In C++, you cannot directly append elements to a standard array since their size is fixed, but you can achieve this using a dynamically allocated array or by using a container like `std::vector`. Here’s a simple snippet using `std::vector`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myArray = {1, 2, 3}; // Initial array
myArray.push_back(4); // Append to the array
for(int num : myArray) {
std::cout << num << " "; // Output: 1 2 3 4
}
return 0;
}
Understanding Arrays in C++
What is an Array?
An array in C++ is a collection of items stored at contiguous memory locations. This data structure allows you to store multiple values of the same type under a single name, accessed using an index. For instance, if you want to store the grades of five students, you can use an array.
Arrays are fixed-size, meaning once you define their size at creation, it cannot be changed. This characteristic makes them efficient in terms of memory usage but also limits flexibility, especially when it comes to manipulating the data—like appending elements.
Why Modify an Array?
Manipulating arrays, such as appending new elements, is essential in various programming scenarios. For example:
- Real-time data collection, where the volume of incoming data may vary.
- Dynamic datasets, like user inputs in applications, where the total amount of data isn't predetermined.
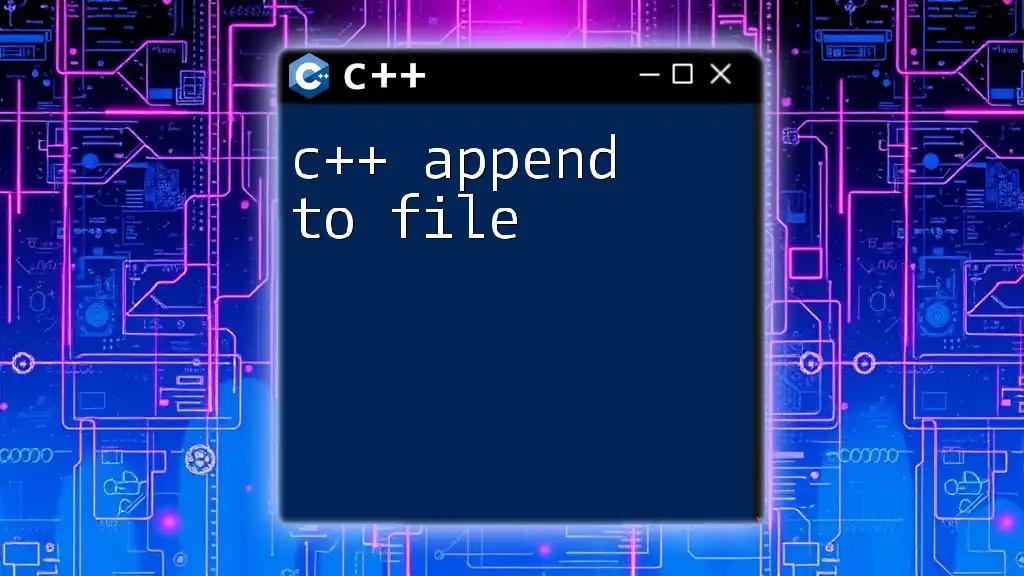
Appending to an Array in C++
The Limitations of Static Arrays
C++ allows the creation of static arrays, which have a predetermined size. For example, if you create an array of size 5, you won't be able to add more than five elements. Attempting to add more would result in accessing out-of-bounds memory, leading to undefined behavior. Thus, understanding how to append elements effectively is crucial.
Using Dynamic Arrays
What is a Dynamic Array?
Dynamic arrays address the limitations of static arrays by enabling dynamic memory allocation. This means you can allocate more memory as needed during the program's execution using the `new` and `delete` operators.
Creating a Dynamic Array
To declare a dynamic array, you allocate memory with `new`. Here’s how you could create a dynamic array of integers:
int* myArray = new int[5]; // Creating a dynamic array with size 5
This line allocates memory for five integers, which can be accessed like any normal array with indexes from `0` to `4`.
Appending Elements to a Dynamic Array
Appending elements to a dynamic array involves resizing the array and copying existing elements to the new memory allocation. Let’s break this down step-by-step.
Step-by-Step Guide to Appending
- Resize the Array: Create a new array larger than the original.
- Copy Existing Elements: Move all elements from the original array to the new one.
- Add New Elements: Place the new element at the end of the new array.
- Deallocate Old Array: Free memory associated with the old array.
- Update Pointer and Size: Point to the new array and increment the size.
Code Example: Appending an Element
Here’s how you might implement the appending functionality:
void append(int*& array, int& size, int newElement) {
int* newArray = new int[size + 1]; // Create a larger array
for (int i = 0; i < size; i++) {
newArray[i] = array[i]; // Copy existing elements
}
newArray[size] = newElement; // Add new element
delete[] array; // Free old array memory
array = newArray; // Point to new array
size++; // Increment size
}
With this function, you can dynamically add an element, ensuring that your array grows as needed.
Handling Memory Management
Importance of Memory Management
In C++, managing memory correctly is crucial. Neglecting to deallocate memory when it is no longer needed can lead to memory leaks, which degrade performance and can crash the program.
