The C++ `length()` function is used to determine the number of characters in a string, and it can be invoked on a string object as shown in the following example:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
std::cout << "Length of the string: " << myString.length() << std::endl;
return 0;
}
What is the Length Function in C++?
The C++ length function is essential for developers, enabling them to determine the size of containers, particularly strings. Understanding this function is vital as it plays a significant role in memory management and data processing. The length function should not be confused with similar concepts like the size function, which can sometimes lead to confusion among beginners.
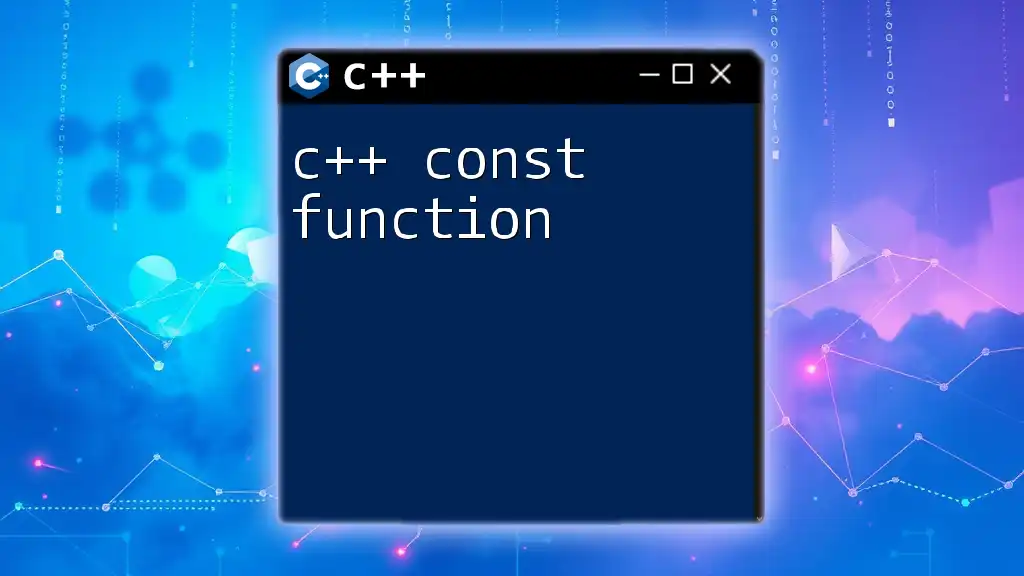
Understanding String Length in C++
The std::string Class
In C++, the `std::string` class provides a robust way to handle strings as compared to traditional C-style strings (character arrays). The class encapsulates various functionalities, making it easier to manipulate string data effectively.
Using the Length Function
The `length()` method is a member function of the `std::string` class used to retrieve the number of characters in a string. The syntax is straightforward: `str.length()`.
Here's a simple example illustrating its use:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::cout << "Length of string: " << str.length() << std::endl;
return 0;
}
In this example, the output would yield `Length of string: 13`, as the calculation includes letters, punctuation, and spaces.
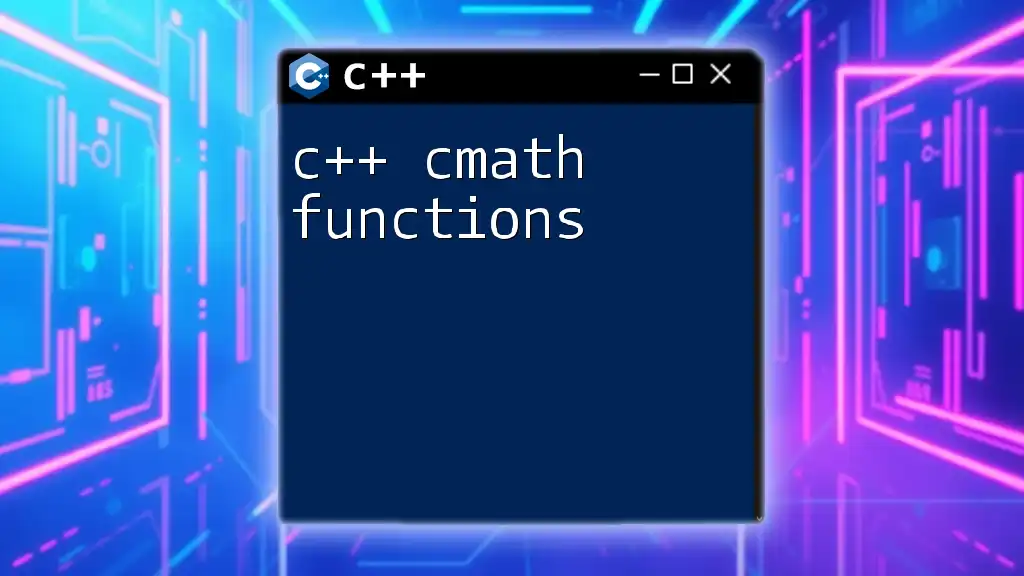
The Len Function in C++
Is There a len() Function in C++?
