A C++ final exam typically assesses a student's understanding of key concepts and syntax in the language, evaluating their ability to write efficient and correct code.
Here's a simple code snippet that demonstrates the use of a class with a final method:
#include <iostream>
class Base {
public:
virtual void show() final { // 'final' prevents overriding this method
std::cout << "Base class show method." << std::endl;
}
};
class Derived : public Base {
public:
// void show() override {} // This would cause a compilation error
};
int main() {
Base b;
b.show(); // Outputs: Base class show method.
return 0;
}
Understanding C++ Fundamentals
Overview of C++
C++ is a powerful programming language that has evolved significantly since its inception in the early 1980s. It combines high-level and low-level programming features, making it versatile for both application development and systems programming. Understanding its history and evolution helps learners appreciate the language's capabilities and its relevance in diverse domains, from game development to high-performance computing.
Core Concepts to Master
Variables and Data Types
In C++, variables are the foundational building blocks that store data. Each variable has a type that defines what kind of data it can hold. The basic data types in C++ include:
- int: Stores integer values.
int count = 10;
- float: Stores floating-point numbers.
float temperature = 36.6;
- char: Stores single characters.
char grade = 'A';
- bool: Stores boolean values (true/false).
bool isPassed = true;
Understanding how to declare and manipulate these basic types is crucial for handling data effectively in any C++ program.
Control Structures
Control structures dictate the flow of the program based on conditions. Common control structures in C++ are:
- if-else statements allow branching logic.
if (score >= 50) {
cout << "Pass";
} else {
cout << "Fail";
}
- Switch cases simplify multiple conditions based on a single variable.
switch (grade) {
case 'A':
cout << "Excellent!";
break;
case 'B':
cout << "Good!";
break;
default:
cout << "Keep Trying!";
}
- Loops, such as `for` and `while`, enable repetitive execution of code.
for (int i = 0; i < 5; i++) {
cout << i;
}
Functions and Scope
Functions are reusable blocks of code, enabling modular programming. Understanding local and global scope helps maintain clean and manageable code. A simple function might look like this:
int add(int a, int b) {
return a + b;
}
Here, `a` and `b` are function parameters that operate within the local scope. In contrast, global variables can be accessed anywhere in the program, which is useful but can lead to unintended side effects if not managed well.
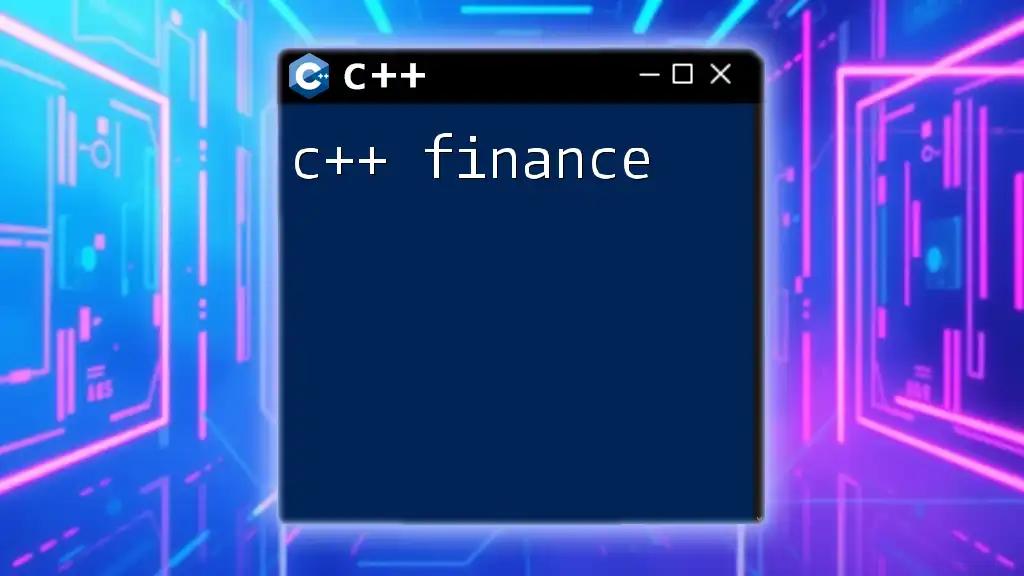
C++ Object-Oriented Programming (OOP) Principles
Introduction to OOP
Object-Oriented Programming (OOP) is a paradigm that allows programmers to model real-world entities. It is rooted in four key principles: Encapsulation, Inheritance, Polymorphism, and Abstraction. Mastering these principles is vital for efficiently structuring complex C++ applications.
