C++ JIT (Just-In-Time) compilation optimizes the execution of C++ code at runtime, allowing for performance improvements by compiling code on the fly.
Here’s an example of how you might use JIT compilation in C++ with the LLVM library:
#include <llvm/ExecutionEngine/ExecutionEngine.h>
#include <llvm/ExecutionEngine/MCJIT.h>
#include <llvm/IR/LLVMContext.h>
#include <llvm/IR/Module.h>
#include <llvm/IR/Function.h>
#include <llvm/IR/IRBuilder.h>
#include <llvm/Support/TargetSelect.h>
using namespace llvm;
int main() {
InitializeNativeTarget();
LLVMContext context;
Module *module = new Module("JITExample", context);
IRBuilder<> builder(context);
// Create a function prototype
FunctionType *funcType = FunctionType::get(builder.getInt32Ty(), false);
Function *function = Function::Create(funcType, Function::ExternalLinkage, "myFunction", module);
// Create a basic block and a return statement
BasicBlock *block = BasicBlock::Create(context, "entry", function);
builder.SetInsertPoint(block);
builder.CreateRet(builder.getInt32(42)); // Return 42
// JIT compile the module
ExecutionEngine *engine = EngineBuilder(std::unique_ptr<Module>(module)).create();
int (*func)() = (int (*)())engine->getFunctionAddress("myFunction");
// Call the JIT-compiled function
int result = func();
return result; // Should return 42
}
Understanding JIT Compilation in C++
Introduction to JIT Compilation
Just-In-Time (JIT) compilation is a vital technique in modern programming languages, merging interpretation and compilation to optimize execution. Rather than translating the entire program to native code before execution, JIT compilers convert parts of the program during runtime, allowing for faster execution through optimizations tailored to actual usage patterns. This approach contributes significantly to performance enhancements in dynamic programming environments.
How JIT Works
The JIT compilation process involves several steps. First, the Source Code is translated into an Intermediate Representation (IR) by a traditional compiler. At runtime, a JIT Compiler translates this IR into Native Code. This means that some parts of the program can be executed as they are needed while additional parts are compiled on the fly.
To understand this better, here’s a simplified breakdown:
- Interpreter: Initially interprets the bytecode, allowing for immediate execution.
- Compiler: At runtime, it identifies frequently executed paths and compiles them into optimized native code to improve performance.
This dual approach leverages the strengths of both interpretation and compilation.
The Role of JIT in C++
Benefits of JIT Compilation in C++
Using JIT compilation in C++ offers numerous advantages:
-
Performance: JIT compilers can improve the performance of applications by optimizing the frequently executed code paths at runtime, allowing them to adapt based on actual workload.
-
Flexibility: Dynamic optimization enables the JIT compiler to adjust the code based on how the application is being used, achieving better performance without requiring intervention from the programmer.
-
Portability: JIT makes it possible to run a C++ application on multiple architectures, as the native code can be generated specific to the environment in which it runs, maintaining consistency while improving performance.
Popular JIT Compilers for C++
Several notable JIT compilers optimize C++ code:
-
LLVM: The LLVM framework not only provides a JIT compiler but also emphasizes modularity, enabling a variety of optimizations suitable for C++ applications. Its capabilities can significantly enhance application performance.
-
Microsoft's CLR: The Common Language Runtime (CLR) includes a JIT compiler that benefits C++/CLI applications, providing seamless integration with .NET features while enhancing performance.