Unlike Python, where a built-in `len()` function exists, C++ does not have a dedicated `len()` function. This often confuses newcomers. However, in C++, the `length()` and `size()` functions serve the same purpose when used on a `std::string` object, providing flexibility depending on the programmer’s preference.
Differences Between length() and size()
Both methods, `str.size()` and `str.length()`, return the same result for strings but can vary in meaning within different contexts or data types. Here's an illustrative example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
std::cout << "Length using length(): " << str.length() << std::endl;
std::cout << "Length using size(): " << str.size() << std::endl;
return 0;
}
This will output:
Length using length(): 5
Length using size(): 5
Both methods return the same value, highlighting their interchangeability.
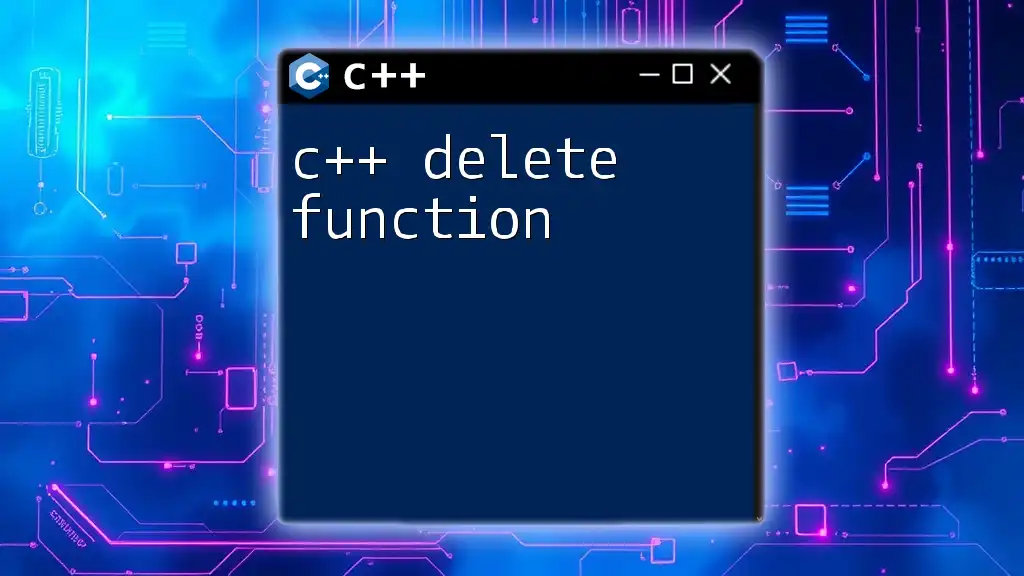
Length Function for Arrays
Determining the Length of Arrays
When it comes to arrays, C++ does not offer a direct method to calculate their length like the `length()` function for strings. Instead, you’ll often need to use the `sizeof` operator in combination with custom macros.