Practical Examples
Encapsulation
Encapsulation is the bundling of data and methods that operate on that data within a single unit, or class. This limits exposure and enhances security. For example:
class Student {
private:
string name;
public:
void setName(string studentName) {
name = studentName;
}
string getName() {
return name;
}
};
Here, `name` is encapsulated and can only be accessed through its public methods.
Inheritance
Inheritance allows one class (derived class) to inherit properties and behaviors from another (base class). It promotes code reuse and establishes a hierarchy. For example:
class Person {
public:
void speak() {
cout << "Hello!";
}
};
class Student : public Person {
public:
void study() {
cout << "Studying";
}
};
Now the `Student` class can utilize the `speak` method from the `Person` class.
Polymorphism
Polymorphism allows methods to do different things based on the object that it is acting upon. This can be achieved through function overloading and function overriding. Here's an example of function overloading:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
Function overriding demonstrates polymorphism in a derived class:
class Base {
public:
virtual void display() {
cout << "Base class";
}
};
class Derived : public Base {
public:
void display() override {
cout << "Derived class";
}
};
Abstraction
Abstraction allows hiding complex implementation details while exposing only the necessary parts. It can be achieved using abstract classes. An abstract class cannot be instantiated and is defined as follows:
class AbstractClass {
public:
virtual void pureVirtualFunction() = 0; // Pure virtual function
};
Derived classes must implement the pure virtual function to become concrete classes.
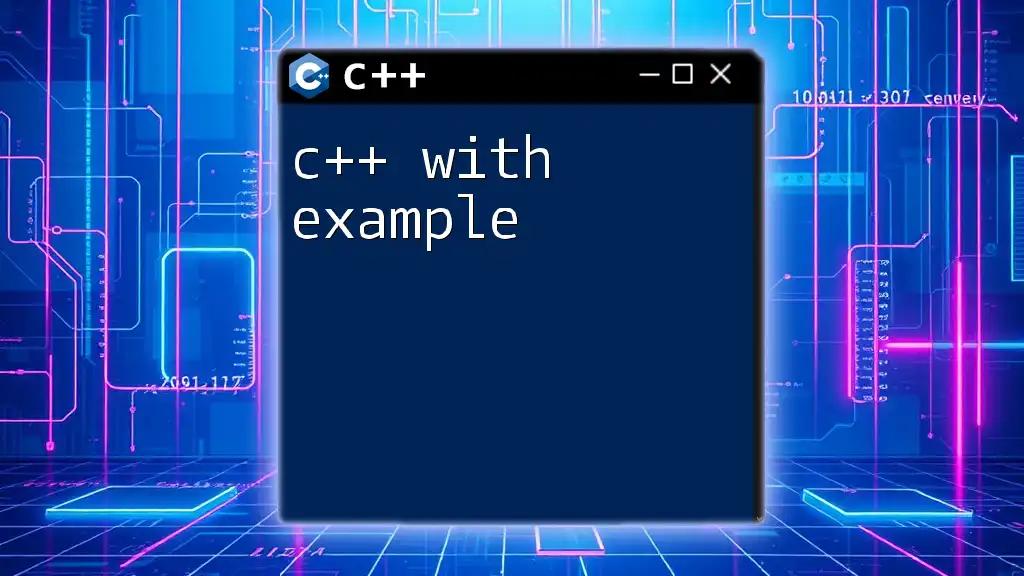
Preparing for the Final Exam
Study Techniques
Effective study techniques are crucial to grasping C++ concepts thoroughly. Employing strategies like active recall and spaced repetition can enhance retention. Utilize resources like textbooks, online tutorials, and coding practice websites to reinforce learning.
Key Topics to Review Before Exam
Before sitting for the C++ final exam, refresh key topics including but not limited to:
- Data Structures: Arrays, strings, vectors, and their manipulations.
