A static function in a C++ class is a member function that belongs to the class itself rather than to any specific object instance, allowing it to be called without creating an instance of the class.
Here’s an example:
#include <iostream>
class MyClass {
public:
static void staticFunction() {
std::cout << "This is a static function." << std::endl;
}
};
int main() {
MyClass::staticFunction(); // Calling the static function
return 0;
}
Understanding C++ Static Member Functions
What is a Static Member Function?
A static member function in C++ is a function that belongs to the class rather than any particular object instance. Unlike non-static member functions, static functions can be called without creating an instance of the class. This feature makes them particularly useful for utility functions or operations that don't require access to instance-specific data.
Syntax of a C++ Static Member Function
The syntax for declaring a static member function is straightforward. Here’s how you can define it within a class:
class Example {
public:
static void staticFunction() {
// Function body
}
};
With this definition, you can call `staticFunction()` without creating an object of the `Example` class.
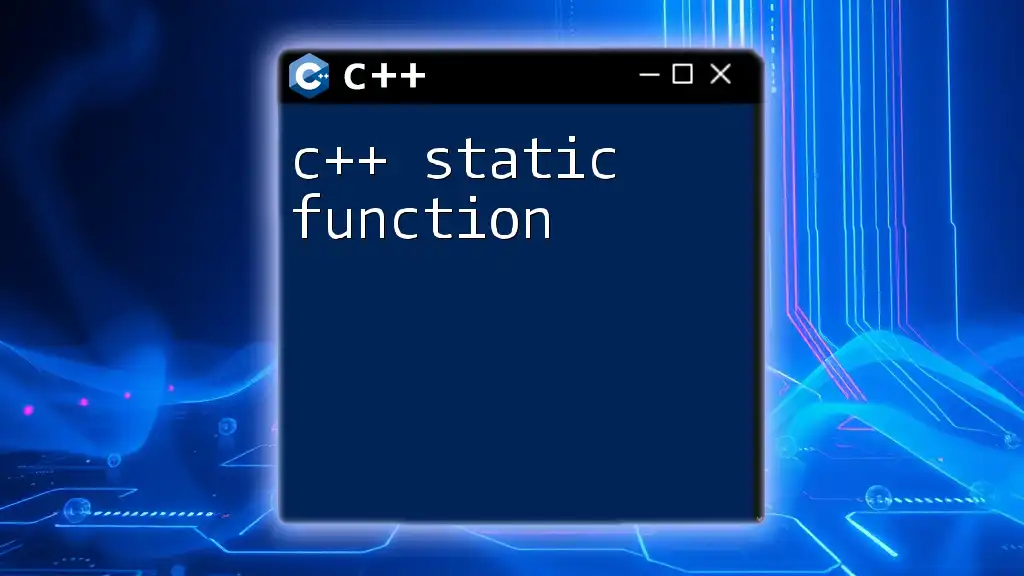
Characteristics of Static Member Functions
No `this` Pointer
One of the essential characteristics of static member functions is the absence of the `this` pointer. This means that static functions do not have access to instance variables or non-static members of the class, which enforces a level of encapsulation. This characteristic helps ensure that the function operates solely with its own parameters or static members of the class.
Accessing Static Members
You can call a static member function using the class name followed by the scope resolution operator `::`. Here’s an example:
Example::staticFunction();
Inside the class, static member functions can also access other static members directly. For instance:
class Example {
public:
static int count;
static void increment() {
count++;
}
};
In this example, `increment()` can modify the static variable `count` because both the function and the variable are static.
Sharing Data Across Instances
A crucial aspect of static member functions is their ability to share common data across all instances of the class. This is achieved through static variables. For example:
class Counter {
public:
static int count;
static void increment() {
count++;
}
static int getCount() {
return count;
}
};
Counter::count = 0;
Counter::increment();
std::cout << Counter::getCount(); // Output: 1
This illustrates that the static variable `count` is shared among all instances of `Counter`.
