In Arduino programming, C++ (cpp) commands are used to establish serial communication between the Arduino and a computer or other devices, enabling data exchange through the Serial library.
Here’s a simple code snippet to set up serial communication on an Arduino:
void setup() {
Serial.begin(9600); // Initialize serial communication at 9600 baud rate
}
void loop() {
Serial.println("Hello, world!"); // Send message over serial
delay(1000); // Wait for 1 second
}
Understanding Serial Communication
What is Serial Communication?
Serial communication is a method for transmitting data sequentially, one bit at a time, over a communication channel. This approach is essential in many electronics and computer applications because it simplifies wiring and allows for distance communication using fewer lines.
Key Differences Between Serial and Parallel Communication:
- Serial Communication sends one bit at a time, which is generally slower but requires fewer physical wires, making it cost-effective for long distances.
- Parallel Communication sends multiple bits simultaneously, which allows for faster data transfer over short distances but requires many wires, making it less feasible for longer connections.
Types of Serial Communication
-
Asynchronous Communication: This type does not require a clock signal to synchronize data. Start and stop bits indicate when the transmission begins and ends. This allows for flexible timing but can lead to errors if devices are out of sync.
-
Synchronous Communication: Requires a shared clock signal between two devices, ensuring they read the data at the same time. This method is faster but needs precise timing and more complex wiring arrangements.
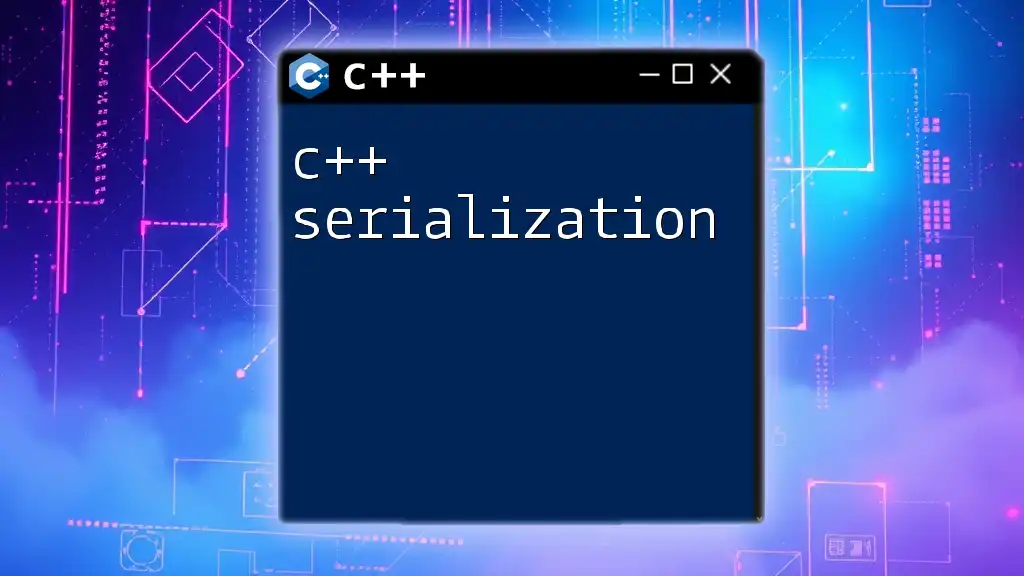
Setting Up the Arduino Development Environment
Installing the Arduino IDE
To start programming with C++ Arduino serial communication, you first need to install the Arduino Integrated Development Environment (IDE). Download the IDE from the official Arduino website and follow the installation instructions for your operating system.
After installation, open the IDE, which provides a simple interface to write and upload your code to the Arduino board.
Selecting the Right Board and Port
Selecting the appropriate Arduino board and port is crucial for successful programming. In the IDE, navigate to Tools > Board and select your specific Arduino model. Next, go to Tools > Port and choose the correct port that your board is connected to. This ensures that your code is uploaded to the right device.