- Memory Management: Pointers, dynamic memory allocation, and smart pointers.
- Practical Coding Challenges: Regularly practice coding problems that test understanding of these concepts.
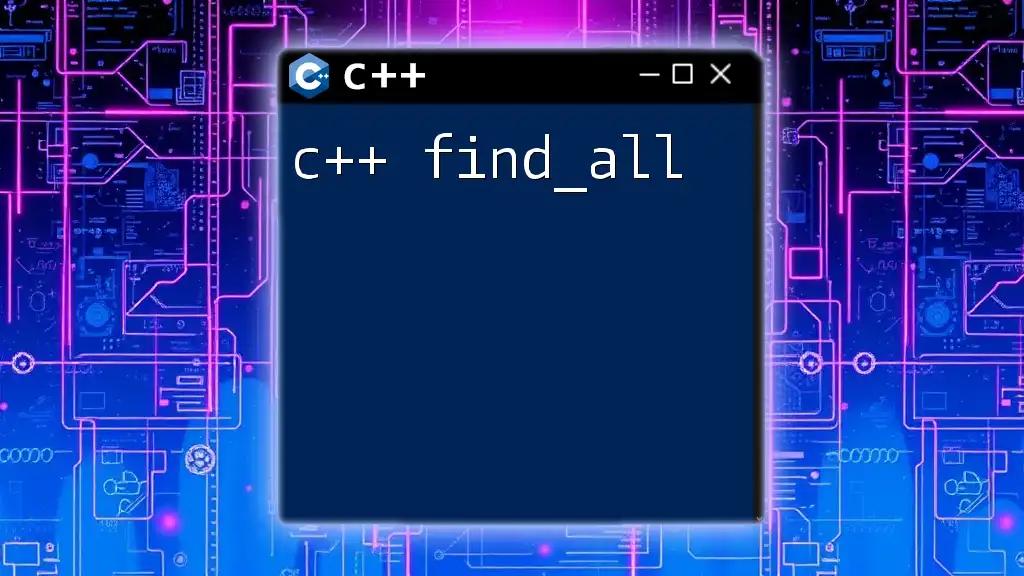
Sample Final Exam Questions
Multiple Choice Questions
During the final exam, expect questions that test your theoretical understanding. Here are a few sample questions:
- What does the `new` keyword do in C++?
- Allocates memory dynamically.
- Which of the following is not a C++ data type?
- `float64`
Coding Exercises
Coding exercises are essential for demonstrating practical skills. Here’s an example task:
Problem: Write a C++ function to reverse a string.
Solution:
string reverseString(string str) {
int n = str.length();
for (int i = 0; i < n / 2; i++) {
swap(str[i], str[n - i - 1]);
}
return str;
}
This function uses a loop to iterate through the string, swapping characters from opposite ends.
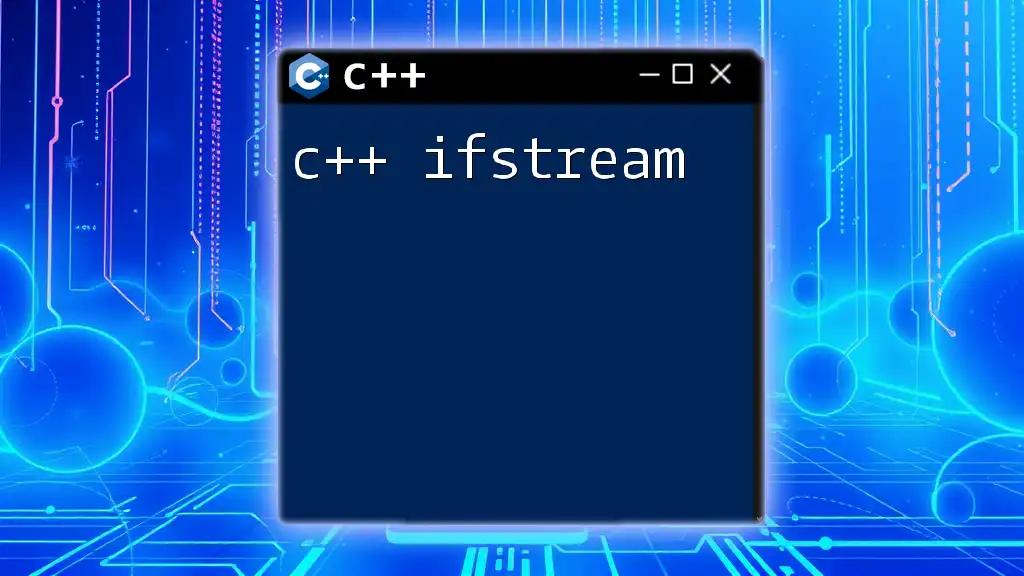
Common Mistakes in C++ Exams
Pitfalls to Avoid
Many students make common mistakes such as:
- Not initializing variables before use, which leads to undefined behavior.
