C++ can effectively interact with SQLite databases using the SQLite C/C++ interface, allowing for operations such as creating, querying, and managing data.
Here's a simple example of how to open a SQLite database and create a table in C++:
#include <sqlite3.h>
#include <iostream>
int main() {
sqlite3 *db;
char *errMsg = 0;
// Open the database
if (sqlite3_open("example.db", &db)) {
std::cerr << "Can't open database: " << sqlite3_errmsg(db) << std::endl;
return 1;
}
// Create SQL statement
const char *sql = "CREATE TABLE IF NOT EXISTS USERS (ID INT PRIMARY KEY NOT NULL, NAME TEXT NOT NULL);";
// Execute SQL statement
if (sqlite3_exec(db, sql, 0, 0, &errMsg) != SQLITE_OK) {
std::cerr << "SQL error: " << errMsg << std::endl;
sqlite3_free(errMsg);
} else {
std::cout << "Table created successfully!" << std::endl;
}
// Close the database
sqlite3_close(db);
return 0;
}
What is SQLite?
SQLite is a lightweight, serverless, and self-contained SQL database engine, making it a highly versatile choice for applications that require a data storage solution without the overhead of a full database server. Its compact size and ease of integration allow it to be used across various platforms and programming languages, including C++. Some key features of SQLite include:
- Cross-platform compatibility—works on virtually any operating system.
- Zero-configuration—no setup or administration is required.
- High-performance for small to medium workloads.
- Atomic transactions—supports transactions and provides ACID compliance.
By leveraging SQLite in your C++ applications, you can achieve efficient data management with minimal complexity.
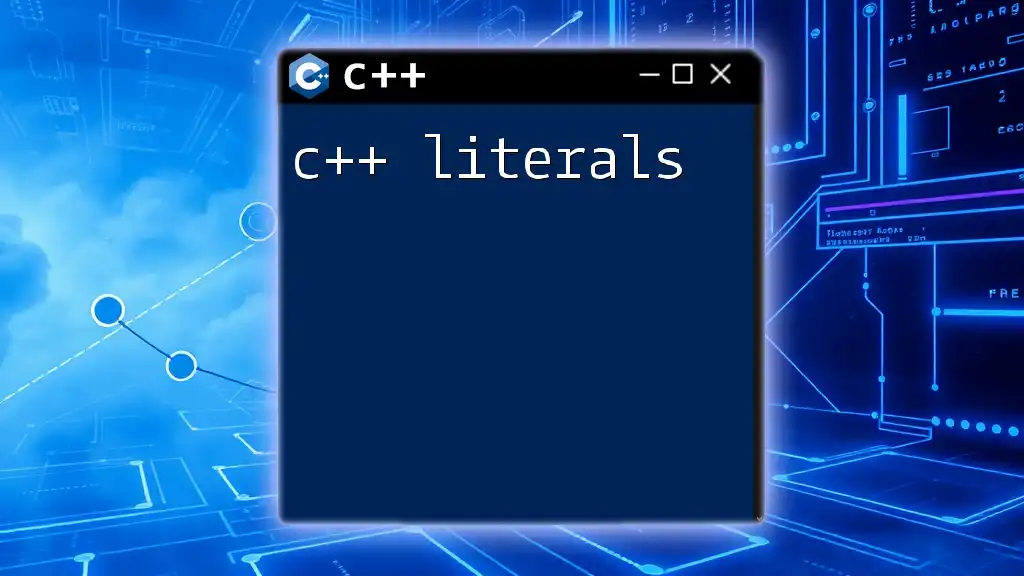
Why Use SQLite with C++?
Integrating SQLite with C++ offers several advantages:
- Lightweight: SQLite minimizes resource usage, making it ideal for embedded systems or applications where memory and storage are constrained.
- Ease of Use: Creating, managing, and querying databases in SQLite is straightforward and can often be accomplished with just a few lines of code.
- Integrated Functionality: Since SQLite is a complete database engine, it includes all necessary functionalities (like transactions, indexing, etc.) without needing external dependencies.
