C++ inheritance with virtual functions allows derived classes to override base class methods, enabling polymorphism, where the appropriate method is called based on the derived class type during runtime.
#include <iostream>
using namespace std;
class Base {
public:
virtual void show() { cout << "Base class show function called." << endl; }
};
class Derived : public Base {
public:
void show() override { cout << "Derived class show function called." << endl; }
};
int main() {
Base* b; // Base class pointer
Derived d; // Derived class object
b = &d; // Base class pointer pointing to Derived class object
b->show(); // Calls Derived's show function
return 0;
}
What is Virtual Inheritance?
Virtual inheritance is a special form of inheritance in C++ that allows classes to share a common base class in the presence of multiple derived classes, effectively resolving the Diamond Problem. In traditional inheritance, when two derived classes inherit from a single base class, and another class inherits from both of these derived classes, the base class becomes ambiguous for the final derived class. Virtual inheritance addresses this ambiguity by ensuring that only one instance of the base class exists regardless of how many times it has been derived.
Key Concepts of Virtual Inheritance
Understanding the Diamond Problem
The Diamond Problem presents a scenario where ambiguity arises in multiple inheritance. Consider the following class hierarchy:
- Base Class
- Derived Class 1 (inherits from Base)
- Derived Class 2 (inherits from Base)
- Final Derived Class (inherits from both Derived Class 1 and Derived Class 2)
In this hierarchy, `FinalDerived` would inherit two copies of `Base`, leading to ambiguity when accessing base class members. Virtual inheritance resolves this by ensuring that `FinalDerived` holds a single instance of `Base`.
Syntax of Virtual Inheritance
To declare a virtual base class in C++, you use the keyword `virtual` when inheriting from the base class. Here's how you can do it:
class Base {
public:
void display() { std::cout << "Base Class\n"; }
};
class Derived1 : virtual public Base { };
class Derived2 : virtual public Base { };
In this example, both `Derived1` and `Derived2` inherit from `Base` virtually. This setup guarantees that any class deriving from `Derived1` or `Derived2` will have only one instance of `Base`.
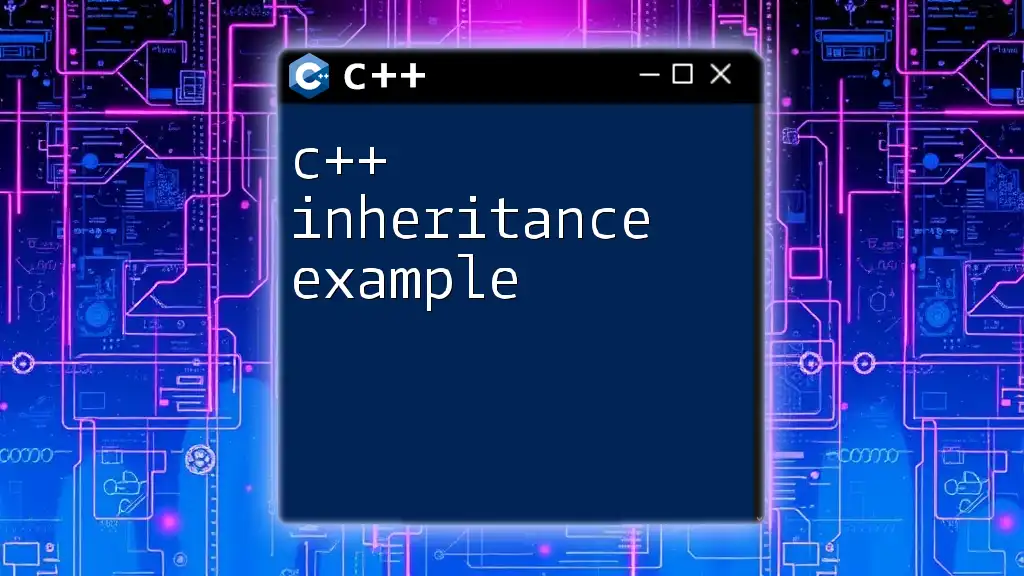
C++ Virtual Inheritance: Detailed Examples
Example 1: Basic Virtual Inheritance
Let's look at a simple implementation of virtual inheritance:
class Base {
public:
void show() { std::cout << "Base Class\n"; }
};
class Derived1 : virtual public Base {
public:
void show() { std::cout << "Derived1 Class\n"; }
};
class Derived2 : virtual public Base {
public:
void show() { std::cout << "Derived2 Class\n"; }
};
class FinalDerived : public Derived1, public Derived2 {
};
int main() {
FinalDerived obj;
obj.show(); // Output
obj.Base::show(); // Clarifying which 'show' to call
}
Explanation of the Example
In this code, both `Derived1` and `Derived2` classes inherit from a common base class `Base`. When we create an object of `FinalDerived`, we can invoke both its own methods and methods inherited from `Base`. However, if we only called `obj.show()`, C++ would choose to execute the `show` method from `Derived1` due to the way the inheritance hierarchy is structured. Explicitly calling `obj.Base::show();` allows us to directly access the base class method.