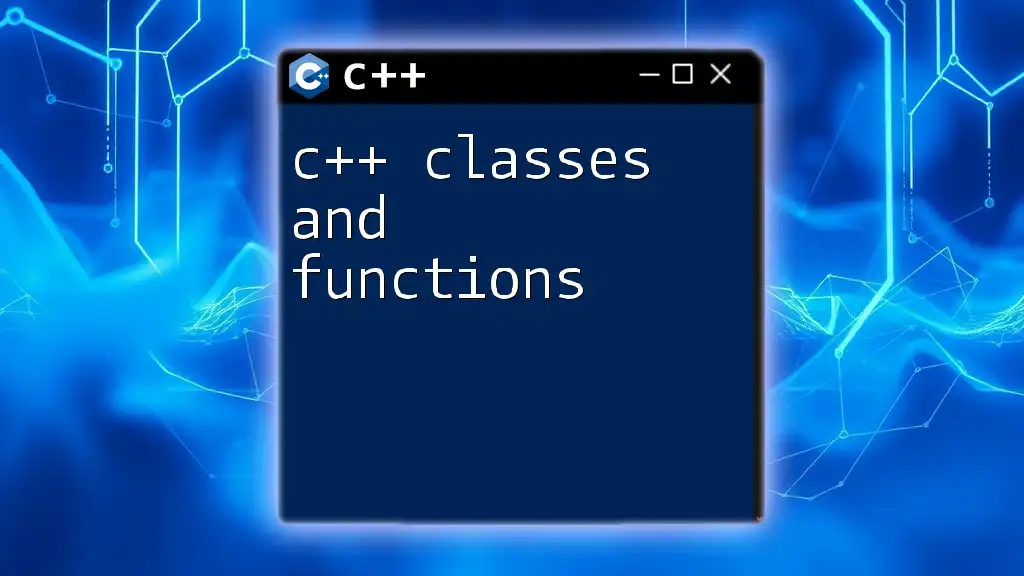
Advantages of Using Static Member Functions
Memory Efficiency
Static functions save memory since they don't require an object instantiation. This allows for creating utility functions that do not need to hold data but can still perform actions.
Utility Functions
Static member functions are perfect for defining utility functions that can be called without needing an instance. For example:
class MathUtilities {
public:
static int square(int value) {
return value * value;
}
};
You can now invoke `MathUtilities::square(5);` directly.
Encapsulation and Organization
Static member functions promote better organization in code. By grouping related functions within a class, you enhance code modularity and encapsulation, making it easier to maintain and understand.
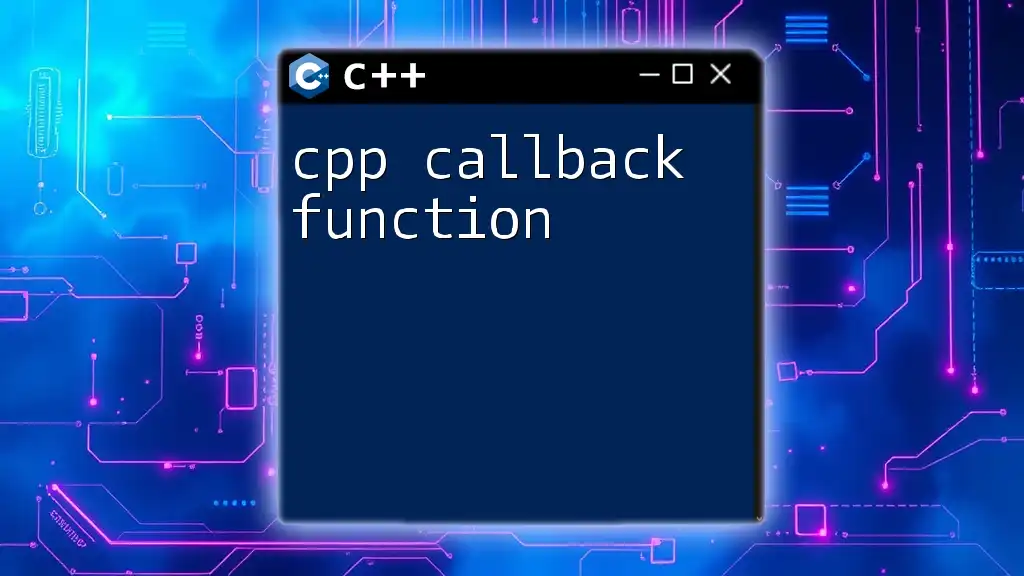
Limitations of Static Member Functions
No Access to Instance Variables
A significant limitation is that static member functions cannot access instance variables. If you try to use `this` or access non-static members, the compiler will throw an error. Here’s an illustration:
class Sample {
public:
int instanceVar;
static void staticFunction() {
// Error: cannot access instanceVar without an instance
// instanceVar = 10;
}
};
This restriction emphasizes the purpose of static functions — they operate at the class level.
Overhead of Static Context
While static functions offer several benefits, they can introduce overhead if erroneously overused. Relying on static functions excessively might lead to tightly-coupled code, compromising flexibility and robustness.