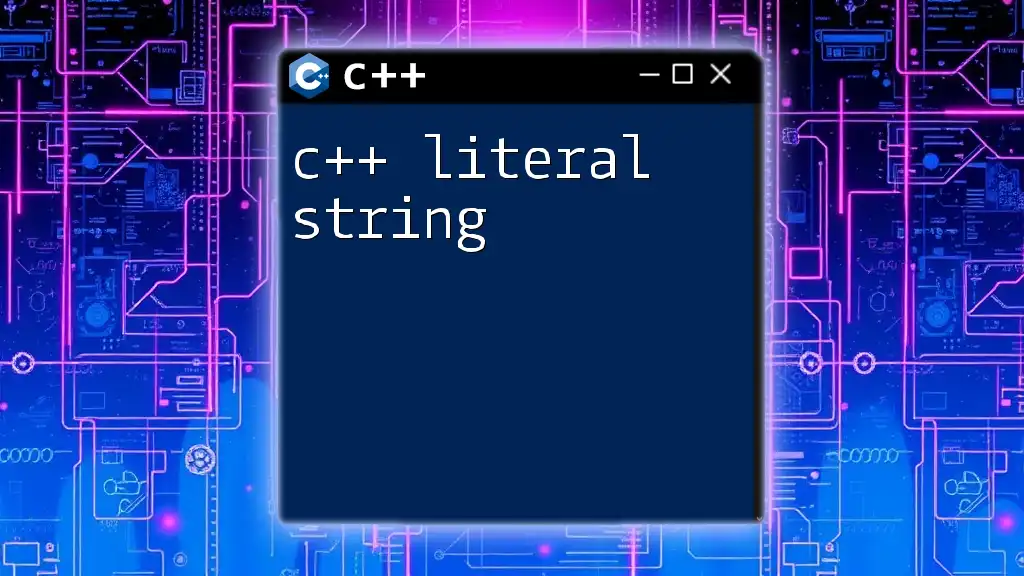
Setting Up SQLite in a C++ Environment
Installing SQLite
To get started, you need to install SQLite. This process typically involves downloading the appropriate binaries or source code from the [SQLite official website](https://www.sqlite.org/download.html). For Windows users, precompiled DLLs are available, while Linux distributions usually provide packages through their package managers.
Setting Up Your C++ Project
Once SQLite is installed, you need to set up your project:
- Create a new C++ project in your preferred development environment (e.g., Visual Studio, Code::Blocks).
- Add the SQLite library to your project dependencies. This might involve including the SQLite header file and linking against the SQLite library in your project settings.
- Include the SQLite header in your C++ source files to enable database functionalities.
#include <sqlite3.h>
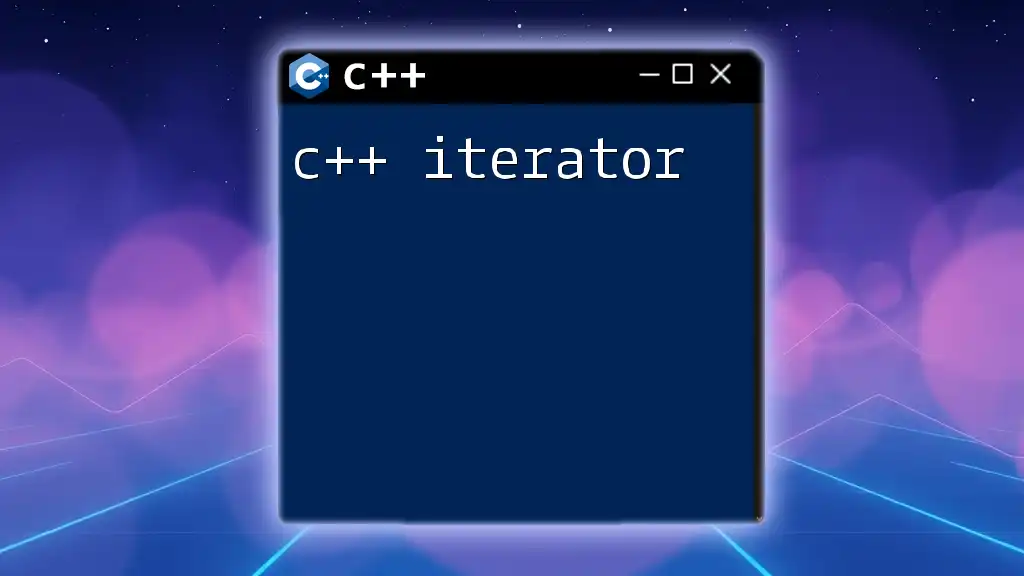
Getting Started with SQLite in C++
Creating a Database
To create a new SQLite database in C++, you can use the `sqlite3_open` function. Here’s an example of how to do this:
sqlite3 *db;
int exit = sqlite3_open("example.db", &db);
if (exit) {
std::cerr << "Error open DB: " << sqlite3_errmsg(db) << std::endl;
} else {
std::cout << "Opened Database Successfully!" << std::endl;
}
In this snippet, we attempt to open a database named `example.db`. If the database does not exist, it will be created automatically.
