In C++, you can convert a `std::vector` to an array by using the `data()` method of the vector, which returns a pointer to the underlying array.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
int* arr = vec.data(); // Convert vector to array
for (size_t i = 0; i < vec.size(); ++i) {
std::cout << arr[i] << " "; // Accessing elements via the array
}
return 0;
}
Understanding Vectors in C++
A vector in C++ is a dynamic array that allows for flexibility in managing a collection of elements. It is provided by the Standard Template Library (STL) and can grow or shrink in size as needed, making it an excellent choice for various programming situations.
Key Characteristics of Vectors
- Resizing Capabilities: Unlike traditional arrays, vectors can automatically resize. When the current capacity is exceeded, a vector can allocate a larger memory block and move existing elements to this new location.
- Usage: To utilize vectors, the `#include <vector>` directive is required, and they can be created simply as `std::vector<Type> vectorName;`.
Common Use Cases for Vectors
Vectors are advantageous when:
- You need a collection that changes in size.
- You want to utilize STL algorithms for efficient data manipulation.
- You wish to minimize memory management concerns, as vectors handle this automatically.
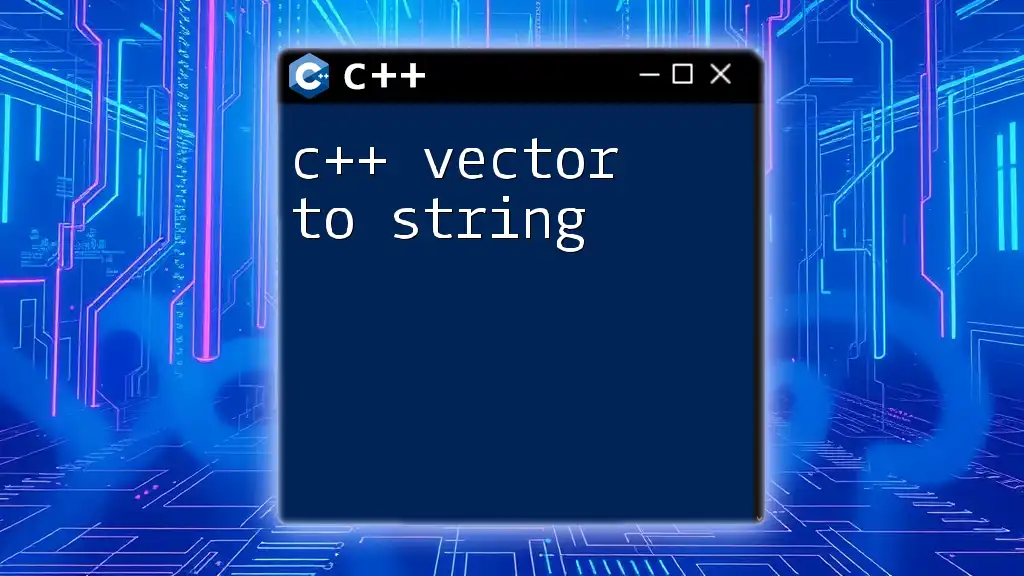
Understanding Arrays in C++
An array in C++ is a fixed-size, contiguous block of memory that holds a collection of variables of the same data type. The size of an array must be defined at compile time, which means it cannot be changed during program execution.
Differences Between Vectors and Arrays
- Size Limitations: Arrays have a fixed size, while vectors can dynamically resize.
- Memory Management: Vectors manage memory automatically, while arrays require manual handling.
- Safety: Vectors provide built-in features like bounds checking, reducing potential errors.
Common Use Cases for Arrays
Arrays are ideal when:
- The number of elements is known and will not change.
- Performance is critical, such as when working with large datasets in performance-sensitive algorithms.
