C++ is the primary programming language used in Arduino development, enabling users to write concise code for controlling hardware and interacting with various sensors and modules.
Here's a simple code snippet for blinking an LED using Arduino with C++:
// Constants
const int ledPin = 13; // Pin number for the LED
// Setup function
void setup() {
pinMode(ledPin, OUTPUT); // Initialize the LED pin as an output
}
// Loop function
void loop() {
digitalWrite(ledPin, HIGH); // Turn the LED on
delay(1000); // Wait for one second
digitalWrite(ledPin, LOW); // Turn the LED off
delay(1000); // Wait for one second
}
What is Arduino?
Arduino is an open-source electronics platform based on easy-to-use hardware and software. It consists of a microcontroller board (such as the Arduino Uno) and an Integrated Development Environment (IDE) that enables you to write, compile, and upload code to the board. The platform has gained immense popularity among hobbyists, educators, and professionals due to its simplicity and versatility. Common applications include robotics, automation, home projects, and even art installations.
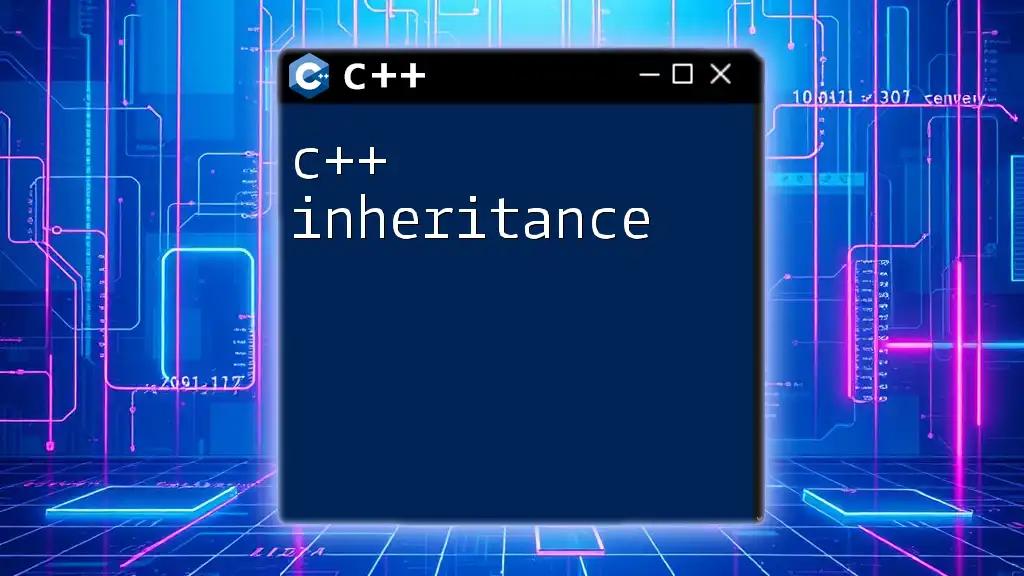
Understanding C++
C++ is a high-level programming language that extends the C language with object-oriented features. It's widely used in system/software development, game programming, and embedded systems. In the context of Arduino, C++ serves as the primary language for writing sketches (the programs you upload to your board), providing a rich set of features, such as object-oriented programming, templates, and more.
The importance of C++ in embedded systems cannot be overstated. It allows developers to write efficient code that interacts directly with hardware, making it an ideal choice for Arduino projects, where hardware control and performance are critical.
