In C++, a "bit" is the smallest unit of data, representing a binary value of either 0 or 1, and can be manipulated using bitwise operators to perform efficient low-level operations on integer data.
Here’s an example of using the bitwise AND operator in C++:
#include <iostream>
int main() {
int a = 12; // 1100 in binary
int b = 5; // 0101 in binary
int result = a & b; // Bitwise AND operation
std::cout << "The result of " << a << " & " << b << " is: " << result; // Outputs 4 (0100 in binary)
return 0;
}
Understanding Bits in C++
What is a Bit?
A bit, short for binary digit, is the most basic unit of data in computing and digital communications. It can hold a value of either 0 or 1, which corresponds to the state of a binary condition (like off/on or false/true). Bits are fundamental because they form the basis of all modern computing, as all data and instructions in a computer are ultimately represented in binary format.
Binary Number System
The binary number system uses only two symbols: 0 and 1. In contrast to the decimal system, which has ten symbols (0-9), the binary system is crucial for digital devices to process information efficiently. A set of bits can represent any number or character. For example, the binary string `0101` represents the number five in decimal.
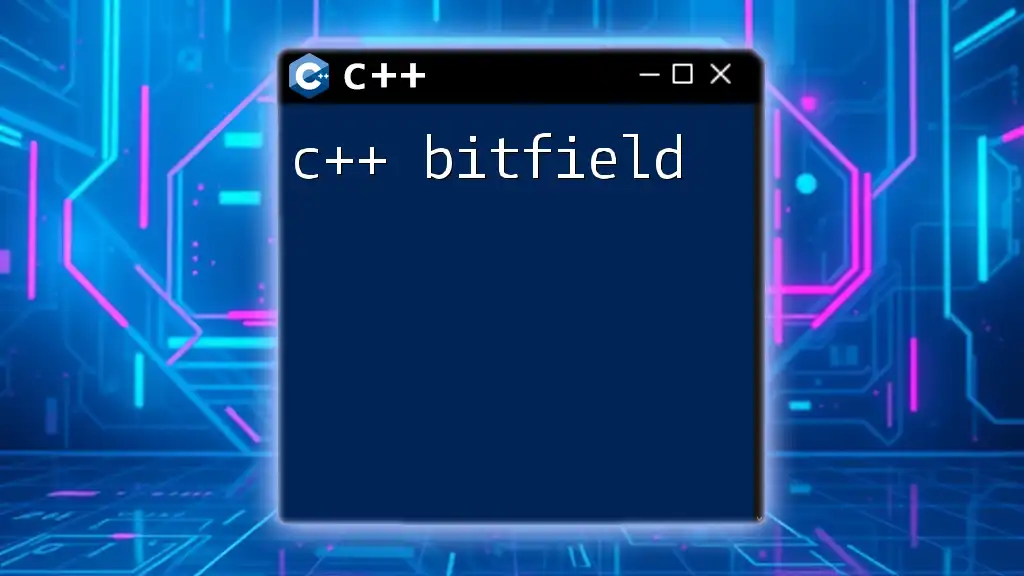
C++ Bitwise Operators
Overview of Bitwise Operators
Bitwise operators are special operators that perform operations at the bit level. They allow programmers to redefine how data is manipulated in ways that can be faster and more efficient than traditional arithmetic operators. In C++, the primary bitwise operators include:
- AND (`&`)
- OR (`|`)
- XOR (`^`)
- NOT (`~`)
- Left Shift (`<<`)
- Right Shift (`>>`)
Bitwise AND Operator
The AND operator (`&`) compares each bit of two numbers, returning a new number where the bits are set to 1 only if both corresponding bits are 1.
Use Case: It can be used to check for specific flags within a binary representation.
Example:
#include <iostream>
int main() {
int a = 5; // 0101 in binary
int b = 3; // 0011 in binary
int result = a & b; // result will be 1 (0001 in binary)
std::cout << "Bitwise AND: " << result << std::endl;
return 0;
}
Output: `Bitwise AND: 1`
Bitwise OR Operator
The OR operator (`|`) compares two bits and returns a new number where the bits are set to 1 if at least one of the corresponding bits is 1.
Use Case: This operator can be used to set specific bits to 1.