Example 2: Real-World Application
Consider a situation in an application managing pet sounds:
class Animal {
public:
void makeSound() { std::cout << "Animal Sound\n"; }
};
class Dog : virtual public Animal {
public:
void makeSound() { std::cout << "Bark\n"; }
};
class Cat : virtual public Animal {
public:
void makeSound() { std::cout << "Meow\n"; }
};
class Pet : public Dog, public Cat {
};
int main() {
Pet myPet;
myPet.makeSound(); // Output: Bark
myPet.Animal::makeSound(); // Output: Animal Sound
}
Explanation of the Real-World Example
In this example, `Dog` and `Cat` both derive from `Animal`. When we create an instance of `Pet`, calling `makeSound()` defaults to `Dog`'s implementation, which outputs "Bark". However, we can still access the `makeSound` method of `Animal` directly using `myPet.Animal::makeSound();`. This flexibility shows how virtual inheritance can aid in structuring a coherent class hierarchy in real-world applications.
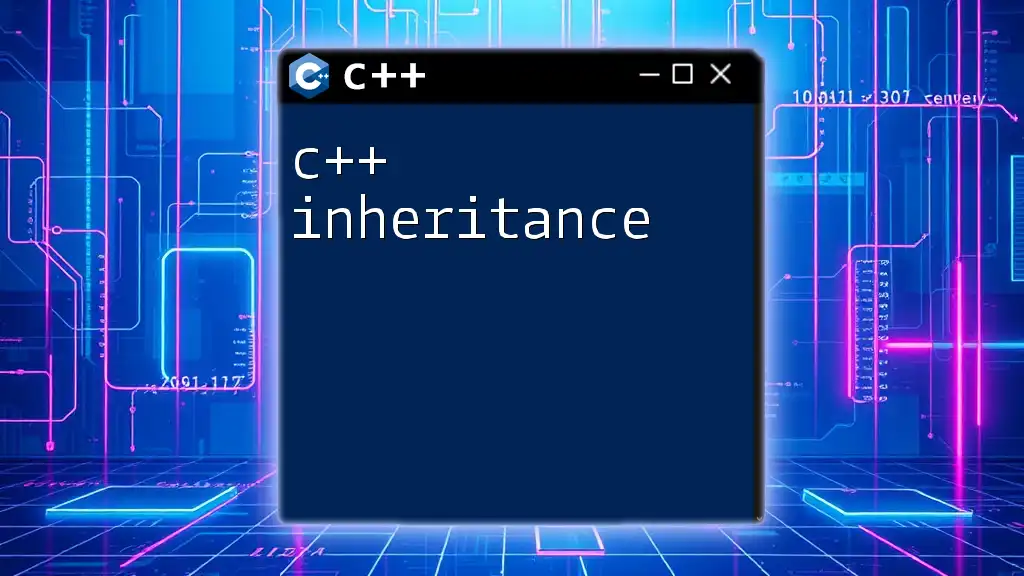
Benefits of Using Virtual Inheritance
Using virtual inheritance offers numerous advantages:
-
Reduces Ambiguity: It allows the derived classes to share a single instance of the base class, minimizing confusion about which base class method to invoke.
-
Encourages Code Reuse: Since only one object of the base class exists, it promotes reuse of base class functionality across multiple derived classes.
-
Enhances Maintainability: When modifications are made to the base class, they propagate through the hierarchy without the risk of having multiple copies causing discrepancies.
