The C++ "bit or" operation, represented by the `|` operator, performs a bitwise OR between two integers, resulting in a number that has bits set to 1 wherever either of the original integers has a bit set to 1.
Here’s an example:
#include <iostream>
int main() {
int a = 5; // Binary: 0101
int b = 3; // Binary: 0011
int result = a | b; // Result: 0111 (7)
std::cout << "Result of bitwise OR: " << result << std::endl; // Output: 7
return 0;
}
What is the C++ Bitwise OR Operator?
The C++ Bitwise OR Operator is a fundamental part of C++ programming that allows you to perform operations on the individual bits of an integer. Its syntax is straightforward and involves using the vertical bar (`|`). The bitwise OR operator is crucial for manipulating binary representation directly, making it a powerful tool in systems programming, graphics development, and anywhere low-level data manipulation is necessary.
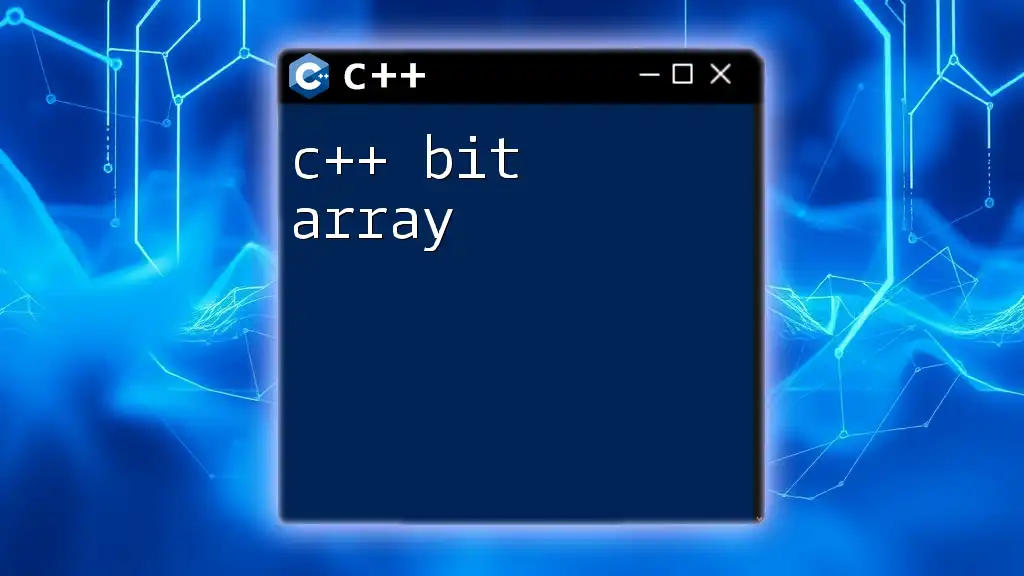
Understanding Bitwise Operations in C++
In C++, bitwise operations enable programmers to manipulate data at the bit level. This efficiency can lead to significantly optimized performance in certain applications. Understanding how these operations work alongside logical operators is essential for effective programming.
Types of Bitwise Operators in C++
C++ provides several bitwise operators:
-
C++ Bitwise AND Operator (`&`): Compares each bit of two operands and returns a new integer whose bits are set to 1 only where both operands have bits set to 1.
int a = 5; // 0101 in binary int b = 3; // 0011 in binary int result = a & b; // result will be 1 (0001 in binary)
-
C++ Bitwise OR Operator (`|`): This operator works by comparing each bit and returning a new integer with bits set to 1 wherever at least one of the operands has a corresponding bit set to 1.
int a = 5; // 0101 in binary int b = 3; // 0011 in binary int result = a | b; // result will be 7 (0111 in binary)
-
C++ Bitwise XOR Operator (`^`): Sets each bit to 1 where the corresponding bits of either but not both operands are 1.
-
C++ Bitwise NOT Operator (`~`): Flips all bits of its operand.
