A C++ multiset is an associative container that allows multiple occurrences of equivalent elements and maintains their order using a balanced binary tree.
Here’s a simple code snippet demonstrating how to declare and use a multiset in C++:
#include <iostream>
#include <set>
int main() {
std::multiset<int> numbers;
numbers.insert(4);
numbers.insert(1);
numbers.insert(4);
numbers.insert(2);
std::cout << "Multiset elements: ";
for (const auto &num : numbers) {
std::cout << num << " ";
}
return 0;
}
What is a Multiset in C++?
A multiset in C++ is a specialized associative container that allows multiple occurrences of the same element. Unlike a standard set, which only stores unique elements, a multiset enables you to store duplicate values. This feature makes it perfect for scenarios where counting occurrences is necessary or where you want to maintain an ordered collection of elements.
Characteristics of multisets include:
- Allow Duplicates: You can insert the same value multiple times.
- Ordered by Value: Elements are automatically sorted, typically in ascending order.
- Efficient Insertions and Removals: Using a balanced binary tree structure, a multiset optimizes insertions and deletions.
When comparing multisets to sets, the key distinction is their treatment of duplicates. In a set, attempting to insert a duplicate simply has no effect, whereas a multiset will increment the count of that element.
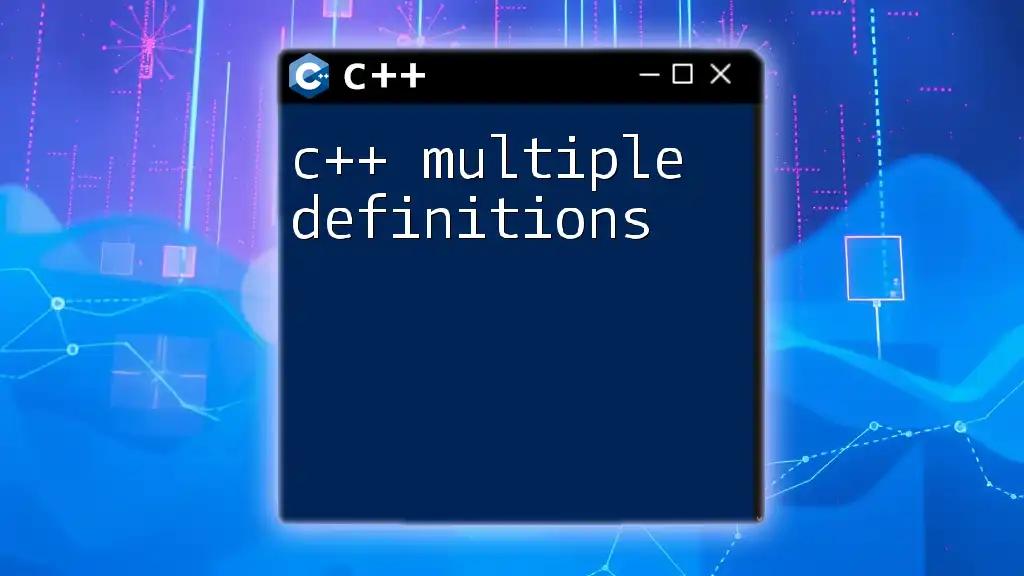
How Multisets Work
Multisets rely on a balanced tree structure, most commonly a Red-Black Tree. This structure ensures:
- Time Complexity: Searching for an element has an average complexity of O(log n), and inserting or deleting elements also retains O(log n) complexity. Such efficiency makes multisets suitable for scenarios requiring frequent insertions and deletions.
Understanding these fundamental aspects sets the stage for effective utilization of multisets in C++ programming.