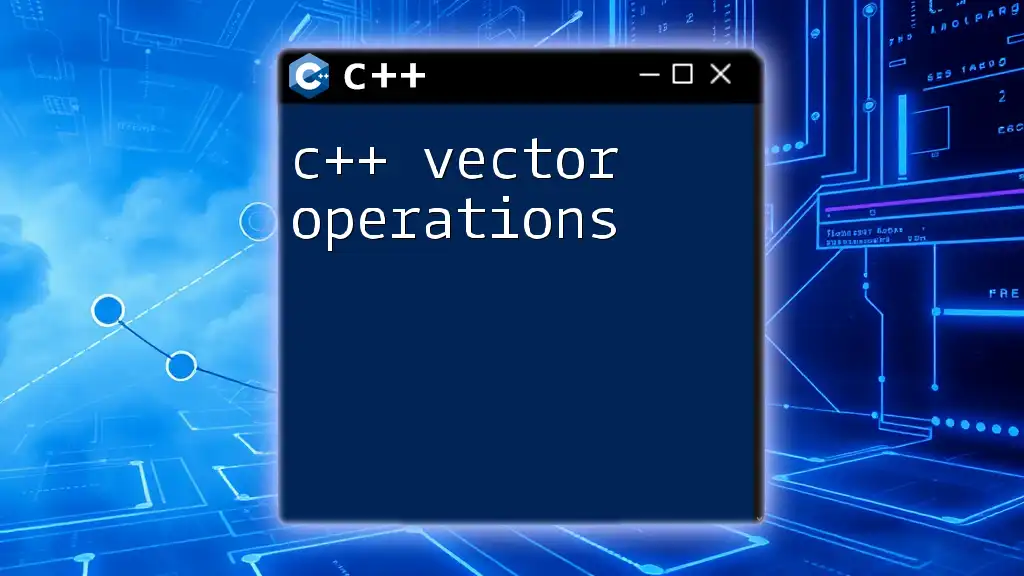
Converting a C++ Vector to an Array
Understanding how to convert a C++ vector to an array is a common requirement in programming. There are various methods of doing this, each with its own pros and cons.
Why Convert a Vector to an Array?
There are multiple reasons why you might want to convert a vector to an array. For example, you may want to interface with APIs or libraries that require raw arrays, or you may need a fixed-size array for performance-critical applications.
Methods for Conversion
Using `std::vector::data()` Method
The `data()` method of a vector can be used to get a pointer to the underlying array representation of the vector.
Code Example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
int* array = vec.data();
// Output array contents
for (size_t i = 0; i < vec.size(); ++i) {
std::cout << array[i] << " ";
}
return 0;
}
Pros & Cons: This method is simple and provides direct access to the vector's elements. However, the pointer returned is not a separate copy of the elements, which means modifications to the vector will reflect in the "array" as well.
Using `std::copy()` Function
Another effective method for converting a vector to an array is using the standard `std::copy()` function.
Code Example:
#include <iostream>
#include <vector>
#include <algorithm> // For std::copy
int main() {
std::vector<int> vec = {10, 20, 30};
int array[3];
std::copy(vec.begin(), vec.end(), array);
// Output array contents
for (size_t i = 0; i < 3; ++i) {
std::cout << array[i] << " ";
}
return 0;
}
Pros & Cons: This method offers flexibility in managing the size of the target array. However, it may introduce additional overhead due to the copying process, especially for larger datasets.
Using a Loop for Manual Copying
For complete control over the copying process, you can use a manual loop.
Code Example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {100, 200, 300, 400};
int array[4];
for (size_t i = 0; i < vec.size(); ++i) {
array[i] = vec[i];
}
// Output array contents
for (size_t i = 0; i < 4; ++i) {
std::cout << array[i] << " ";
}
return 0;
}
Pros & Cons: This method allows for customization, such as filtering elements during copying. However, it has higher overhead in terms of code complexity and is prone to errors if the loop bounds are not handled correctly.