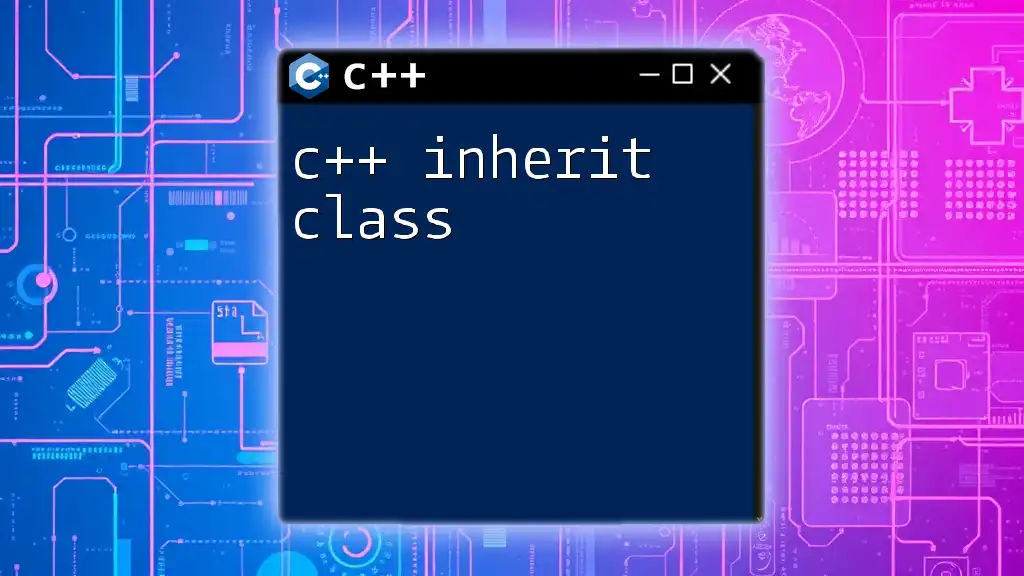
Limitations of Virtual Inheritance
Despite its benefits, virtual inheritance comes with its own set of limitations:
-
Increased Memory Usage: Each derived class may introduce additional overhead due to the necessity for pointers to manage the single instance of the base class.
-
Complexity: Designing a class hierarchy using virtual inheritance can complicate class interactions and may lead to challenging debugging scenarios.
-
Performance Considerations: Although the performance cost is generally negligible, the added indirection can potentially lead to performance hits under heavy use.
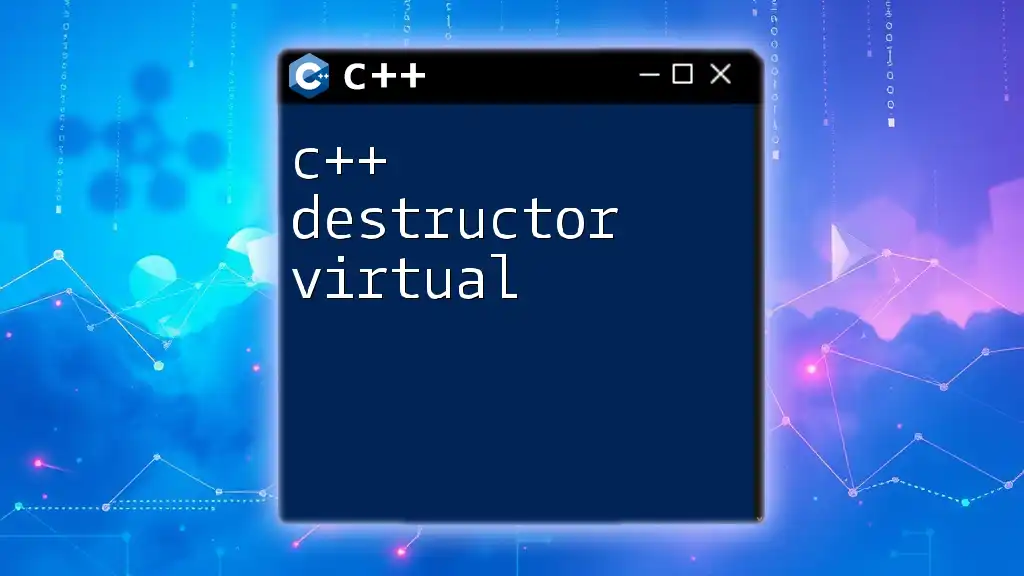
Conclusion
In summary, mastering C++ inheritance virtual is pivotal for developers aiming to construct efficient and maintainable object-oriented systems. By understanding how virtual inheritance consolidates instances of base classes and resolves ambiguity, programmers can effectively utilize this powerful feature in their software design.
