A C++ bit array is a compact representation of an array of bits, allowing for efficient storage and manipulation of binary data.
Here’s a simple example of how to use a bit array in C++:
#include <iostream>
#include <vector>
class BitArray {
public:
BitArray(size_t size) : bits((size + 7) / 8, 0), size(size) {}
void set(size_t index) {
if (index < size) bits[index / 8] |= (1 << (index % 8));
}
void clear(size_t index) {
if (index < size) bits[index / 8] &= ~(1 << (index % 8));
}
bool get(size_t index) const {
return index < size && (bits[index / 8] & (1 << (index % 8))) != 0;
}
private:
std::vector<uint8_t> bits;
size_t size;
};
int main() {
BitArray bitArray(10);
bitArray.set(2);
std::cout << bitArray.get(2); // Output: 1
bitArray.clear(2);
std::cout << bitArray.get(2); // Output: 0
return 0;
}
Understanding Bit Arrays in C++
What is a Bit Array?
A bit array is an efficient data structure that compactly stores a sequence of bits, where each bit represents a binary value of either 0 or 1. Unlike traditional arrays, which store larger data types (like integers or characters), a C++ bit array uses each bit to represent information, significantly reducing memory usage.
The advantages of using bit arrays are numerous. They provide optimal storage for states in applications that require large sets of on/off flags, like bitmap graphics or configuration settings. Understanding the differences in memory usage between a standard integer array and a bit array can have significant implications for performance and storage efficiency.
Memory Efficiency
Bit arrays excel in memory efficiency. One bit can represent a boolean state, while an integer array (which typically consists of 4 bytes per integer) may be an overkill for simple on/off states.
For example, consider the storage of 100 boolean values. An integer array would require:
- 4 bytes per integer × 100 integers = 400 bytes.
In contrast, a bit array would require only:
- 1 byte for 8 bits × 13 bytes (for 104 bits) = 13 bytes.
This stark difference emphasizes the effectiveness of bit arrays in memory-constrained environments.
#include <bitset>
#include <iostream>
int main() {
std::bitset<100> myBitArray; // Bit array for 100 bits
std::cout << "Size of myBitArray: " << myBitArray.size() / 8 << " bytes" << std::endl; // Output in bytes
return 0;
}
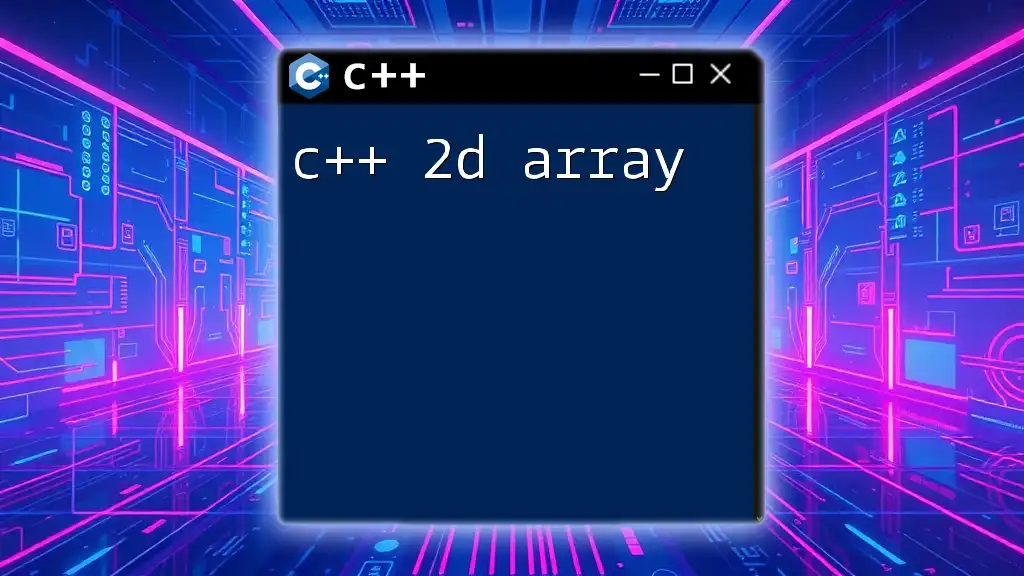
Creating a Bit Array in C++
Using Standard Template Library (STL) `std::bitset`
The C++ Standard Template Library (STL) provides the `std::bitset` class for implementing bit arrays. This class allows you to define a bit array of a fixed size, which can be specified at compile time.
