C++ binary refers to the compiled executable files generated from C++ source code, which is platform-specific and optimized for performance.
Here’s an example demonstrating how to create a binary file using the `ofstream` class in C++:
#include <iostream>
#include <fstream>
int main() {
std::ofstream outfile("example.bin", std::ios::binary);
int number = 42;
outfile.write(reinterpret_cast<char*>(&number), sizeof(number));
outfile.close();
return 0;
}
What is a C++ Binary?
A C++ binary is a compiled version of a C++ program that your computer can execute. When you write code in C++, it exists as human-readable text known as source code. However, computers cannot run this text directly; they require the code to be translated into a binary format. This binary format is composed of machine code that the computer understands, allowing your program to perform the desired operations.
The importance of a binary lies in its execution efficiency; once converted, it can be run on the machine without needing to recompile, thus saving time and resources during execution.

How C++ Compiles to Binary
The Compilation Process
Understanding how C++ code compiles into a binary format is crucial for any C++ programmer. The compilation process typically includes several stages:
-
Preprocessing: This stage involves handling directives within your source code, like `#include` for libraries or `#define` for macros. The preprocessor prepares the code for compilation by expanding these directives into full code.
-
Compilation: In this stage, the compiler translates the preprocessed source code into assembly code, a low-level language that's still somewhat human-readable.
-
Assembly: The assembler takes the assembly code and converts it into machine code, a binary representation that a computer can understand.
-
Linking: Finally, the linker combines various pieces of code and libraries into a single executable file. It resolves references between modules and prepares the final output.
Example Code Compilation
Consider the following simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
- Preprocessing: The preprocessor resolves `#include <iostream>`, embedding the contents of the iostream library into your source code.
- Compilation: The compiler converts the resulting code into assembly language.
- Assembly: The assembler translates assembly into machine code.
- Linking: Finally, the linker creates the executable binary.
By the end of this process, this straightforward program will transform into a binary that can be executed directly by your machine.
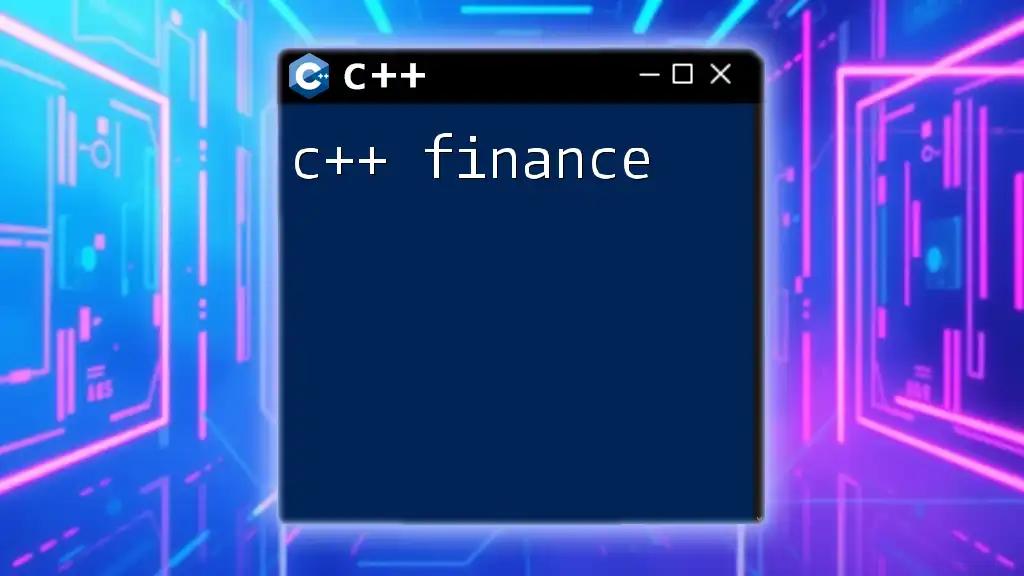
Understanding the Executable Binary
What is an Executable?
An executable is a type of binary file that contains a program that can be run on a computer. In the context of C++, common file extensions for executables include `.exe` (on Windows) and `.out` or no extension (on Unix-like systems).
Structure of a C++ Binary
A typical C++ binary structure consists of several key sections:
- Header: Contains metadata about the binary, such as machine type and entry point.
- Code Section: Holds the actual machine code generated from your C++ source code.
- Data Section: Stores global and static variables, along with constants.
Understanding the structure allows programmers to optimize and troubleshoot binaries more effectively.

