To append data to a file in C++, you can use the `std::ofstream` class with the `std::ios::app` flag to ensure that new content is added to the end of the file without overwriting existing data.
Here's a code snippet demonstrating this:
#include <iostream>
#include <fstream>
int main() {
std::ofstream file("example.txt", std::ios::app);
if (file.is_open()) {
file << "Appending this text to the file.\n";
file.close();
} else {
std::cerr << "Unable to open file";
}
return 0;
}
Setting Up Your C++ Environment
To begin working with file handling in C++, you need to include the necessary library. The `<fstream>` library provides all the features required for file manipulation, including reading from and writing to files.
Required Libraries
To handle file operations, make sure to include the following line at the top of your C++ program:
#include <fstream>
This library enables you to work with different types of file streams, including input (`ifstream`) and output (`ofstream`), as well as file handling features.
Compilation and Execution Basics
Before diving into coding, ensure you have a proper setup for compiling and executing C++ programs. You can use any standard C++ compiler like g++, and your command in the terminal might look like this:
g++ your_program.cpp -o your_program
./your_program
DO ensure that your development environment is set up correctly to avoid compilation errors related to file handling.
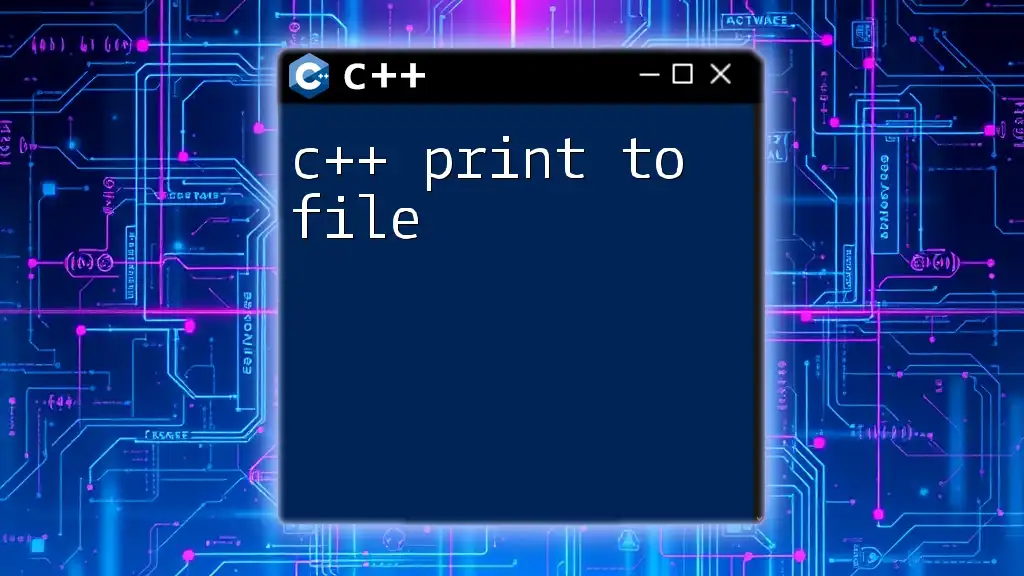
Understanding the Append Mode
When you want to add data to an existing file without altering its current content, you'll use the append mode. This mode enables you to append new data directly at the end of a file.
What is Append Mode?
Append mode is a specific file mode that allows data to be added to the end of a file. When a file is opened in append mode, the file pointer is automatically positioned at the end of the file, ensuring that any data written will be added without overwriting existing content.
Open the File in Append Mode
To open a file in append mode, you can pass the `std::ios::app` flag to the `ofstream` constructor. Here is an example:
std::ofstream outFile("example.txt", std::ios::app);
This line of code ensures that `outFile` is set up to append data to `example.txt`. If the file does not already exist, it will be created.