Here’s an example using a macro to determine the length of an array:
#include <iostream>
#define LENGTH(array) (sizeof(array)/sizeof(array[0]))
int main() {
int arr[] = {1, 2, 3, 4, 5};
std::cout << "Length of array: " << LENGTH(arr) << std::endl;
return 0;
}
This will output:
Length of array: 5
Limitations of Using sizeof
While using `sizeof`, it’s crucial to understand its limitations, especially in the context of dynamic arrays. The formula may lead to incorrect results if you apply it to pointers or arrays that are passed to functions. As arrays decay to pointers in functions, the length information is lost. Always ensure that you are in the correct scope to avoid unexpected behaviors.
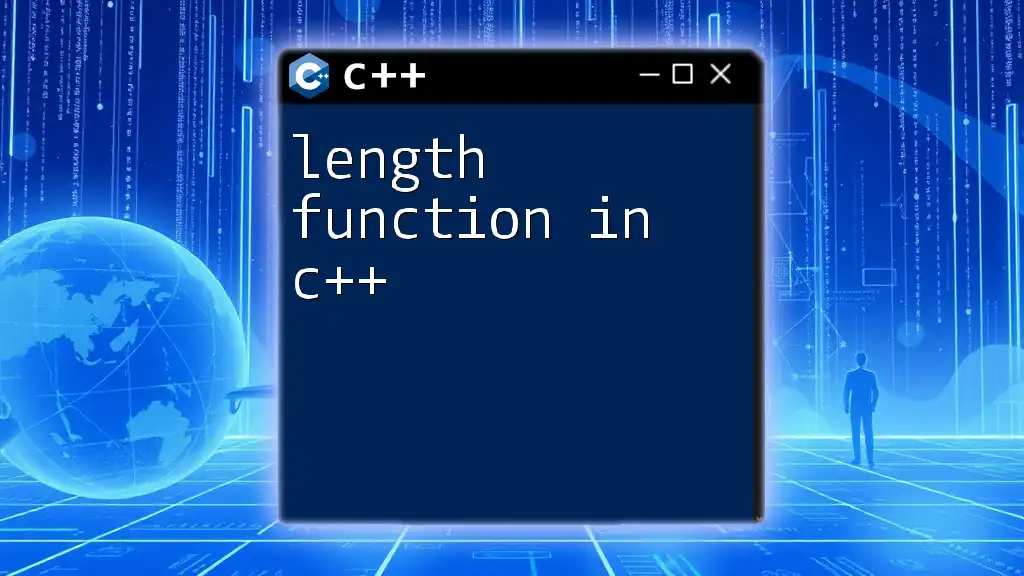
Custom Length Functions
Creating Your Own Length Function
You might find custom length functions useful, particularly when dealing with C-style strings. Below is an example of how you might create a custom function for this purpose:
#include <iostream>
int customLength(const char* str) {
int length = 0;
while (*str++) length++;
return length;
}
int main() {
const char* myString = "Hello";
std::cout << "Custom length function output: " << customLength(myString) << std::endl;
return 0;
}
The output will be:
Custom length function output: 5
This example demonstrates how the custom function iterates over each character until it reaches the null terminator (`'\0'`), showing how length can be calculated manually.
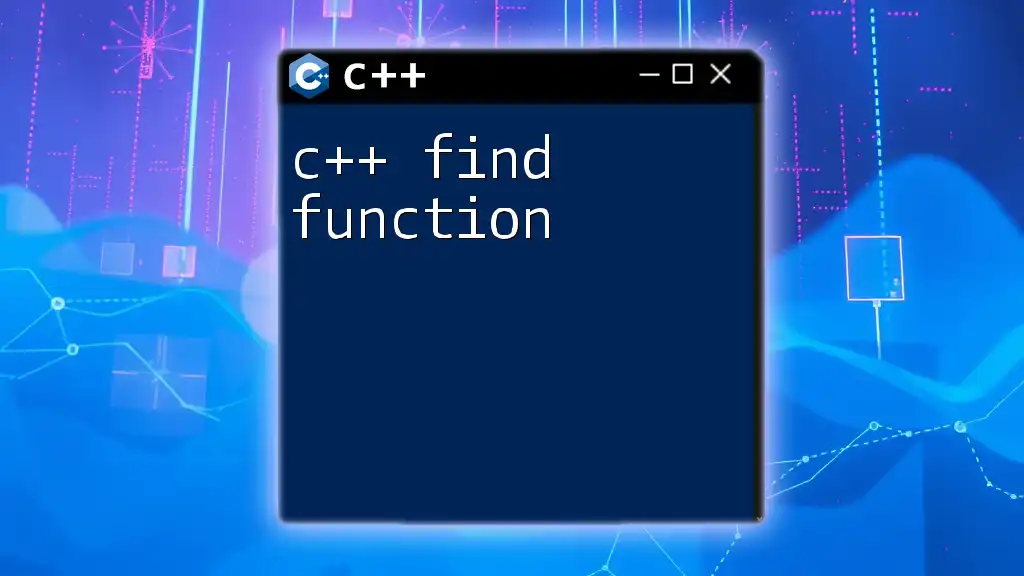
Common Use Cases for the Length Function
The C++ length function plays a crucial role in several programming scenarios. For example:
- Input validation: Ensuring that strings conform to specific size requirements before processing.