Best Practices
Always remember to use `delete[]` to free up the memory once you are done using the dynamic array to prevent leaks. For instance:
delete[] myArray; // Always deallocate memory when it's no longer needed
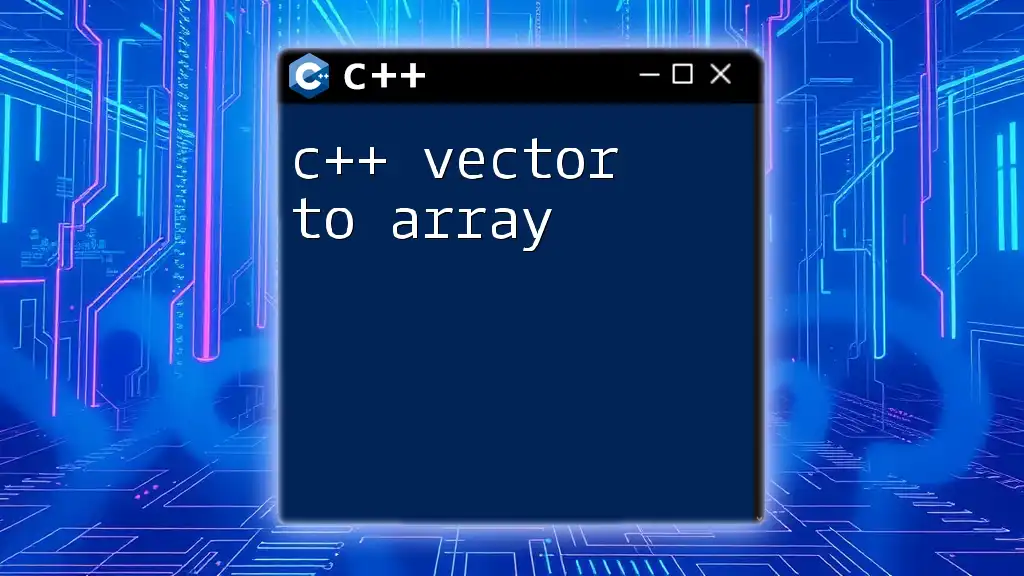
Alternatives to Appending to an Array
Using `std::vector`
What is `std::vector`?
`std::vector` is part of the C++ Standard Template Library (STL) and provides a dynamic array implementation. It abstracts away the complexity tied to resizing and appending elements.
Advantages of `std::vector`
One significant advantage of using a vector is its ability to automatically resize itself. This built-in feature allows developers to focus on the application logic rather than manual memory management.
Example: Appending Using `std::vector`
Appending an element using `std::vector` is straightforward and requires only one function call:
#include <vector>
void appendWithVector(std::vector<int>& vec, int newElement) {
vec.push_back(newElement); // Append element
}
This line effortlessly adds a new element to the end of the vector, and the vector handles resizing automatically.
Choosing Between Arrays and Vectors
While raw arrays provide raw performance and minimal overhead, `std::vector` offers enhanced flexibility and ease of use. When deciding which to use, consider the nature of your data and whether you need the flexibility of dynamic size.
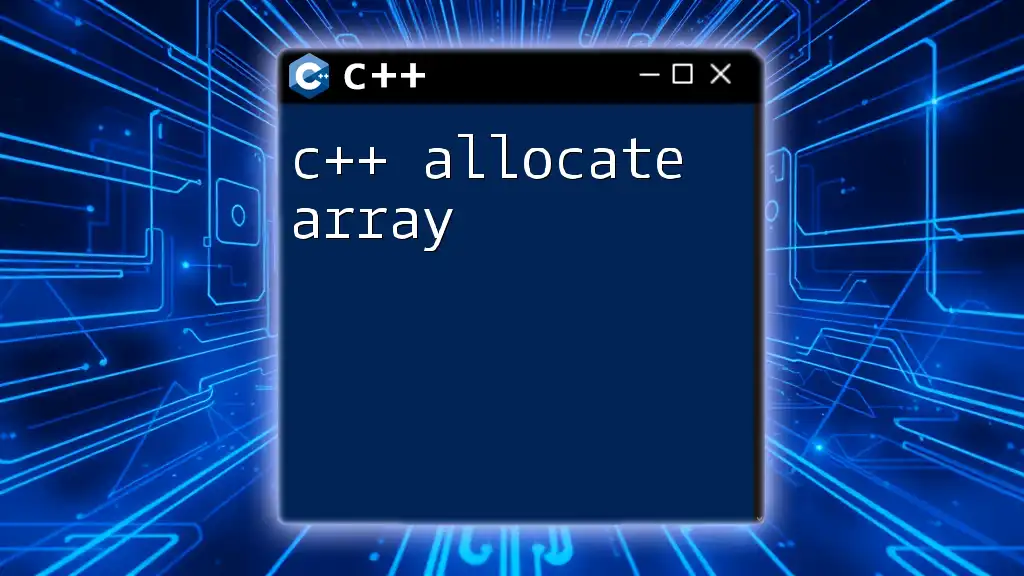
Performance Considerations
Time Complexity of Appending
The efficiency of appending in dynamic arrays and vectors can vary. Appending an element to a dynamic array has an average time complexity of O(n) due to the need for resizing. Conversely, appending to a `std::vector` is typically O(1) on average, thanks to its ability to manage memory automatically.