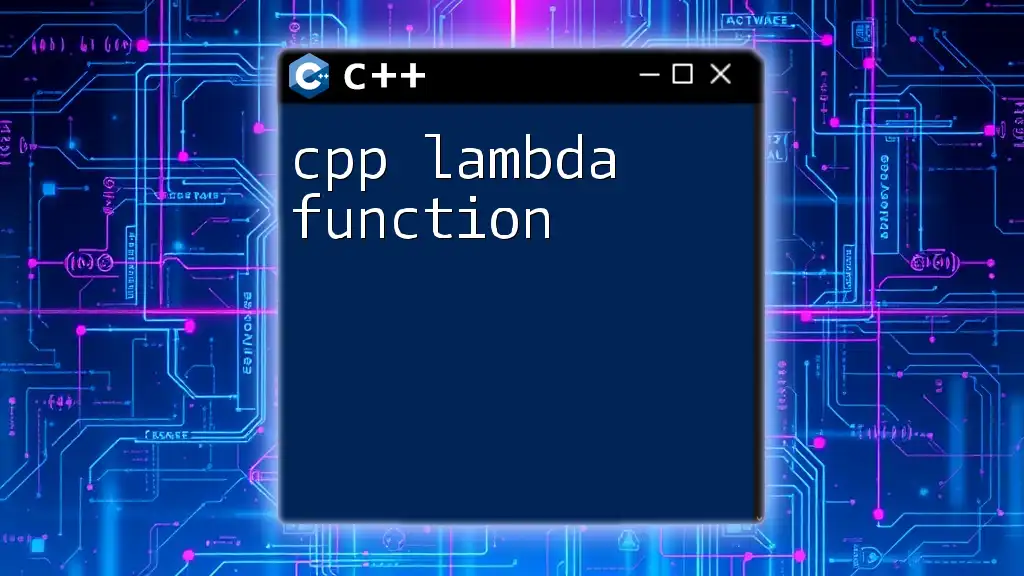
Common Use Cases for C++ Static Functions
Factory Methods
Static member functions often serve as factory methods, which help in creating and returning instances of a class without exposing object creation logic. Consider this example:
class Factory {
public:
static Example createInstance() {
return Example();
}
};
Using `Factory::createInstance()` allows for a clean way to instantiate objects.
Singleton Design Pattern
In the Singleton design pattern, static functions play a vital role by ensuring that only one instance of a class can exist. Here’s a simple example:
class Singleton {
private:
static Singleton* instance;
Singleton() {} // Private constructor
public:
static Singleton* getInstance() {
if (!instance) {
instance = new Singleton();
}
return instance;
}
};
Singleton* Singleton::instance = nullptr;
Logger or Utility Classes
Static member functions are frequently used in utility or logger classes since logging doesn't require object state. For instance:
class Logger {
public:
static void log(const std::string& msg) {
std::cout << msg << std::endl;
}
};
You can log messages without needing to instantiate `Logger`.
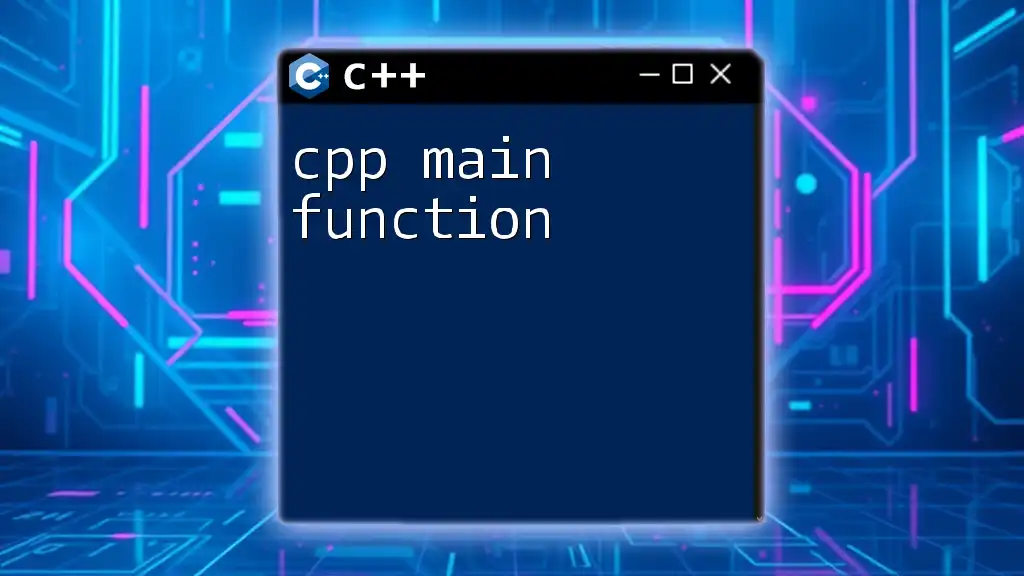
Best Practices for Using Static Member Functions
When to Use Static Member Functions
Use static member functions when you need functionality that doesn’t depend on object state. Situations like performing mathematical calculations, data parsing, or simple utility operations are ideal scenarios.
Avoiding Overuse of Static Functions
While static functions can simplify certain tasks, it's essential to avoid overusing them. They should not be used as a replacement for object-oriented principles. Strive for a balance between static and non-static design to maintain code clarity.