- Loop iterations: When iterating through strings or collections, length plays a vital role in deciding loop conditions.
- Memory management: Understanding the size of data being manipulated allows for better memory allocation and deallocation.
Consider a situation where you check if a user input matches the expected format by checking its length—this helps maintain data integrity.
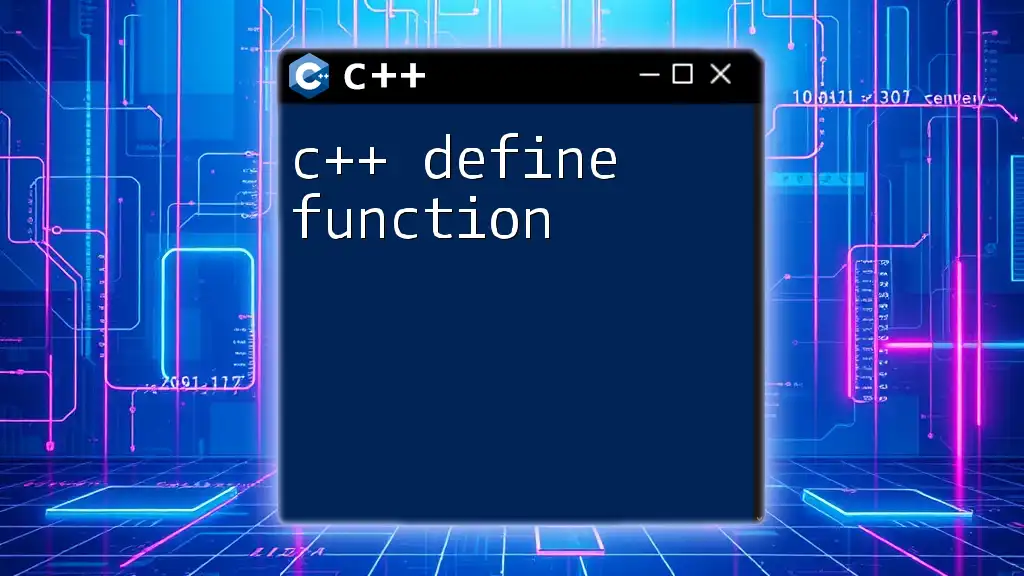
Best Practices
When to Use length() vs size()
While both methods essentially achieve the same outcome, it’s recommended to use `length()` for strings, as it explicitly communicates your intent about measuring string size. Results between the two functions are identical, but context matters when readability is concerned.