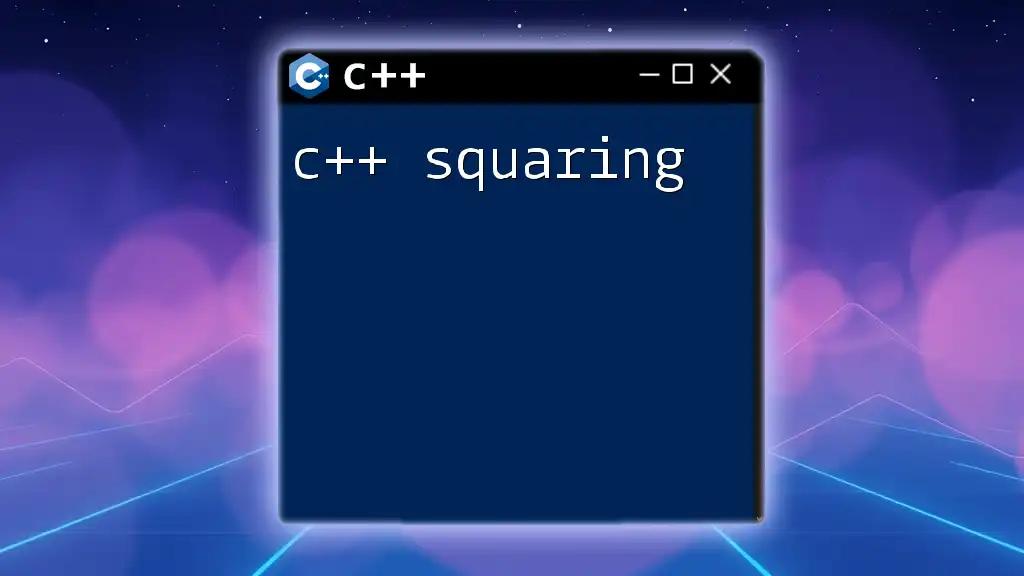
Why Use C++ with Arduino?
Using C++ with Arduino comes with numerous advantages:
-
Performance: C++ is a compiled language, which means that the Arduino IDE translates your code into machine language before execution. This results in faster execution compared to interpreted languages.
-
Control: C++ provides fine-grained control over system resources, such as memory and processing power, essential for achieving efficiency in embedded systems.
-
Modularity: C++ allows for modular programming through functions and classes, enabling you to create reusable code.
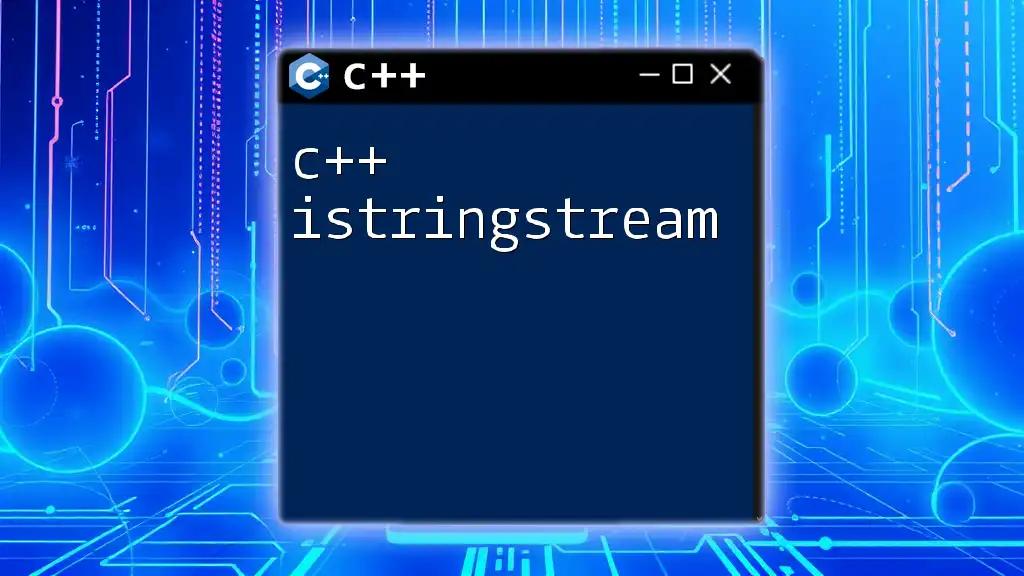
Setting Up Your Arduino Development Environment
To get started with C++ in Arduino, begin by installing the Arduino IDE. Follow these steps:
- Navigate to the official Arduino website and download the IDE for your operating system.
- Complete the installation process.
- Connect your Arduino board to your computer via USB.
- Open the Arduino IDE and select your board type from the “Tools” menu.
Your First C++ Arduino Sketch
Once your IDE is set up, it's time to write your first C++ sketch. The basic structure of an Arduino sketch consists of two essential functions: `setup()` and `loop()`.
- The `setup()` function runs once at the beginning and is used to initialize your variables, pin modes, and other settings.
- The `loop()` function runs continuously after `setup()` and is where the main logic of your program resides.