In its simplest form, you can define it using the following syntax:
#include <bitset>
#include <iostream>
int main() {
std::bitset<8> myBits; // A bit array with 8 bits
myBits.set(0); // Set the first bit to 1
myBits.set(2); // Set the third bit to 1
std::cout << myBits << std::endl; // Output: 00000101
return 0;
}
In this example, we create a bit array of 8 bits, setting specific bits to represent their state.
Implementing a Custom Bit Array Class
Designing the Class
For more complex needs, you might want to implement a custom bit array class. A basic bit array class could encapsulate an `unsigned int` to store bits while providing member functions to manipulate the bits.
Here is a basic structure of such a class:
#include <iostream>
#include <vector>
class BitArray {
public:
BitArray(size_t size) : size(size), bits((size + 31) / 32, 0) {}
void set(size_t position) {
bits[position / 32] |= (1 << (position % 32));
}
void clear(size_t position) {
bits[position / 32] &= ~(1 << (position % 32));
}
bool get(size_t position) const {
return bits[position / 32] & (1 << (position % 32));
}
private:
size_t size;
std::vector<unsigned int> bits;
};
This simple class can store bits and provides methods to set, clear, and check their state.
Adding Functionality
You can expand the bit array class to include additional functionality such as toggling bits and iterating through them.
void toggle(size_t position) {
bits[position / 32] ^= (1 << (position % 32));
}
bool any() const {
for (unsigned int word : bits) {
if (word) return true;
}
return false;
}
Working with Bit Arrays
Manipulating Bit Arrays
The ability to manipulate bits is crucial for using bit arrays effectively. The `set()` method assigns a bit to `1`, while the `clear()` method assigns it to `0`.
Here's how you can use this functionality:
BitArray arr(10);
arr.set(3);
arr.set(5);
std::cout << arr.get(3) << std::endl; // Output: 1
arr.clear(3);
std::cout << arr.get(3) << std::endl; // Output: 0
Accessing Bit Values
Accessing individual bits can be straightforward with methods defined in the bit array class. Using `get()`, you can check the state of a particular bit.
for (size_t i = 0; i < 10; ++i) {
std::cout << "Bit " << i << " is: " << arr.get(i) << std::endl;
}
Iterating Through a Bit Array
To iterate through the bits of a bit array, you can implement a loop that checks each bit's state.
for (size_t i = 0; i < arr.size(); ++i) {
std::cout << "Bit " << i << ": " << (arr.get(i) ? "1" : "0") << std::endl;
}
Functional Operations on Bit Arrays
AND, OR, NOT Operations
Bitwise operations allow you to perform logical operations across multiple bit arrays. Suppose you want to perform an AND operation between two bit arrays. Here's an example implementation:
BitArray andOperation(const BitArray &a, const BitArray &b) {
BitArray result(a.size());
for (size_t i = 0; i < a.size(); ++i) {
if (a.get(i) && b.get(i)) {
result.set(i);
}
}
return result;
}
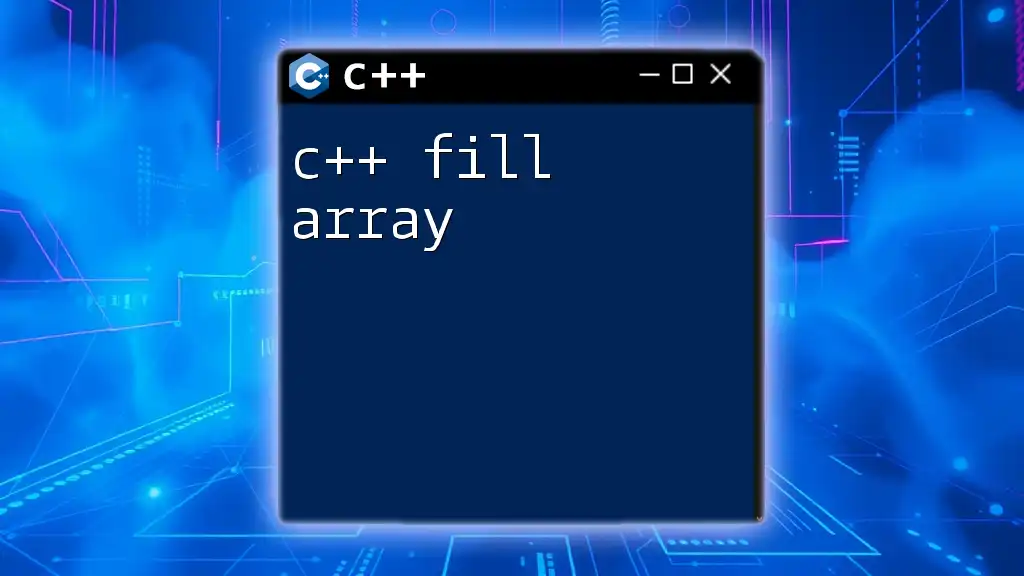
Performance Considerations
Time Complexity
The time complexity for querying, setting, or clearing bits in a bit array is O(1). This efficiency can be crucial when handling large datasets or performing frequent updates.