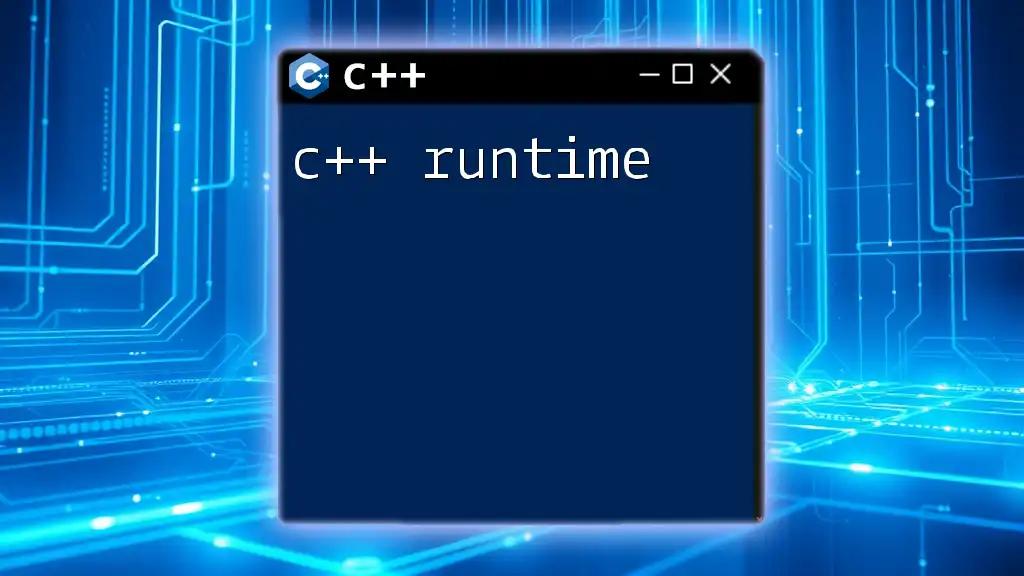
Getting Started with Multiset C++
Including Headers and Basic Syntax
To use multisets in C++, you need to include the header file `<set>`. Here’s how you set up your basic program:
#include <iostream>
#include <set>
int main() {
std::multiset<int> ms;
return 0;
}
Creating a Multiset
You can create a multiset in several ways, including initializing it with specified elements, creating an empty multiset, or copying from another multiset.
Example of Initializing with Elements:
std::multiset<int> ms = {1, 2, 3, 2, 1, 4};
This code initializes a multiset containing integers, with duplicates.
Adding Elements to a Multiset
To add elements to a multiset, you can use:
- `insert()`: This method adds elements and allows duplicates.
- `emplace()`: This function constructs the element in place, which can be more efficient.
Here’s a code snippet demonstrating these methods:
ms.insert(5);
ms.emplace(6);
Both commands add the specified values to the multiset without any issues, even if duplicates exist.
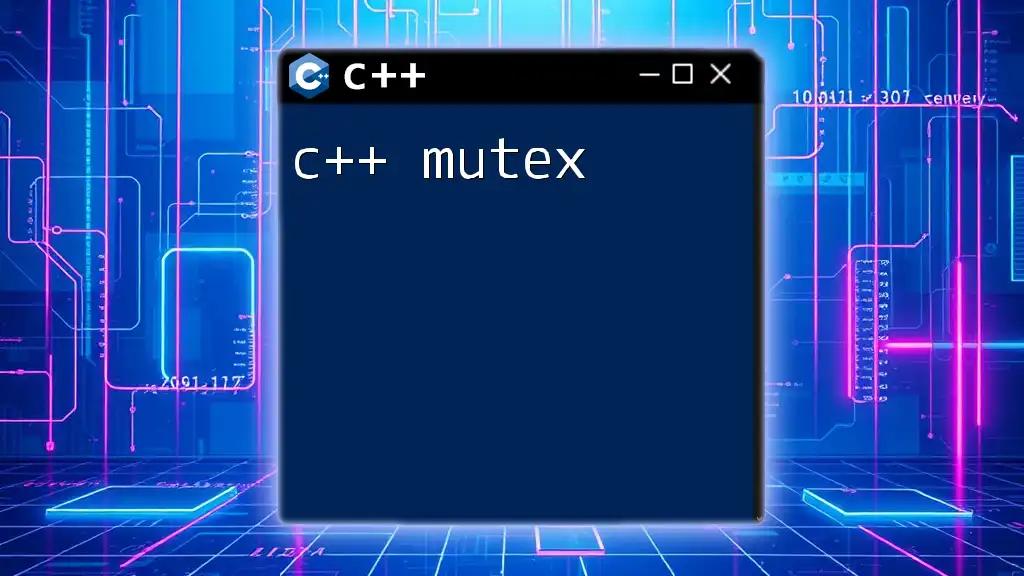
Operations on Multisets
Accessing and Finding Elements
Multisets provide easy access through iterators. You can use iterators to loop through each element, reading them one by one.