Here’s a simple sketch example that prints "Hello, World!" to the serial monitor:
void setup() {
Serial.begin(9600); // Initializes serial communication at 9600 bps
}
void loop() {
Serial.println("Hello, World!"); // Output to serial monitor
delay(1000); // Wait for one second
}
This straightforward example introduces you to the syntax and fundamental functions of C++ in Arduino programming.
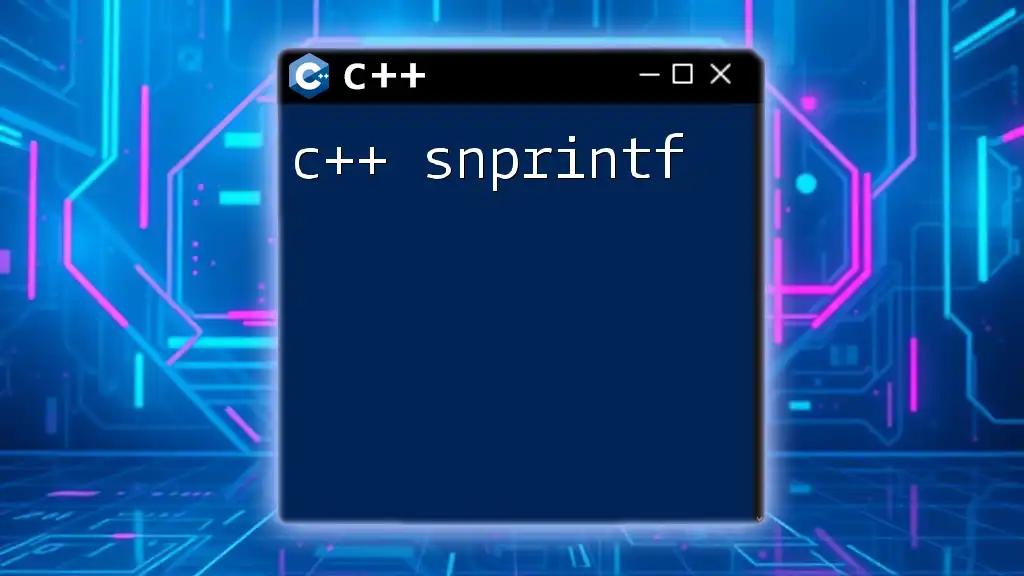
Exploring C++ Features in Arduino Projects
Fundamentals of C++ Syntax
C++ programming in Arduino allows for the declaration of variables using a variety of data types:
- int: for integers.
- float: for decimal values.
- char: for characters.
- bool: for boolean values (true or false).
An example of declaring a variable in C++ for Arduino is:
int ledPin = 13; // Define LED pin
Control Structures
Control structures in C++ help you manage the flow of the program based on conditions or iterations.
- Conditionals: Use `if`, `else if`, and `else` to make decisions based on conditions.
- Loops: Use `for` and `while` to repeat actions.
Here’s a code snippet demonstrating a `for` loop that prints numbers from 0 to 9:
for (int i = 0; i < 10; i++) {
Serial.println(i); // Prints numbers from 0 to 9
}
Functions and Modular Programming
Creating reusable functions in C++ simplifies your code and enhances readability. Here’s an example of a function that blinks an LED:
void blinkLED(int pin, int delayTime) {
digitalWrite(pin, HIGH);
delay(delayTime);
digitalWrite(pin, LOW);
delay(delayTime);
}
This function can be called with specific pin numbers and delay times, promoting modularity and reusability.