Example:
#include <iostream>
int main() {
int a = 5; // 0101 in binary
int b = 3; // 0011 in binary
int result = a | b; // result will be 7 (0111 in binary)
std::cout << "Bitwise OR: " << result << std::endl;
return 0;
}
Output: `Bitwise OR: 7`
Bitwise XOR Operator
The XOR operator (`^`) outputs 1 where the bit values differ (one is 0 and the other is 1) and 0 where they are the same.
Use Case: This operator can be useful for toggling bits and finding differences between two binary numbers.
Example:
#include <iostream>
int main() {
int a = 5; // 0101 in binary
int b = 3; // 0011 in binary
int result = a ^ b; // result will be 6 (0110 in binary)
std::cout << "Bitwise XOR: " << result << std::endl;
return 0;
}
Output: `Bitwise XOR: 6`
Bitwise NOT Operator
The NOT operator (`~`) inverts all bits of the operand, transforming 1s to 0s and 0s to 1s.
Use Case: This operator can be used in situations where you need the complement of a binary number.
Example:
#include <iostream>
int main() {
int a = 5; // 0101 in binary
int result = ~a; // result will be -6 (invert all bits)
std::cout << "Bitwise NOT: " << result << std::endl;
return 0;
}
Output: `Bitwise NOT: -6`
Left Shift Operator
The left shift operator (`<<`) shifts all bits in a number to the left by the specified number of positions, filling in from the right with 0s. Each shift to the left effectively multiplies the number by 2.
Use Case: Efficiently performs multiplication by powers of two.
Example:
#include <iostream>
int main() {
int a = 5; // 0101 in binary
int result = a << 1; // result will be 10 (1010 in binary)
std::cout << "Left Shift: " << result << std::endl;
return 0;
}
Output: `Left Shift: 10`
Right Shift Operator
The right shift operator (`>>`) shifts all bits in a number to the right by a specified number of positions. Each right shift effectively divides the number by 2.
Use Case: Useful for quickly implementing division operations by powers of two.
Example:
#include <iostream>
int main() {
int a = 5; // 0101 in binary
int result = a >> 1; // result will be 2 (0010 in binary)
std::cout << "Right Shift: " << result << std::endl;
return 0;
}
Output: `Right Shift: 2`
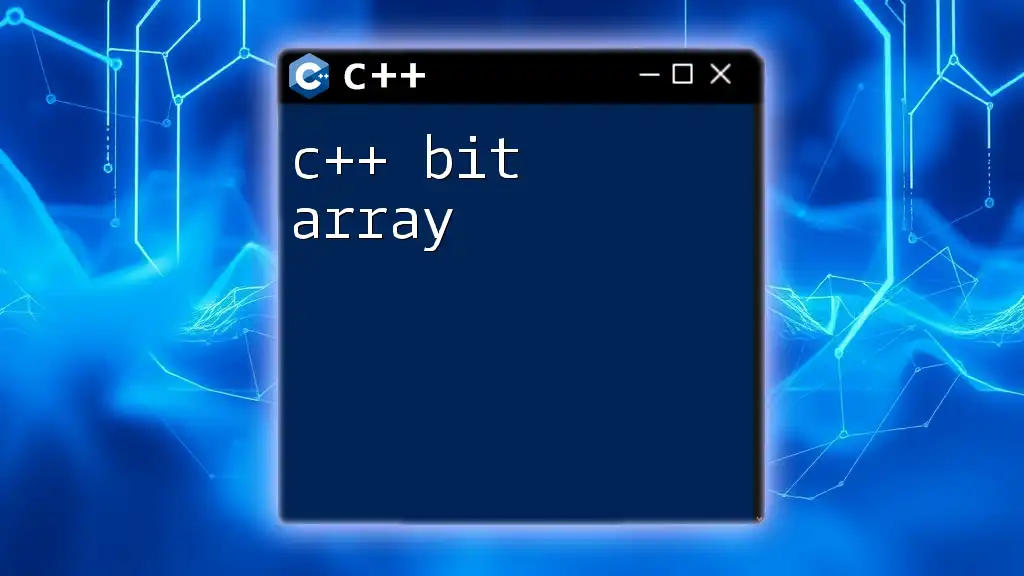
Applications of Bit Manipulation in C++
Data Compression
Bit manipulation plays a vital role in data compression by allowing efficient packing of data. By manipulating bits directly, unnecessary overhead can be eliminated, leading to a reduced data size which saves storage space and bandwidth.