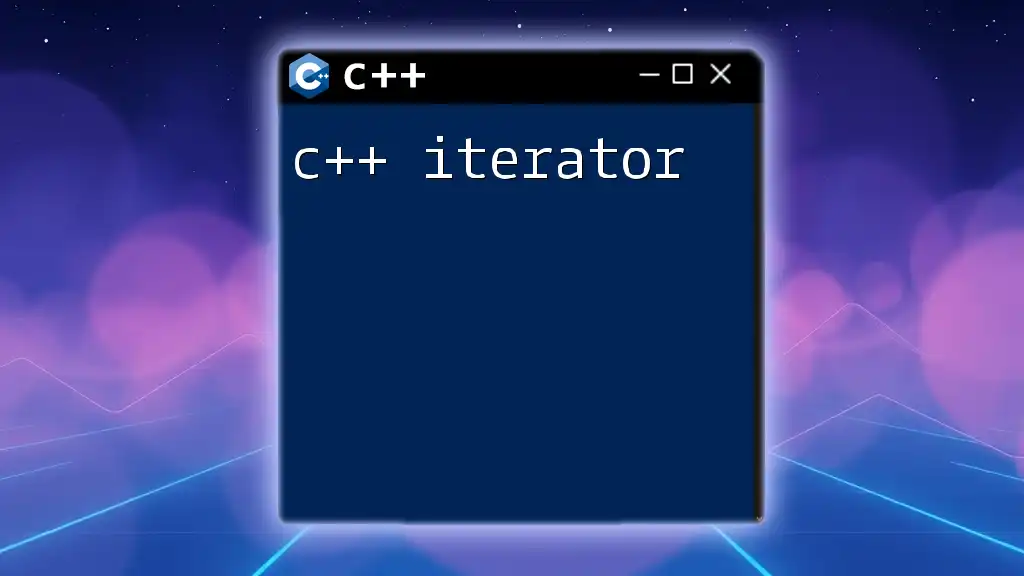
Integrating JIT Compilation into C++
Setting Up a JIT Compiler with LLVM
To leverage JIT compilation in C++, setting up a JIT compiler with LLVM is a practical approach. Here are the steps involved:
Prerequisites: Before you begin, make sure you have LLVM installed. You can refer to the official LLVM documentation for installation instructions suitable for your operating system.
Example: Hello World with LLVM JIT
Below is a simple example that demonstrates how to use LLVM's JIT capabilities to compile and run a basic "Hello World" program.
#include <llvm/ExecutionEngine/ExecutionEngine.h>
#include <llvm/ExecutionEngine/MCJIT.h>
#include <llvm/ExecutionEngine/GenericValue.h>
#include <llvm/IR/Module.h>
#include <llvm/IR/Function.h>
#include <llvm/IR/IRBuilder.h>
#include <llvm/Support/TargetSelect.h>
#include <llvm/ExecutionEngine/MCJIT.h>
// Function to generate and execute code
void createAndRunFunction() {
llvm::LLVMContext context;
llvm::Module *module = new llvm::Module("MyModule", context);
llvm::IRBuilder<> builder(context);
// Create a function prototype: int main()
llvm::FunctionType *funcType = llvm::FunctionType::get(builder.getInt32Ty(), false);
llvm::Function *mainFunc = llvm::Function::Create(funcType, llvm::Function::ExternalLinkage, "main", module);
// Create a basic block and set the insertion point
llvm::BasicBlock *block = llvm::BasicBlock::Create(context, "entry", mainFunc);
builder.SetInsertPoint(block);
// Create the return instruction
builder.CreateRet(llvm::ConstantInt::get(builder.getInt32Ty(), 0));
// Initialize the JIT
llvm::InitializeNativeTarget();
llvm::InitializeNativeTargetAsmPrinter();
llvm::ExecutionEngine *engine = llvm::EngineBuilder(std::unique_ptr<llvm::Module>(module))
.setOptLevel(llvm::CodeGenOpt::Default)
.create();
// Execute the function
int returnValue = engine->runFunction(mainFunc, llvm::ArrayRef<llvm::GenericValue>()).IntVal.getZExtValue();
printf("Returned from main: %d\n", returnValue);
}
// Entry point
int main() {
createAndRunFunction();
return 0;
}
In this example, we build a simple LLVM module that contains a `main` function which returns an integer. After defining the function, we use LLVM's JIT capabilities to compile and run it.
Using JIT for Performance Optimization
To fully benefit from JIT compilation, it's essential to analyze the hot paths in your C++ code. Hot paths are segments of code that are run frequently and are thus prime candidates for optimization.
Example: Profiling tools like `gprof` can help identify these paths. By focusing your JIT optimizations on these critical sections, you improve overall application performance. Techniques may include optimizing data structures, minimizing unnecessary calculations, or caching results for frequently called functions.
