In C++, include files allow you to incorporate external code, such as libraries or header files, into your program for reusability and functionality enhancement. Here’s a code snippet demonstrating how to include a standard library header:
#include <iostream>
Understanding C++ Include Header Files
Include files play a crucial role in organizing and managing C++ code. They allow programmers to separate declarations and implementations, leading to more manageable, reusable code.
What are Header Files?
Header files in C++ are files that typically contain declarations of functions, classes, and variables. They serve as an interface for the implementation files (the `.cpp` files), allowing code to be organized distinctly. Unlike regular source files, header files usually have the `.h` extension and are included at the top of your C++ source files using the `#include` directive.
Common Header Files in C++
C++ offers a wealth of standard libraries through header files. Some of the most frequently used standard header files include:
- `<iostream>`: Contains functionality for input and output using streams.
- `<vector>`: Provides a dynamic array implementation.
- `<string>`: Facilitates operations involving strings.
In addition to standard headers, programmers can create user-defined header files to encapsulate specific functionalities tailored to their applications.

Syntax of Including Files in C++
The Include Directive
The `#include` preprocessor directive is essential in C++. It tells the compiler to include the content of a specified file into the current source file. The syntax varies slightly based on whether you are including standard libraries or user-defined headers.
Including Standard Header Files
When you want to utilize standard libraries, you typically include them using angle brackets. For instance:
#include <iostream>
This line instructs the compiler to look within the system directories for the specified header.
Including User-defined Header Files
User-defined headers, which you're likely to create, are included with quotes. Here's how you would include a custom header file named `myHeader.h`:
#include "myHeader.h"
This tells the compiler to first search in the local directory for the specified file before looking elsewhere.
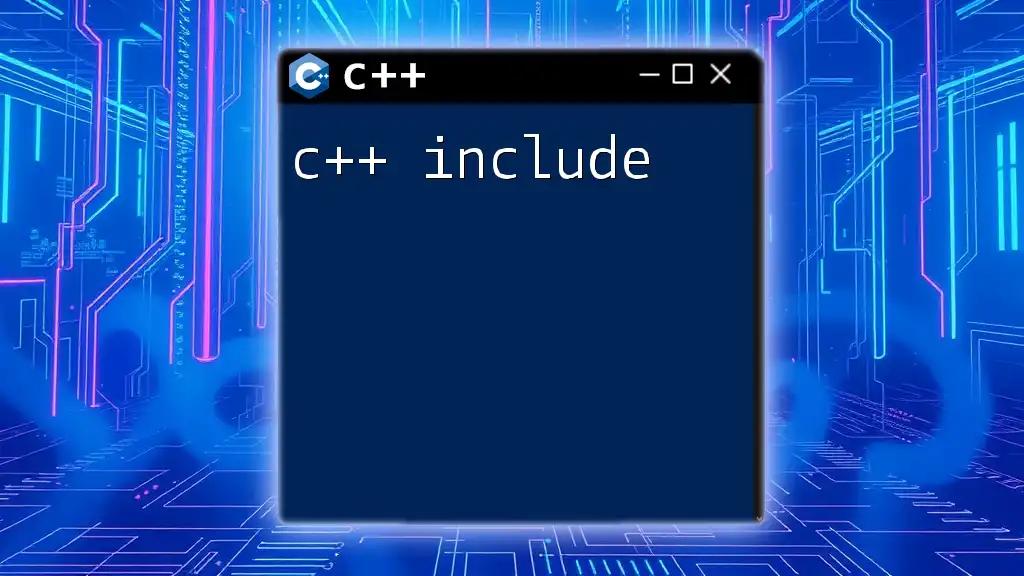
Types of Include Files in C++
Angle Bracket vs. Quotes
Understanding the difference between using angle brackets (`< >`) and quotes (`" "`) is crucial.
- Angle Brackets (`<filename>`): When you use this format, the preprocessor searches for header files in the system directories. This should be used for standard library files.
- Quotes (`"filename"`): This format tells the preprocessor to search the local directory for the file. If it is not found, then it will search the system directories, making it ideal for custom header files.
