C++ classes encapsulate data and functions together, allowing for object-oriented programming, while functions define reusable blocks of code that can be called with different parameters to perform specific tasks.
Here's an example showcasing a simple class with a member function:
#include <iostream>
using namespace std;
class Rectangle {
public:
int width, height;
// Member function to calculate area
int area() {
return width * height;
}
};
int main() {
Rectangle rect;
rect.width = 5;
rect.height = 10;
cout << "Area: " << rect.area() << endl; // Output: Area: 50
return 0;
}
What is a Class in C++?
To understand C++ classes and functions, we first need to define what a class is. A class is essentially a blueprint for creating objects in C++, encapsulating data (attributes) and functionality (methods) related to that data. In the realm of object-oriented programming, classes are crucial as they allow developers to model real-world entities more intuitively.
Creating a class in C++ involves defining its attributes, methods, and the access levels for each. Classes allow us to encapsulate data and functions together, which promotes data hiding and modular programming.
Key Components of a C++ Class
Attributes (Data Members)
Attributes are the data stored within a class. They describe the properties of an object created from the class. For instance, consider a class `Car` which might have attributes for its brand, model, and year of manufacture.
Here’s an example:
class Car {
public:
string brand;
string model;
int year;
};
In this example, `brand`, `model`, and `year` are the attributes of the `Car` class.
Methods (Member Functions)
Methods are functions defined within a class that operate on the data. They define the behavior of the objects created from the class. Here’s how you might define a method to display the car's details:
class Car {
public:
void displayInfo() {
cout << "Brand: " << brand << ", Model: " << model << ", Year: " << year;
}
};
In this snippet, the `displayInfo` method allows us to output the attributes of the car.

Creating a C++ Class
Defining a C++ Class
Defining a class in C++ is straightforward. The class keyword is followed by the class name and a set of curly braces containing its members. Here’s how you can define a simple `Dog` class:
class Dog {
public:
string name;
int age;
void bark() {
cout << name << " says Woof!";
}
};
In this example, the `Dog` class has two attributes (`name` and `age`) and one method (`bark`).
Access Modifiers in C++
Access modifiers determine the accessibility of class members. The three primary access modifiers in C++ are:
- Public: Members are accessible from outside the class.
- Private: Members are accessible only within the class.
- Protected: Members are accessible within the class and by inheriting classes.
For example:
class Person {
private:
string ssn; // Only accessible within the class
public:
string name; // Accessible from outside the class
};
In this example, `name` is a public attribute that can be accessed externally, while `ssn` is a private attribute protected from outside access.
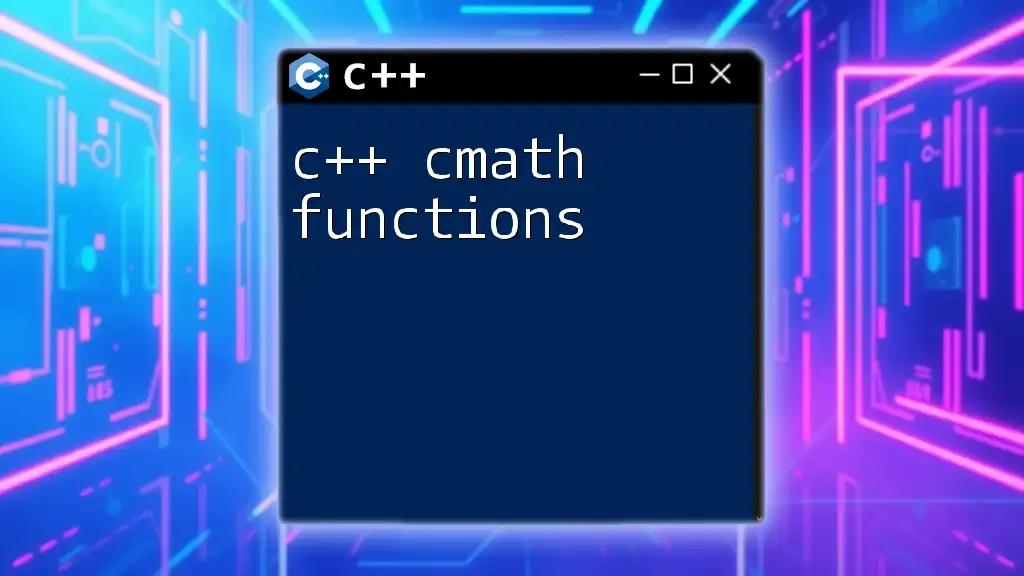
What are Functions in C++?
