In C++, the `pop_back()` function is used to remove the last element from a `vector`, effectively decreasing its size by one.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.pop_back(); // Removes the last element (5)
std::cout << "Last element removed. New size: " << vec.size() << std::endl; // Outputs: New size: 4
return 0;
}
What is a C++ Vector?
C++ vectors are a part of the Standard Template Library (STL) and provide a dynamic array-like data structure. Vectors are capable of growing and shrinking in size dynamically, which makes them highly versatile compared to traditional arrays.
Definition and Purpose
Vectors are defined in the `<vector>` header and can store data of a single type, allowing for the storage of multiple elements in a contiguous memory space. One of the key strengths of vectors is their ability to manage storage automatically, meaning you can focus on using them without worrying about memory management issues.
Basic Operations on Vectors
Vectors come with a host of operations, including:
- `push_back(value)`: Adds a new element to the end of the vector.
- `size()`: Returns the current number of elements in the vector.
- `empty()`: Checks if the vector is empty.
These operations make vectors more flexible and inherently easier to work with compared to static arrays.

Understanding the pop_back Function
Definition
The `pop_back` function is designed to remove the last element from a vector. This operation effectively reduces the size of the vector by one, but it does not return any value.
Syntax of pop_back in C++
The syntax for the `pop_back` method in C++ is straightforward:
void pop_back();
Key Characteristics
- No Return Value: Unlike some other functions, `pop_back` does not return the value of the removed element.
- Undefined Behavior: If `pop_back` is called on an empty vector, it results in undefined behavior. Therefore, it is crucial to check whether the vector is empty before invoking this function.
- Impacts on Vector Capacity: While `pop_back` reduces the size of the vector, it does not necessarily reduce its capacity. This means the memory allocated for the vector may still be larger than necessary.
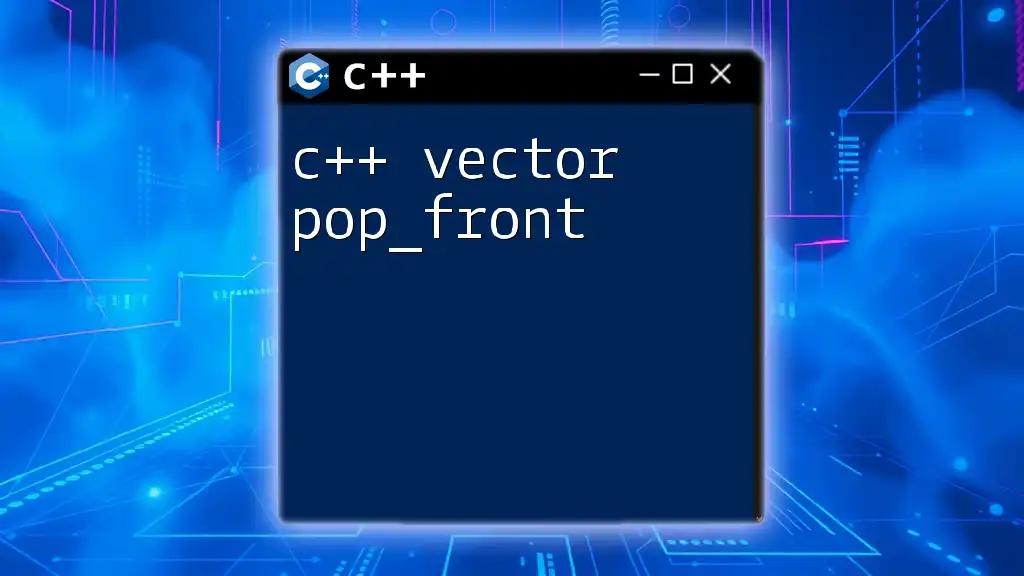
How to Use pop_back
Basic Example
Here’s a simple example demonstrating the use of `pop_back`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.pop_back();
// Now numbers contains {1, 2, 3, 4}
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
In the example above, the `pop_back` method removes the last element (5) from the `numbers` vector. The final output will display the remaining elements, showcasing the effectiveness of `pop_back`.
Common Use Cases
- Removing the Last Element: Use `pop_back` when you need to discard the most recently added item.
- Managing Game States: In game development, `pop_back` can help manage player actions, allowing for an easy "undo" functionality.
- Implementing Stacks: Vectors can simulate stack behavior with the combination of `push_back` and `pop_back`.
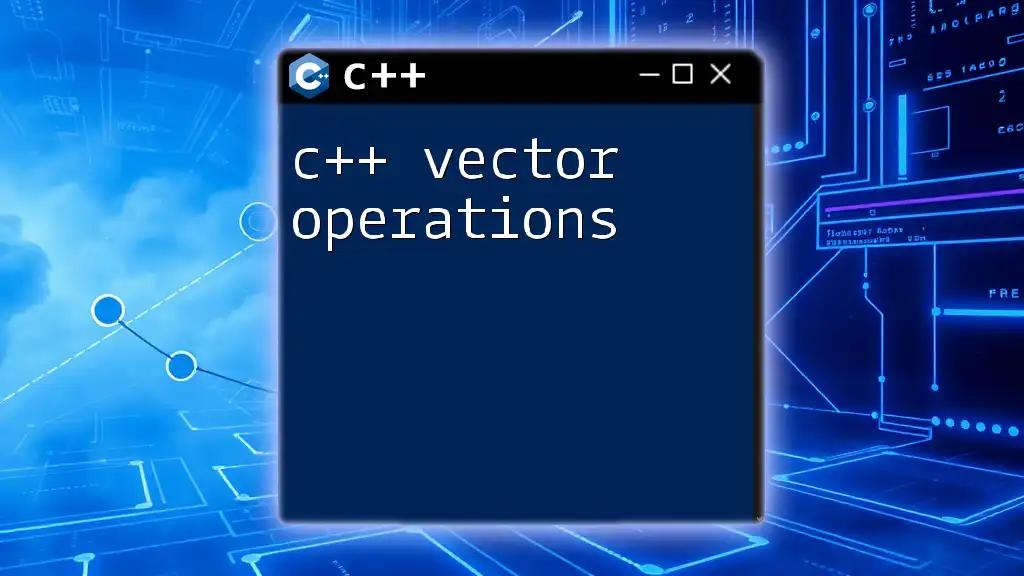
Best Practices for Using pop_back
Error Handling
Always check if the vector is empty before calling `pop_back` to avoid undefined behavior:
if (!numbers.empty()) {
numbers.pop_back();
}
Performance Considerations
The `pop_back` operation has a time complexity of O(1), which means it operates in constant time, making it efficient even in performance-sensitive applications. However, keep in mind the possible memory implications when performing a large number of operations that might lead to frequent dynamic memory reallocations.
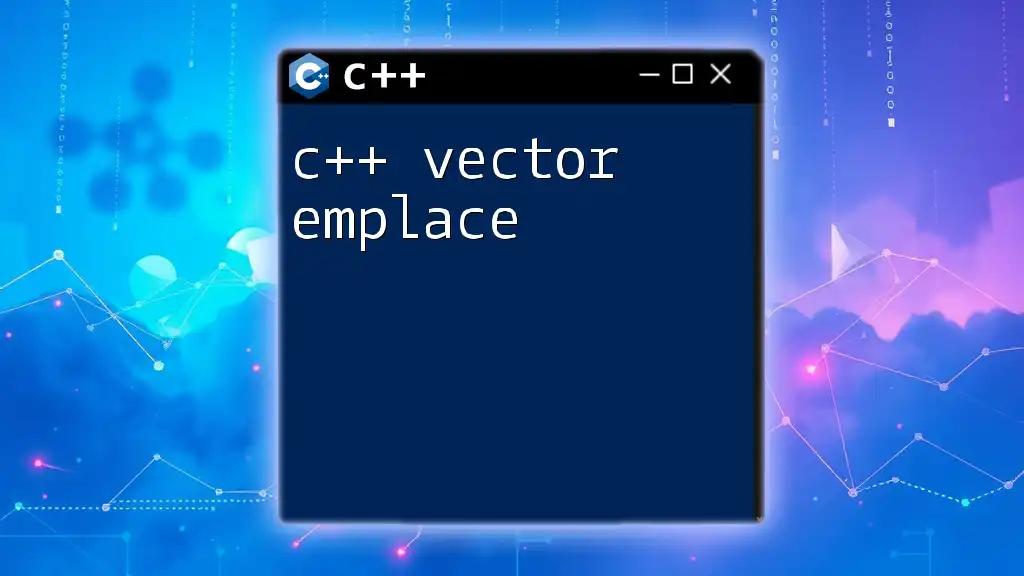
Comparing pop_back with Other Vector Functions
Pop vs. Other Modifying Functions
While both `pop_back` and `erase` can remove elements from a vector, they differ in functionality. The `erase` function can remove elements from any position in the vector, while `pop_back` specifically targets the last element.
Example of using `erase`:
numbers.erase(numbers.end() - 1); // Removes last element.
Choose `pop_back` when you specifically need to remove the last element, as it is more efficient for that operation.
Combination of pop_back with Other Functions
`pop_back` can be effectively used in loops or conditional statements to control the flow of your program:
while (!numbers.empty()) {
std::cout << "Removing: " << numbers.back() << std::endl;
numbers.pop_back();
}
This loop continues to remove elements until the vector is empty, demonstrating the synergy between `back()` and `pop_back`.