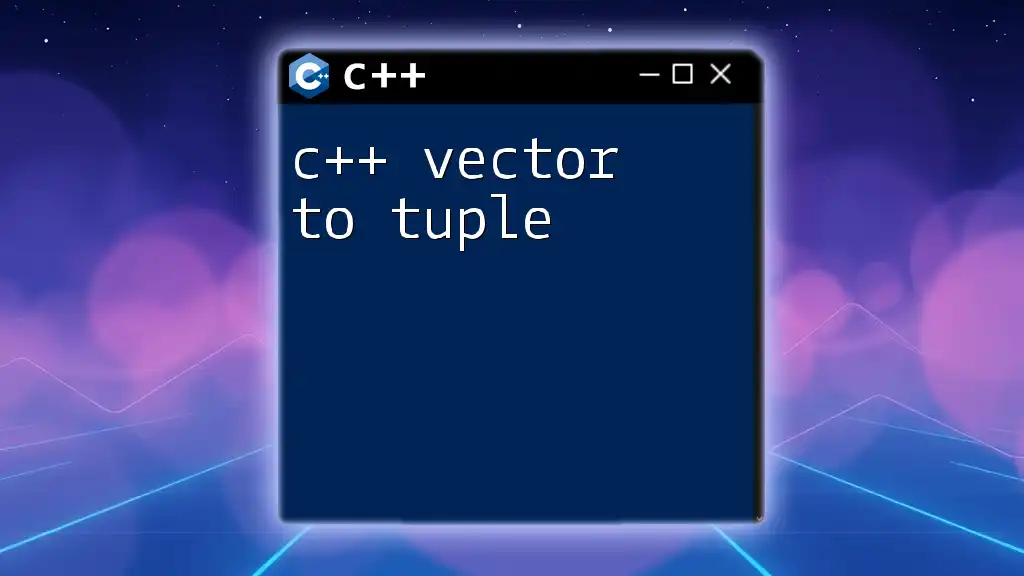
Best Practices for Conversion
When choosing the right method for converting a C++ vector to an array, consider the following:
- Selecting the Most Appropriate Method: If you need a simple reference, use `data()`. For a full copy, consider `std::copy()` for medium-sized data or manual copying for specific needs.
- Handling Edge Cases: Be mindful of situations such as empty vectors which can lead to out-of-bounds access if not adequately checked.
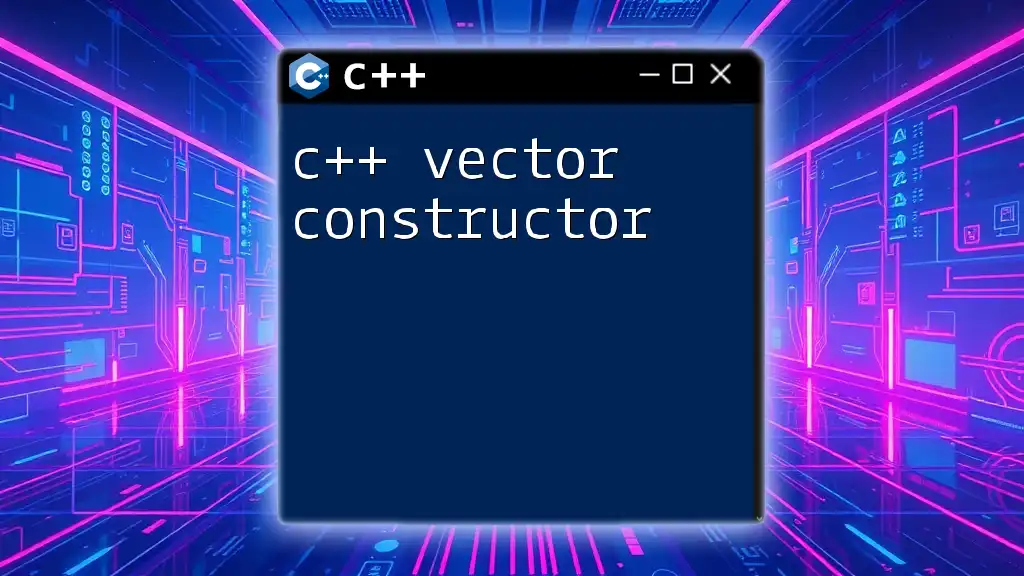
Performance Considerations
It’s crucial to understand when to prefer vectors over arrays and vice versa. Vectors generally provide better flexibility and safety due to their dynamic nature. However, for performance-critical operations, especially where memory allocation needs to be minimal, arrays can be more efficient.
When to Prefer Vectors Over Arrays
Vectors are a better choice:
- When you need to frequently add or remove elements.
- When working with modern C++ features and STL algorithms.
- When dealing with potentially undefined sizes.