Tools for Working with C++ Binaries
Compilers
Popular compilers used for C++ development include:
- GCC (GNU Compiler Collection): A widely used open-source compiler known for its robustness and optimization capabilities.
- Clang: Known for its performance and user-friendly error messages, Clang is often preferred in projects that emphasize modern C++ features.
- MSVC (Microsoft Visual C++): The go-to compiler for Windows development, providing seamless integration with Visual Studio.
Each compiler has its unique features and capabilities, and understanding these can aid in selecting the right tool for your specific situations.
Debuggers
Debugging binaries is essential for any developer. Tools like GDB (GNU Debugger) and Visual Studio Debugger allow programmers to inspect and manipulate their running binaries. This capability is vital for identifying bugs and understanding how binaries behave in execution.

Creating and Executing a C++ Binary
Compiling a C++ Program
To create a C++ binary from your source code, you'll typically use the command line. Here’s an example command using GCC:
g++ -o hello hello.cpp
In this command:
- `g++` is the compiler being used.
- `-o hello` specifies the name of the output binary.
- `hello.cpp` is the source file being compiled.
Running the Executable
Once compiled, running your binary is straightforward. On Unix-like systems, you’d execute:
./hello
Upon execution, you should see the output:
Hello, World!
This simple example illustrates how an executable binary functions and reinforces the concept of translation from source code to binary.
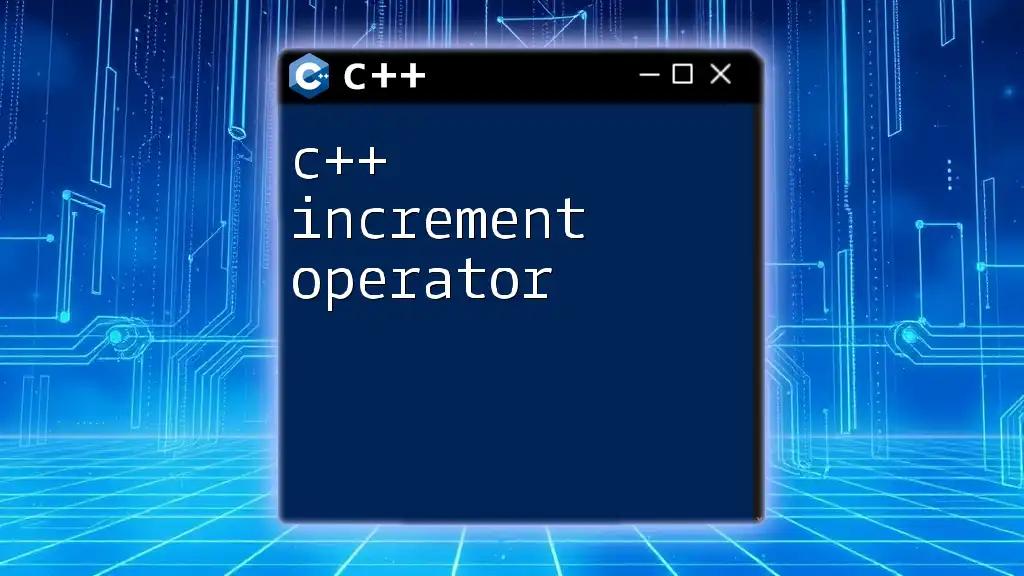
Common Issues with C++ Binaries
Errors during Compilation
While compiling a C++ program, you may encounter various errors, such as:
- Syntax Errors: These arise from incorrect code structure. Pay close attention to error messages to understand what needs fixing.
- Linker Errors: These occur when the compiler can't find referenced code. Ensure all libraries are correctly linked.
Binary Compatibility Issues
Compatibility issues can sometimes arise, especially when running binaries across different platforms (e.g., Windows vs. Unix-like systems).
There are also distinctions between static and dynamic linking, where static libraries are included in the binary at compile time, while dynamic libraries are linked at runtime.