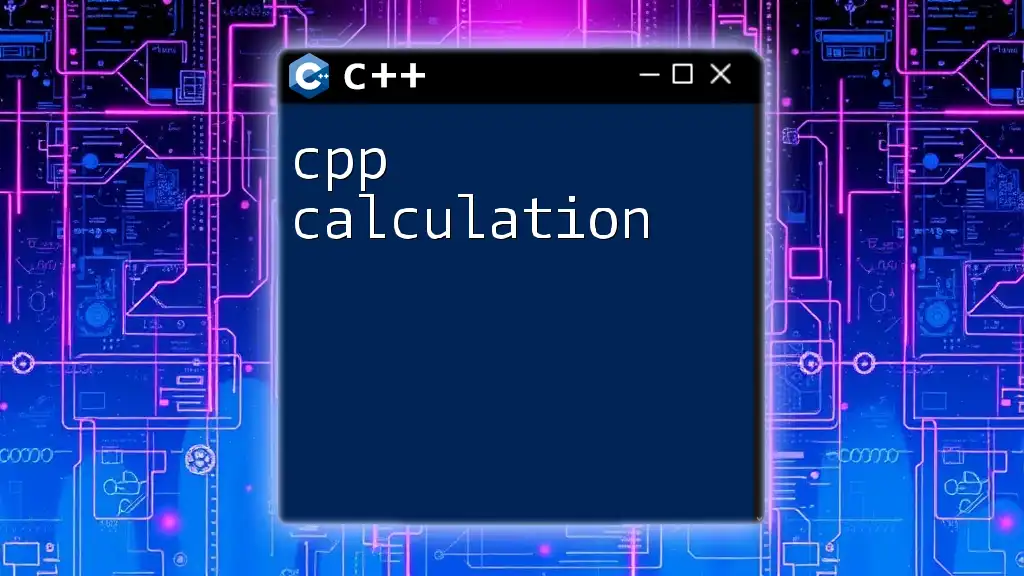
Basics of Arduino Serial Library
Introduction to the Serial Library
The Serial library is a core component of Arduino programming, providing functions to communicate over the serial port. It simplifies sending and receiving data, making it easier to integrate various components and sensors in your projects.
Key Functions in the Serial Library
-
Serial.begin(): This function initializes serial communication at a specified baud rate, setting the speed for data transmission. For example, `Serial.begin(9600);` starts communication at 9600 bits per second, a commonly used setting for many projects.
-
Serial.print() and Serial.println(): These functions are used to send data from the Arduino to the serial monitor. The difference is that println() appends a newline character, making the output more readable.
-
Serial.read() and Serial.available(): read() retrieves incoming data one byte at a time, while available() checks if data is ready to be read, which is essential for handling user input or data from sensors.
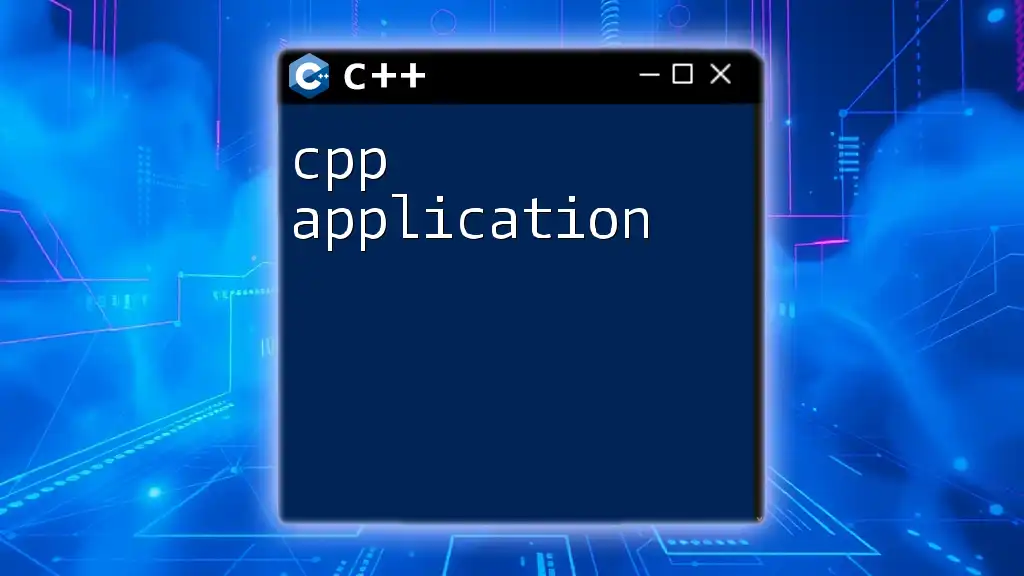
Writing Your First Serial Communication Program
Setting Up the Program
Getting started with your first Arduino serial communication program is straightforward. Here’s a basic example that sends the message “Hello, World!” to the serial monitor:
void setup() {
Serial.begin(9600); // Start serial communication at 9600 baud rate
}
void loop() {
Serial.println("Hello, World!"); // Send data to serial monitor
delay(1000); // Wait for a second
}
Explanation of the Code
-
Serial.begin(9600): This sets up serial communication at a baud rate of 9600. The baud rate determines how fast the data is sent and must be matched on both the sending and receiving devices.