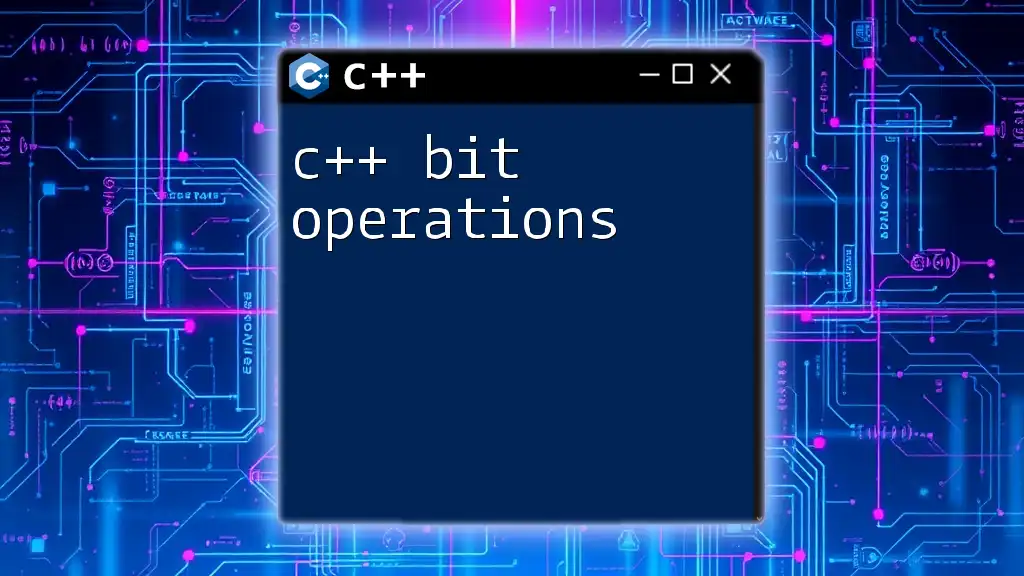
How Does the Bitwise OR Work?
When using the C++ bitwise OR operator, each bit position in the operands is compared. If at least one of the bits is 1, the corresponding result bit will also be 1. This can be visualized through binary representation:
For example, the operation `5 | 3` becomes:
0101 (5 in binary)
| 0011 (3 in binary)
------------
0111 (7 in binary)
This is a simple yet powerful operation. The Practical Examples of Bitwise OR in Action include:
-
Combining Flags: In many applications, flags are represented as bits within an integer. Using bitwise OR allows combining multiple flags into one integer without losing any individual flag information.
const int FLAG_A = 1; // 0001 const int FLAG_B = 2; // 0010 const int FLAG_C = 4; // 0100 int combinedFlags = FLAG_A | FLAG_B; // 0011
-
Setting Bits: The bitwise OR can be used effectively to set specific bits in an integer without affecting other bits.
int num = 0; // 0000 num |= 4; // Set the third bit, num becomes 0100
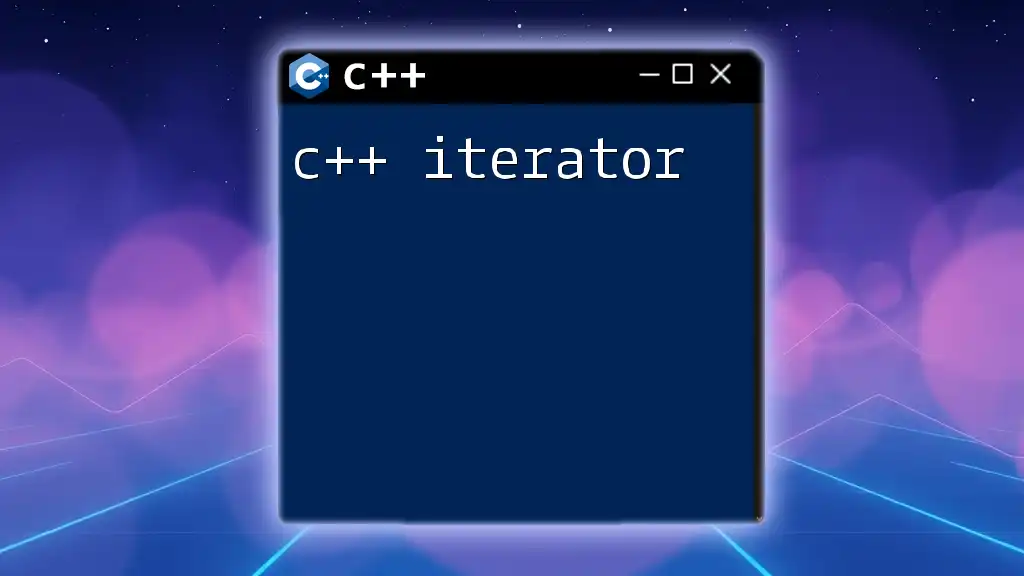
Important Properties of C++ Bitwise OR
Understanding the properties of the bitwise OR operator enhances its usefulness. The most notable properties are:
-
Associative Property: `(a | b) | c` is equal to `a | (b | c)`.
