C++ composition is a design principle where a class is composed of one or more objects from other classes, promoting a "has-a" relationship, while inheritance is a way to create a new class based on an existing class, highlighting an "is-a" relationship.
Here's a code snippet demonstrating both concepts:
#include <iostream>
// Base class
class Engine {
public:
void start() {
std::cout << "Engine starting..." << std::endl;
}
};
// Composition: Car "has-a" Engine
class Car {
private:
Engine engine; // Composition
public:
void startCar() {
engine.start();
std::cout << "Car is running." << std::endl;
}
};
// Inheritance: ElectricCar "is-a" Car
class ElectricCar : public Car {
public:
void startCar() {
std::cout << "Electric Car ready." << std::endl;
Car::startCar();
}
};
int main() {
Car myCar;
myCar.startCar(); // Demonstrating composition
ElectricCar myElectricCar;
myElectricCar.startCar(); // Demonstrating inheritance
return 0;
}
Understanding Inheritance in C++
Definition of Inheritance
Inheritance is a fundamental concept in Object-Oriented Programming (OOP) that allows one class to inherit properties and behaviors (methods) from another. This establishes an is-a relationship, where a derived class (child) builds upon the base class (parent). For instance, if we have a class `Animal`, a class `Dog` can inherit from `Animal`, indicating that a dog is an animal.
Types of Inheritance
Understanding the different types of inheritance is crucial in leveraging the power of OOP.
Single Inheritance
In single inheritance, a class derives from a single base class. This is straightforward and promotes a simple class hierarchy.
Example:
class Animal {
public:
void speak() { cout << "Animal speaks"; }
};
class Dog : public Animal {
public:
void bark() { cout << "Dog barks"; }
};
In this example, `Dog` inherits the method `speak()` from `Animal` while also defining its own method `bark()`.
Multiple Inheritance
Multiple inheritance allows a derived class to inherit from more than one base class, creating a complex hierarchy. While powerful, it can introduce ambiguity in certain situations.
Example:
class Flyer {
public:
void fly() { cout << "Flying"; }
};
class Swimmer {
public:
void swim() { cout << "Swimming"; }
};
class Duck : public Flyer, public Swimmer {
public:
void quack() { cout << "Quacking"; }
};
In this example, `Duck` inherits capabilities from both `Flyer` and `Swimmer`.
Multilevel Inheritance
In multilevel inheritance, a class is derived from another derived class, creating a chain of inheritance that further extends functionality.
Example:
class Animal {
public:
void eat() { cout << "Eating"; }
};
class Dog : public Animal {
public:
void bark() { cout << "Barking"; }
};
class Puppy : public Dog {
public:
void weep() { cout << "Weeping"; }
};
Here, `Puppy` inherits from `Dog`, which in turn inherits from `Animal`, showcasing how properties and behaviors are passed down.
Hierarchical Inheritance
Hierarchical inheritance occurs when multiple derived classes inherit from a single base class.
Example:
class Vehicle {
public:
void start() { cout << "Vehicle starts"; }
};
class Car : public Vehicle {
public:
void drive() { cout << "Driving Car"; }
};
class Bike : public Vehicle {
public:
void ride() { cout << "Riding Bike"; }
};
In this scenario, both `Car` and `Bike` inherit the `start()` method from the `Vehicle` class, demonstrating shared functionality among classes.
Code Example: Inheritance in Action
class Animal {
public:
void speak() { cout << "Animal speaks"; }
};
class Dog : public Animal {
public:
void bark() { cout << "Dog barks"; }
};
int main() {
Dog dog;
dog.speak(); // Output: Animal speaks
dog.bark(); // Output: Dog barks
}
In this example, the `Dog` class effectively reuses the `speak()` method from its parent class `Animal` while also defining its own method, demonstrating the utility of inheritance in C++.