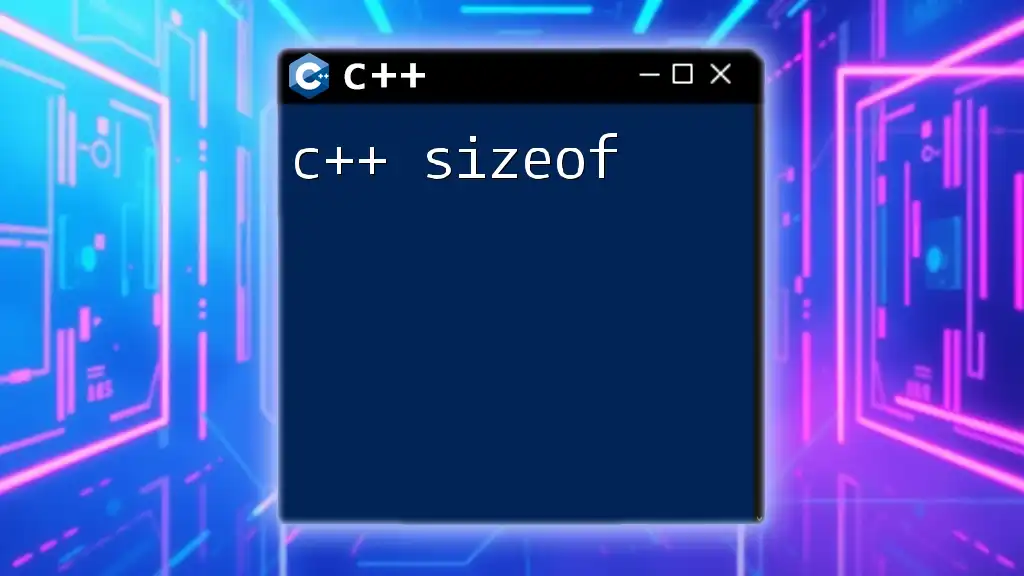
Executing SQL Commands in C++
SQLite allows you to execute SQL commands through your C++ code, enabling you to perform CRUD (Create, Read, Update, Delete) operations efficiently.