When to Use Bit Arrays
Bit arrays are particularly beneficial in scenarios where memory consumption is critical, and operations on individual bits are frequent. Compare them to vectors or lists that use larger data types, resulting in unnecessary memory overhead.
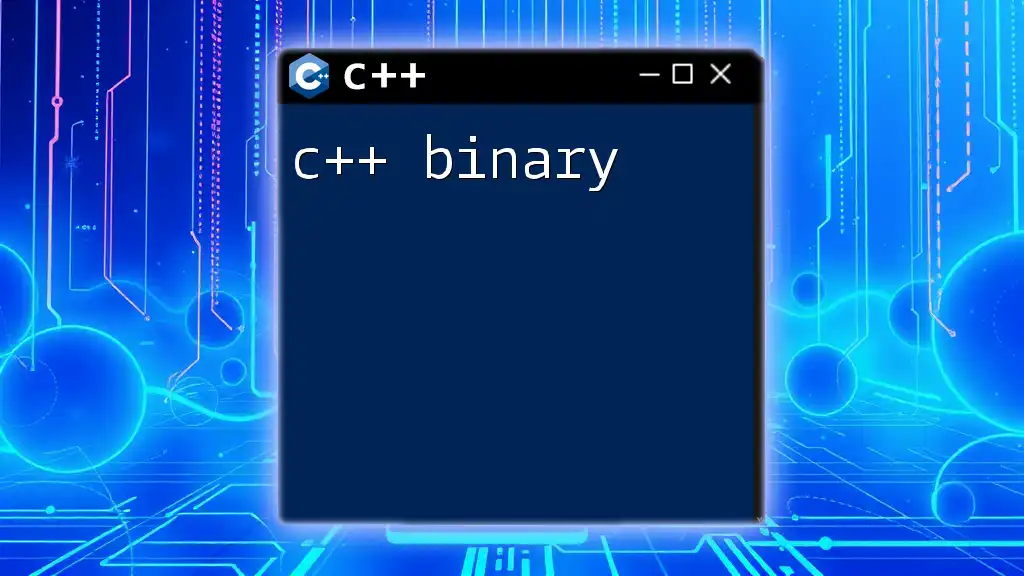
Advanced Topics
Bit Arrays in Multi-threading
When integrating bit arrays into multi-threaded applications, it’s essential to account for concurrent access. You might need mutexes to ensure thread safety, especially when performing write operations:
#include <mutex>
class ThreadSafeBitArray : public BitArray {
public:
void safeSet(size_t position) {
std::lock_guard<std::mutex> lock(mutex_);
set(position);
}
private:
std::mutex mutex_;
};
Serialization of Bit Arrays
In applications where you need to save and load the state of a bit array, serialization becomes necessary. You can implement serialization using byte arrays:
std::vector<unsigned char> serialize() const {
std::vector<unsigned char> data(bits.size() * sizeof(unsigned int));
std::memcpy(data.data(), bits.data(), data.size());