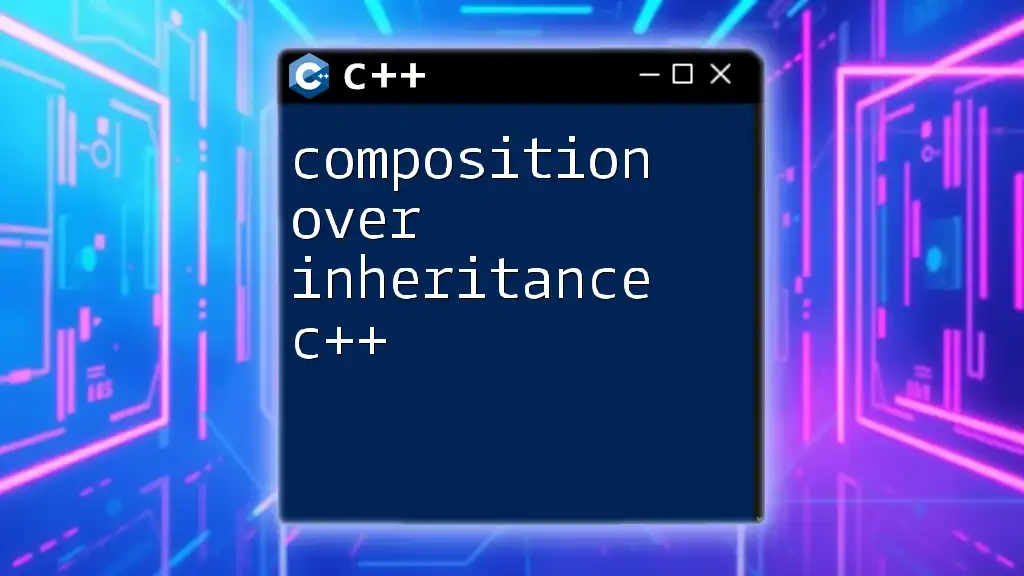
Understanding Composition in C++
Definition of Composition
Composition is another fundamental principle in OOP that represents a has-a relationship. Unlike inheritance, which incorporates behaviors from another class, composition involves creating more complex types by combining simple or existing objects. For example, a `Car` has an `Engine`—the car cannot operate without the engine, but the engine does not solely define the car.
Benefits of Composition
Composition provides several advantages over inheritance:
-
Flexibility and Reusability: By composing classes, you can easily interchange components. For instance, you could replace the `Engine` class with a `HybridEngine` class without affecting the `Car` class structure.
-
Easier Maintenance: Since composition builds complex systems from smaller, independent components, it simplifies updates without necessitating changes across an inheritance hierarchy.
-
Avoiding Inheritance Pitfalls: Composition avoids common inheritance issues like tight coupling and the diamond problem associated with multiple inheritance.
Code Example: Composition in Action
class Engine {
public:
void start() { cout << "Engine starts"; }
};
class Car {
private:
Engine engine; // Car 'has-a' Engine
public:
void start() { engine.start(); }
};
int main() {
Car car;
car.start(); // Output: Engine starts
}
In this example, the `Car` class has an instance of `Engine` and delegates the responsibility of starting to the engine. This clearly demonstrates the has-a relationship of composition.
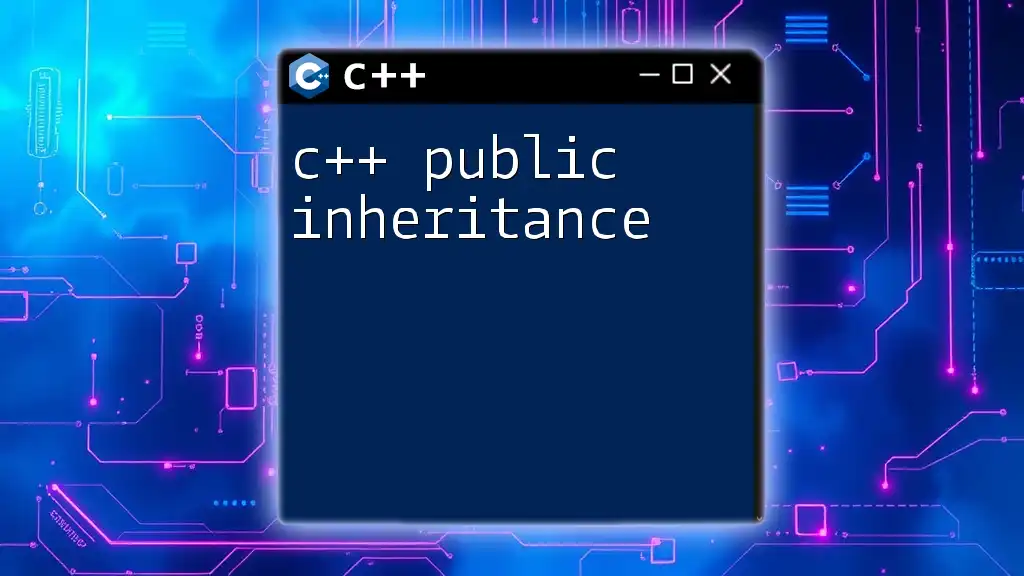
C++ Inheritance vs Composition
Similarities and Differences
Both inheritance and composition are critical paradigms in C++, yet they serve different purposes:
- Definition Comparison: Inheritance establishes an is-a relationship, while composition creates a has-a relationship.
