1
i: 1, j: 0
i: 1, j: 1
i: 2, j: 0
i: 2, j: 1
This output illustrates how the inner loop executes fully for each iteration of the outer loop.
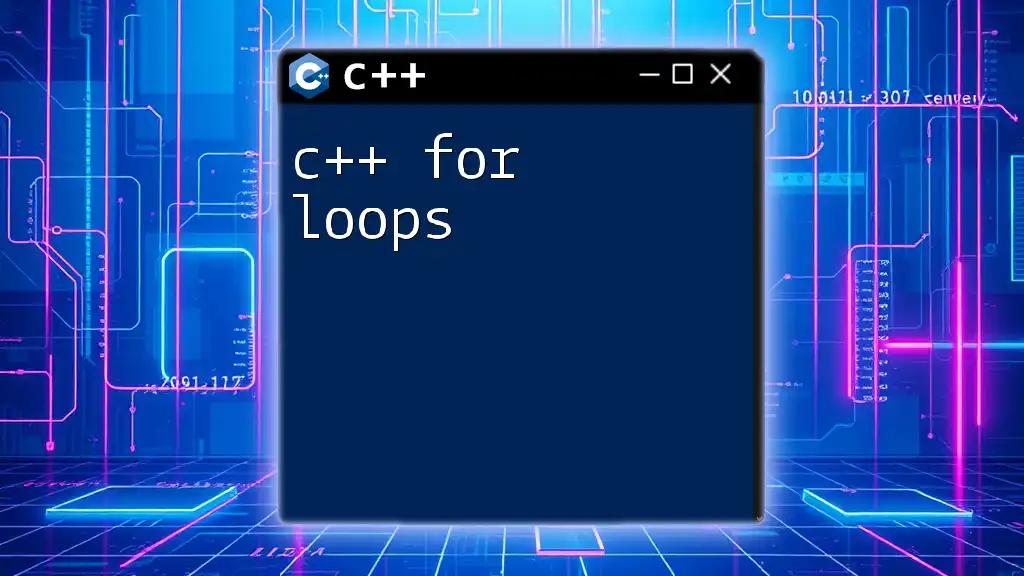
Practical Applications of Nested For Loops
Working with Multidimensional Arrays
One of the most common applications of nested loops is traversing multidimensional arrays, such as a matrix. Here’s a simple example demonstrating this:
#include <iostream>
using namespace std;
int main() {
int arr[2][2] = {{1, 2}, {3, 4}};
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 2; j++) {
cout << arr[i][j] << " ";
}
cout << endl;
}
return 0;
}
In this code, the outer loop iterates through the rows of the array while the inner loop processes the elements of each row. The output would be:
1 2
3 4
This example highlights an essential skill in programming: iterating through data structures to extract or manipulate their contents.
Creating Patterns with Nested Loops
Nested loops are also useful when generating patterns. For instance, consider creating a simple diamond shape:
#include <iostream>
using namespace std;
int main() {
int rows = 5;
for (int i = 0; i < rows; i++) {
for (int j = 0; j <= i; j++) {
cout << "* ";
}
cout << endl;
}
return 0;
}
When you run this code, the output looks like this:
*
* *
* * *
* * * *
* * * * *
This output illustrates how the number of `` characters per line corresponds directly to the current iteration of the outer loop. Each new iteration results in an additional ``, forming a triangular pattern.
