In C++, you can copy a file using the standard library's `<fstream>` by reading from the source file and writing to the destination file, as shown below:
#include <fstream>
#include <iostream>
int main() {
std::ifstream src("source.txt", std::ios::binary);
std::ofstream dest("destination.txt", std::ios::binary);
dest << src.rdbuf();
return 0;
}
Understanding File Streams in C++
C++ file handling is primarily done through the concept of file streams. A file stream allows you to read from or write to files by setting up a data flow between the program and the file on disk. In C++, there are three major categories of file streams:
- Input File Stream (`ifstream`): Used for reading data from files.
- Output File Stream (`ofstream`): Used for writing data to files.
- Bidirectional Stream (`fstream`): Used for both reading and writing.
Understanding these streams is crucial for performing file operations like the `c++ copy file` operation effectively.

Setting Up Your Development Environment
Before diving into file copying in C++, you need a suitable development environment. Recommended IDEs include:
- Visual Studio
- Code::Blocks
- CLion
- Eclipse with CDT
Make sure you have a C++ compiler installed, such as GCC or MSVC. A basic project structure can include:
/your_project
├── src/
│ └── main.cpp
└── data/
└── source.txt
This structure helps keep files organized and accessible when coding your file operations.
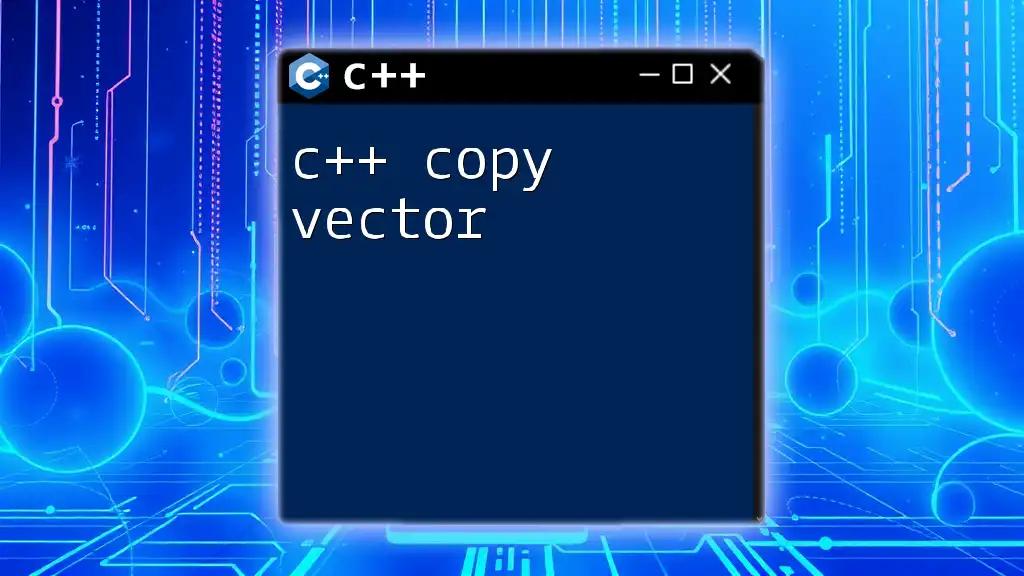
Basic File Copying Methods in C++
Method 1: Using `ifstream` and `ofstream`
One of the most straightforward methods to copy a file in C++ is by utilizing `ifstream` for reading and `ofstream` for writing. This method is efficient for small to medium-sized files. Here is a simple example:
#include <iostream>
#include <fstream>
void copyFile(const std::string &source, const std::string &destination) {
std::ifstream sourceFile(source, std::ios::binary);
std::ofstream destFile(destination, std::ios::binary);
destFile << sourceFile.rdbuf();
sourceFile.close();
destFile.close();
}
In this code:
- The `ios::binary` flag is used to ensure that the files are treated as binary, avoiding any unintended translation of line endings (which can occur when dealing with text files).
- The `rdbuf()` function reads the entire content of the source file stream and writes it to the destination file stream in one concise line.
Method 2: Using Standard Library Functions
C++17 introduced the `std::filesystem` library, which greatly simplifies the process of copying files. By using this approach, you can copy files with minimal code:
#include <iostream>
#include <filesystem>
void copyFileUsingFilesystem(const std::string &source, const std::string &destination) {
std::filesystem::copy(source, destination);
}
This method is particularly advantageous because it encapsulates many error-checking mechanisms and optimizations internally, making it a robust choice for copying files.
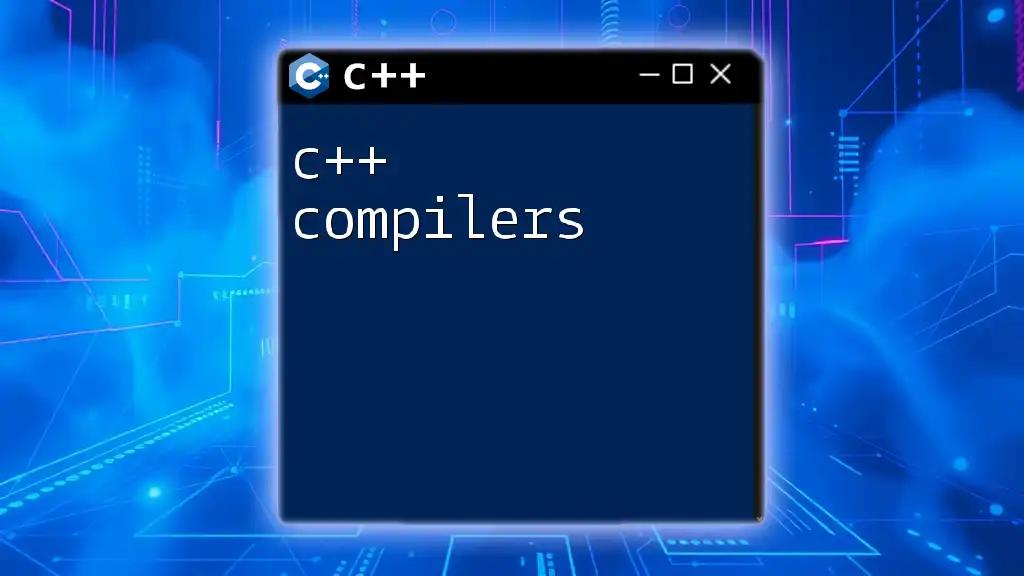
Error Handling While Copying Files
Error handling is a critical part of file operations. When copying files in C++, you should ensure the source file exists and handle potential permission issues.
Here’s how you can add error handling to your file copy function:
#include <iostream>
void copyFileWithErrorHandling(const std::string &source, const std::string &destination) {
std::ifstream sourceFile(source, std::ios::binary);
if (!sourceFile) {
std::cerr << "Source file cannot be opened!" << std::endl;
return;
}
std::ofstream destFile(destination, std::ios::binary);
if (!destFile) {
std::cerr << "Destination file cannot be created!" << std::endl;
return;
}
destFile << sourceFile.rdbuf();
sourceFile.close();
destFile.close();
}
In this enhanced version:
- It checks if the source file is opened successfully.
- It checks if the destination file has been created properly.