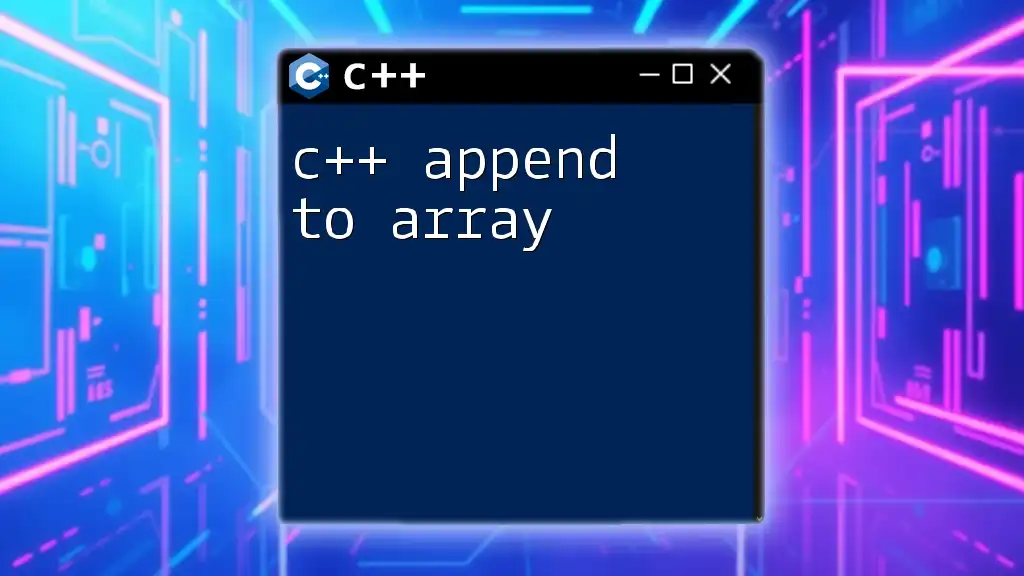
Appending to a File in C++
Writing First Example
Now that you understand how to open a file in append mode, let’s write a simple example where we append a line of text to a file:
#include <iostream>
#include <fstream>
int main() {
std::ofstream outFile("example.txt", std::ios::app);
if (outFile.is_open()) {
outFile << "Hello, World!\n";
outFile.close();
} else {
std::cerr << "Unable to open file";
}
return 0;
}
In this code snippet:
- We start by including the necessary libraries.
- We create an instance of `ofstream`, opening `example.txt` in append mode.
- We check whether the file is open. If so, we write "Hello, World!" to the file followed by a newline.
- Finally, we close the file to ensure all data is saved and that any resources are released.
Explanation of the Code
Each line plays a crucial role:
- `std::ofstream outFile("example.txt", std::ios::app);` opens the file for appending.
- The `if` statement checks if the file was successfully opened.
- `outFile << "Hello, World!\n";` appends the text to the file.
- `outFile.close();` is essential to free resources and ensure all data has been written.
Appending Multiple Lines
You can also append multiple lines of text to a file efficiently. Here’s an example that demonstrates how to do so using a loop:
#include <iostream>
#include <fstream>
#include <vector>
int main() {
std::ofstream outFile("example.txt", std::ios::app);
if (outFile.is_open()) {
std::vector<std::string> lines = {"Line 1", "Line 2", "Line 3"};
for (const auto& line : lines) {
outFile << line << "\n";
}
outFile.close();
}
return 0;
}
In this example:
- We create a `std::vector` containing the lines we want to append.
- A `for` loop iterates through each line and appends it to the file, making it an efficient approach to handle multiple entries.
Explanation of Batch Appending
Here, the use of a vector allows the easy management of multiple strings, while the `for` loop systematically writes each line to the file. This approach minimizes redundancy in file operations and enhances code maintainability.