Handling Edge Cases
An understanding of edge cases, such as empty strings, is also paramount. An empty string using `length()` or `size()` will return `0`. It's essential to handle these cases gracefully to avoid runtime errors:
std::string emptyStr = "";
std::cout << "Length of empty string: " << emptyStr.length() << std::endl; // Outputs 0
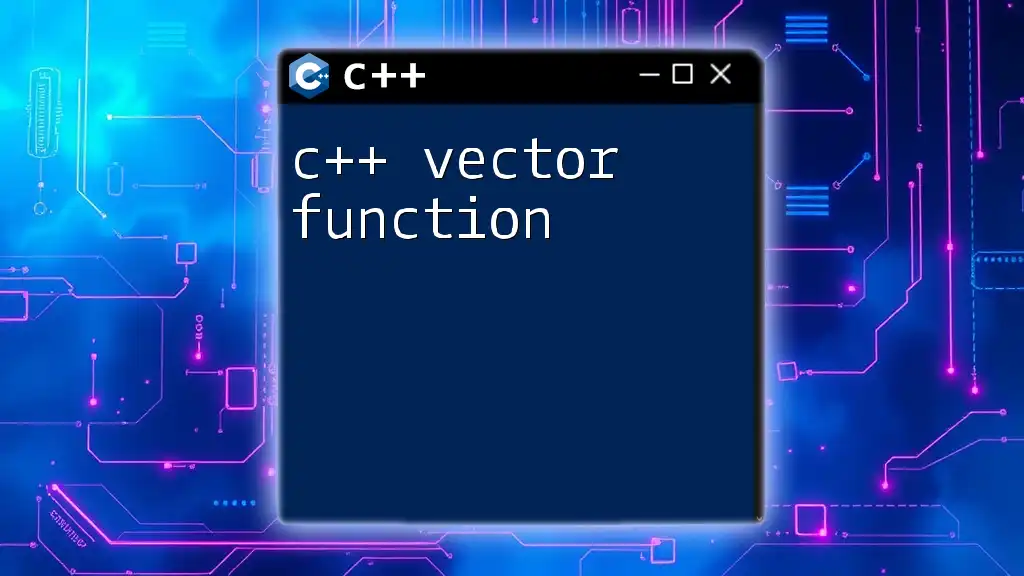
Conclusion
In summary, the C++ length function is an essential tool for any developer working with strings or array data structures. It’s vital to familiarize yourself with the distinctions between member functions like `length()` and `size()`, as well as creating your own custom solutions when necessary. Understanding how to utilize these functions effectively will significantly enhance your C++ programming skill set. Don’t hesitate to practice using these functions in diverse scenarios for a better grip on their functionality!
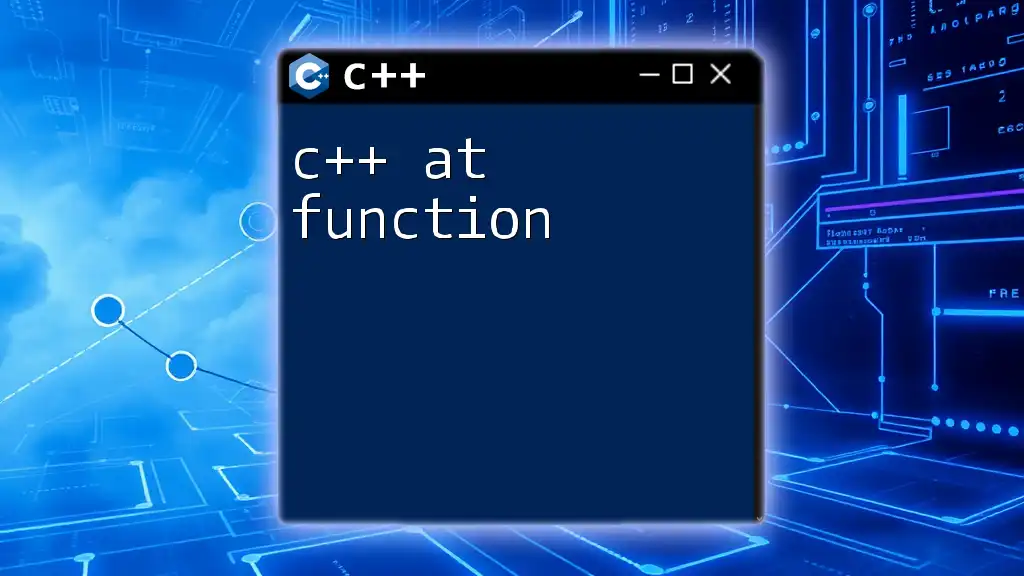
Additional Resources
For further understanding, consider referring to the official C++ documentation on the `std::string` class and seeking out additional tutorials and guides on C++ string manipulation and memory management for enhanced learning.