Creating Tables
To create a table within your database, you will need to define the SQL command. Here’s an example:
const char* sql = "CREATE TABLE IF NOT EXISTS USERS("
"ID INT PRIMARY KEY NOT NULL, "
"NAME TEXT NOT NULL);";
You can execute this SQL command using `sqlite3_exec`:
char *errMsg;
if (sqlite3_exec(db, sql, nullptr, 0, &errMsg) != SQLITE_OK) {
std::cerr << "SQL error: " << errMsg << std::endl;
sqlite3_free(errMsg);
} else {
std::cout << "Table created successfully!" << std::endl;
}
Inserting Data
To insert data into your newly created table, you can use an SQL insert statement like this:
const char* sqlInsert = "INSERT INTO USERS (ID, NAME) VALUES (1, 'John Doe');";
Executing this command is also done through `sqlite3_exec`:
if (sqlite3_exec(db, sqlInsert, nullptr, 0, &errMsg) != SQLITE_OK) {
std::cerr << "SQL error: " << errMsg << std::endl;
sqlite3_free(errMsg);
} else {
std::cout << "Data inserted successfully!" << std::endl;
}
Reading Data
Reading data from a SQLite database involves using SELECT statements. Here’s how you can implement it in your C++ application:
const char* sqlSelect = "SELECT * FROM USERS;";
sqlite3_stmt *stmt;
if (sqlite3_prepare_v2(db, sqlSelect, -1, &stmt, nullptr) != SQLITE_OK) {
std::cerr << "Failed to prepare statement: " << sqlite3_errmsg(db) << std::endl;
return;
}
You'll need to loop through the results after executing the prepared statement:
while (sqlite3_step(stmt) == SQLITE_ROW) {
int id = sqlite3_column_int(stmt, 0);
const unsigned char* name = sqlite3_column_text(stmt, 1);
std::cout << "ID: " << id << ", Name: " << name << std::endl;
}
sqlite3_finalize(stmt);
Updating Data
Updating existing records can be done via the UPDATE statement. For instance:
const char* sqlUpdate = "UPDATE USERS SET NAME = 'Jane Doe' WHERE ID = 1;";
Similarly, you can execute this command using `sqlite3_exec` like before.
Deleting Data
To remove a record from your database, use the DELETE statement:
const char* sqlDelete = "DELETE FROM USERS WHERE ID = 1;";
if (sqlite3_exec(db, sqlDelete, nullptr, 0, &errMsg) != SQLITE_OK) {
std::cerr << "SQL error: " << errMsg << std::endl;
sqlite3_free(errMsg);
} else {
std::cout << "Data deleted successfully!" << std::endl;
}
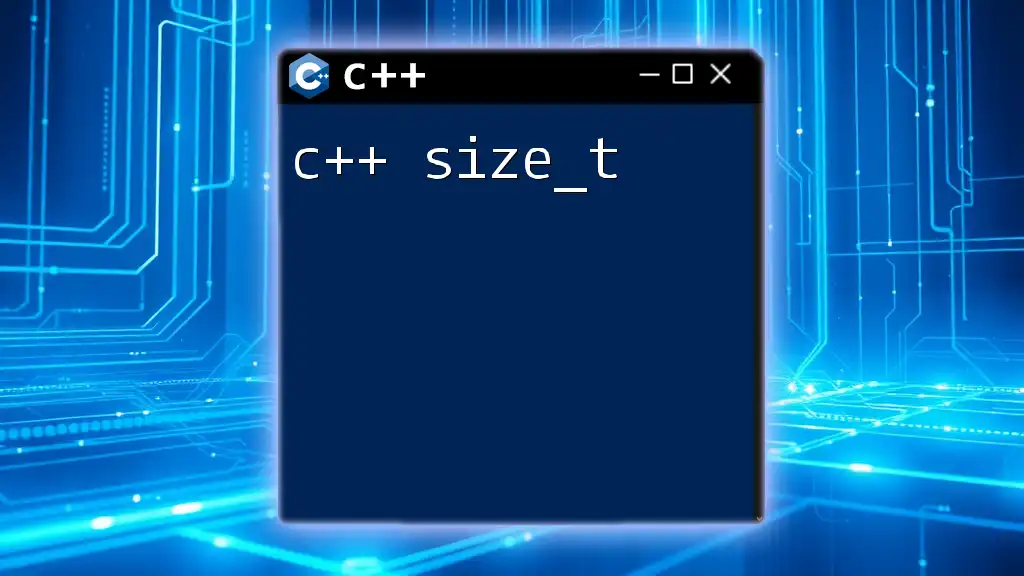
Handling Errors and Exceptions
Error handling is crucial in any database interaction. SQLite provides error codes to indicate what went wrong during the execution of statements. Always check the return code of your SQLite functions and report errors effectively:
if (sqlite3_exec(db, sql, nullptr, 0, &errMsg) != SQLITE_OK) {
std::cerr << "SQL error: " << errMsg << std::endl;
sqlite3_free(errMsg);
}
By checking for errors, you can take corrective actions or log issues for investigation later.