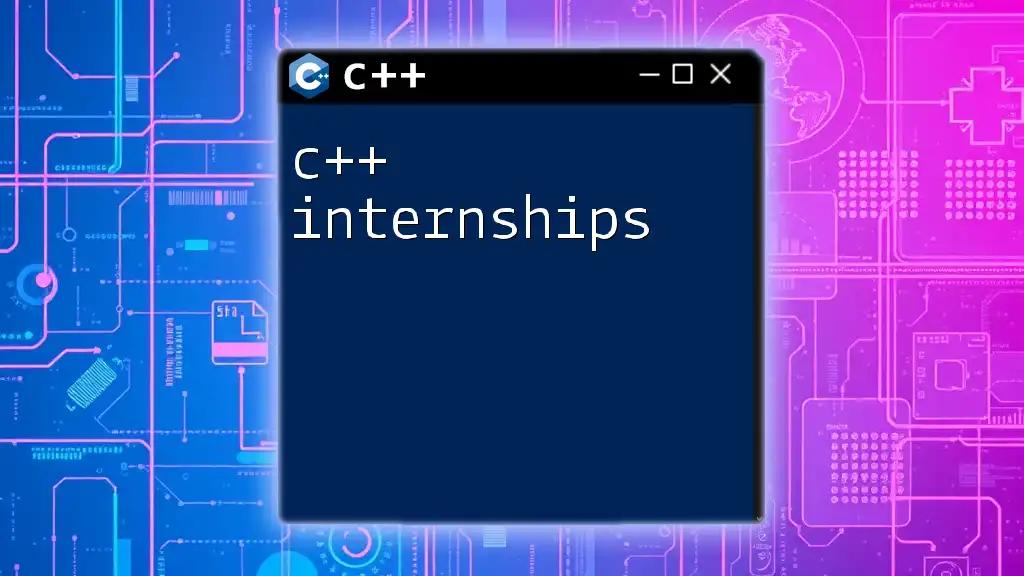
Advanced C++ Concepts for Arduino
Using Classes and Objects
C++ supports Object-Oriented Programming (OOP), which enables you to define classes and create objects. Using OOP principles can enhance the organization and modularity of your code.
Here’s an example of a simple class for controlling an LED:
class LED {
private:
int pin;
public:
LED(int p) {
pin = p;
pinMode(pin, OUTPUT);
}
void on() {
digitalWrite(pin, HIGH);
}
void off() {
digitalWrite(pin, LOW);
}
};
This class encapsulates the properties and behaviors of an LED, making your code cleaner and easier to manage.
Templates and Standard Template Library (STL)
C++ templates enable you to write generic and highly reusable code. In Arduino projects, you can leverage the Standard Template Library (STL) to utilize data structures such as vectors and lists, which are helpful for managing dynamic data.
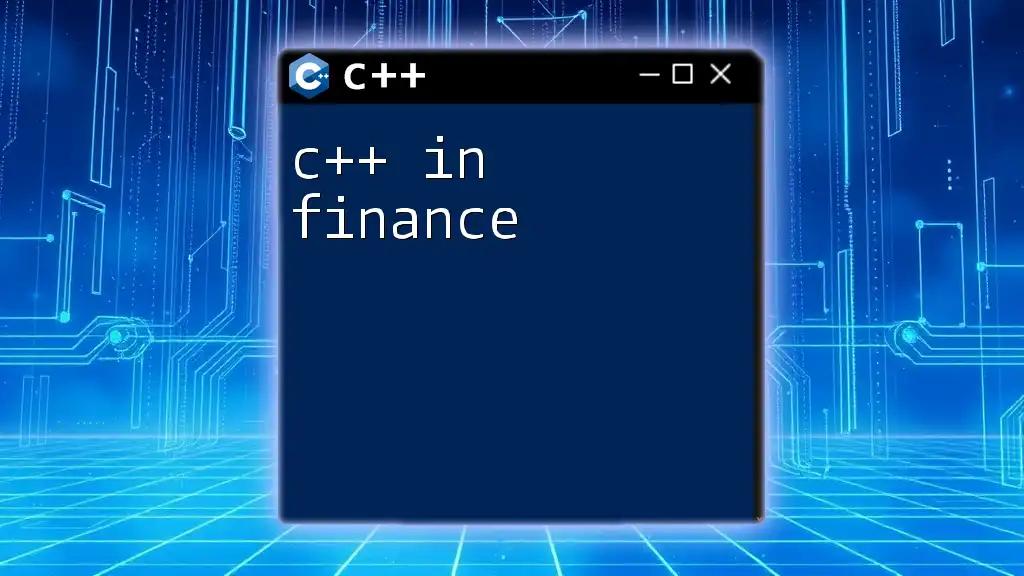
Practical Applications of C++ in Arduino Projects
Integrating C++ Libraries
Arduino supports a variety of libraries that extend its capabilities. To include a library in your project, use the `#include` directive. An example is the `Servo` library, which simplifies controlling servo motors. Here's how to use it:
#include <Servo.h>
Servo myServo;
void setup() {
myServo.attach(9); // Attaches the servo on pin 9
}
void loop() {
myServo.write(90); // Move servo to 90 degrees
delay(1000);
}
Sensor Interfacing with C++
Reading sensor values and responding to environmental changes is a common Arduino application. For example, to read temperature from a DHT sensor, you would set it up like this:
#include <DHT.h>
DHT dht(2, DHT11); // DHT sensor on pin 2
