The C++ GCD function calculates the greatest common divisor of two integers using the Euclidean algorithm, and can be implemented as follows:
#include <iostream>
#include <algorithm>
int gcd(int a, int b) {
return std::gcd(a, b);
}
int main() {
int num1 = 36, num2 = 60;
std::cout << "GCD of " << num1 << " and " << num2 << " is " << gcd(num1, num2) << std::endl;
return 0;
}
What is GCD?
The Greatest Common Divisor (GCD) is the largest positive integer that divides two or more integers without leaving a remainder. For instance, the GCD of 8 and 12 is 4 because 4 is the highest number that divides both 8 and 12 evenly. Understanding GCD is essential in various fields, including mathematics, computer science, and algorithm design.
Importance of GCD in Mathematics and Programming
The GCD plays a crucial role in number theory and is often used in reducing fractions to their simplest forms, finding least common multiples, and factoring integers. In programming, it is frequently employed in algorithms that necessitate common divisors, such as those used in cryptography and optimization problems.
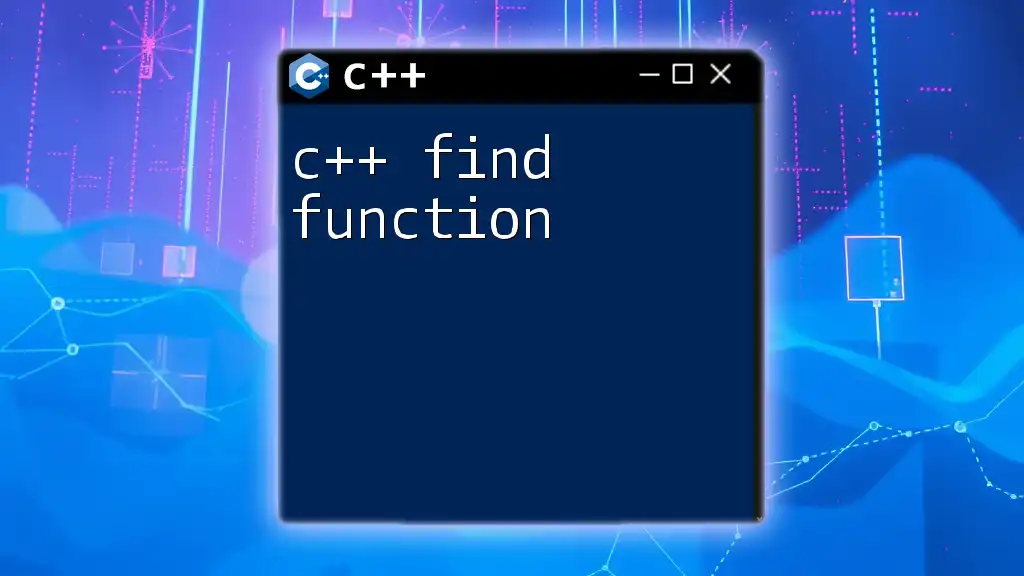
Understanding the C++ GCD Function
The C++ GCD function provides a straightforward way to calculate the GCD of two numbers. It helps simplify problems related to fractions and can optimize several algorithms by reducing the size of the input numbers. There are different methods to calculate the GCD, and understanding these methods can enhance your problem-solving skills in C++ programming.