-
Commutative Property: `a | b` is equal to `b | a`.
These properties can simplify expressions and improve clarity in code.
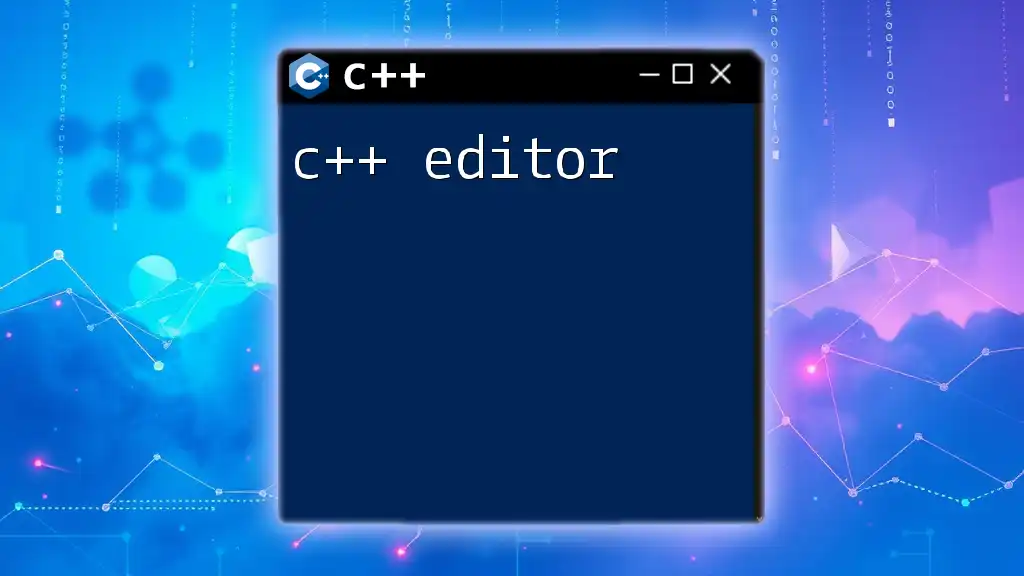
When to Use Bitwise OR in C++
Using the C++ Bit OR operator can often lead to Performance Optimization. For instance, operations that manipulate flags are significantly faster when performed using bitwise operators compared to using regular arithmetic or conditional statements.
Practical use cases include:
- Combining multiple error flags or status bits.
- Configuring settings where binary on-off (true/false) states are necessary.
By using the bitwise OR operator, developers can efficiently handle multiple states without adding excessive computational overhead.
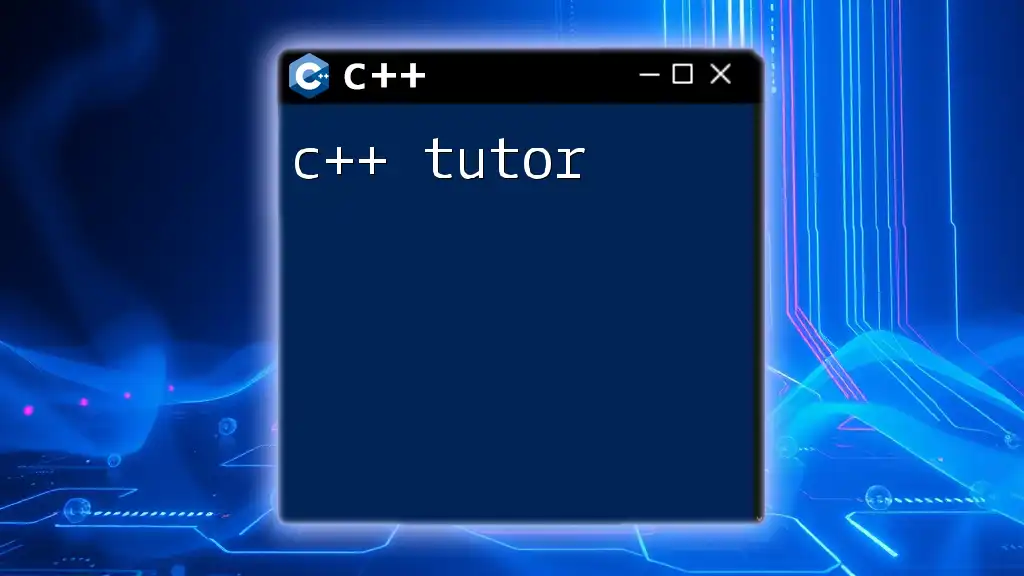
Common Mistakes and Pitfalls
Misusing the bitwise OR operator can lead to confusion and bugs in your code.