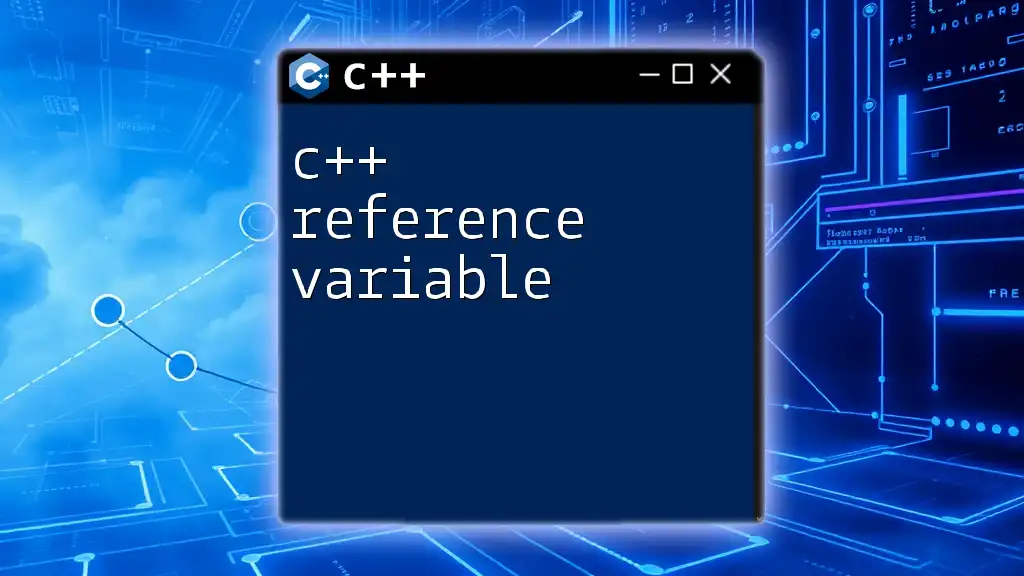
Additional Resources
For those looking to delve deeper into C++, consider the following resources:
- Books: "Effective C++" by Scott Meyers and "C++ Primer" by Stanley B. Lippman are excellent starting points.
- Online Courses: Platforms like Coursera and Udemy offer extensive courses on C++ programming.
- Community Engagement: Join forums such as Stack Overflow and Reddit’s r/cpp for discussions with fellow C++ enthusiasts.
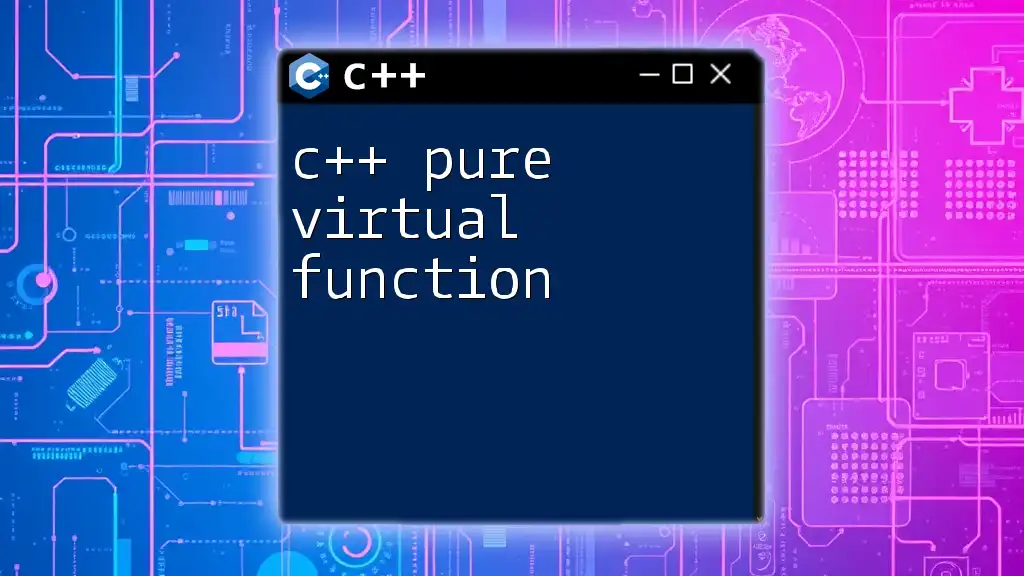
FAQs About C++ Virtual Inheritance
What is the purpose of virtual base classes?
Virtual base classes create a structure that mitigates ambiguity in multiple inheritance scenarios, making it clearer which base class members are accessible.
Can you have multiple virtual base classes?
Yes, it's possible to declare multiple virtual base classes in one class. However, this can lead to complexity and should be used judiciously.
How does virtual inheritance affect performance?
While the performance overhead associated with virtual inheritance is often minimal, it may impact efficiency due to added indirection. Nonetheless, the trade-off is often worth it for the clarity and maintainability it brings to a codebase.