To get the file size in C++, you can use the `std::ifstream` class along with `std::ifstream::seekg` and `std::ifstream::tellg` to determine the size of a file in bytes.
Here's a code snippet demonstrating this:
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("example.txt", std::ios::binary);
file.seekg(0, std::ios::end);
std::streampos fileSize = file.tellg();
file.close();
std::cout << "File size: " << fileSize << " bytes." << std::endl;
return 0;
}
Understanding File Handling in C++
What is File Handling?
File handling in C++ refers to the processes of creating, reading, updating, and deleting files on the filesystem. It is a crucial aspect of programming, allowing applications to interact with data stored outside of their runtime environments. Mastering file handling in C++ involves understanding streams, which are abstractions that allow reading and writing data.
Why Knowing File Size is Important
Understanding how to retrieve file sizes can significantly benefit various programming tasks. For example, you may need to ensure efficient resource management, verify if a file meets certain specifications before processing, or optimize loading times for applications that handle large amounts of data. Knowing file size is vital in performance-critical applications, data streaming, and resource-constrained environments.
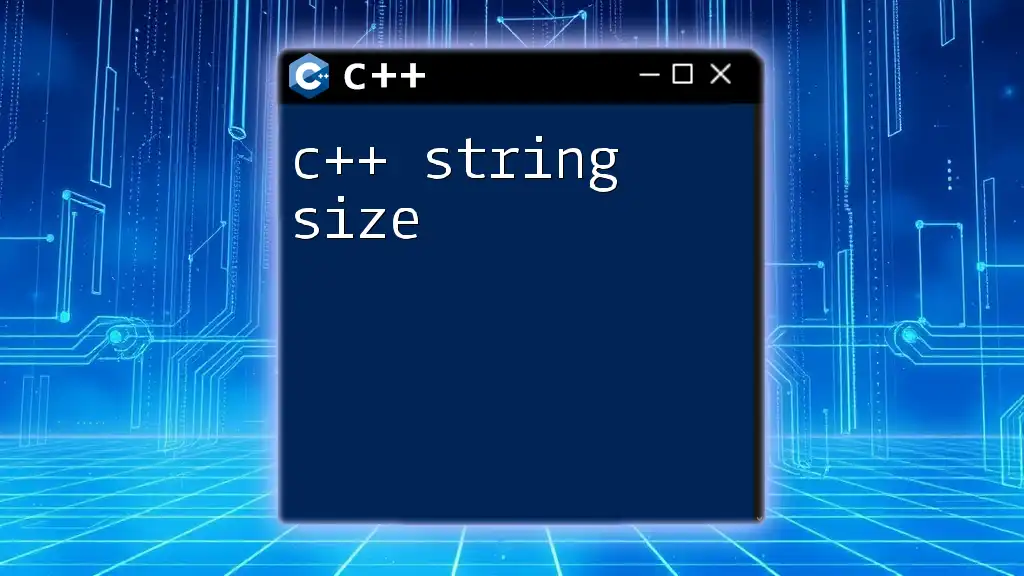
Methods to Get File Size in C++
Using `<fstream>` Library
The `<fstream>` library in C++ provides a means to handle files through input and output streams. This library supports processes like file reading, writing, and obtaining file metadata.
Example: Using `std::ifstream`
To get the size of a file using `std::ifstream`, you essentially open the file, seek to the end to determine the length, and get the current position of the stream pointer. Here’s how you can do it:
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("example.txt", std::ios::binary);
if (file) {
file.seekg(0, std::ios::end); // Move the cursor to the end
std::streampos size = file.tellg(); // Get current position
std::cout << "File size: " << size << " bytes" << std::endl;
} else {
std::cerr << "File not found." << std::endl;
}
return 0;
}
Explanation of the code:
- The program opens `example.txt` in binary mode using `std::ifstream`.
- `seekg(0, std::ios::end)` moves the file pointer to the end of the file, allowing us to measure the total length.
- `tellg()` returns the current position of the stream, which corresponds to the file size in bytes.
Using `stat()` Function (POSIX Systems)
For applications running on POSIX compliant systems (like Linux and macOS), the `stat` function provides a straightforward way to access file metadata, including file size.
Example: Using `stat()`
Here’s how to utilize the `stat()` function to get a file size:
#include <iostream>
#include <sys/stat.h>
int main() {
struct stat file_info;
if (stat("example.txt", &file_info) == 0) {
std::cout << "File size: " << file_info.st_size << " bytes" << std::endl;
} else {
std::cerr << "File not found." << std::endl;
}
return 0;
}
Explanation of the code:
- The `stat` function populates the `file_info` structure with metadata about `example.txt`.
- The `st_size` member of the struct contains the file size in bytes. It’s returned directly, making the process efficient.
- Always check the return value of `stat()` to ensure the file exists before proceeding.
Using Modern C++ (C++17 and Above)
As of C++17, the `<filesystem>` library was introduced, which makes file handling even easier and more intuitive. This library allows developers to perform various file operations in a concise manner.