Overview of Functions
Functions are a foundational concept in C++, allowing code to be organized into reusable blocks. A function consists of a sequence of statements that perform a task. While functions can exist independently, member functions (or methods) are bound to the classes, dealing directly with the attributes of the class.
Types of Functions in C++
Functions in C++ can be classified into:
-
Standard Functions: Defined outside of classes and can access global variables or be passed arguments.
-
Member Functions: Defined within classes, allowing access to the class's attributes. They act on the class instance and usually manipulate or retrieve data stored as attributes.
Here's an example of a member function in a simple `Calculator` class:
class Calculator {
public:
int add(int a, int b) {
return a + b;
}
};
This `add` method takes two integers as parameters and returns their sum.
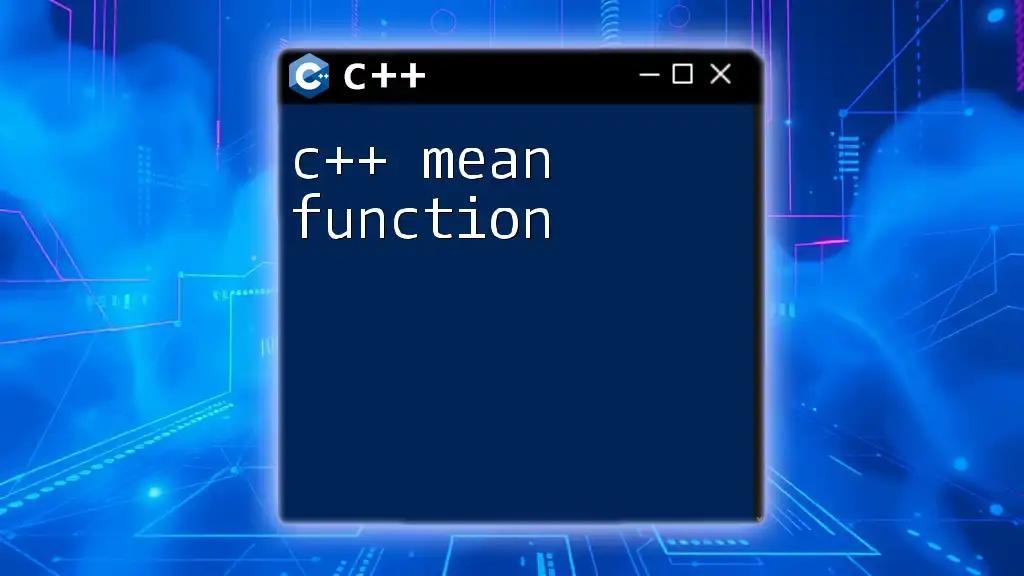
How to Use Functions within Classes
Calling Member Functions
Once a class is defined and an object is instantiated, its member functions can be called. For example, if we create an instance of the `Calculator` class and call its `add` method:
Calculator calc;
int result = calc.add(5, 3); // result will be 8
This demonstrates a simple call to a member function, showcasing how functions encapsulated in classes operate on the data of class instances.
Function Overloading
C++ supports function overloading, which allows multiple functions with the same name to coexist if they have different parameter lists. This feature is useful for creating more readable and intuitive code.
For example:
class Display {
public:
void print(int i) {
cout << "Integer: " << i;
}
void print(double d) {
cout << "Double: " << d;
}
};
In this case, the `Display` class has two `print` methods—one for integers and another for doubles, showcasing how function overloading can enhance usability.
