C++ ABI (Application Binary Interface) defines how different components of compiled C++ programs interact at the binary level, ensuring compatibility across different compilers and environments.
Here's a simple code snippet demonstrating a C++ function that could be affected by ABI considerations:
#include <iostream>
// A simple function to demonstrate ABI
extern "C" void sayHello() {
std::cout << "Hello, World!" << std::endl;
}
In this example, the `extern "C"` linkage specifies that the function `sayHello` should use C-style names to facilitate compatibility with C and other languages.
Understanding C++ ABI
What is C++ ABI?
C++ ABI, or Application Binary Interface, refers to the low-level interface that defines how various components of binary code interact. ABI encompasses details such as data types, object representations, and the calling conventions that dictate how functions receive parameters and return values. Understanding C++ ABI is crucial for any developer working with compiled languages because it ensures that different code objects can effectively communicate at runtime.
Differences Between C++ ABI and C ABI
While both C and C++ share similar syntax and concepts, they differ significantly at the ABI level. The C ABI is primarily focused on procedural programming and does not account for features unique to C++, such as class hierarchies or function overloading.
Key Differences
One major difference lies in name mangling: to support function overloading, C++ compilers encode additional information in function names, making them unique based on their parameter types. In contrast, the C ABI does not require this feature, leading to possible name collisions when using C++ libraries in C environments.

The Role of C++ ABI in Development
How ABI Affects Compatibility
ABI plays a crucial role in binary compatibility. This refers to the ability of different binary modules to work together seamlessly. For example, if a software library is compiled with a different compiler version or settings than the application that uses it, ABI mismatches can lead to unexpected behavior or crashes. Understanding these compatibility issues is vital for developers who intend to create or use libraries across different environments.
The Impact of C++ ABI on Libraries
When creating shared libraries, maintaining ABI stability is essential. An ABI-breaking change occurs when modifications to the code cause binary incompatibility. Examples of such changes include altering the size of a class or changing the order of member variables. When ABI changes occur, it can necessitate recompilation of any dependent modules, complicating the development process.

C++ ABI and Name Mangling
Understanding Name Mangling
Name mangling is a technique used by C++ compilers to encode additional information into function names, primarily to support function overloading and namespaces. This process transforms a standard function name into a more complex string that includes details about the function, such as the types of its parameters and its namespace.
Example of Name Mangling
Consider the following example:
class Example {
public:
void method();
};
In a compiled binary, the method `method` may be transformed into something like `Example::method()`, including information about the class it belongs to and its parameters. This allows the compiler to recognize that (and differentiate between) overloaded functions.

Versioning and C++ ABI
What is ABI Versioning?
ABI versioning refers to the practice of assigning version numbers to ABI definitions to ensure compatibility across different builds. It is important for libraries that are frequently updated, as changing the ABI can break existing binaries that depend on them.
Strategies for Managing ABI Changes
To mitigate issues with ABI breakage, developers can adopt several strategies. These may include:
- Graceful deprecation of old interfaces while introducing new ones, allowing users time to transition.
- Utilizing versioned symbols in shared libraries, enabling older binaries to link against the previous version of a function or method.
- Focusing on "ABI-stable" designs from the outset, which could involve designing public interfaces that remain unchanged even when internal implementations evolve.

Examining C++ ABI on Different Platforms
ABI Differences Across Compilers
Different compilers can implement their own ABIs, leading to significant differences in how programs interact at runtime. Major compilers, such as GCC, Clang, and MSVC, have distinct rules governing ABI behavior. For example, GCC has specific conventions for name mangling and data alignment, while MSVC has its own set of conventions.
Platform-Specific ABI Details
Linux
In Linux environments, the Itanium C++ ABI is commonly used, generally adhering to the rules laid out by the C++ standard. This ABI promotes compatibility across different compilers targeting Linux.