void setup() {
Serial.begin(9600);
dht.begin(); // Initialize sensor
}
void loop() {
float temperature = dht.readTemperature();
Serial.print("Temperature: ");
Serial.println(temperature);
delay(2000);
}
Creating User Interfaces
With libraries like `LiquidCrystal`, you can create user interfaces for your Arduino projects. This allows for real-time feedback and interactions with users. For example:
#include <LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 5, 4, 3, 2); // Initialize with pins
void setup() {
lcd.begin(16, 2); // Set up a 16x2 LCD
lcd.print("Hello, World!");
}
void loop() {
// Your repeat code here
}
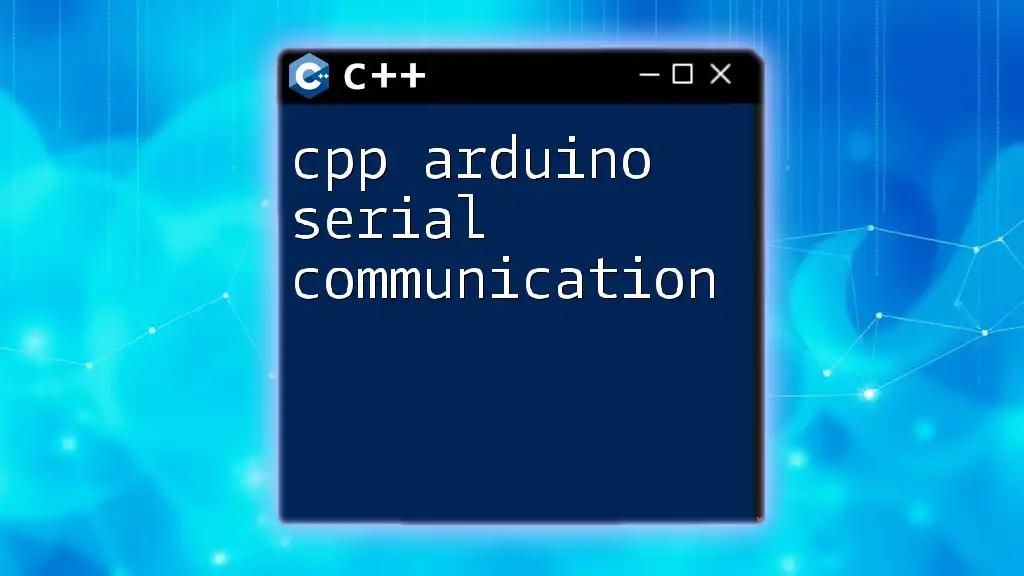
Debugging and Troubleshooting C++ Code on Arduino
Common Errors and Solutions
When working with C++, errors can range from syntax issues to logical mishaps. Common mistakes include:
- Syntax Errors: Ensure all semicolons and brackets are appropriately placed.
- Logical Errors: Review your logic carefully, especially conditions and iterations.
Using Serial Monitor for Debugging
The Serial Monitor in the Arduino IDE is a powerful tool for debugging. Utilizing `Serial.print()` statements throughout your code can help you track variable values and program flow in real-time. Make it a habit to use it frequently to ensure your code behaves as expected.
Best Practices for C++ Coding in Arduino
To write clean and effective C++ code for Arduino, adhere to these best practices:
- Organize your code into functions and classes for modularity.
- Comment your code generously to document your thought process.
- Maintain consistent naming conventions for variables and functions to promote readability.