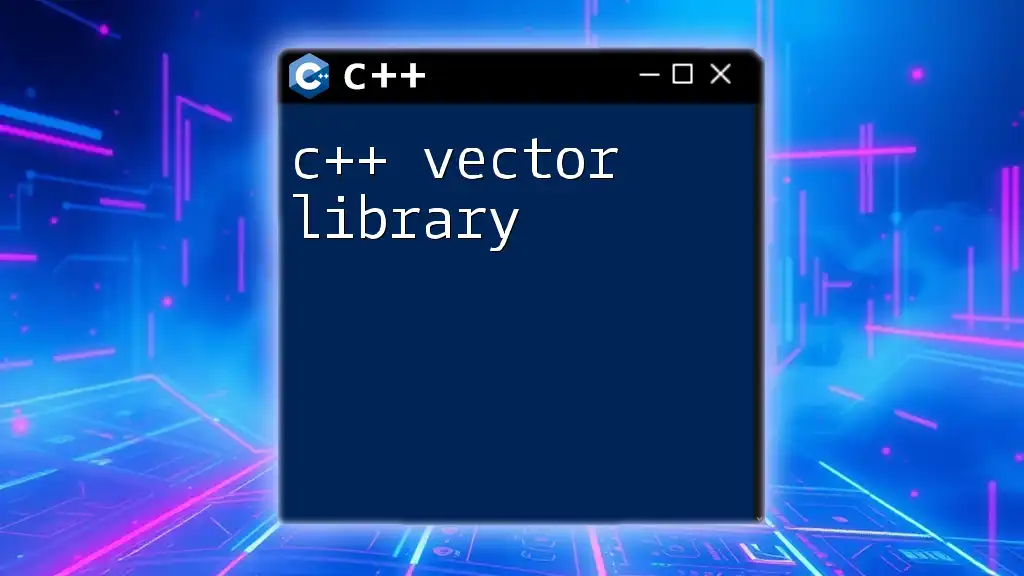
Conclusion
Understanding how to convert a C++ vector to an array is a valuable skill that enhances your data management capabilities. Selecting the right method based on context and requirement is crucial for effective programming.
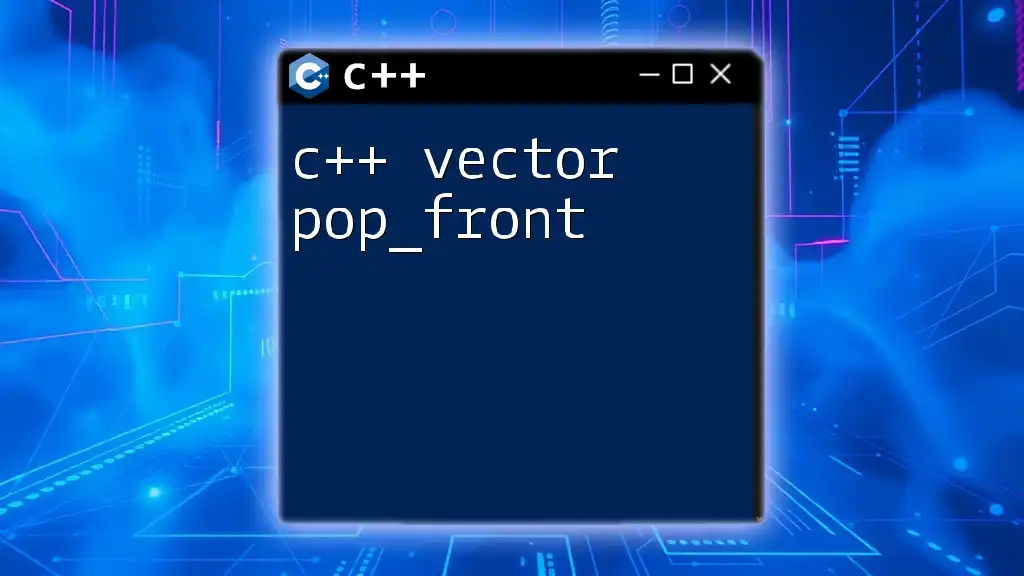
Call to Action
If you’re eager to dive deeper into the world of C++ programming and data manipulation, consider enrolling in our course! Join our community today for additional resources and connect with fellow learners.