Windows
On Windows, the Microsoft Visual C++ ABI (MSVC) is the standard. It has distinct features, such as a different approach to calling conventions and name mangling, that can lead to compatibility issues when mixing different compiler binaries.
MacOS
MacOS uses a combination of the Itanium C++ ABI and platform-specific rules enforced by Apple's toolchain. Developers should be aware of these nuances when creating and distributing libraries for macOS applications.
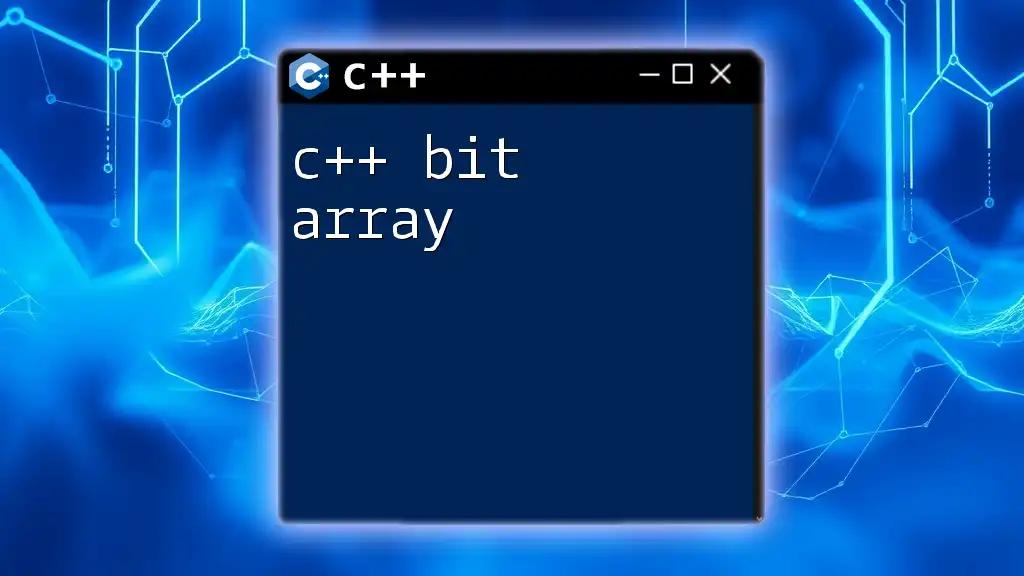
Tools for Analyzing and Modifying C++ ABI
ABI Compatibility Checkers
Tools like abi-dumper or abi-compliance-checker provide developers with the means to analyze their binaries for ABI compatibility. These tools can help you determine whether changes made in a library will affect the applications that depend on it.
Code Snippet for Using ABI Checkers
To use `abi-dumper`, the command may look like:
abi-dumper mylibrary.so
This command generates an ABI report that you can analyze to identify any compatibility issues that could arise from changes made in the library.
