C++ copy elision is an optimization technique that allows the compiler to skip the creation of temporary objects and directly construct the final object, enhancing performance.
Here’s a simple example demonstrating copy elision:
#include <iostream>
class Example {
public:
Example() { std::cout << "Constructor called\n"; }
Example(const Example&) { std::cout << "Copy constructor called\n"; }
};
Example createExample() {
return Example(); // Copy elision occurs here
}
int main() {
Example obj = createExample(); // No copy constructor call
return 0;
}
What is Copy Elision?
Definition
C++ copy elision refers to a compiler optimization technique that eliminates unnecessary copying of objects. This optimization allows the compiler to construct objects directly in their final location, thereby avoiding the overhead associated with creating temporary copies. Copy elision is a crucial aspect of modern C++ programming, facilitating optimal performance and efficient resource management.
It is essential to distinguish between copy elision and standard copy/move semantics. While copy elision prevents copies altogether, copy semantics creates duplicates of objects explicitly. Understanding this difference is vital for C++ developers aiming to write efficient code.
Historical Context
Historically, copy elision was not universally applied across all versions of C++. The introduction of Return Value Optimization (RVO) in C++98 was an early attempt at enabling this optimization. However, it wasn’t until C++11 that copy elision became more prevalent due to the standardization of move semantics and the introduction of the "Rule of Zero," which encourages developers to minimize the need for copy constructors and assignment operators.
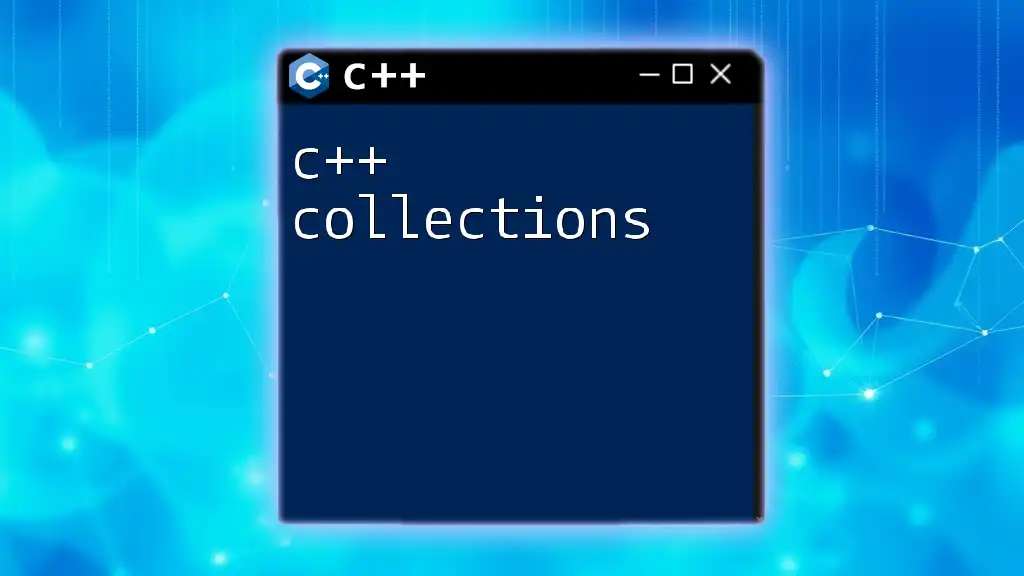
Why is Copy Elision Important?
Performance Optimization
One of the primary benefits of C++ copy elision is its ability to significantly enhance application performance. By eliminating redundant copy operations, the compiler reduces the time spent creating temporary objects, which can be particularly beneficial in performance-critical applications such as games or real-time systems.
Reducing the Overhead of Object Copying
In many programming scenarios, copying objects can introduce substantial overhead, particularly when working with large or complex data structures. This overhead often involves memory allocation, constructor calls, and destructor calls, all of which can degrade runtime efficiency. Copy elision effectively circumvents these issues, especially when returning objects from functions, allowing developers to write cleaner and faster code.