One of the most common misconceptions is the difference between the Bitwise OR operator (|) and the Logical OR operator (||). While the Bitwise OR evaluates each bit, the Logical OR evaluates the overall truthiness of expressions.
For instance:
bool result = (a | b) || (c | d); // Misleading usage
This code will evaluate each operand in the bitwise context first, which may not produce the intended logical outcome.
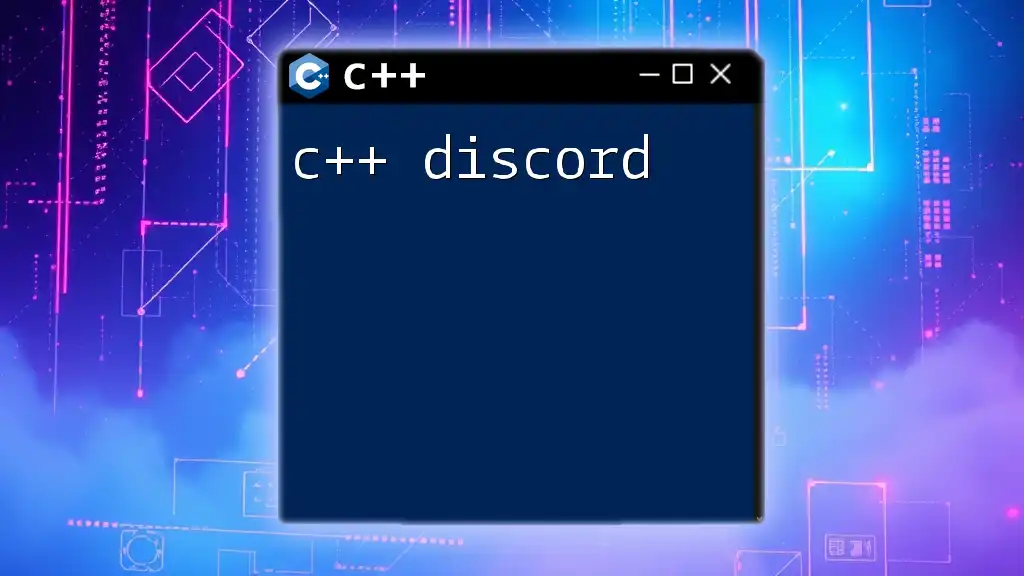
Practical Examples
Example 1: Using Bitwise OR in Flag Manipulation
Consider manipulating various status flags using the bitwise OR operator:
#include <iostream>
enum Permissions {
READ = 1 << 0, // 0001
WRITE = 1 << 1, // 0010
EXECUTE = 1 << 2 // 0100
};
int main() {
int userPermissions = READ | WRITE; // Set read and write permissions
if (userPermissions & WRITE) {
std::cout << "User has write permissions.\n";
}
return 0;
}
Example 2: Color Manipulation in Graphics
In graphics programming, the bitwise OR operator is often used to combine color values represented as integers.