-
Serial.println("Hello, World!"): This line sends the string “Hello, World!” followed by a newline character to the serial monitor, where you can see the output.
-
delay(1000): This function pauses the program for 1000 milliseconds (or one second) between messages, allowing you to read the output on the serial monitor easily.
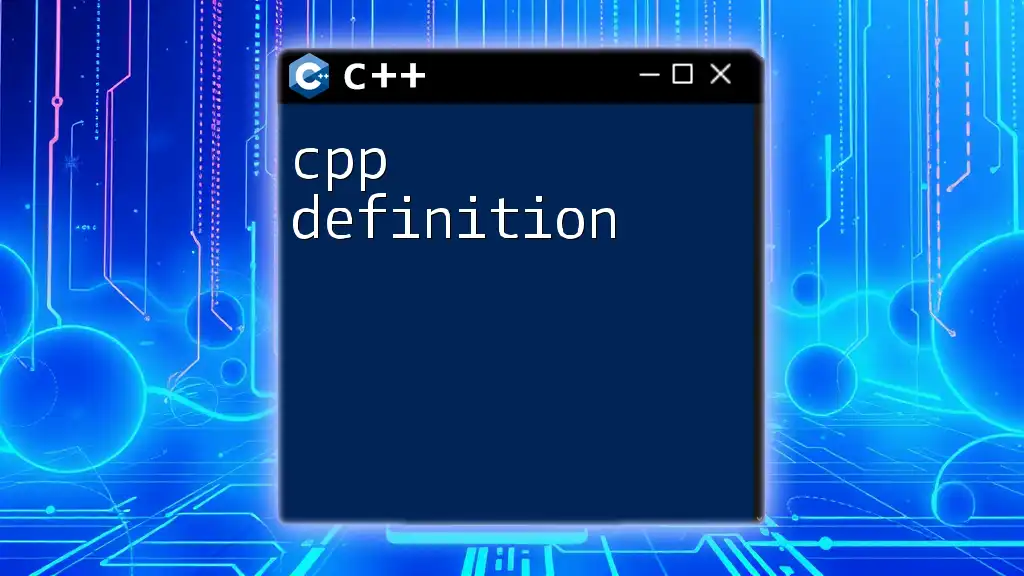
Advanced Serial Communication Techniques
Reading User Input from Serial Monitor
Reading user input is crucial for interactive projects. The following code snippet demonstrates how to read a character input from the serial monitor:
void setup() {
Serial.begin(9600);
}
void loop() {
if (Serial.available()) {
char input = Serial.read(); // Read the incoming data
Serial.print("Received: ");
Serial.println(input);
}
}
Explanation: In this program, `if (Serial.available())` checks if there are any bytes available to read. If so, it reads a byte of input and prints it back to the serial monitor, which is useful for debugging and user interaction.