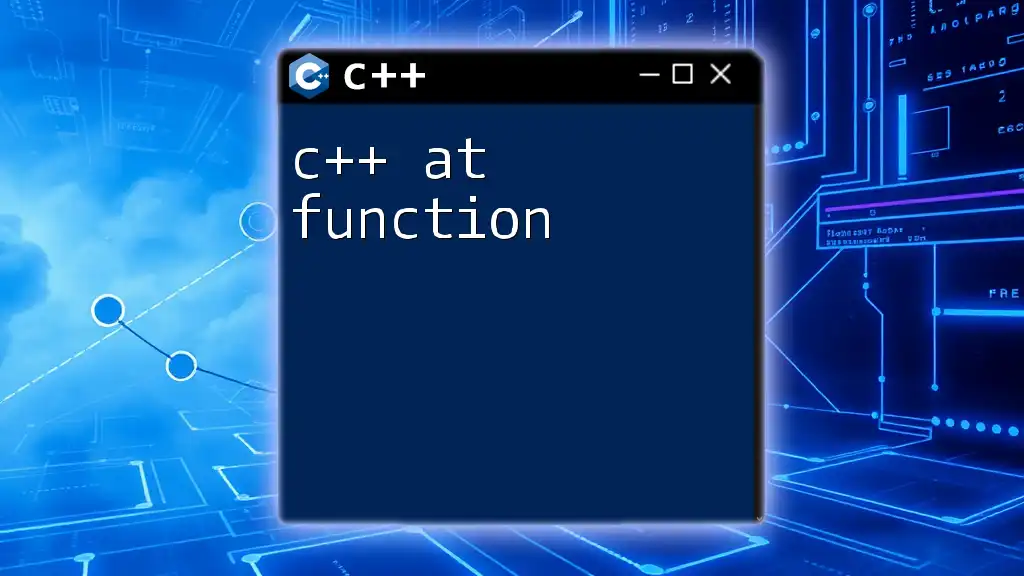
Methods to Calculate GCD in C++
Euclidean Algorithm
The Euclidean Algorithm is one of the oldest methods to compute the GCD of two integers. This algorithm is based on the principle that the GCD of two numbers also divides their difference. It can be summarized in the following steps:
- Given two non-negative integers a and b, where a ≥ b, compute the remainder of a divided by b.
- Replace a with b and b with the remainder.
- Repeat this process until b becomes zero. At that point, a will contain the GCD.
Code Snippet: GCD using the Euclidean Algorithm
#include <iostream>
int gcd(int a, int b) {
while (b != 0) {
int t = b;
b = a % b;
a = t;
}
return a;
}
int main() {
int num1 = 56, num2 = 98;
std::cout << "GCD of " << num1 << " and " << num2 << " is " << gcd(num1, num2) << std::endl;
return 0;
}
In this code snippet, the `gcd` function utilizes a while loop to continually compute the remainder until `b` reaches zero. The final value of `a` is the GCD.
Recursive GCD Calculation
Recursion can also be employed to compute the GCD. In this approach, the function calls itself with updated parameters until the base condition is met.
Advantages and Disadvantages of Recursion
Advantages:
- The recursive solution is often shorter and can be easier to understand due to its mathematical simplicity.
Disadvantages:
- It may result in stack overflow errors for large inputs because of deep recursion levels.
- Performance can be slightly lower compared to iterative solutions due to the overhead of multiple function calls.
Code Snippet: Recursive GCD Implementation
#include <iostream>
int gcd(int a, int b) {
if (b == 0)
return a;
return gcd(b, a % b);
}
int main() {
int num1 = 56, num2 = 98;
std::cout << "GCD of " << num1 << " and " << num2 << " is " << gcd(num1, num2) << std::endl;
return 0;
}
This recursive implementation of the GCD function emphasizes the principle that if b is zero, a is the GCD. If not, the function calls itself with the new set of values.
