C++ slicing occurs when a derived class object is assigned to a base class object, leading to the loss of data unique to the derived class.
Here's a code snippet demonstrating C++ slicing:
#include <iostream>
class Base {
public:
void display() { std::cout << "Base class\n"; }
};
class Derived : public Base {
public:
void display() { std::cout << "Derived class\n"; }
};
int main() {
Derived d;
Base b = d; // Slicing occurs here
b.display(); // Outputs: Base class
return 0;
}
Understanding C++ Slicing
What is C++ Slicing?
C++ slicing refers to a phenomenon in object-oriented programming where an object of a derived class is assigned to an object of a base class. This operation effectively "slices off" the specific attributes and behaviors defined in the derived class, resulting in the loss of important information.
When you pass a derived class object by value to a function that takes a base class object, the derived part of the object is not preserved. This can lead to unintended behavior in your programs if you're not aware of how slicing works.
Why Slicing Happens
C++ slicing primarily occurs due to the way objects are treated when they are passed around in the program, especially in the context of inheritance. Inheritance allows a derived class to extend the functionalities of a base class. However, when an object of a derived class is treated as an instance of a base class, only the base class portion of that object is retained, while the derived class component is lost, leading to slicing.
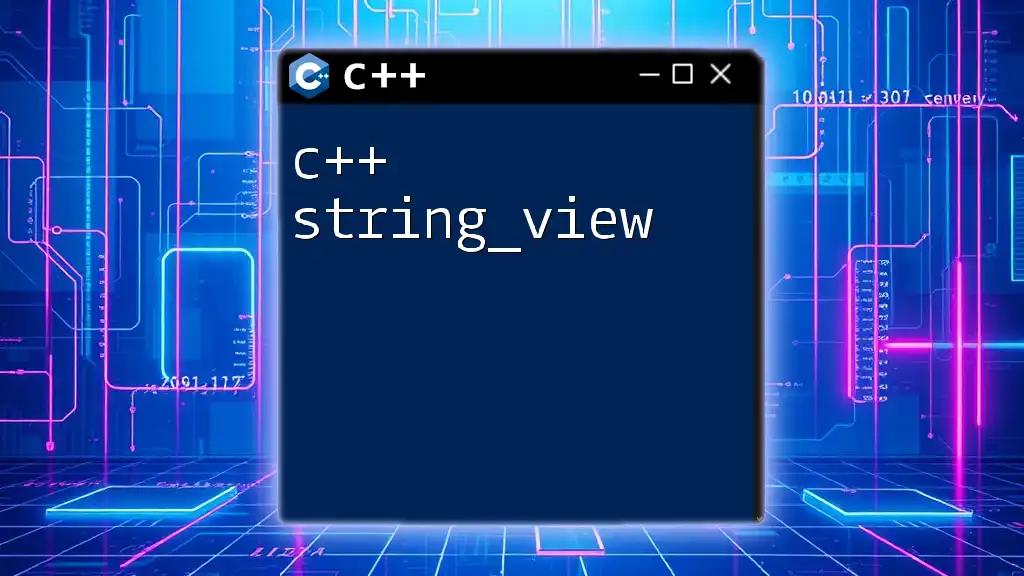
Demonstrating C++ Slicing
Example of C++ Slicing
Let's say we have a simple class hierarchy with a base class `Base` and a derived class `Derived`.
class Base {
public:
void display() { std::cout << "Base class" << std::endl; }
};
class Derived : public Base {
public:
void display() { std::cout << "Derived class" << std::endl; }
};
void function(Base b) {
b.display();
}
int main() {
Derived d;
function(d); // Slicing occurs here
return 0;
}
In this code, when we call `function(d)`, we are passing an object of type `Derived` to a function that accepts a `Base` object by value. Here, slicing occurs, and only the `Base` part of the `Derived` object is passed to the function. When `b.display()` is called, the output will be:
Base class
Analyzing the Example
The output indicates that although we created an instance of `Derived`, the function only has access to the `Base` part of the object. This results in the derived class's behavior being lost in the process. The implications of slicing can lead to bugs and unintended behavior in larger applications, as the derived functions or data members that exist in the `Derived` class cannot be accessed.