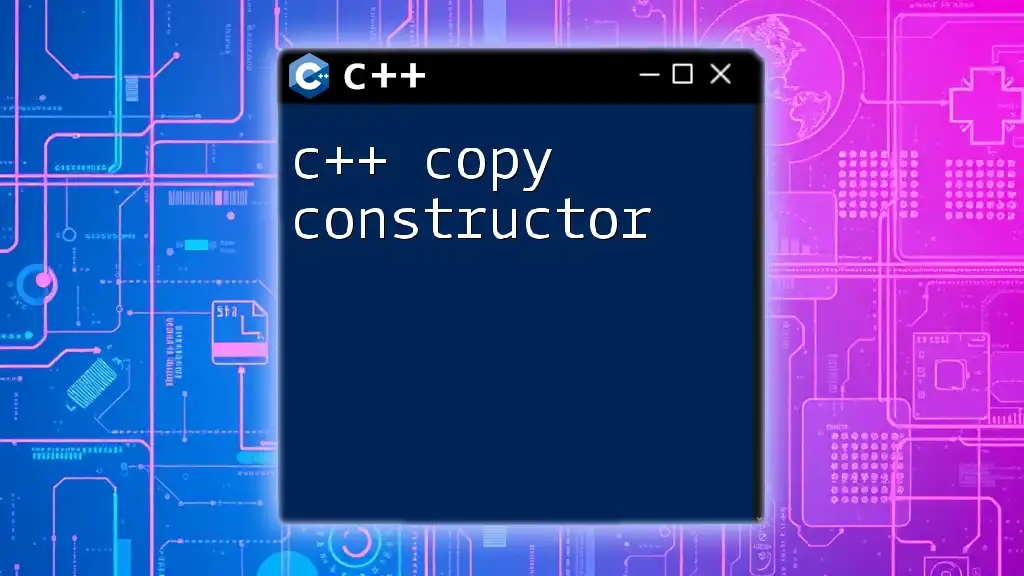
Mechanisms of Copy Elision
Mandatory Copy Elision
Certain contexts in C++ mandate copy elision. For example, when a temporary object is returned from a function, the compiler has an obligation to optimize away the copy.
Consider the following code:
class MyClass {
public:
MyClass() { /* constructor */ }
MyClass(const MyClass&) { /* copy constructor */ }
};
MyClass createMyClass() {
return MyClass(); // Mandatory copy elision applied here
}
In this example, the temporary `MyClass` object created within `createMyClass()` is constructed directly in the location where it will be used, thus optimized out of any copying.
Optional Copy Elision
However, copy elision is not always guaranteed. Certain conditions allow the compiler to opt for copy elision, provided specific criteria are met. This is known as optional copy elision.
In contrast to mandatory copy elision, the compiler may choose to skip this optimization under certain scenarios. One typical situation is when returning an object from a function where it cannot predict the outcomes or where optimizations may increase complexity without performance benefits.
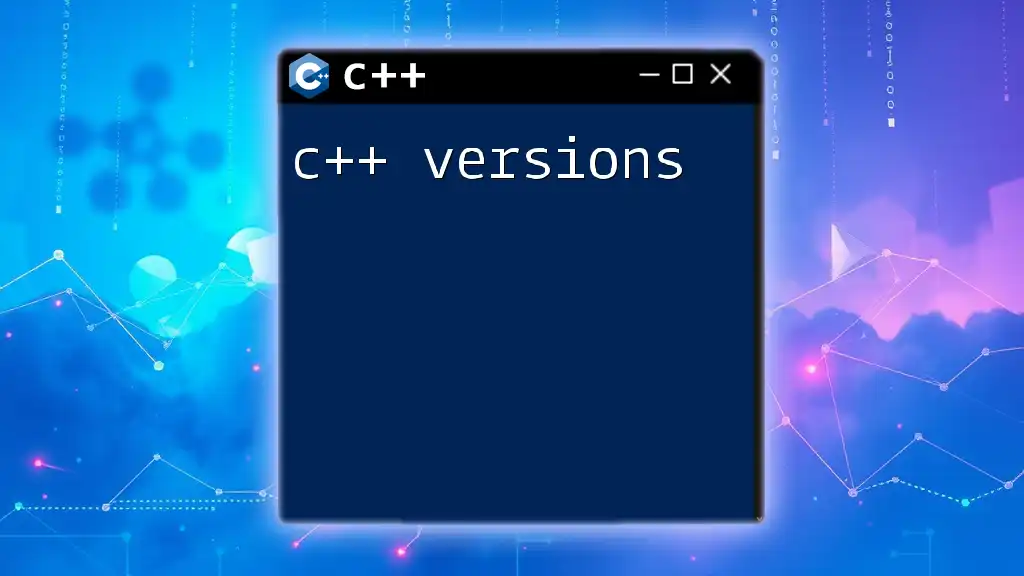
How to Enable Copy Elision
Contexts Where Copy Elision Occurs
Copy elision can commonly occur in contexts such as:
- Stack allocation: When objects are created on the stack, they may be optimized away quicker than heap-allocated objects due to their predictable lifetimes.