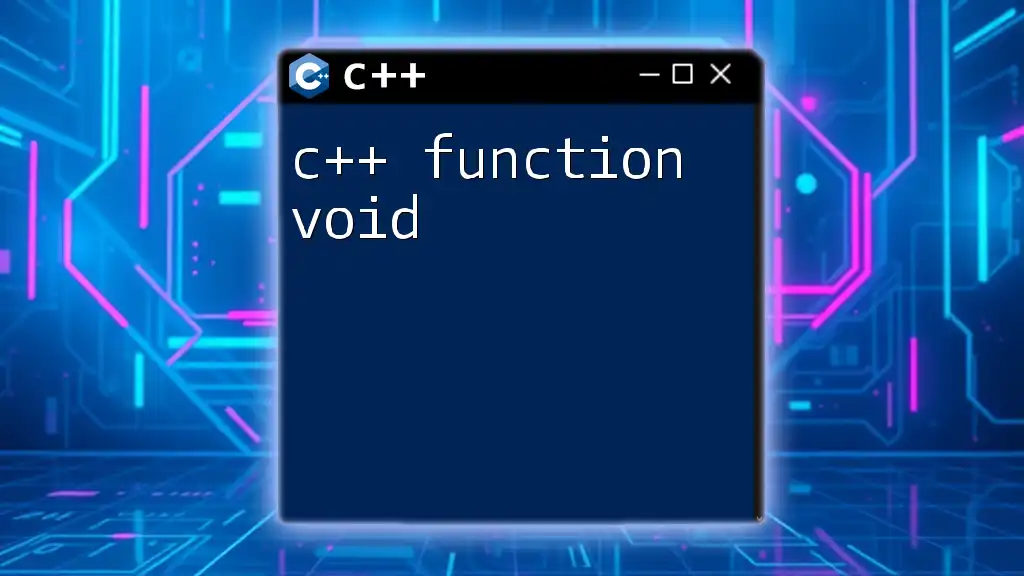
Advanced GCD Concepts
Using C++ Standard Library
Starting from C++17, the Standard Library introduced the `std::gcd` function in the `<numeric>` header. This function simplifies GCD calculations, allowing developers to leverage the robustness and efficiency of the standard library.
Code Snippet: Using std::gcd
#include <iostream>
#include <numeric> // For std::gcd
int main() {
int num1 = 56, num2 = 98;
std::cout << "GCD of " << num1 << " and " << num2 << " is " << std::gcd(num1, num2) << std::endl;
return 0;
}
The `std::gcd` function provides a built-in way to compute the GCD, enhancing code readability and ensuring optimal performance as it is typically implemented in a highly efficient manner.
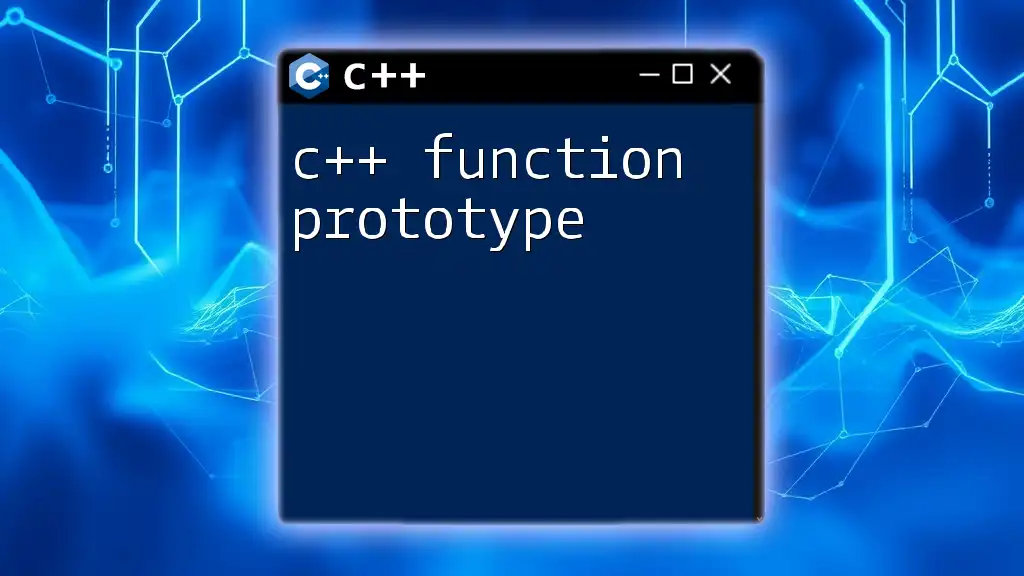
Performance Considerations
Efficiency of GCD Algorithms
The efficiency of GCD computation can vary based on the method used. The Euclidean algorithm is particularly noted for its efficiency, operating in logarithmic time. In contrast, naive methods may involve more significant computations and take longer.