- Use Cases: Inheritance is suitable for extending functionality or establishing category hierarchies, whereas composition is ideal for forming complex objects from simpler ones.
- Performance Considerations: Generally, composition can sometimes yield better performance due to reduced overhead from hierarchy and the ability to reuse existing components effectively.
When to Use Inheritance
Inheritance is an excellent choice under certain conditions, such as:
- When you want to extend functionality from a base class, allowing derived classes to inherit and override methods.
- When creating a well-defined class hierarchy and relationships that truly reflect the real-world scenario.
When to Use Composition
Composition shines in scenarios where:
- You need to build complex systems from flexible, interchangeable components.
- You prefer loose coupling between components, enhancing maintainability and testability.
Real-World Examples
Consider a situation involving vehicles. Using inheritance, we may form a class hierarchy with `Vehicle` as a base and `Car`, `Bike`, etc., as derived classes. However, if a `Car` needs various types of engines (electric, hybrid), using composition allows for easily swapping these without altering the core `Car` class.
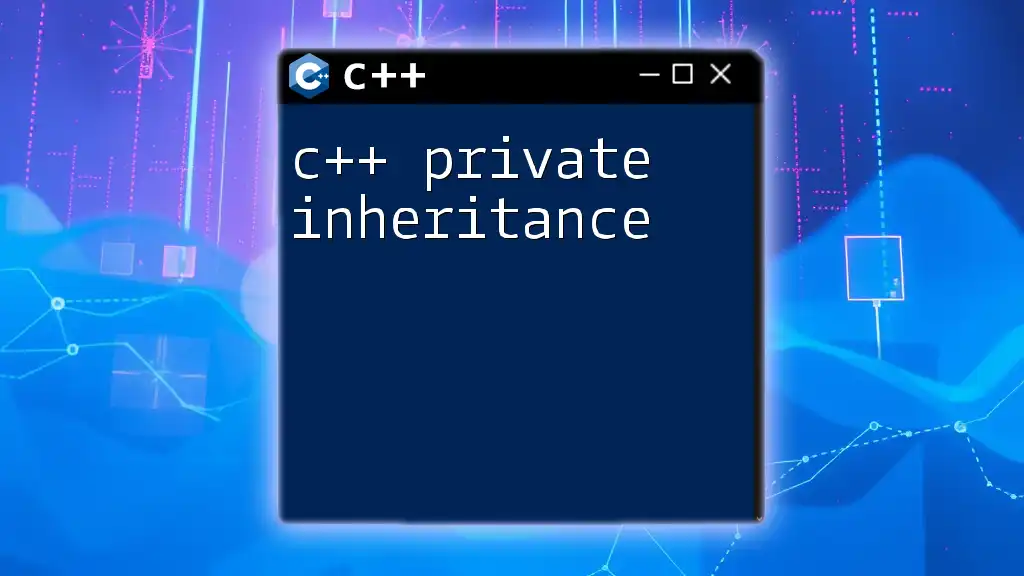
Composition and Inheritance Together in C++
Combining Both Techniques
C++ allows for using both inheritance and composition effectively. Keeping a balance between the two is essential; using inheritance for structural relationships and composition for behavioral relationships often yields the best design.
Code Example: Using Both Composition and Inheritance
class Engine {
public:
void start() { cout << "Engine starts"; }
};
class Vehicle { // Base class
public:
virtual void move() = 0; // Abstract method
};
class Car : public Vehicle { // Inheritance
private:
Engine engine; // Composition
public:
void start() { engine.start(); }
void move() { cout << "Car moves"; }
};
int main() {
Car car;
car.start(); // Output: Engine starts
car.move(); // Output: Car moves
}
In this example, `Car` inherits from `Vehicle` while also holding an `Engine` object, demonstrating how both principles can coexist harmoniously.