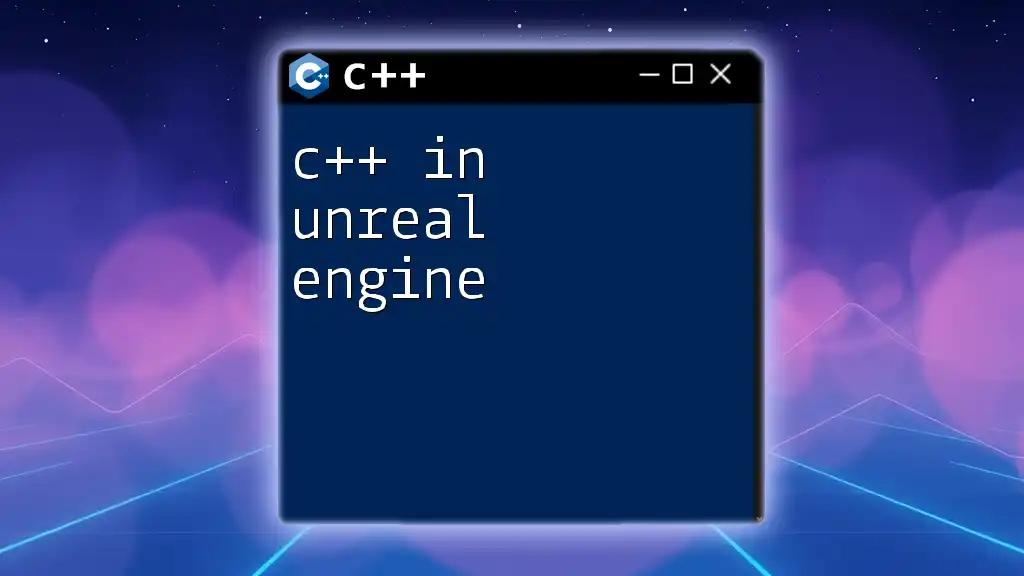
Conclusion
Using C++ in Arduino projects allows for powerful programming capabilities that enhance performance, control, and modularity. The flexibility of C++ combined with the accessibility of Arduino creates endless opportunities for innovation and creativity in your projects. Now that you're equipped with the foundational knowledge, it's time to explore, experiment, and bring your ideas to life.
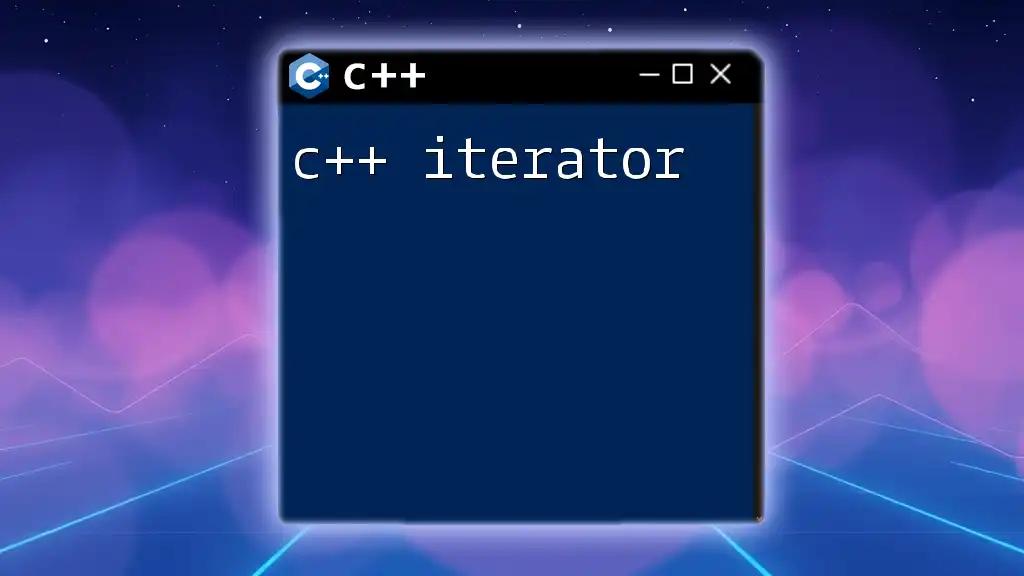
Additional Resources
To further your knowledge and skills in C++ for Arduino, consider exploring recommended books, online tutorials, and courses that can provide deeper insights and advanced concepts. Don't hesitate to challenge yourself with intricate projects that utilize everything you've learned!