Code Maintainability
Always prioritize code readability and maintainability. Even though static functions can be convenient, ensure that you structure code logically. Well-placed static functions can enhance modularity, but excessive static functions might lead to confusion.
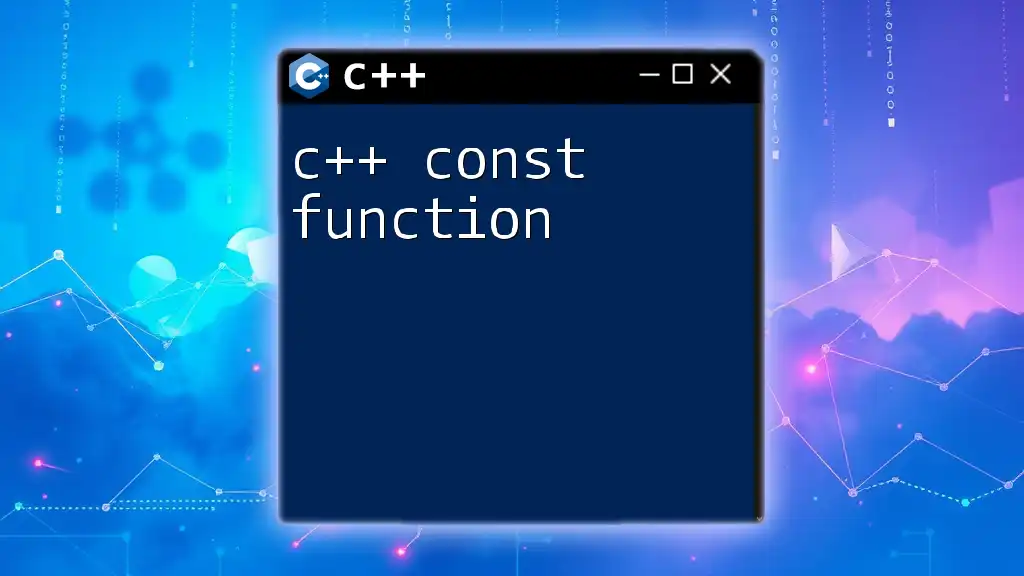
Summary
In conclusion, understanding cpp class static functions opens up vast possibilities for streamlined, efficient programming in C++. By leveraging static member functions, you can create memory-efficient, organized, and encapsulated code. However, it is crucial to be aware of their limitations and best practices to maintain clean and maintainable code. Embrace static functions as a powerful feature of C++, but use them judiciously to reap their full benefits in your programming ventures.
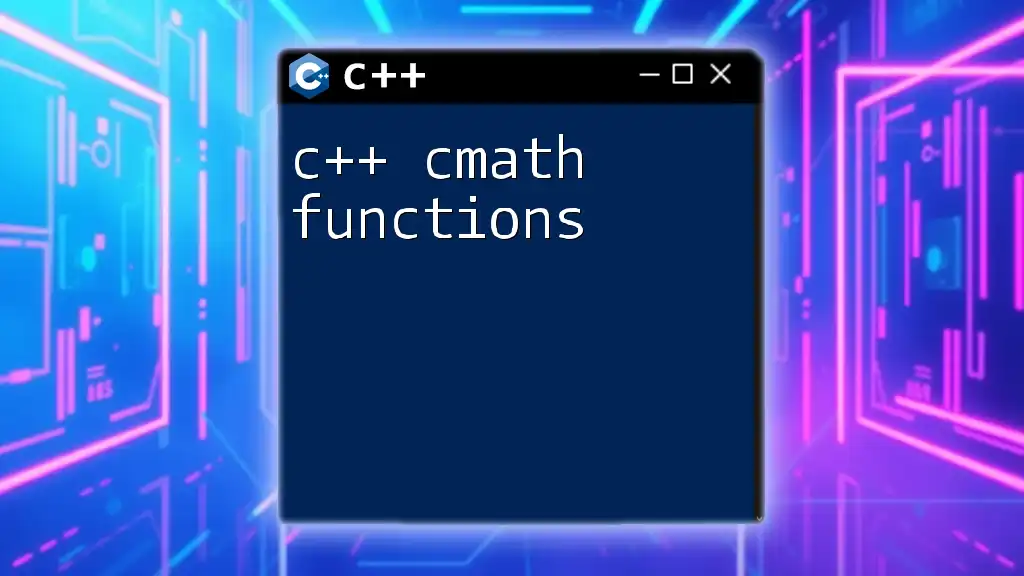
Additional Resources
Explore further by delving into recommended books and online tutorials that cover C++ in-depth. Familiarize yourself with C++ documentation for specific details and join community forums for discussions and questions that can enhance your understanding and application of C++ static member functions.