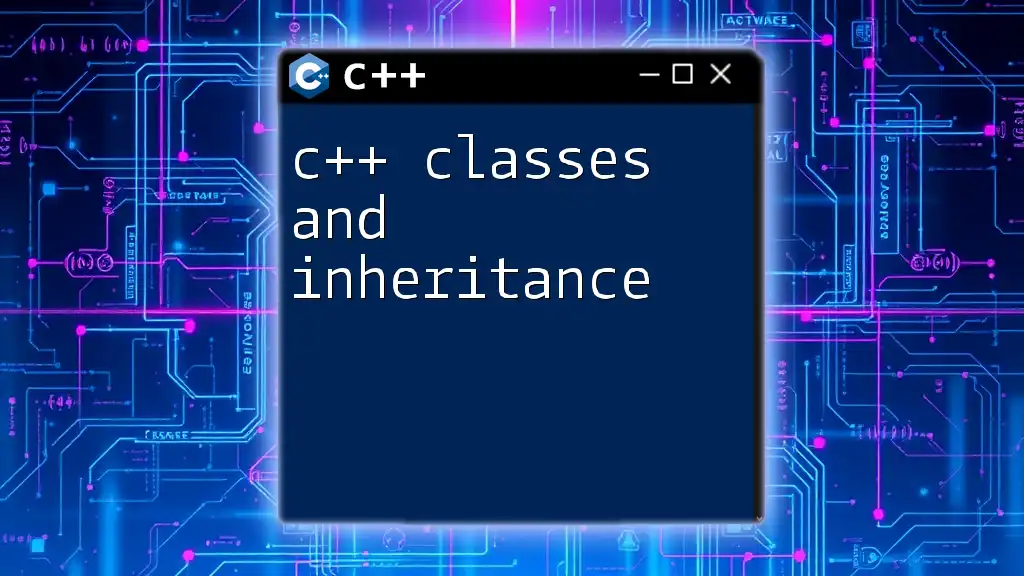
Key Takeaways
In the discussion of C++ composition vs inheritance, it is crucial to understand their distinctions and the contexts where each is appropriate. Inheritance provides a straightforward method to extend and build upon existing class features, whereas composition offers flexibility and a means to create complex behaviors through simpler components. Mastering both approaches enables developers to write robust, maintainable code that adheres to good OOP principles.
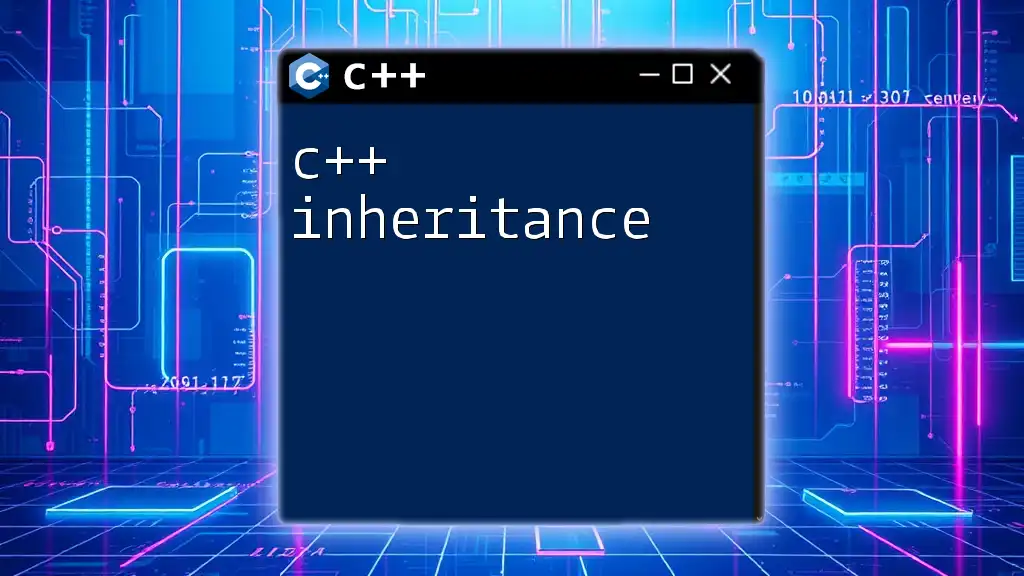
Conclusion
In summary, understanding the differences and applications of C++ composition and inheritance can significantly enhance your programming skills. Both paradigms play integral roles in OOP, allowing for more scalable, maintainable, and flexible software designs. As you advance in C++, consider the unique advantages each offers and apply them appropriately to maximize the effectiveness of your code.