- Misunderstanding pointer arithmetic, resulting in incorrect memory access.
- Confusing overloading vs. overriding, which can lead to logic errors.
Troubleshooting Techniques
Debugging is a valuable skill in C++. Familiarize yourself with common compiler errors and warnings. Analyzing these messages can provide clues about what went wrong in your code and how to fix it.
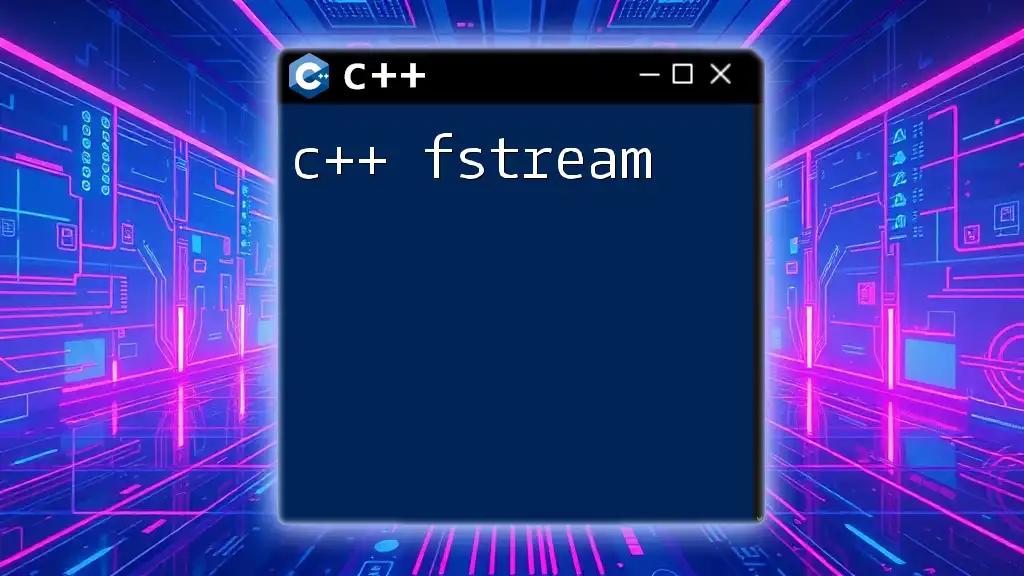
Final Preparations
Day Before the Exam
The day before the C++ final exam, ensure you have a checklist of topics to review. Focus on summarizing concepts rather than cramming new information. Additionally, get plenty of rest to ensure your mind is sharp for the exam.
During the Exam
Effective time management is essential during the exam. Allocate time to each section based on difficulty, and be mindful of not getting stuck on any single question. Read questions carefully to grasp what is being asked before formulating an answer.