Low-Level Programming
In low-level programming, such as in embedded systems, there is a need to communicate directly with hardware. Bit manipulation allows programmers to access specific bits in memory addresses, control hardware settings, and configure device registers precisely.
Performance Optimization
When performance is a concern, using bitwise operations can enhance execution time significantly. Unlike arithmetic operations that require multiple CPU cycles, bitwise operations are generally executed in a single cycle, making them a preferred choice in performance-critical applications.
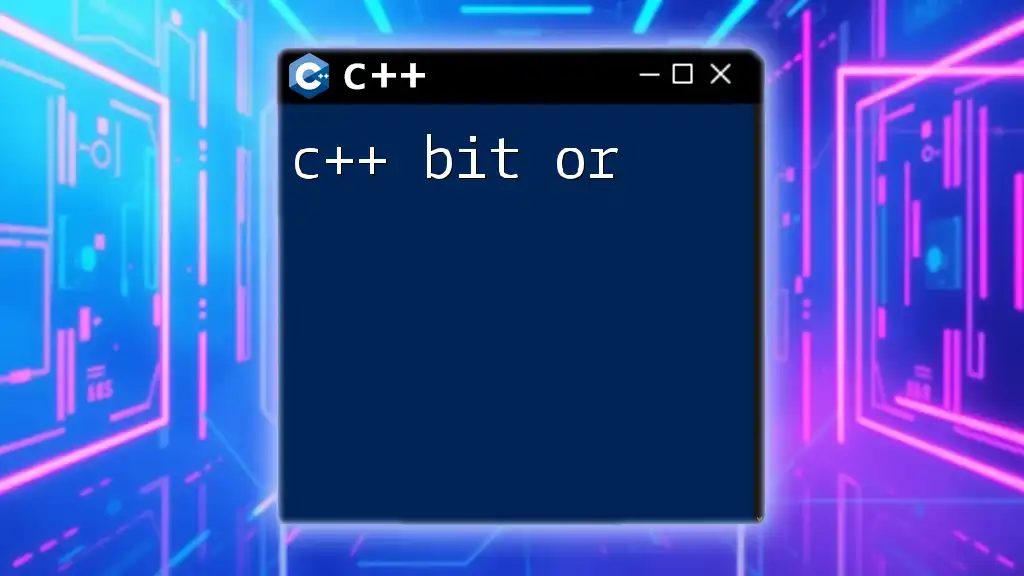
Practical Examples of Bit Manipulation
Swapping Two Numbers Using XOR
You can swap two integers without using a temporary variable by using the XOR operation cleverly.
Example:
#include <iostream>
int main() {
int a = 5, b = 10;
a = a ^ b;
b = a ^ b;
a = a ^ b;
std::cout << "Swapped: a = " << a << ", b = " << b << std::endl;
return 0;
}
Output: `Swapped: a = 10, b = 5`
Counting Set Bits (Hamming Weight)
Counting the number of 1s (set bits) in a binary representation is another common operation. The most efficient way to do this involves using a loop and the AND operator.