Use Cases of JIT in C++
JIT compilation can address various applications in C++, showcasing its versatility:
-
Dynamic Object Creation: JIT enables dynamic assembly of classes and methods at runtime, especially useful for game engines or graphic applications where flexibility is key.
-
Real-Time Data Processing: Industries like financial trading, where seconds matter, benefit from JIT for rapid processing of immense amounts of data.
-
Machine Learning Frameworks: Libraries like TensorFlow or PyTorch employ JIT to enhance performance during model training and inference, as they can compile models at runtime to optimize execution pathways.
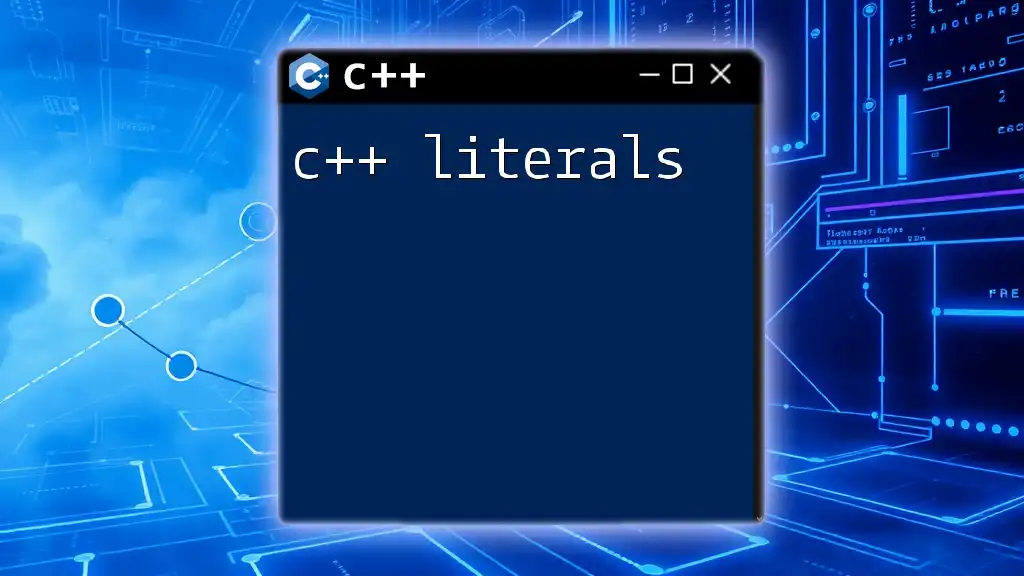
Challenges and Limitations of JIT Compilation
Common Issues Encountered
While JIT offers significant benefits, it is not without challenges.
-
Warm-Up Time: JIT-compiled code often comes with initial latency. The system needs to collect usage data to optimize, which can slow down the application at the beginning.
-
Memory Consumption: The JIT process can lead to increased memory usage compared to ahead-of-time (AOT) compilation since both the original bytecode and the generated native code might reside in memory concurrently.
-
Debugging Complexity: Debugging JIT-generated code can be complicated. The dynamic nature of the code can obscure the runtime state, making it harder to identify issues.
When to Use JIT and When to Avoid It
Selecting between JIT and traditional compilation isn't straightforward. Consider using JIT when your application requires dynamic features and runtime optimizations. Conversely, you might opt for AOT compilation in scenarios where predictable performance and fewer runtime resources are critical, like embedded systems or use cases with stringent memory constraints.