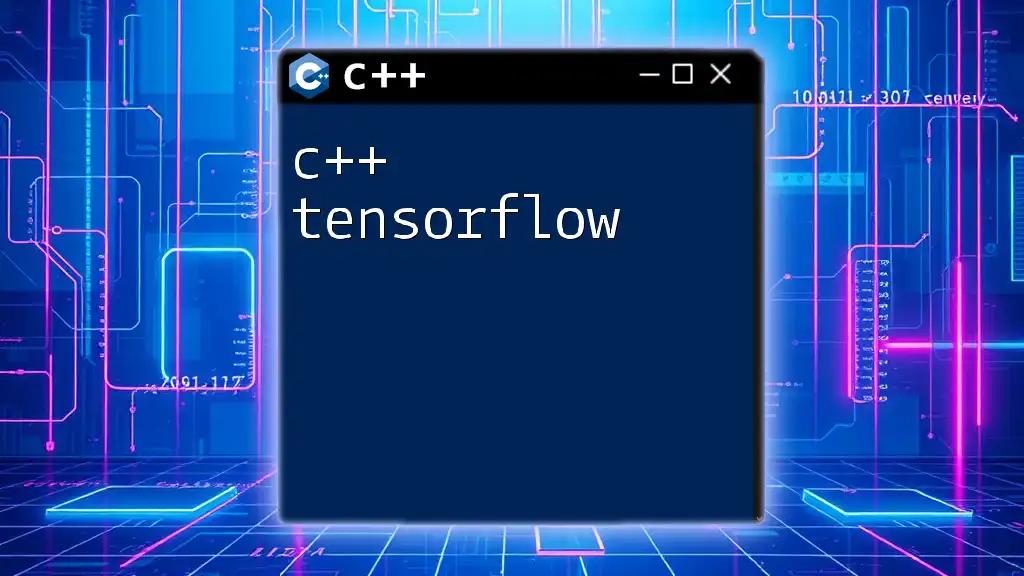
Performance Considerations when Using Nested Loops
Time Complexity
Understanding the performance implications of nested loops is crucial. Each level of nesting multiplies the time complexity of your code. For instance, consider a nested for loop that runs `n` times in the outer loop and `m` times in the inner loop. The overall time complexity would be O(n * m). This means that as the size of your dataset increases, the time taken to execute your code will increase multiplicatively, which can drastically affect performance.
Best Practices
To optimize the performance of your code when using nested loops, consider the following best practices:
- Minimize the Number of Iterations: Where feasible, ensure you are only looping through necessary elements.
- Utilize Break Statements: If a certain condition is met, and further iterations are unnecessary, use `break` to terminate the innermost loop early.
- Consider Alternative Algorithms: Often, restructuring your loop conditions or using more advanced algorithms, like sorting data beforehand, can yield more efficient results.
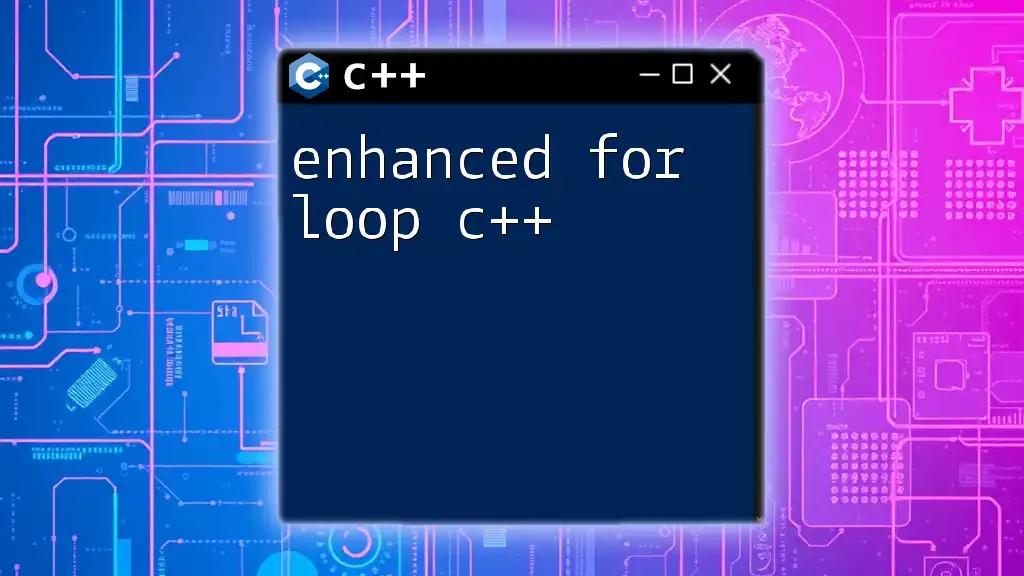
Common Mistakes and How to Avoid Them
Off-by-One Errors
One of the most frequent pitfalls in using nested loops is the off-by-one error, where loop boundaries are defined incorrectly. For example:
// Common mistake
for (int i = 0; i <= n; i++) { // Incorrect condition
// Some code
}
In this snippet, if `n` represents the size of an array, the loop will attempt to access an out-of-bounds index on its final iteration, leading to a potential runtime error. Always double-check loop conditions to ensure you are iterating correctly through your data.
Infinite Loops
Another risk is creating infinite loops. These occur when loop conditions never allow the loop to terminate. For example, missing or incorrect increment statements within a nested loop can cause an infinite cycle, which can hang your program.