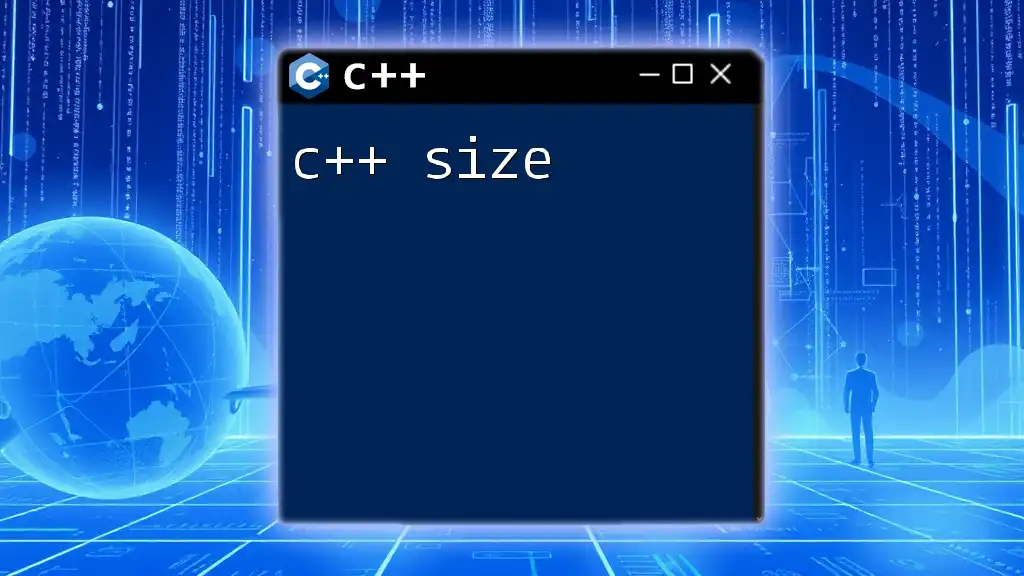
Advanced SQLite Features in C++
Transactions in SQLite
Transactions allow you to perform multiple database operations as a single unit. They ensure that either all operations succeed or none do, maintaining data integrity. In SQLite, you can start a transaction like this:
sqlite3_exec(db, "BEGIN TRANSACTION;", nullptr, 0, &errMsg);
// Perform multiple insertions or updates here
sqlite3_exec(db, "COMMIT;", nullptr, 0, &errMsg);
Prepared Statements
Using prepared statements can significantly enhance performance, especially when executing the same statement multiple times with different parameters. Here’s how to use a prepared statement to insert data:
sqlite3_stmt *stmt;
const char* sqlInsert = "INSERT INTO USERS (ID, NAME) VALUES (?, ?);";
sqlite3_prepare_v2(db, sqlInsert, -1, &stmt, nullptr);
sqlite3_bind_int(stmt, 1, 1); // Bind first parameter
sqlite3_bind_text(stmt, 2, "John Doe", -1, SQLITE_STATIC); // Bind second parameter
sqlite3_step(stmt); // Execute the prepared statement
sqlite3_finalize(stmt); // Clean up
Using prepared statements can also help prevent SQL injection attacks by separating SQL code from data.