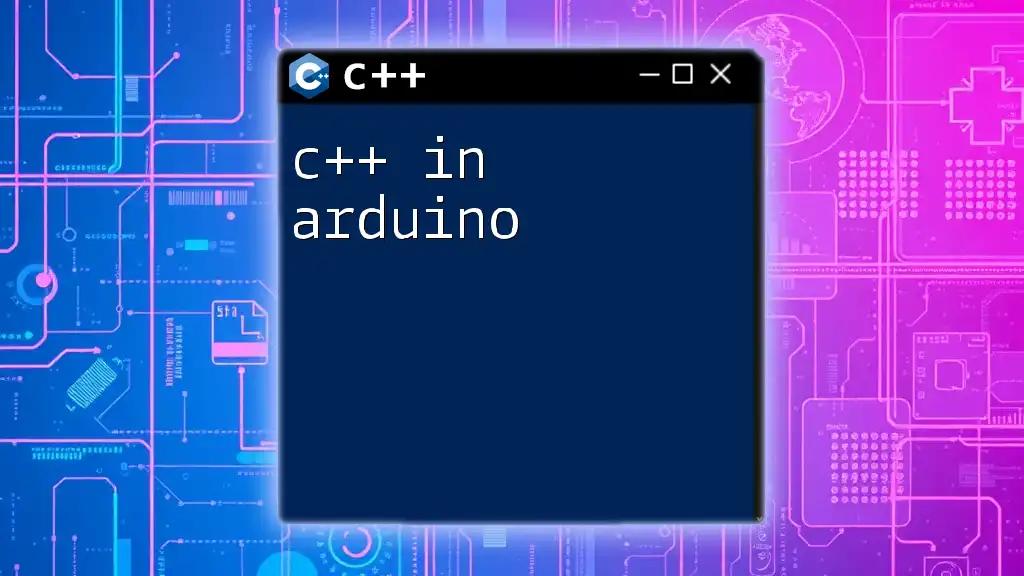
Advanced Concepts
Binary Optimization Techniques
Compiler optimizations significantly affect the performance of your binaries. Using flags like `-O1`, `-O2`, or `-O3` when compiling can guide the compiler into improving performance and reducing executable size.
For instance:
g++ -O2 -o hello hello.cpp
This command compiles your program with optimization level 2, aiming for a balance between compilation time and performance.
Static vs Dynamic Libraries
In C++, you can work with both static and dynamic libraries.
-
Static Libraries: These are bundled into the executable at compile time. They increase binary size but simplify distribution since the binary doesn't rely on external files.
-
Dynamic Libraries: These are loaded at runtime, reducing binary size but requiring that the necessary libraries be present on any machine running the binary.
An example command to compile with a static library might look like this:
g++ -o app main.cpp -static -lmyStaticLib
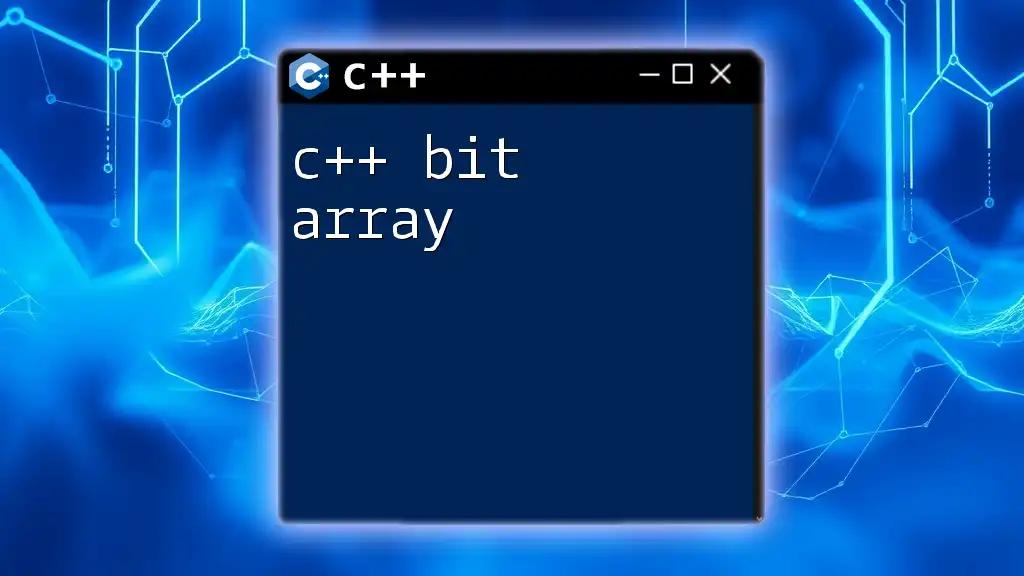
Conclusion
Understanding C++ binaries is essential for anyone looking to master C++ programming. From the compilation process to executing an executable, grasping these concepts enables efficient coding and troubleshooting. With this knowledge, you are well-equipped to dive deeper into programming and create performance-optimized binaries tailored to your needs.