- Function return values: When a function returns an object by value, the compiler can often directly construct the object in the caller's stack frame.
Return Value Optimization (RVO)
RVO refers to the compiler’s ability to construct the return value directly into the space allocated for the caller's variable, thus eliminating the intermediate copies.
Here’s a simple example:
MyClass createMyClass() {
return MyClass(); // RVO will likely apply here
}
Due to RVO, `MyClass` is directly constructed in the `createMyClass()` call site, negating the overhead of temporary storage.
Named Return Value Optimization (NRVO)
NRVO is the specific type of RVO that applies when the return object is a named variable. This optimization is particularly efficient because the allocation and lifetime of named variables provide the compiler with more context for optimization decisions.
For example:
MyClass createMyClass(bool condition) {
MyClass obj; // Referenced NAMED object
if (condition) {
return obj; // NRVO applied, no copy constructed
}
return MyClass(); // RVO for a temporary
}
Even when returning to a non-conditional path, NRVO can often apply, resulting in performance gains.
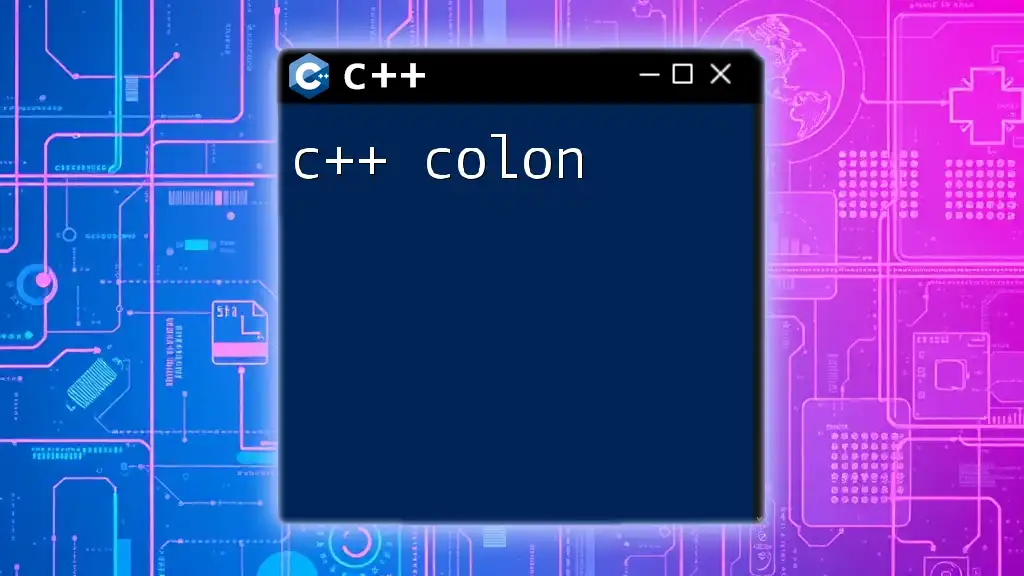
Practical Examples
Example 1: Simple Class with RVO
The following snippet demonstrates RVO clearly:
class MyClass {
public:
MyClass() { /* constructor */ }
MyClass(const MyClass&) { /* copy constructor */ }
};
MyClass createMyClass() {
return MyClass(); // RVO applied here
}
// No copy will occur as MyClass is constructed directly in the calling context.