Memory Usage and Efficiency
Dynamic arrays can lead to wasted memory if over-allocated, while under-allocated arrays can necessitate multiple reallocations. Vectors optimize this by using an internal growth strategy, but the initial allocation does have an overhead. Understanding these aspects can help you make sound design choices.
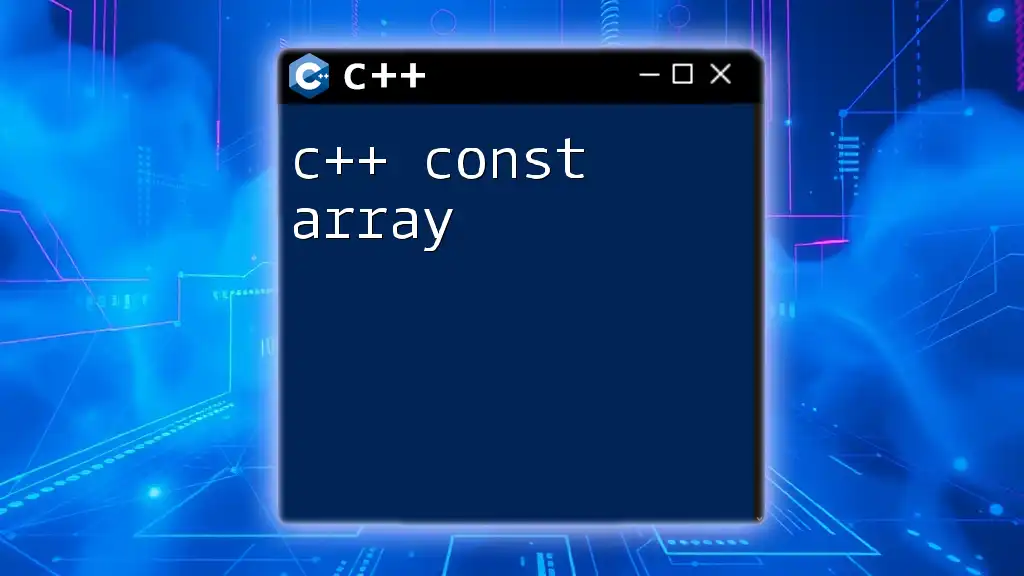
Conclusion
Recap of Key Points
In this guide, we explored how to append to arrays in C++, focusing on both dynamic arrays and the advantages of using `std::vector`. We discussed the memory management implications and best practices necessary for avoiding memory leaks.
Encouragement to Practice
I encourage you to experiment with dynamic arrays and `std::vectors` within your projects. Implementing these concepts will enhance your understanding and proficiency in C++.
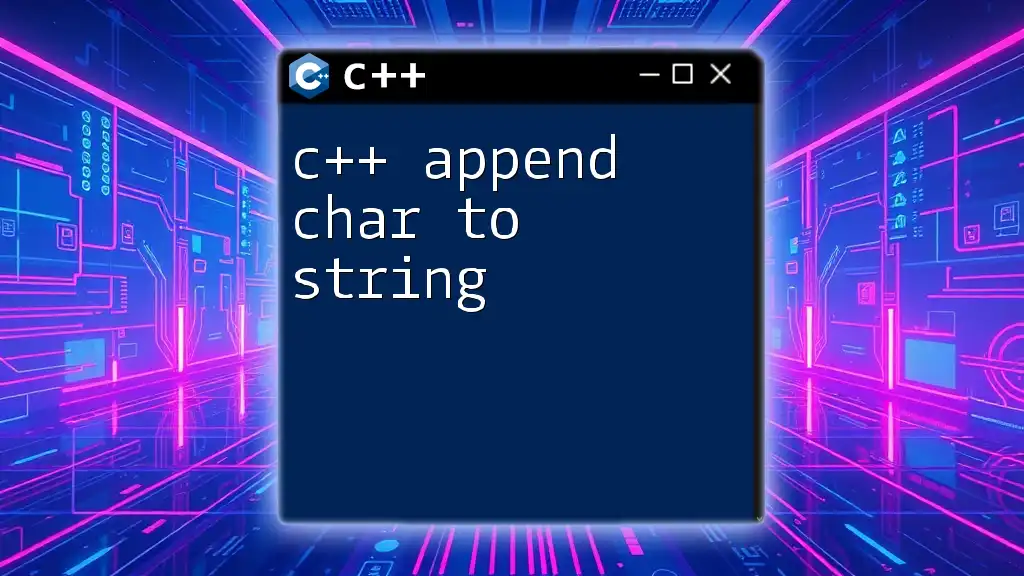
Additional Resources
Recommended Reading and Tutorials
To deepen your knowledge, consider exploring more resources related to arrays, dynamic memory allocation, and the STL. Many online tutorials and documented practices can provide further insights.
Community Support
Don't hesitate to engage with programming communities like Stack Overflow or Reddit for support, collaboration, and sharing your experiences with C++. Building a network can significantly aid in your learning journey.