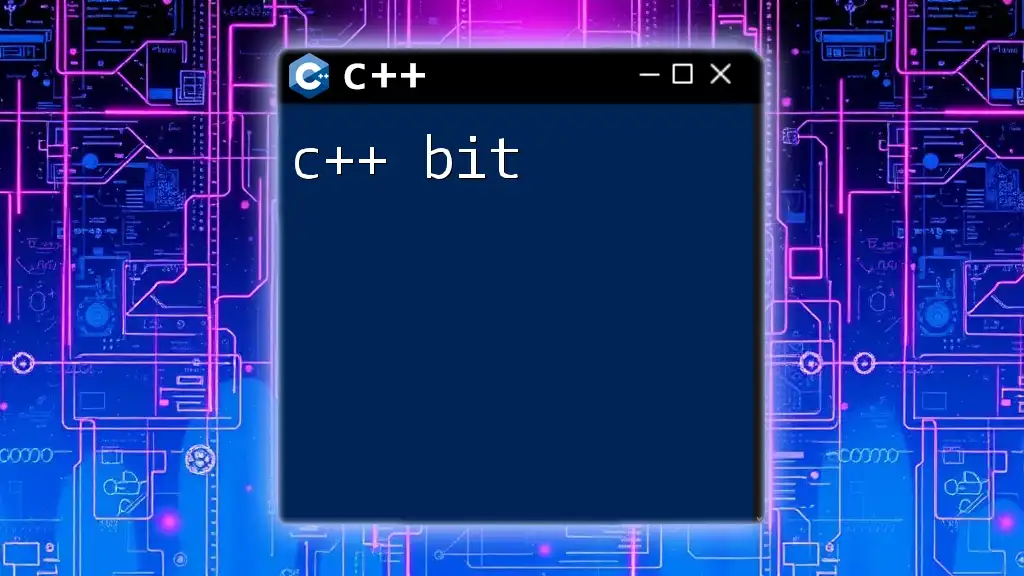
Conclusion
Recap of Key Points
JIT compilation is a powerful technique that significantly enhances C++ application performance by optimizing frequently executed code during runtime. This approach offers flexibility and portability while revealing potential efficiencies in various application contexts.
Future Trends in JIT Compilation
Looking ahead, advancements in JIT technologies are poised to improve their capabilities further. As languages evolve and programming paradigms shift, both the efficiency of JIT compilers and their integration into the development stack of C++ programs will likely expand.
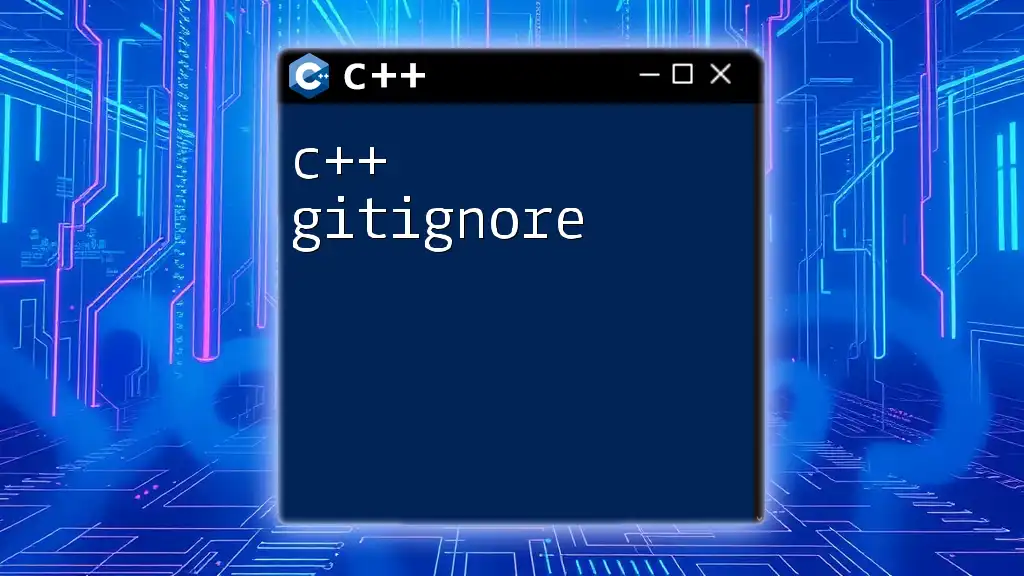
Further Reading and Resources
For those who wish to deeper their understanding of C++ and JIT compilation, consider exploring specialized books, academic articles, and online resources. Community forums and discussion groups can also provide valuable insights and updates regarding ongoing developments in JIT technologies.