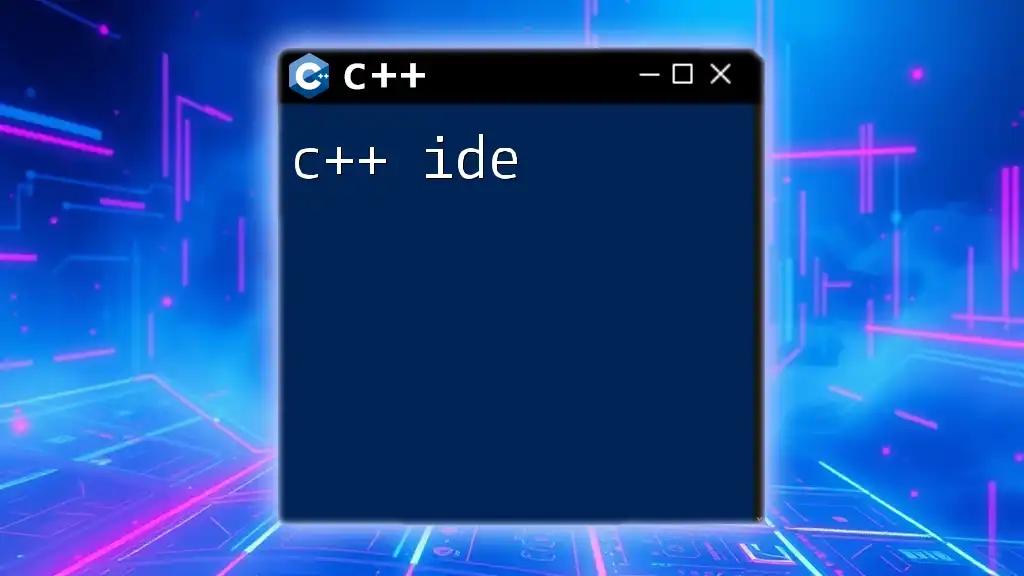
C++ ABI Best Practices
Implementing ABI Stability
To write ABI-stable C++ code, you should adhere to a few best practices. Avoid changing the public interfaces of classes unless absolutely necessary, and when changes are required, provide a clear migration path. Consider the following:
- Limit exposure of internal details: Keep internal data members private and expose only necessary methods.
- Use design patterns: Patterns like the Bridge Pattern can help you maintain separability between implementation and interface, minimizing ABI impact.
Documentation and ABI
Documenting the ABI for libraries is critical for third-party developers who will rely on your code in their applications. Clear ABI documentation will help them understand the interfaces they can depend on across different versions. Always include versioning information and describe any API changes clearly.
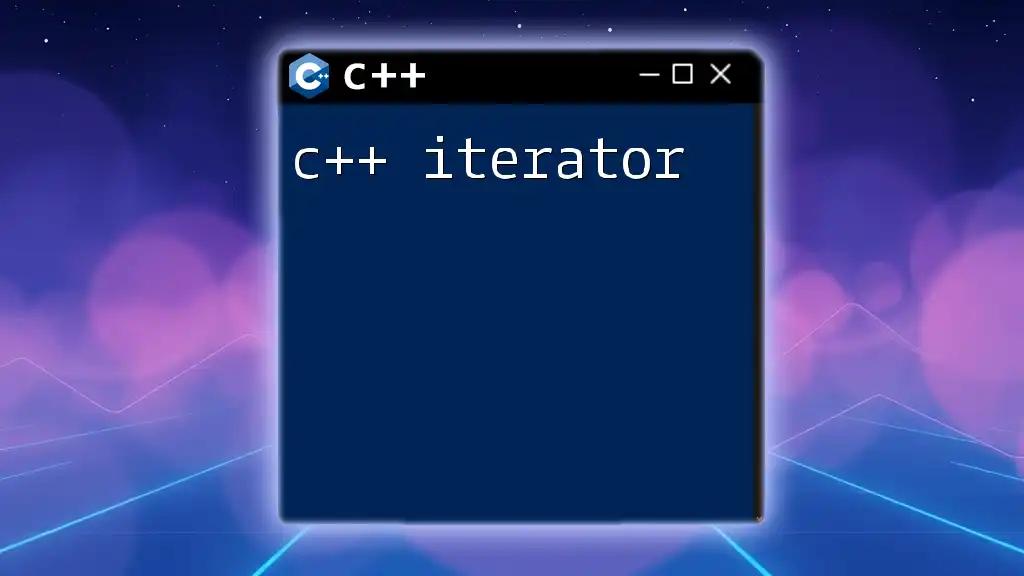
Conclusion
In summary, understanding C++ ABI is essential for any developer working with compiled languages. By recognizing its importance in compatibility, name mangling, versioning, and best practices for maintaining ABI stability, developers can write better, more reliable code. Embracing the principles of ABI will ensure that your applications and libraries work seamlessly together, reducing the time spent debugging ABI-related issues. For further reading, numerous resources are available that delve deeper into C++ ABI and its practical applications in software development.