Real-World Applications of pop_back in C++
Using pop_back in Data Structures
You can leverage `pop_back` when implementing a stack using vectors. Here’s a simple stack implementation:
class Stack {
public:
void push(int value) { data.push_back(value); }
void pop() { if (!data.empty()) data.pop_back(); }
private:
std::vector<int> data;
};
In this example, the `pop()` method allows for the removal of the last element added to the stack, mimicking the Last In, First Out (LIFO) principle.
Gameplay Scenarios
In a turn-based strategy game, you might record player moves using a vector. To implement an undo feature, you can simply `pop_back` the last action taken, effectively reverting to the previous state. This is a practical application of `pop_back` that enhances user experience.

Conclusion
In summary, the `pop_back` function is a powerful method for managing elements within C++ vectors. By understanding its operation, characteristics, and best practices, developers can leverage this function effectively in various programming scenarios. With practice, mastering the `pop_back` function can significantly enhance your capabilities in C++ programming, particularly in dynamic data handling and control flow management.

Additional Resources
Suggested Further Reading
Consider exploring books such as "C++ Primer" by Lippman, et al., or online courses on platforms like Coursera and Udemy focusing on C++ and STL for a deeper understanding of vectors and their operations.
Online Communities and Forums
Engaging with online communities such as Stack Overflow, Reddit, and GitHub allows you to ask questions, share experiences, and learn from other C++ enthusiasts.