Example:
#include <iostream>
int countSetBits(int n) {
int count = 0;
while (n) {
count++;
n &= (n - 1); // Remove the rightmost set bit
}
return count;
}
int main() {
int num = 29; // 11101 in binary
std::cout << "Set bits count: " << countSetBits(num) << std::endl;
return 0;
}
Output: `Set bits count: 4`
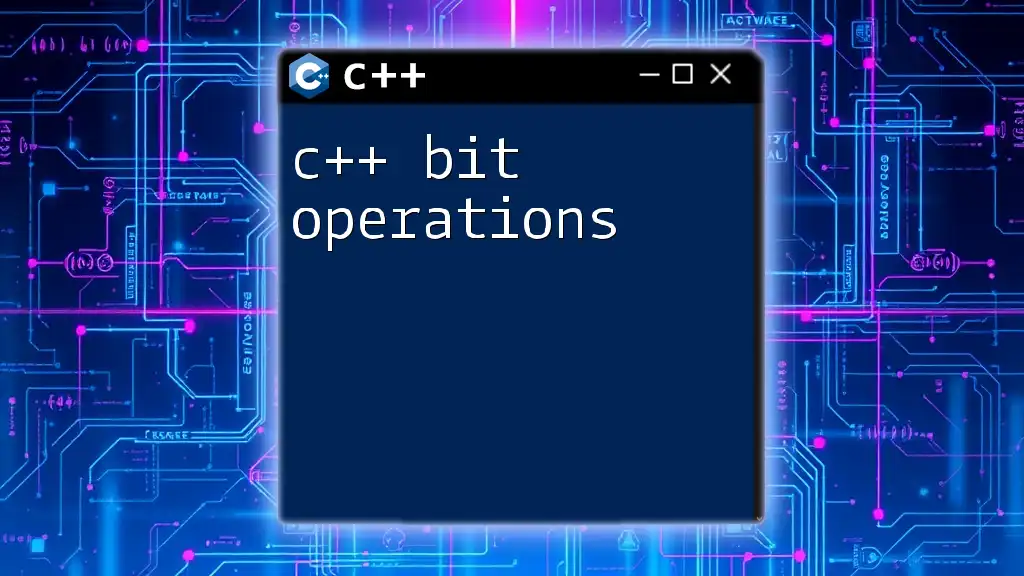
Best Practices for C++ Bit Manipulation
Readability vs. Performance
While bit manipulation can improve performance, it’s crucial to maintain code readability. Ensure your code is well-commented and its intention is clear, especially for complex operations.
Common Pitfalls
Be cautious when performing bit manipulations, as it’s easy to get unexpected results. For instance, shifting an integer too far can lead to loss of data, and improper use of the NOT operator can invert more than anticipated.
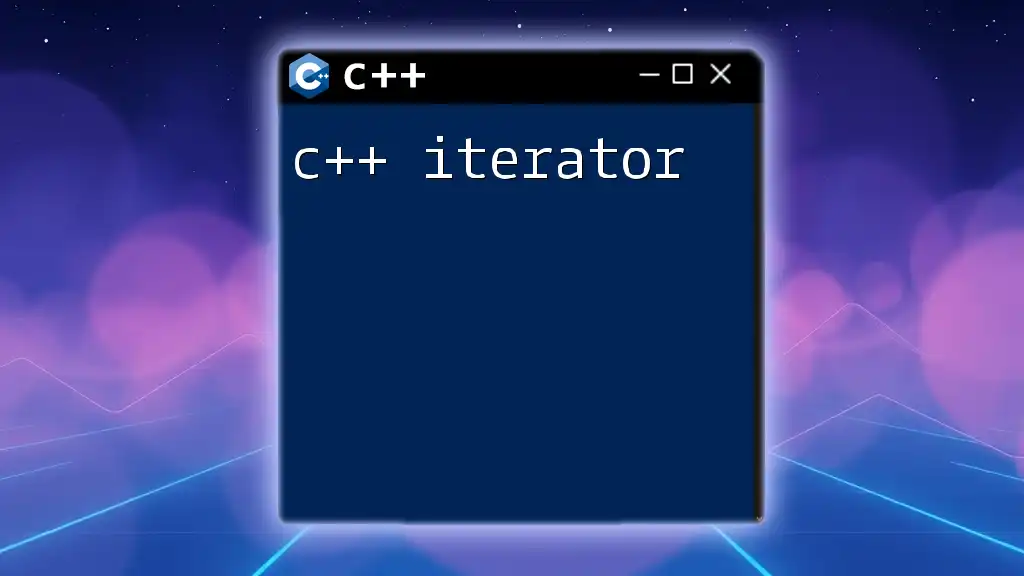
Conclusion
In this guide, we've explored the fundamental aspects of C++ bit manipulation, covering bitwise operators, applications, practical examples, and best practices. Armed with this knowledge, you can leverage C++ bit operations to optimize your code and solve complex problems efficiently. To deepen your understanding of this powerful toolset, consider advancing your studies in C++.