Example 2: Understanding NRVO
This example highlights named return optimization:
MyClass createMyClass(bool condition) {
MyClass obj; // Named object created on the stack
if (condition) {
return obj; // NRVO can eliminate copy
}
return MyClass(); // RVO for temporary object
}
Here, if `condition` is true, `obj` gets returned without needing a copy due to NRVO, showcasing the optimization's effectiveness.
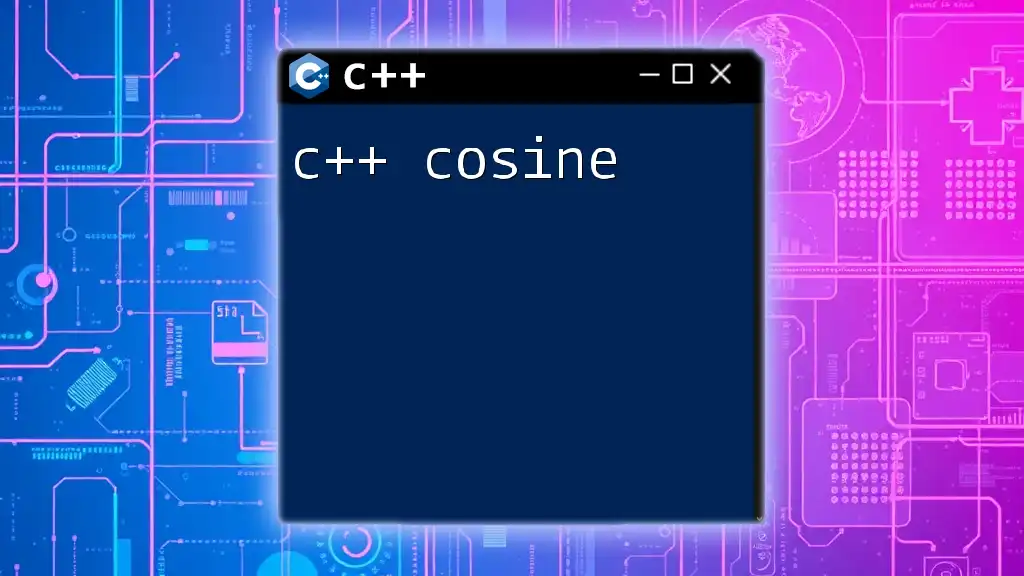
Pitfalls and Limitations of Copy Elision
Moving from C++11 to C++17
As C++ has evolved, the rules surrounding copy elision have changed. In C++17, there are conditions that require copy elision to take place, particularly for certain return types. Developers must be cautious of these changes and understand their implications.
Inference Limitations
Copy elision cannot occur in all circumstances. For complex types, certain copy constructors may prevent copy elision due to their non-trivial nature. Additionally, when returning objects from overloaded functions or non-trivial template cases, the potential for optimization significantly decreases.