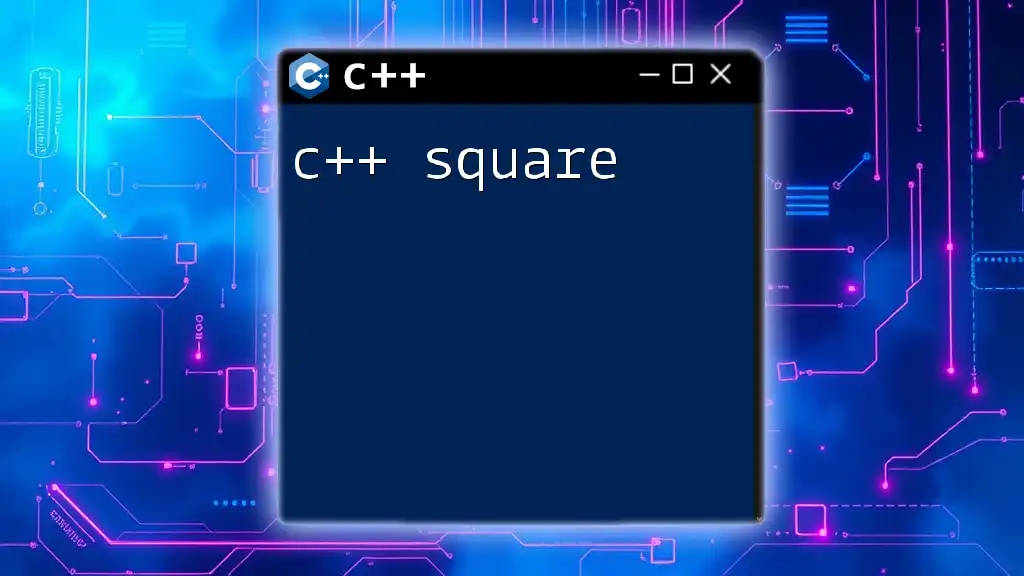
Conclusion
In this comprehensive guide on C++ with SQLite, we've explored the basics and advanced features of integrating SQLite into your C++ applications. Key topics include creating and managing databases, executing SQL commands, handling errors, and leveraging advanced features such as transactions and prepared statements.
As you start using SQLite with C++, experiment with various functionalities and try building small projects to solidify your understanding. The simplicity and efficiency of SQLite makes it a valuable tool for any developer looking to manage data effectively.
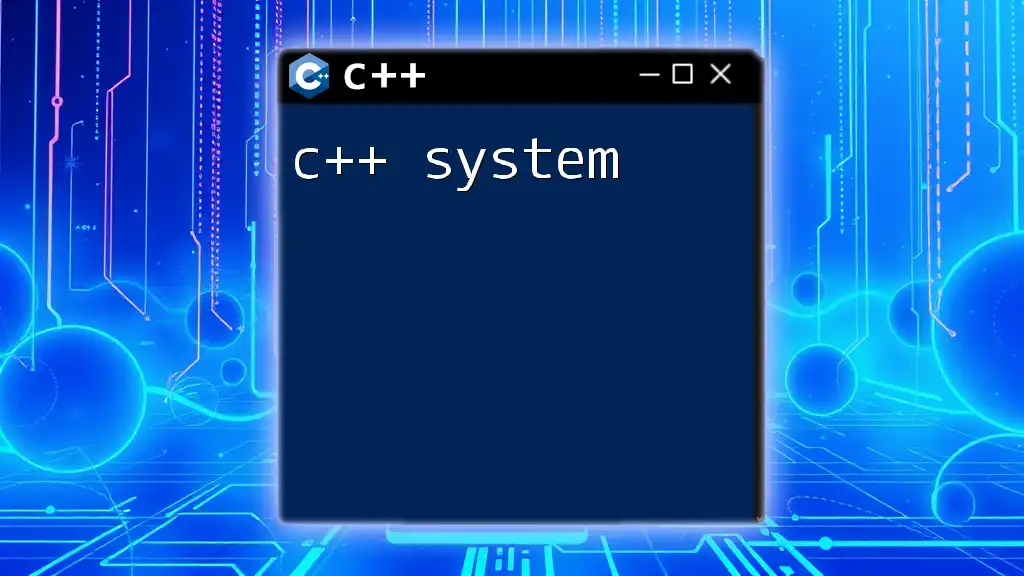
Additional Resources
For further reading and exploration into C++ and SQLite integration, consider visiting:
- The official [SQLite documentation](https://www.sqlite.org/docs.html).
- C++ forums and community groups where you can ask questions and share experiences with other developers.
With this foundation, you're well-equipped to harness the power of SQLite within your C++ projects!