Precompiled Header Files
Precompiled headers serve to speed up the compilation time, especially for large projects. By compiling frequently used headers into a single file and including that precompiled file, developers can enhance build times significantly. For usage, you'll typically start your header file with:
#include "stdafx.h" // Example for Visual Studio

How to Create and Use Include Files in C++
Creating a Header File
Creating a header file involves defining the functions and classes you intend to use. For example, you could create a header file named `mathFunctions.h`:
// mathFunctions.h
#ifndef MATHFUNCTIONS_H
#define MATHFUNCTIONS_H
int add(int a, int b);
int subtract(int a, int b);
#endif
In this snippet, `#ifndef`, `#define`, and `#endif` are preprocessor directives that prevent multiple inclusions, ensuring that the header file is only processed once.
Implementing Functions from Header Files
After defining your functions in the header file, you need to implement them in a separate source file, say `mathFunctions.cpp`:
// mathFunctions.cpp
#include "mathFunctions.h"
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
Using the Header File in a Program
Finally, you can link your header and implementation files in a main program file, `main.cpp`, like so:
// main.cpp
#include <iostream>
#include "mathFunctions.h"
int main() {
std::cout << "Sum: " << add(5, 3) << std::endl;
std::cout << "Difference: " << subtract(5, 3) << std::endl;
return 0;
}
This structure allows you to build applications in a modular way, encouraging code reuse.
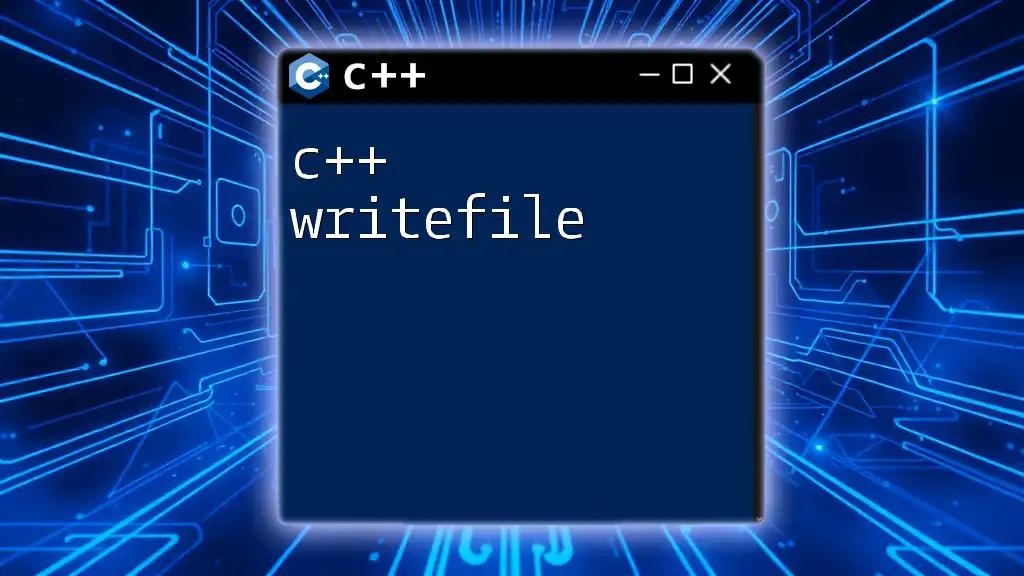
Best Practices for Using Include Files
Guarding Against Multiple Inclusions
To avoid the pitfalls of multiple inclusions—which can trigger linking errors—it is essential to implement include guards. These are simple yet effective preprocessor directives (`#ifndef`, `#define`, `#endif`) that prevent the same header file from being included multiple times in a single compilation unit.
Organizing Header Files
Effective organization of header files enhances readability and maintainability. Group related functions and classes into dedicated headers. For example, if you are building a math library, it may be wise to create separate headers for calculus, algebra, and statistics.