#include <iostream>
const int RED = 0xFF0000; // Red color
const int GREEN = 0x00FF00; // Green color
const int BLUE = 0x0000FF; // Blue color
int combineColors(int color1, int color2) {
return color1 | color2; // Blend colors using bitwise OR
}
int main() {
int blendedColor = combineColors(RED, GREEN); // Creates Yellow
std::cout << "Blended Color: " << std::hex << blendedColor << "\n";
return 0;
}
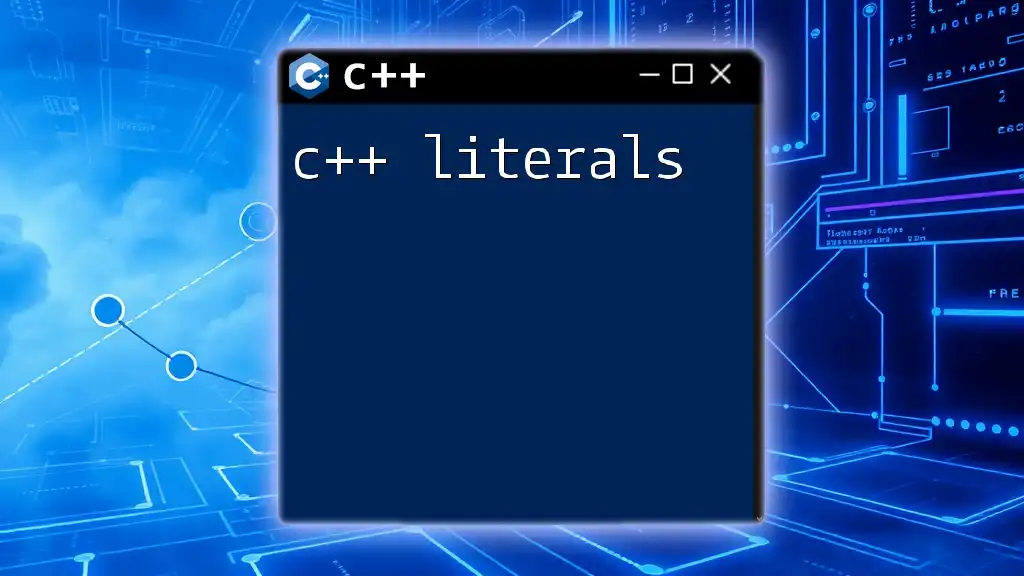
Conclusion
Mastering the C++ Bitwise OR operator allows developers to perform efficient low-level data manipulation, optimize performance, and handle multiple states cleanly. By understanding its properties and applications, programmers can harness the power of bitwise operations to enhance their code's readability and performance.
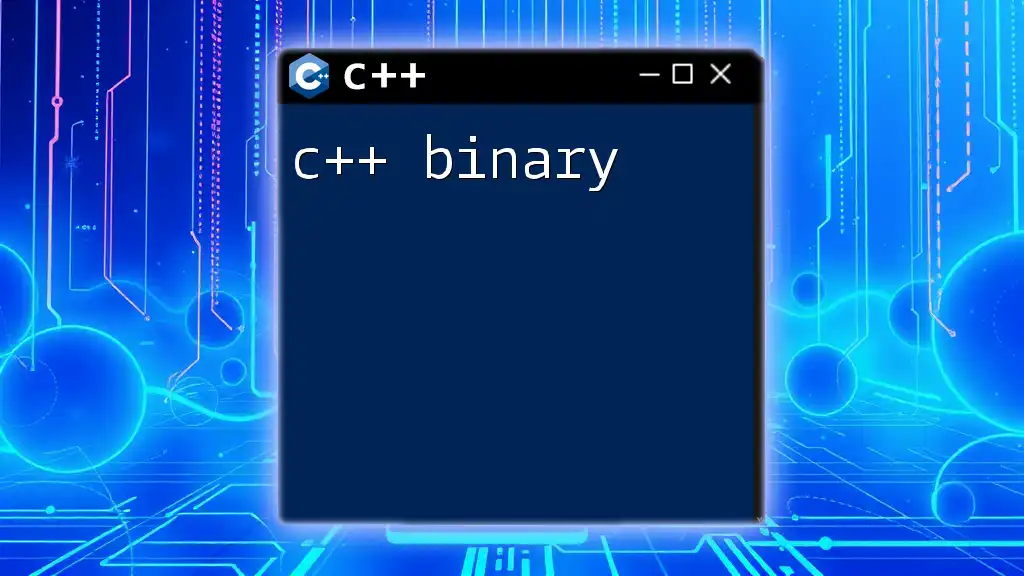
Additional Resources
Look for tutorials that focus on bitwise operations in C++, or delve into books that address lower-level programming concepts for further learning on this topic.