Mechanisms of Slicing
How Slicing Affects Object Behavior
When an object is sliced, only the attributes and methods defined in the base class remain accessible. The `Derived` class's specific implementation, which might override base class methods or contain additional members, is not present anymore. This means polymorphism—a core feature in object-oriented programming—fails to work as expected in cases of slicing.
Solutions to Prevent C++ Slicing
To prevent slicing, it's crucial to use pointers or references rather than passing objects by value. Here are two effective methods to do so:
Using Pointers
Instead of passing by value, you can pass the object by pointer:
void function(Base* b) {
b->display();
}
This way, the function retains access to the entire object, including any derived behavior.
Using References
Similarly, you can utilize references:
void function(Base& b) {
b.display();
}
This technique also ensures the integrity of the derived object, preventing slicing while maintaining the polymorphic behavior.

Best Practices to Avoid Slicing in C++
Designing Class Hierarchies
When designing class hierarchies, it's essential to consider how objects will be passed around—especially when those objects are instances of derived classes. Using abstract classes and interfaces can ensure that derived classes are treated correctly and that their unique behaviors aren't sliced away. Always preferring pointers or references in function parameters helps maintain the full object.
Utilizing Smart Pointers
Smart pointers like `std::shared_ptr` handle object lifetime automatically while ensuring that slicing doesn’t occur:
#include <memory>
void function(std::shared_ptr<Base> b) {
b->display();
}
This approach not only prevents slicing but also helps avert memory leaks by managing the object's lifetime effectively.

Common Mistakes and Misunderstandings
Misunderstanding Polymorphism
A common mistake developers make is conflating static binding with dynamic binding. In C++, when an object is sliced, it doesn't use dynamic binding for method calls because the type of the object passed is the base type, losing the derived type context. Understanding this distinction is key to properly leveraging polymorphism without falling into the slicing trap.
Ignoring Object Lifetimes
Another crucial aspect is recognizing object lifetimes and how they relate to slicing. If objects are not managed properly—say having a local object go out of scope while still referenced elsewhere—you may find yourself exposing uninitialized or dangling references that lead to undefined behavior. Proper use of smart pointers can help mitigate these risks effectively.
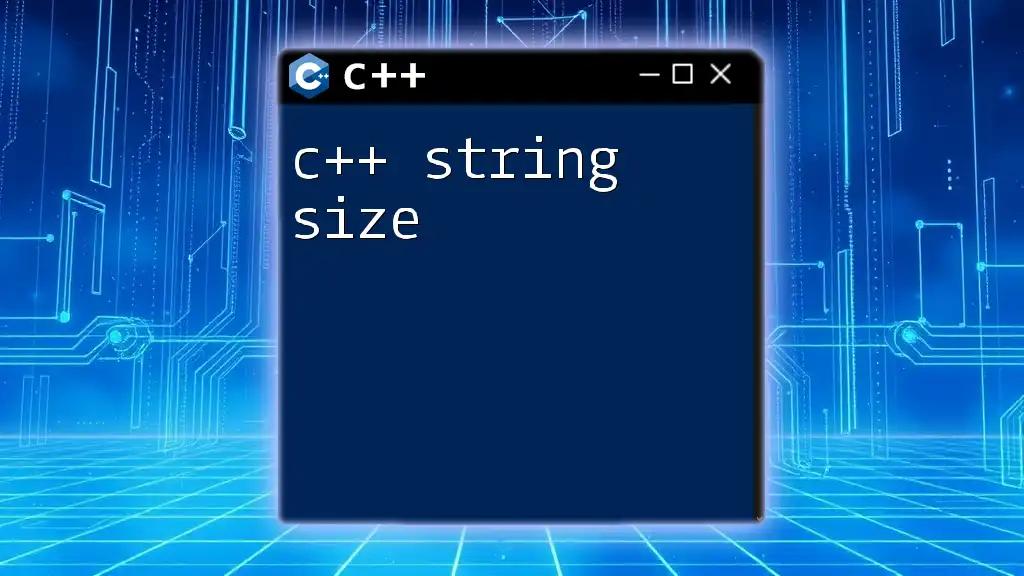
Conclusion
C++ slicing can significantly hinder the capabilities of the object-oriented programming paradigm if not well understood. It is essential to remain cautious when passing class objects, especially in context with inheritance. By adhering to best practices—like utilizing references, pointers, and smart pointers—you can prevent slicing and preserve the integrity of your derived classes.
Understanding C++ slicing not only enhances your coding skills but also empowers you to write more robust and maintainable code. Engage in practice to solidify your grasp of these concepts, and explore further resources to expand your knowledge of C++ and object-oriented programming principles.