When to Use Which Method
-
Iterative Approach: It is typically preferred for larger inputs to avoid the stack overflow problems associated with deep recursion.
-
Recursive Approach: Useful for smaller numbers or when code brevity and readability are prioritized.
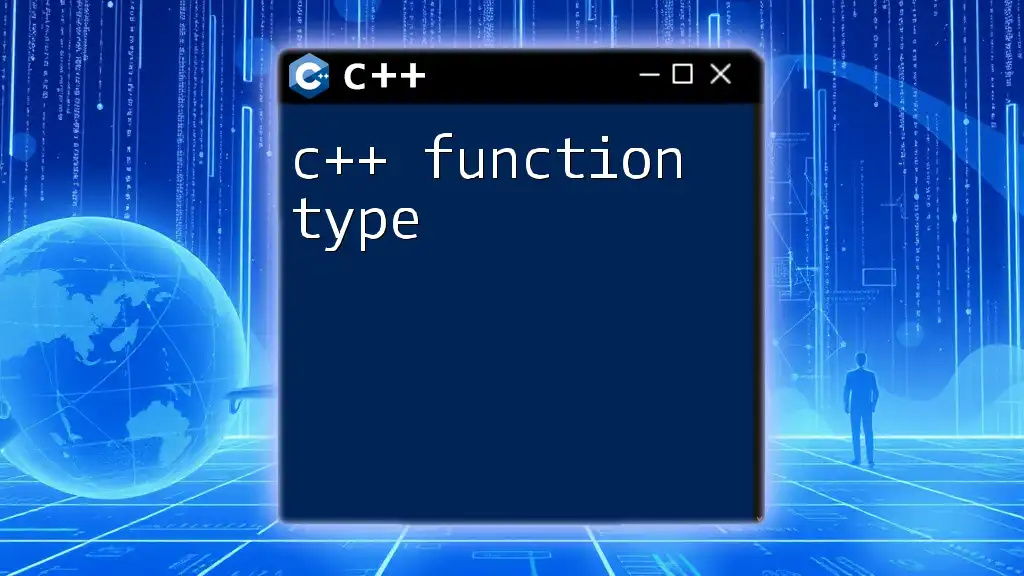
Practical Examples and Scenarios
Finding GCD of Multiple Numbers
When calculating the GCD of more than two numbers, the approach generally involves iteratively applying the GCD function to pairs of numbers. This allows for the reduction of the problem to a simpler form.
Code Snippet: GCD of Multiple Numbers
#include <iostream>
#include <numeric>
#include <vector>
int gcdMultiple(const std::vector<int>& numbers) {
int result = numbers[0];
for (size_t i = 1; i < numbers.size(); i++) {
result = std::gcd(result, numbers[i]);
}
return result;
}
int main() {
std::vector<int> nums = {56, 98, 42};
std::cout << "GCD of array is " << gcdMultiple(nums) << std::endl;
return 0;
}
In this example, the `gcdMultiple` function calculates the GCD of an array of numbers by using `std::gcd` iteratively. This approach highlights the flexibility and efficiency of the C++ GCD function when working with more complex data structures.