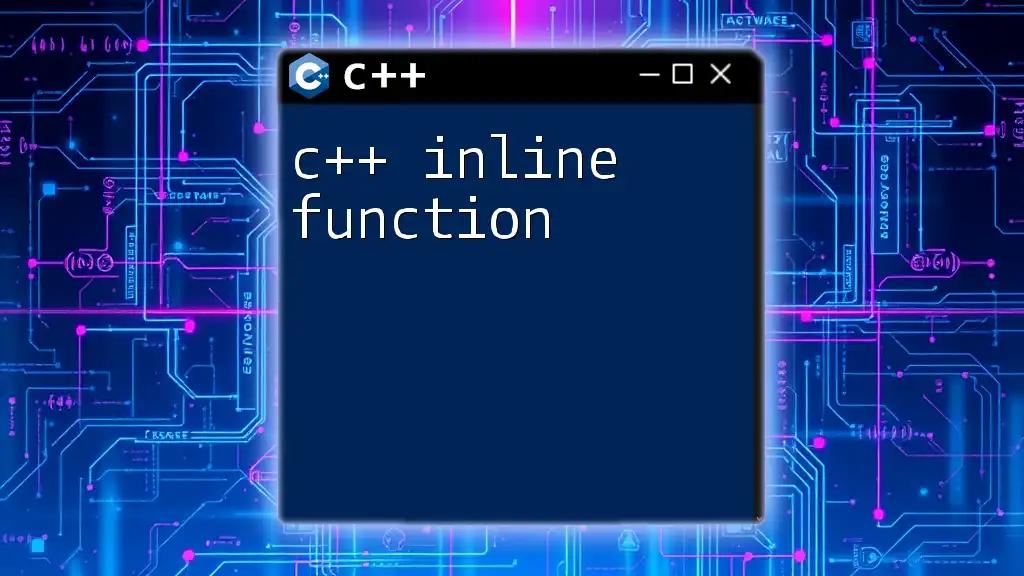
Common Issues and Troubleshooting
Compilation Errors Related to Include Files
When you encounter compilation errors related to include files, two common problems arise:
- File Not Found: This often occurs if the file path is incorrect or if the file is missing. Double-check the path and confirm the file exists.
- Multiple Definition Errors: This may happen if the same symbols are declared in different files without proper include guards. Ensure that your headers use guards correctly.
Circular Dependencies
Circular dependencies occur when two or more header files include each other, leading to confusion during compilation. Resolving this often requires rethinking your design. In many cases, using forward declarations can help solve this issue.

Conclusion
In conclusion, C++ include files are vital in programming, serving as the bedrock for a modular, organized code structure. By understanding and properly utilizing include files and header files, you can significantly enhance your workflow and code maintainability. Embrace the practice of creating and using header files to streamline your development process!
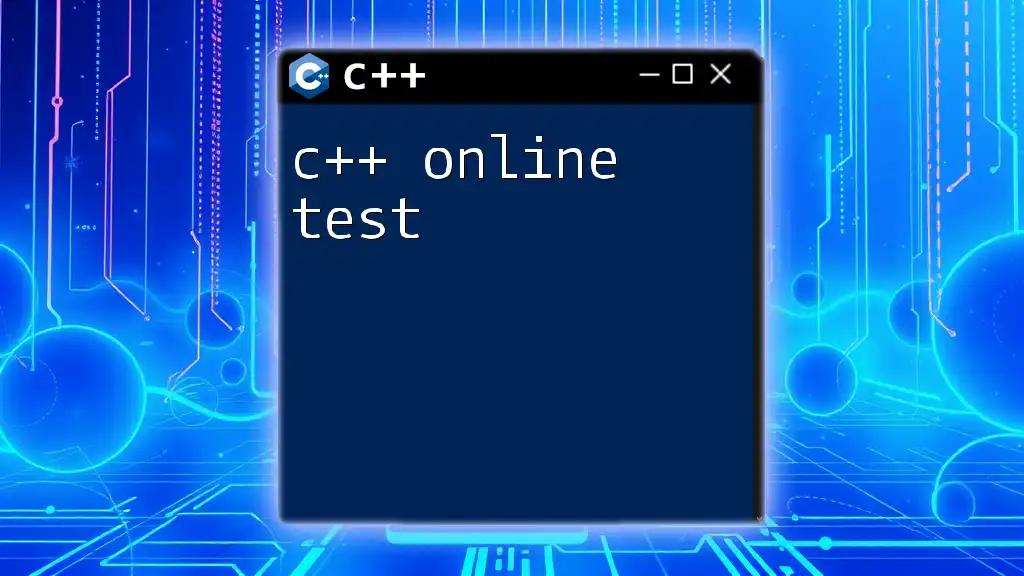
Additional Resources
For further reading and to deepen your understanding, consider looking into C++ programming books or online tutorials that cover advanced topics on header files and code organization.