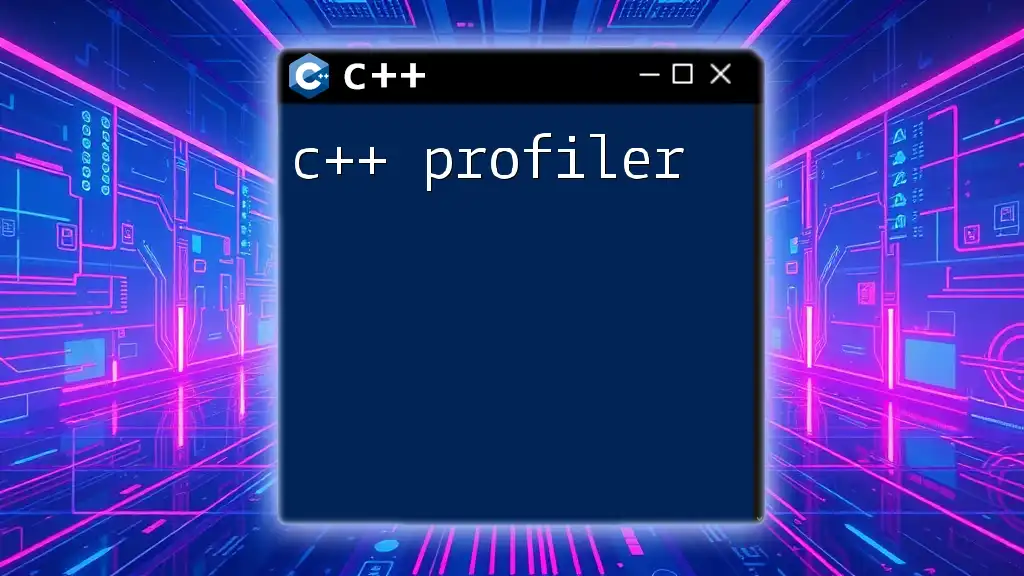
Best Practices for Appending to Files
To ensure that your file handling is robust and efficient, follow these best practices:
Handling File Open Errors
Always check if a file opens correctly. Performing a conditional check after opening the file prevents runtime errors. This best practice ensures that your program behaves predictably even when file access fails.
Closing Files Properly
Make it a habit to always close your files after operations. Failing to do so can lead to issues such as file locks, data loss, or corrupted files. Always use:
outFile.close();
Using Exception Handling
For more robust code, consider using exception handling. By placing your file operations within a `try-catch` block, you can gracefully handle unexpected errors and provide feedback to the user.
try {
std::ofstream outFile("example.txt", std::ios::app);
if (!outFile.is_open()) {
throw std::ios_base::failure("Failed to open file.");
}
// Append operations here...
outFile.close();
} catch (const std::ios_base::failure& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
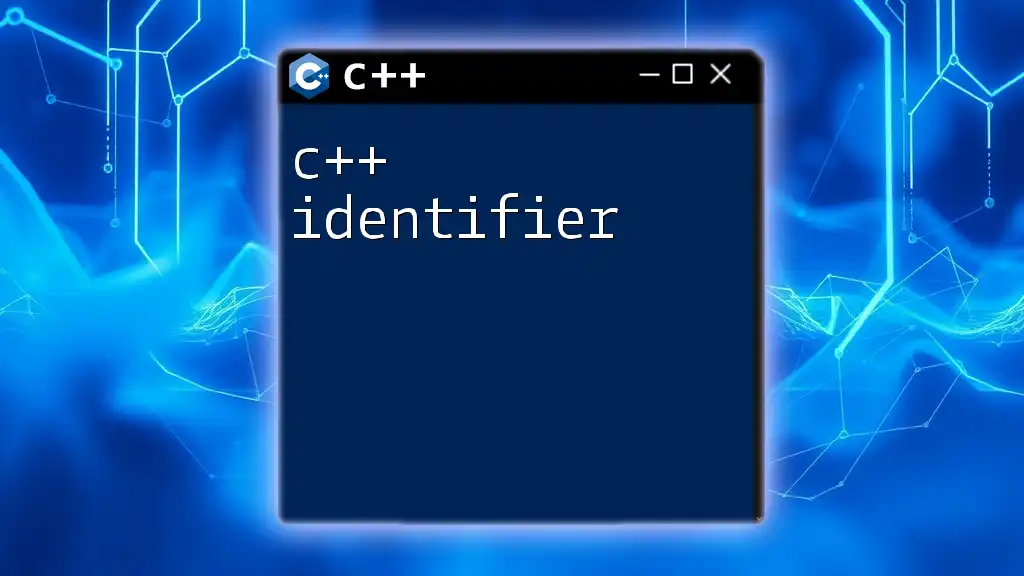
Common Pitfalls and Troubleshooting
Appending vs. Overwriting
One of the common mistakes when handling files is inadvertently overwriting existing content. The append mode should always be specified when you want to add data without losing previous entries.
File Permissions
Be aware of file permission issues that may occur when trying to append data. If your program does not have the necessary rights to access or modify the file, it won’t be able to append data successfully. Always verify the file’s permissions for the user account running the process.