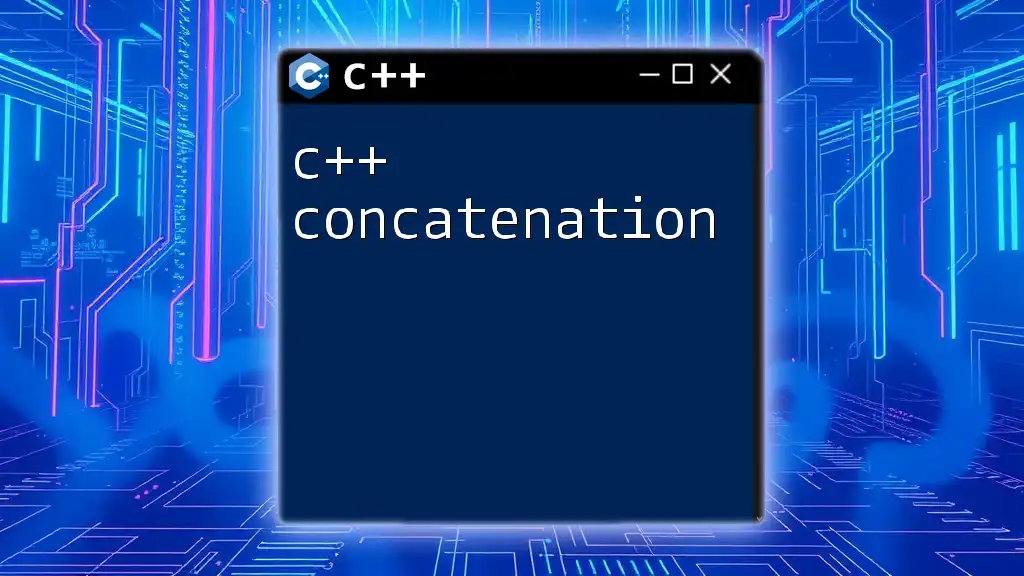
Best Practices for Leveraging Copy Elision
Structuring Your Code for Optimal Performance
To maximize the effectiveness of copy elision:
- Utilize stack-based allocations for temporaries instead of heap allocations when possible.
- When designing classes, adhere to the Rule of Zero, aiming to minimize the definition of constructors, destructors, and copy/move constructors.
Profiling and Measuring Performance Gains
To assess performance gains from using copy elision:
- Use profiling tools like Valgrind or Visual Studio diagnostics to quantify the performance impact.
- Perform benchmark tests to compare the performance of code with and without copy elision to demonstrate tangible benefits.
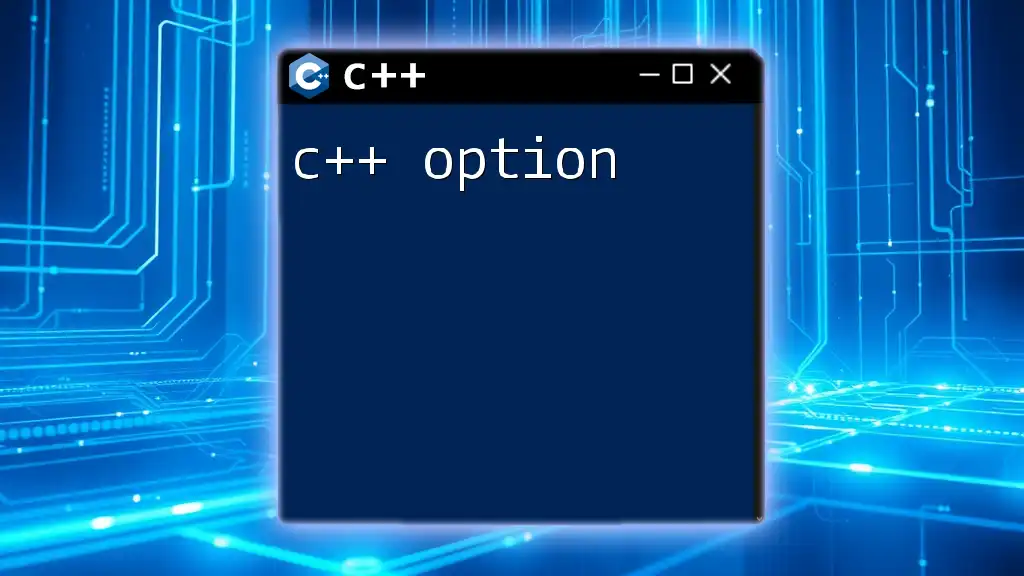
Conclusion
In conclusion, mastering C++ copy elision is essential for any C++ developer looking to optimize their code for performance. By understanding how copy elision works, its implications in modern C++, and how to leverage it effectively, developers can write code that is not only functional but also efficient. Applying the best practices discussed will lead to better resource management and improved runtime performance in C++ applications.