Example: Using `std::filesystem`
Below is an example of how to obtain a file size using `std::filesystem`:
#include <iostream>
#include <filesystem>
int main() {
std::filesystem::path file_path("example.txt");
if (std::filesystem::exists(file_path)) {
auto file_size = std::filesystem::file_size(file_path);
std::cout << "File size: " << file_size << " bytes" << std::endl;
} else {
std::cerr << "File not found." << std::endl;
}
return 0;
}
Explanation of the code:
- The code first defines a `std::filesystem::path` object that represents the file.
- `std::filesystem::exists` checks for the existence of the file.
- `std::filesystem::file_size` fetches the size directly, providing a clean and standard way to obtain this information compared to previous methods.
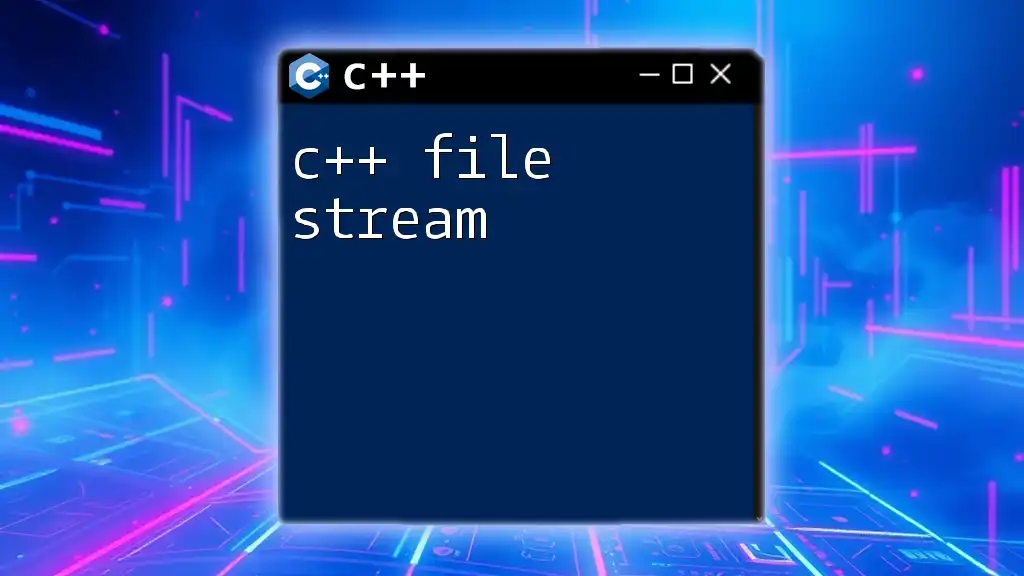
Comparison of Methods
Pros and Cons
-
`std::ifstream` Method
- Benefits:
- Simple and straightforward.
- Widely used across various C++ codebases.
- Drawbacks:
- Requires moving the cursor in the file, which may not be as efficient for large files.
- Benefits:
-
`stat()` Function
- Benefits:
- Provides direct access to various file attributes.
- Works well on POSIX systems, offering reliable performance.
- Drawbacks:
- Limited to operating systems that support the POSIX standard.
- Benefits:
-
`std::filesystem`
- Benefits:
- Offers a more modern, user-friendly API.
- Simplifies file operations, making code more readable.
- Drawbacks:
- Requires C++17 or higher, which may not be available in older compilers or environments.
- Benefits:
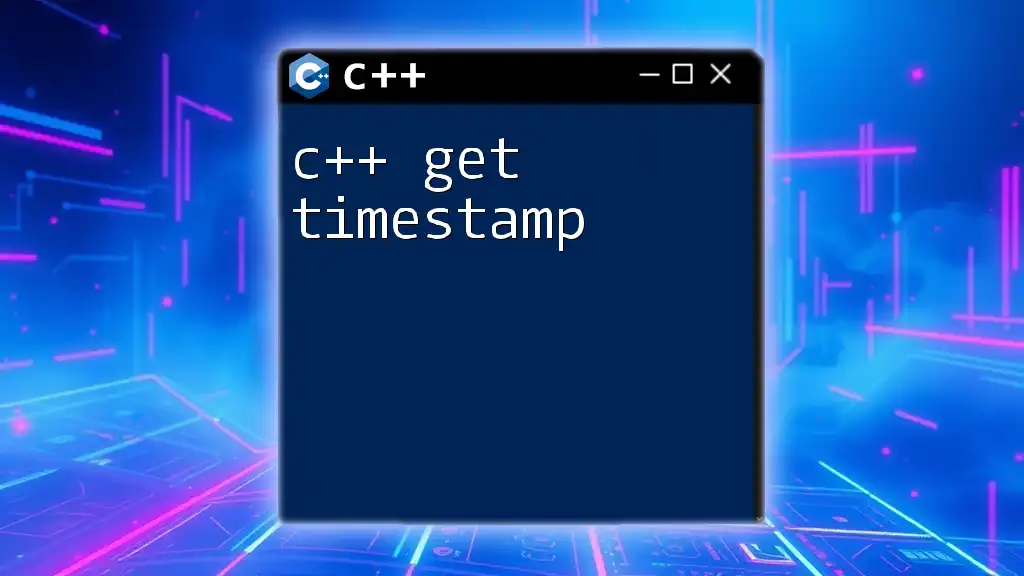
Best Practices for File Size Retrieval
When retrieving file sizes, consider the following best practices:
- Always check for file existence before attempting to retrieve its size. This prevents unnecessary errors and exceptions.