Sending Data to Other Devices
Arduino's serial capabilities allow you to send data to other devices, such as sensors. By integrating a temperature sensor with serial communication, you can easily monitor temperature changes. An example could be connecting a DHT11 temperature sensor and sending the readings to the serial monitor.
Debugging Serial Communication
Issues can arise during serial communication, such as incorrect baud rates or mismatched data formats. Debugging Tips:
- Always ensure that both the sender and receiver are set to the same baud rate.
- Use the Serial monitor to output the state of variables or error messages to understand program behavior better.
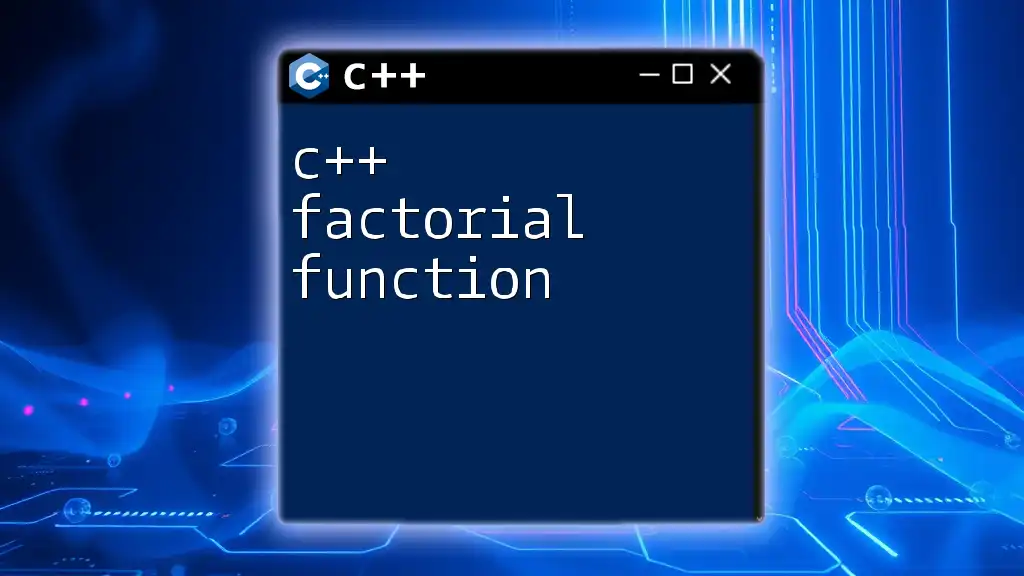
Practical Applications of Arduino Serial Communication
Project Ideas
-
Remote Temperature Monitoring: Create a project that uses a temperature sensor to read and transmit environmental data to a connected computer via serial communication. This application can help monitor room temperature or outdoor conditions.
-
Data Logging System: A serial communication system can log data from various sensors to your computer. By implementing a program that reads sensor data and transmits it over serial, you can easily collect and record environmental data for analysis.
Integrating Arduino with Software
Integrating Arduino with languages like Python or Processing allows for enhanced serial communication. You can read data from the Arduino in real-time and visualize it within a custom application. For instance, you can create a Python script to plot temperature readings received from the Arduino.