Advanced File Copying Techniques
Copying Large Files
When dealing with large files, it’s essential to consider performance. Copying a file in chunks can reduce memory overhead significantly. Here’s how you can implement this technique:
void copyLargeFile(const std::string &source, const std::string &destination) {
std::ifstream sourceFile(source, std::ios::binary);
std::ofstream destFile(destination, std::ios::binary);
const size_t bufferSize = 1024 * 1024; // 1 MB
char buffer[bufferSize];
while (sourceFile.read(buffer, bufferSize) || sourceFile.gcount()) {
destFile.write(buffer, sourceFile.gcount());
}
sourceFile.close();
destFile.close();
}
In this code:
- A buffer of 1 MB is defined to hold chunks of data as they are read from the source file.
- The while loop continues reading until there is no more data left.
Copying Multiple Files
In scenarios where you may want to copy multiple files at once, iterating through a list of file names and copying them can be very useful. Here's an example of how to do this with `std::filesystem`:
#include <vector>
void copyMultipleFiles(const std::vector<std::string> &files, const std::string &destinationFolder) {
for (const auto &file : files) {
std::filesystem::copy(file, destinationFolder + "/" + std::filesystem::path(file).filename().string());
}
}
This function takes in a vector of file names and a destination folder. It copies each file to the specified folder, maintaining the original filename.

Conclusion
In this guide, we have explored various methods for performing a `c++ copy file` operation. From basic approaches using `ifstream` and `ofstream`, to advanced methods leveraging `std::filesystem`, we've covered both straightforward and complex scenarios. Remember, implementing robust error handling and considering performance for large files can significantly enhance your applications.
Further Learning
As you continue to improve your skills in file handling, consider diving deeper into C++ documentation, books, and online resources that focus on advanced topics and best practices for file I/O. The world of C++ file operations is rich and wide, with much more to discover!