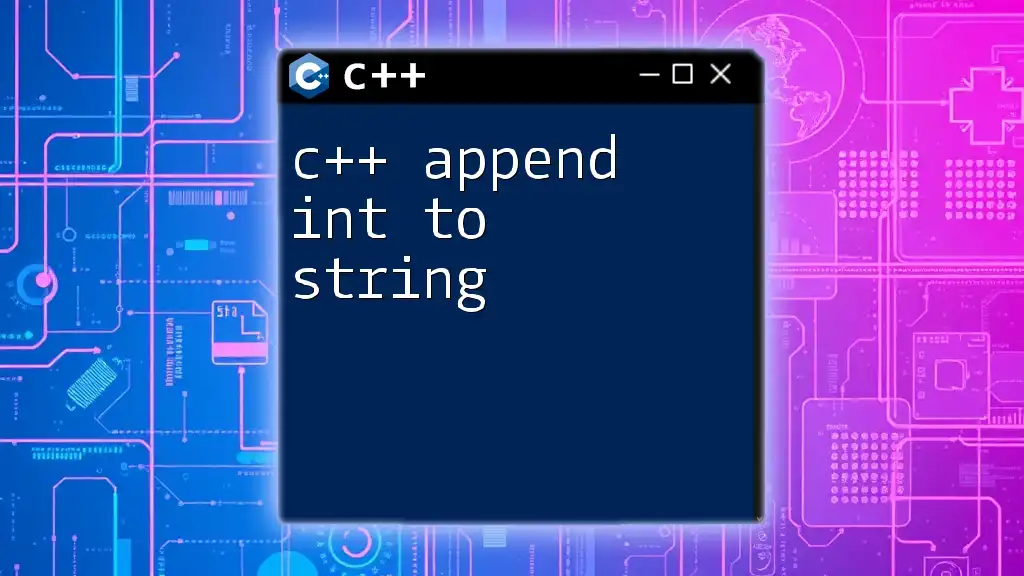
Conclusion
In summary, appending to files in C++ is a powerful feature that allows you to extend existing data without manipulating the original content. By utilizing the correct file mode and following best practices, you can ensure your applications handle file operations efficiently and reliably.
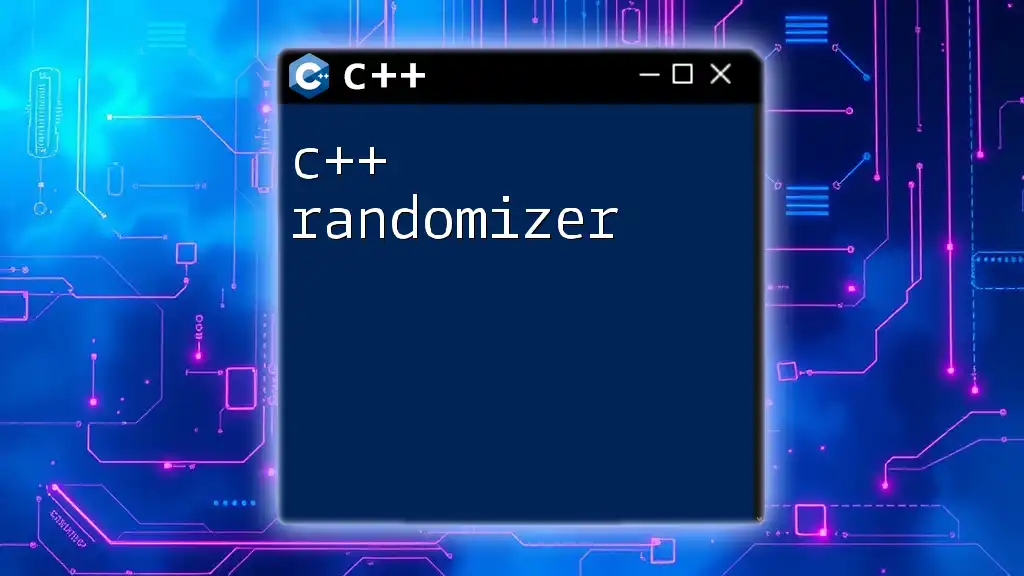
Frequently Asked Questions (FAQ)
Can I append to a file in binary mode?
Yes, C++ allows you to open a file in binary mode for appending. You would use the `std::ios::app | std::ios::binary` flag when opening the file.
What if the file doesn't exist when I attempt to append?
When you open a file in append mode and the file does not exist, C++ will create a new file automatically.
Is there a limit to how much I can append to a file?
In practice, the limits for appending to a file are determined by the available disk space and system constraints rather than specific restrictions in C++. However, exceedingly large files may impact performance.