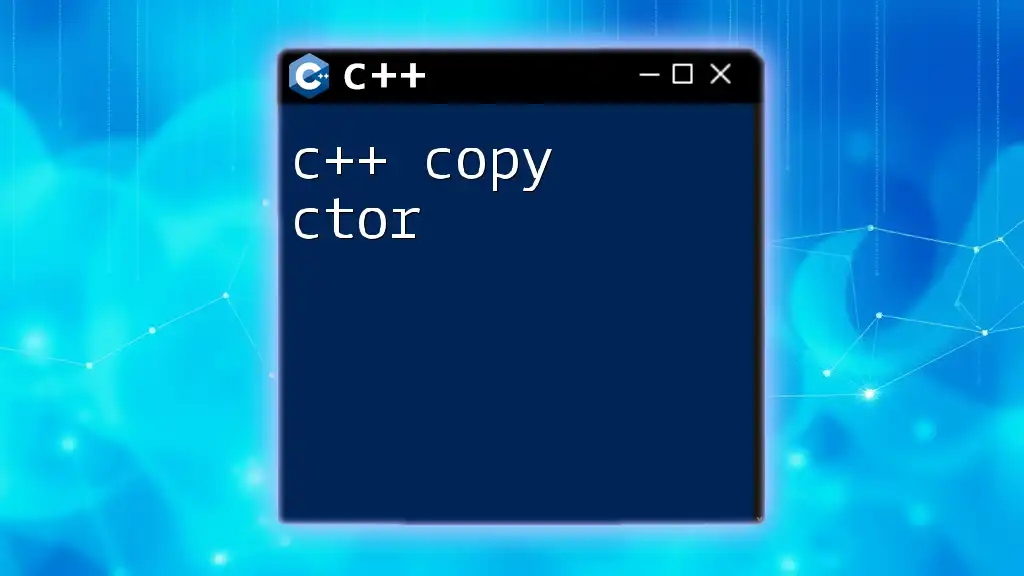
Additional Resources
For readers looking to delve deeper into copy elision and related concepts, explore advanced C++ programming articles, books, and tutorials that address copy semantics, object lifetimes, and optimization techniques in greater depth.
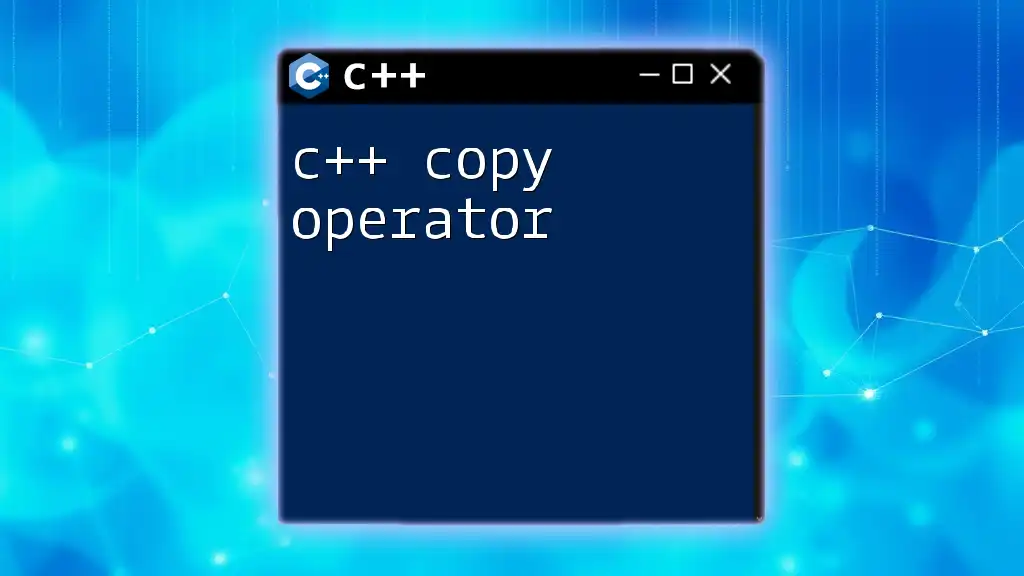
Call to Action
We encourage you to share your experiences using copy elision in your C++ projects. Follow our blog for more insightful tutorials and expert guidance on enhancing your C++ skills!