return data;
}
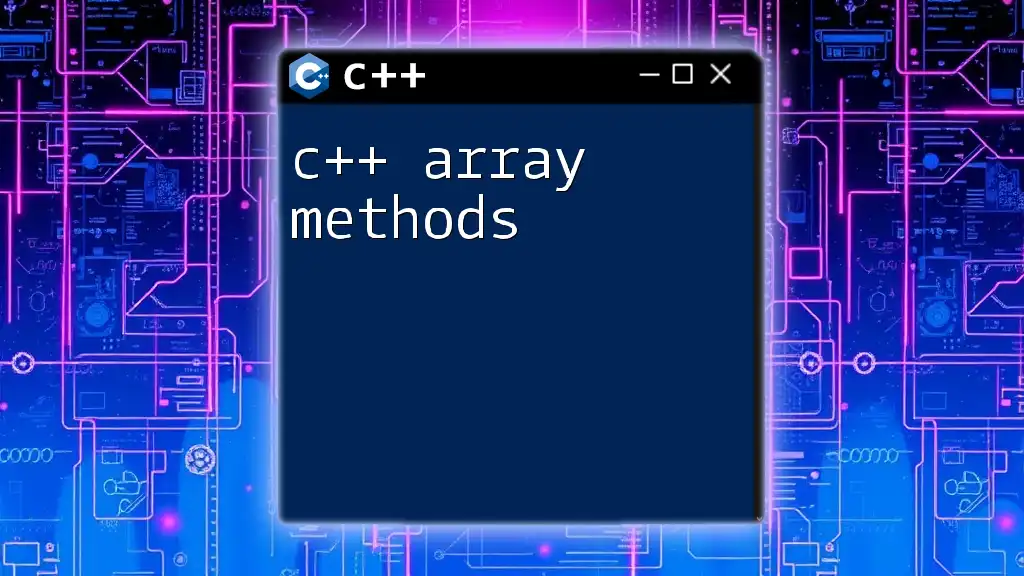
Real-world Applications
Use Cases in Games and Graphics
In game development, bit arrays can represent object states or collision flags efficiently. For example, a large number of projectiles might only need a few bits to indicate their presence or absence on the screen.
Bit Arrays in Machine Learning and Data Science
Bit arrays can optimize data processing in machine learning by compactly storing boolean flags or features, thus reducing memory usage and improving performance in algorithms that heavily depend on large datasets.
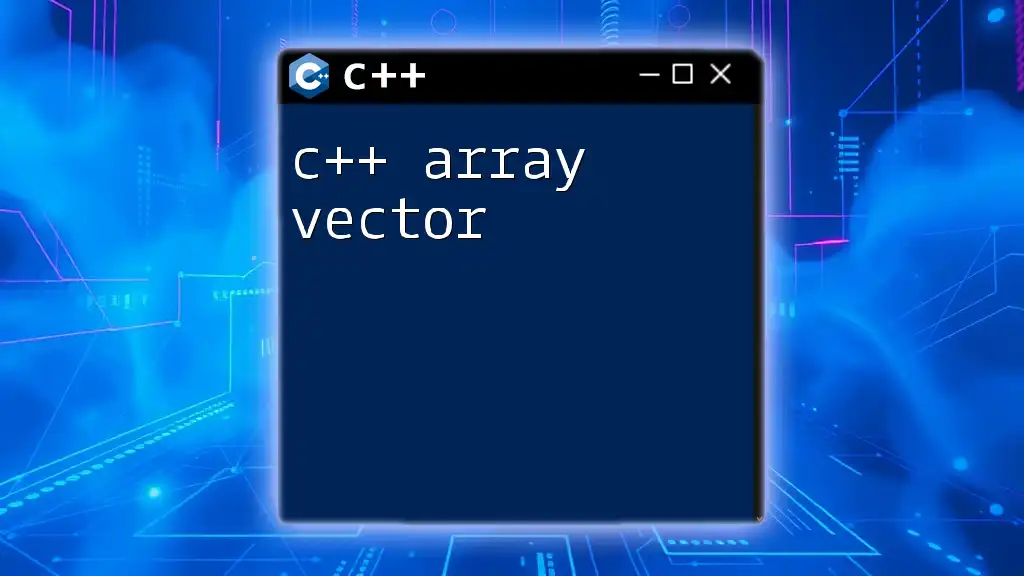
Conclusion
In this comprehensive guide, we delved into the world of C++ bit arrays and understood their significance in memory-efficient data manipulation. Through the use of both STL's `std::bitset` and custom implementations, we explored how bit arrays can be applied across various fields like game development and machine learning. As you further explore C++ programming, don't hesitate to experiment with bit arrays to harness their full potential for your projects.