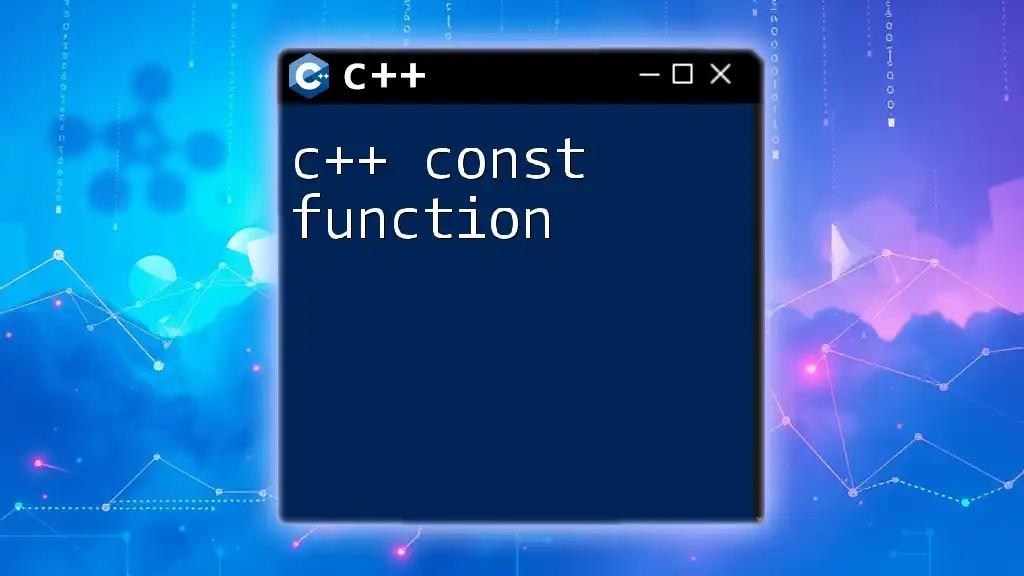
Constructors and Destructors in C++
What are Constructors?
Constructors are special member functions that initialize an object when it is created. A constructor has the same name as the class and does not return a value. Constructors can be default (no parameters), parameterized (with parameters), or copy constructors (that copy another object's data).
Here’s an example of a parameterized constructor in a `Book` class:
class Book {
public:
string title;
Book(string t) {
title = t;
}
};
In this example, we define a `Book` constructor that takes a title as a parameter and assigns it to the `title` attribute when creating a new `Book` object.
What are Destructors?
Destructors complement constructors by performing necessary cleanup when an object goes out of scope or is deleted. A destructor has the same name as the class but is preceded by a tilde (~).
Here’s an example of a destructor in a `Room` class:
class Room {
public:
~Room() {
cout << "Destructor called!" << endl;
}
};
In this code snippet, the destructor prints a message when a `Room` object is destroyed, demonstrating the object's lifecycle management.

The Role of Classes and Functions in C++ Programming
Benefits of Using Classes and Functions
When working with C++, using classes and functions offers numerous advantages:
- Code Reusability: Classes allow for the creation of reusable code units, reducing redundancy.
- Simplification of Complex Problems: By breaking down problems into smaller, manageable components, programmers can tackle complex tasks more effectively.
- Better Organization of Code Structure: Classes help in organizing code into logical units, facilitating easier maintenance and understanding.
Real-World Applications of Classes and Functions
Classes and functions are used extensively in modern software development. From libraries and frameworks to applications and games, the principles of classes and functions form the backbone of structural programs. For instance, standard libraries in C++ often make extensive use of classes to encapsulate complex operations, simplifying development for programmers.

Conclusion
In summary, C++ classes and functions are vital components of the language that empower developers to create efficient, maintainable, and reusable code. By understanding how to define and implement classes, as well as how to use functions, developers can model real-world scenarios effectively in their applications.
Practicing with classes and functions will enhance your programming skills, paving the way for diving into more advanced C++ topics. Keep experimenting and building!