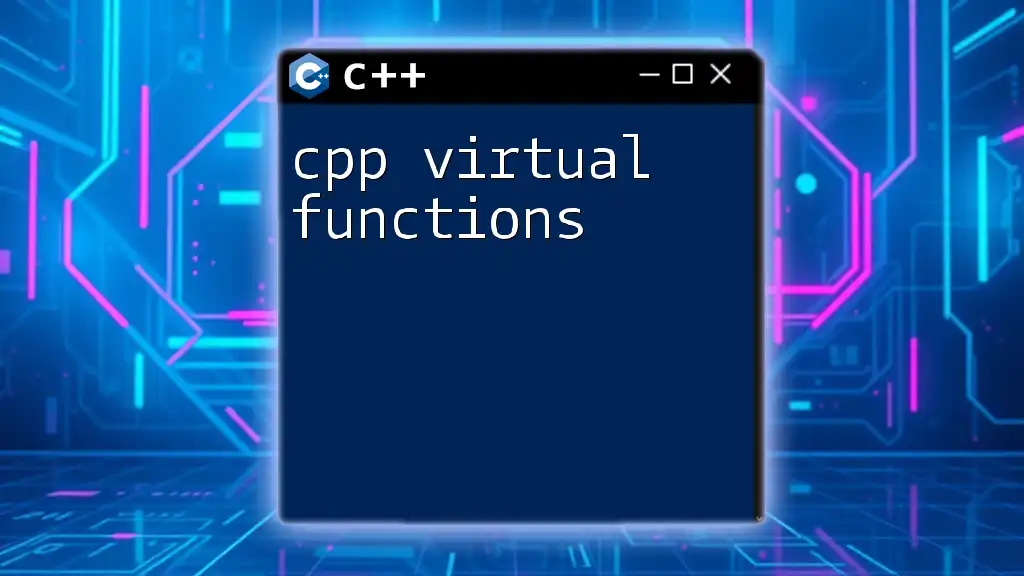
Best Practices for Serial Communication
Optimization Tips
- Minimize data transfer by sending only essential information to avoid overwhelming the communication channel.
- Be cautious when selecting the baud rate; higher rates enable faster data transfer but may lead to increased error rates if not handled correctly.
Avoiding Common Mistakes
- Ensure variable types match expected data types to avoid errors.
- Always initialize the serial port with `Serial.begin()` before attempting to send or receive data.
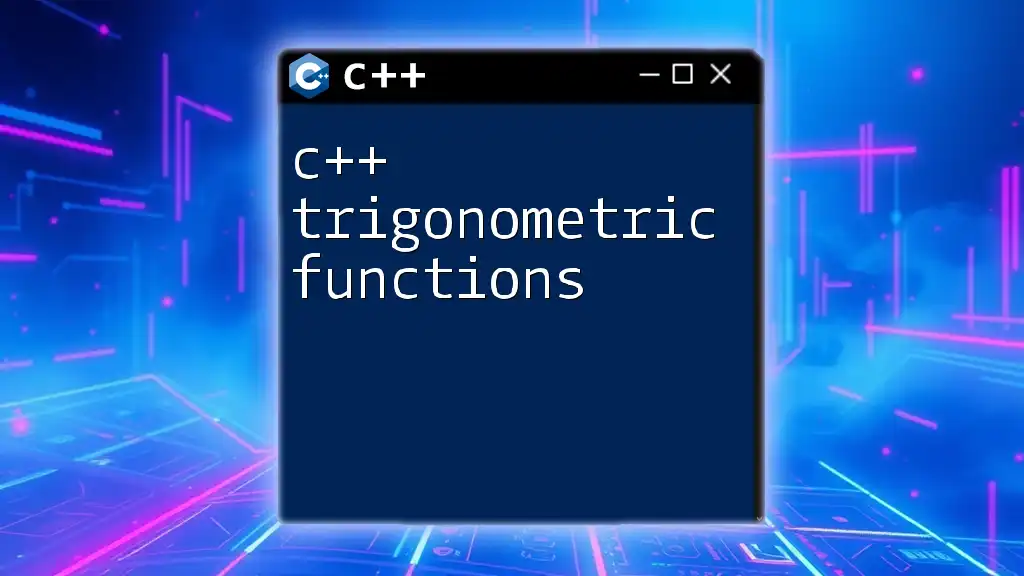
Resources and Further Learning
Books and Online Tutorials
Many resources are available for deeper learning, including Arduino programming books, online courses, and tutorials focusing specifically on serial communication.
Community and Forums
Engaging in community forums like the Arduino Forum or Stack Overflow can provide valuable insights, allowing you to ask questions and share experiences with other enthusiasts.