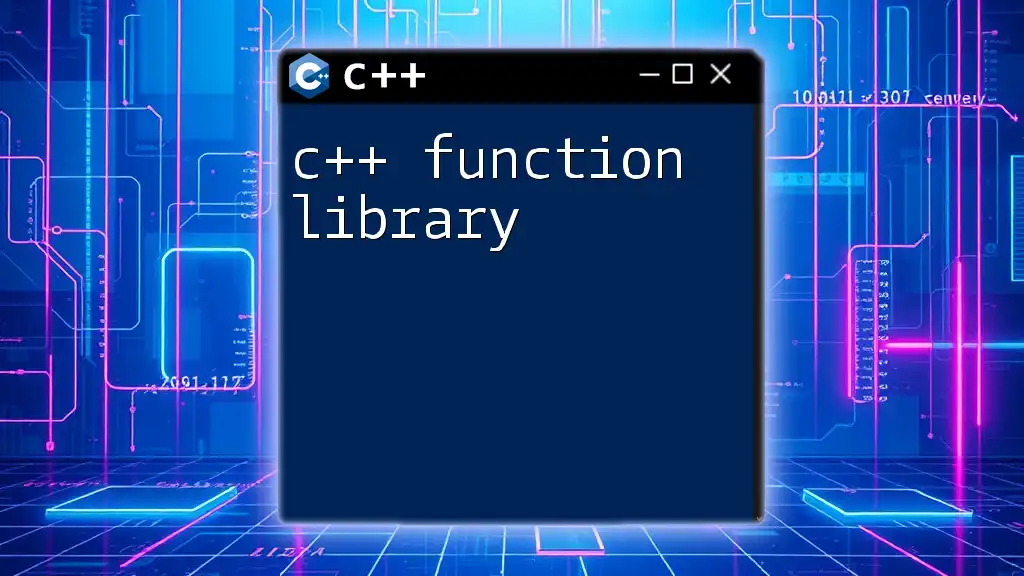
Summary of Key Points
The C++ GCD function is a powerful tool for efficiently computing the greatest common divisor of two or more integers. Through different methods, such as the Euclidean Algorithm and recursive methods, developers can choose the best approach based on their needs. The introduction of the `std::gcd` in C++17 further simplifies the process, promoting efficient and readable code.
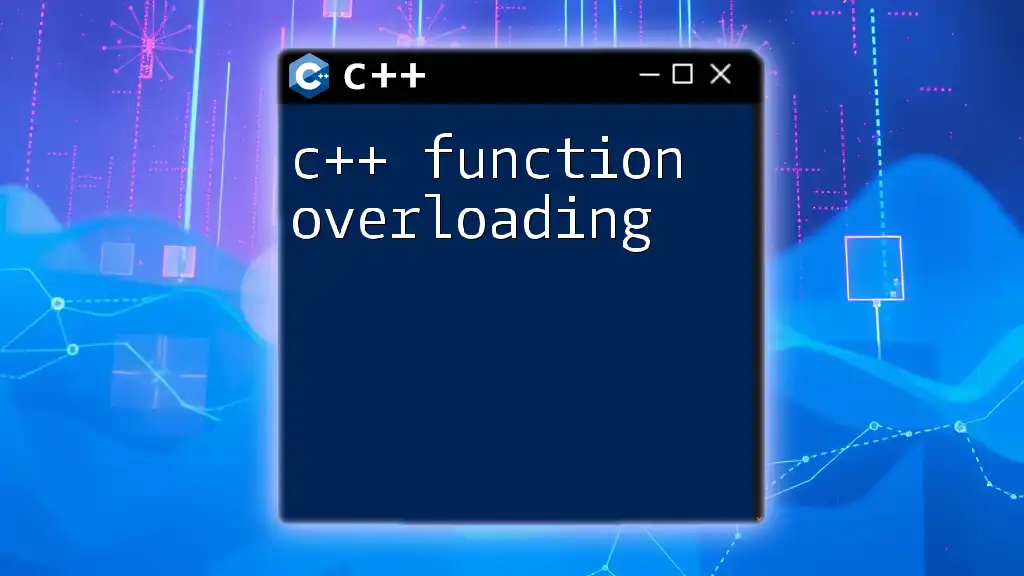
Encouraging Further Learning
To deepen your understanding of algorithms and number theory, consider exploring more advanced topics such as the Chinese Remainder Theorem or Extended Euclidean Algorithm. Experimenting with different GCD algorithms and scenarios can also enhance your problem-solving capabilities in C++.