- Implement error handling mechanisms to provide a graceful recovery or notification when a file is not accessible.
- Keep performance in mind, especially when dealing with large files. Avoid reading files multiple times if only their sizes are needed.
- If your code will run in various environments, consider providing compatibility options for different ways of retrieving file sizes.
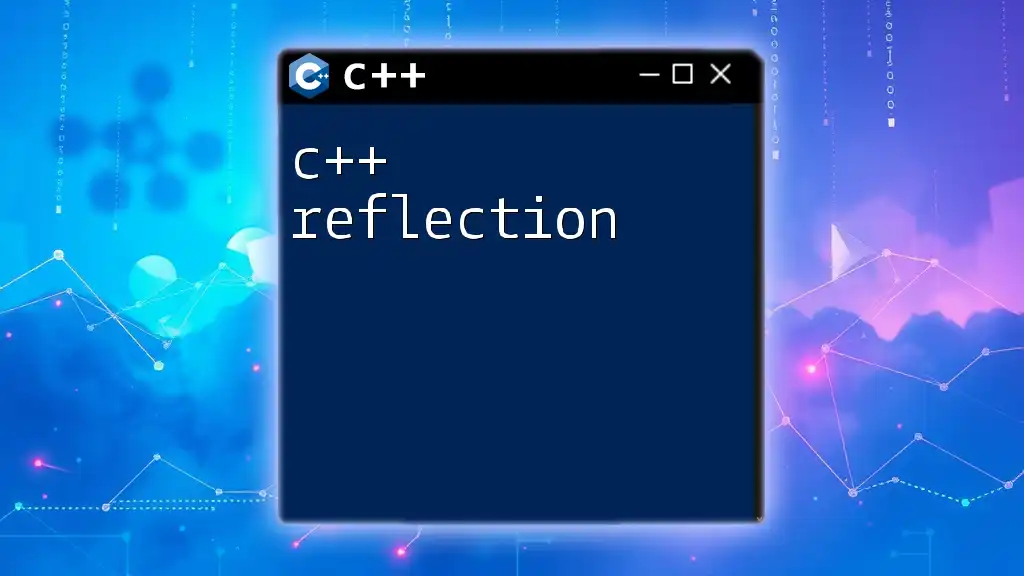
Conclusion
In summary, understanding how to c++ get file size is key to effective file handling. By utilizing methods such as `<fstream>`, `stat()`, and modern approaches like `<filesystem>`, you can manage file operations with ease and efficiency. Each method offers unique advantages depending on your project's requirements, and being proficient in these techniques will enhance your programming skills.
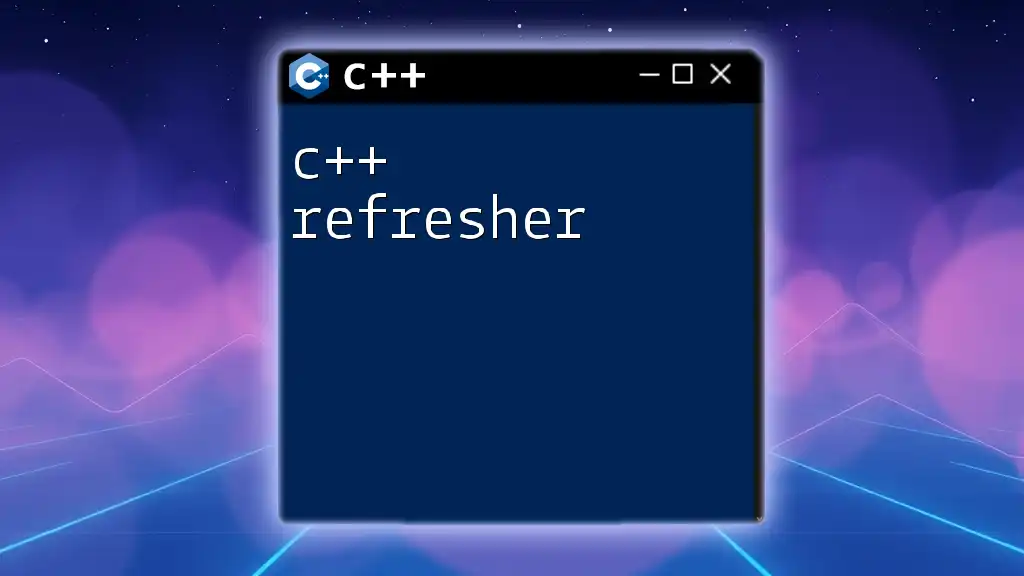
Call to Action
Consider experimenting with the examples provided in this article. Test out different methods to see which one fits your needs best. Share your experiences or any questions you have in the comments below, and don’t forget to follow our blog for more quick C++ tips and tutorials to enhance your programming journey!