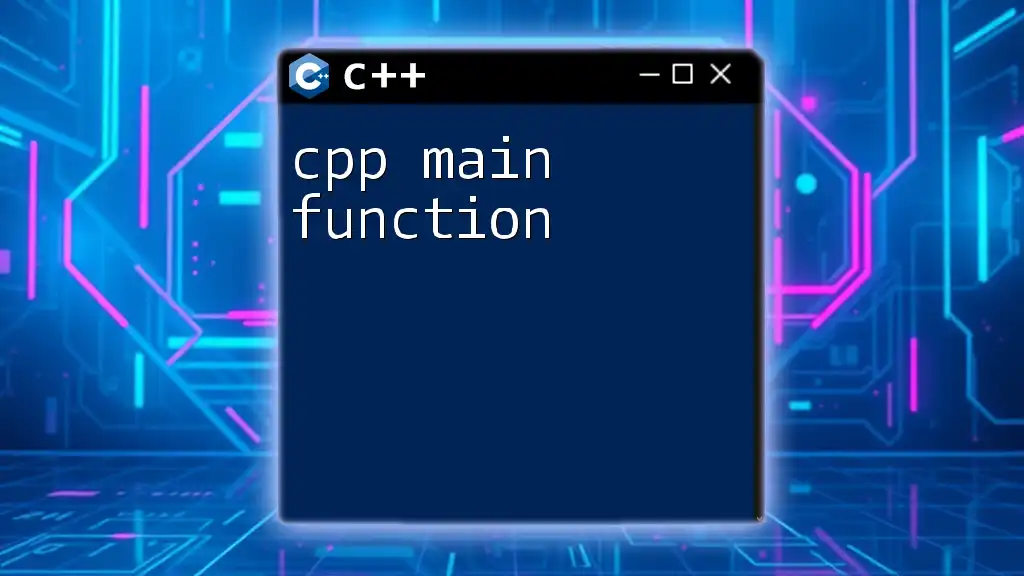
Conclusion
Mastering C++ Arduino serial communication is vital in creating interactive and effective electronic projects. With practice and experimentation, you can significantly enhance your programming skills and take your Arduino projects to new heights.
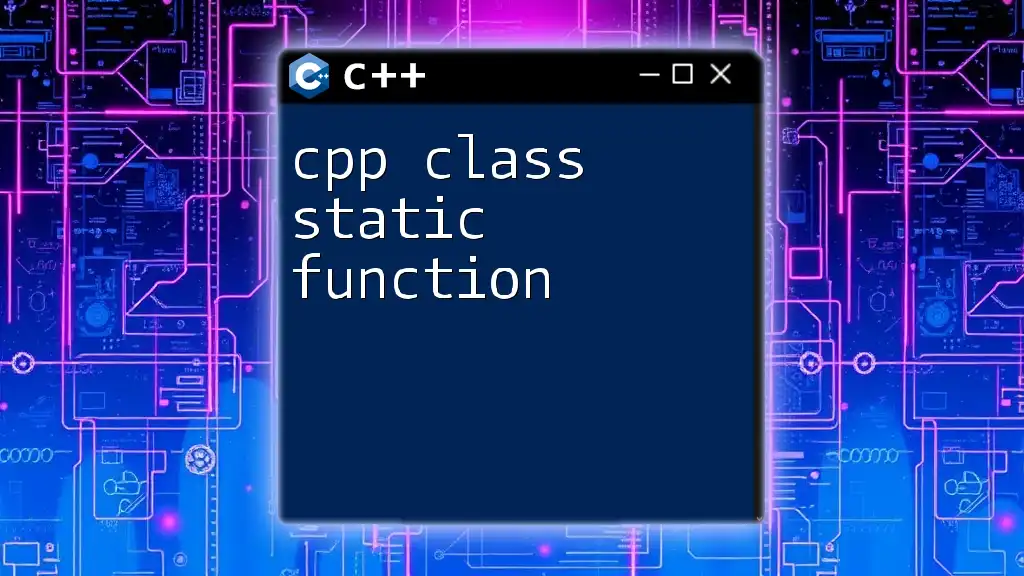
Call to Action
Feel free to share your experiences and projects utilizing various techniques of serial communication in Arduino. For more tips and insights on C++ programming, subscribe to stay updated with the latest in Arduino development!