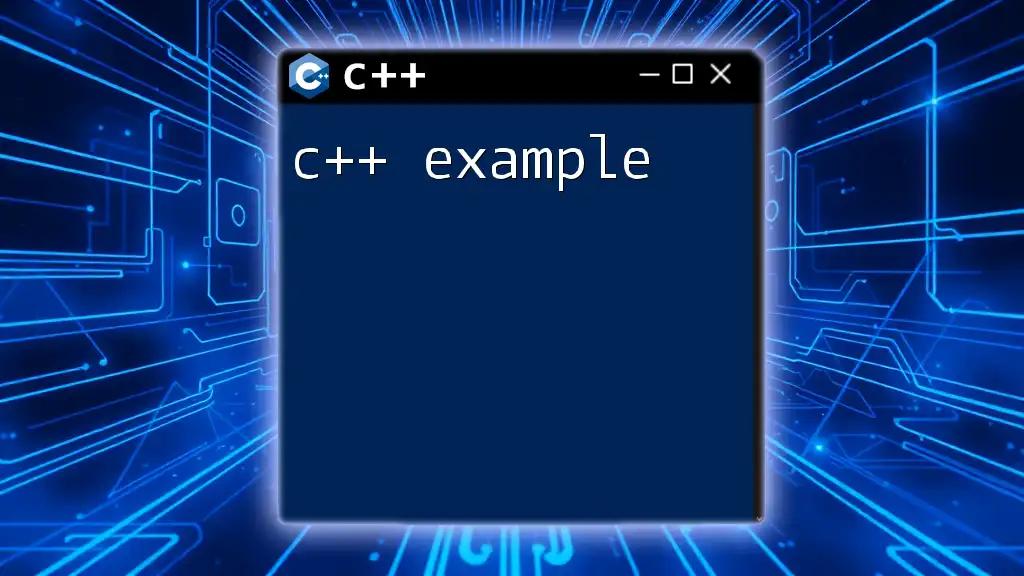
Conclusion
By mastering C++ fundamentals and OOP principles, preparing wisely, and avoiding common pitfalls, you will enhance your ability to succeed in your C++ final exam. Embrace the challenge as a chance to test your knowledge and skills, and remember to explore additional resources and communities to further your understanding of C++.
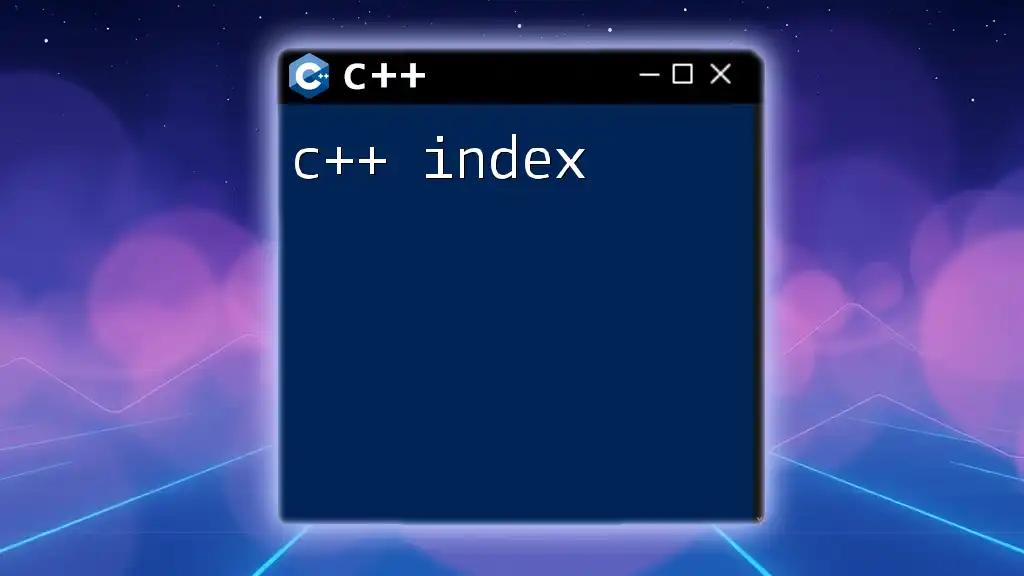
Additional Resources
Seek out online courses and platforms like Codecademy or Udemy for structured learning paths. Books such as "C++ Primer" and "Effective C++" can provide deeper insights and advanced techniques.

FAQs
What topics are usually covered in a C++ final exam?
Typically, exams cover core concepts of C++, including data structures, OOP principles, and memory management.
How can I handle exam anxiety?
Take time to prepare thoroughly and practice relaxation techniques, such as deep breathing, to remain calm during the exam.
What are tips for managing time effectively during the exam?
Review the exam format beforehand to know how to allocate your time, and prioritize questions you are most confident in answering.