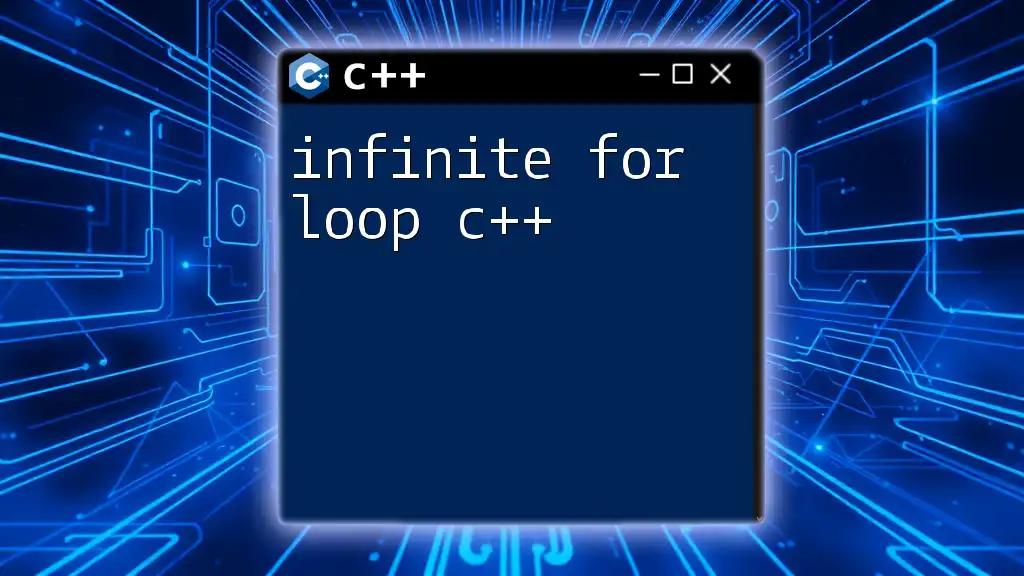
Conclusion
Nested for loops are a foundational concept in C++ programming, enabling complex iterations and handling multidimensional data structures efficiently. Understanding their syntax, practical applications, performance considerations, and common pitfalls is essential for any programmer aiming to master C++. As you practice, try creating your own examples and experimenting with variations. This hands-on experience will strengthen your grasp of this powerful tool in your programming toolkit.
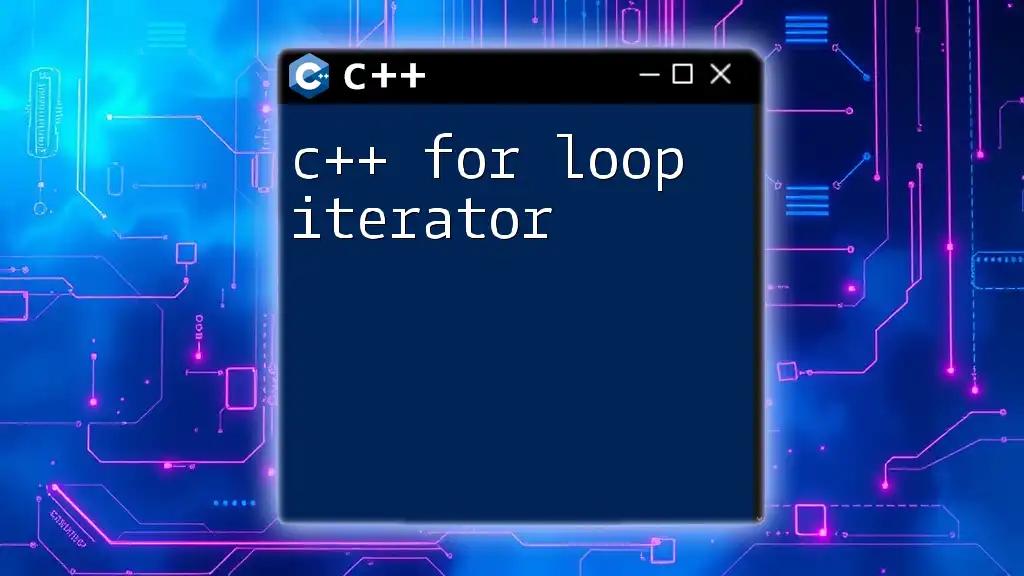
Additional Resources
To expand your knowledge on C++ nested for loops and loops in general, consider diving into books, online tutorials, and official documentation. Engaging with communities like Stack Overflow or specific C++ forums can also provide valuable insights and additional practice opportunities.