Here’s an example that demonstrates how to iterate through a multiset:
for (auto it = ms.begin(); it != ms.end(); ++it) {
std::cout << *it << " ";
}
This loop prints out all elements in the multiset in order.
Counting Elements
To determine how many times a specific element exists in the multiset, use the `count()` method:
int count_of_1 = ms.count(1); // Returns 2
This line of code retrieves the number of occurrences of the element '1'.
Removing Elements
You can remove elements from a multiset using:
- `erase()`: Removes one or multiple occurrences of a specified value.
- `clear()`: Deletes all elements, leaving the multiset empty.
Here’s how you might use these methods effectively:
ms.erase(2); // Remove one occurrence of '2'
ms.erase(ms.find(1)); // Remove a single occurrence of '1'
ms.clear(); // Remove all elements
These operations maintain the properties of the multiset while allowing you to manage its contents flexibly.
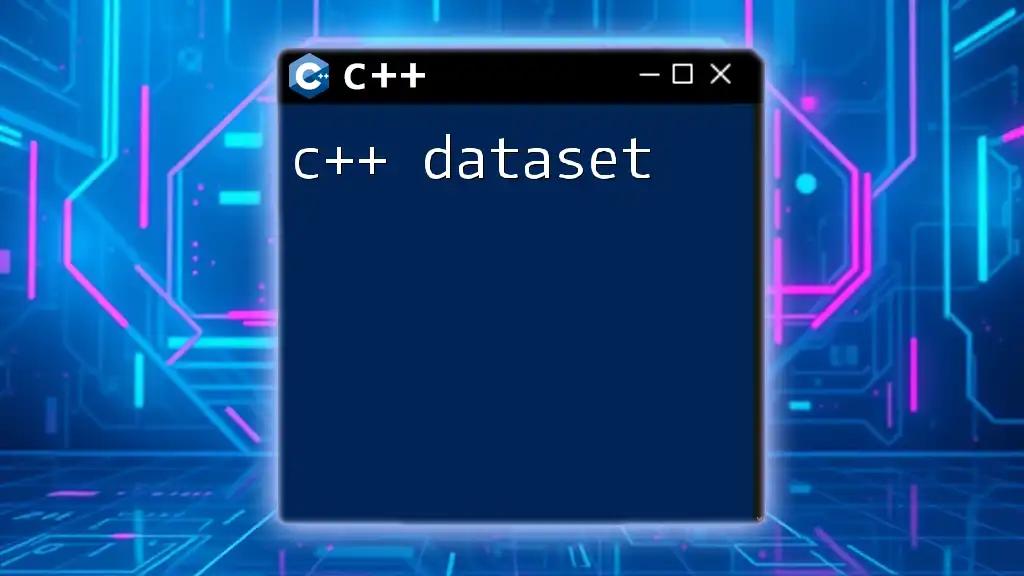
Multiset Algorithms and Custom Comparators
Sorting and Order
The default behavior of a multiset is to automatically sort its elements in ascending order. However, sometimes, you may require a custom sorting order. For this, you can provide a comparator function.
Custom Comparator Example:
struct CustomCompare {
bool operator() (const int& a, const int& b) const {
return a > b; // Descending order
}
};
std::multiset<int, CustomCompare> custom_ms;
By defining a custom comparator, you can alter how the multiset organizes its elements, granting more control over data representation.
Common Use Cases for Multisets
Multisets are particularly useful in scenarios such as counting frequencies of elements, frequency analyses in datasets, or when you require an ordered collection of items that may contain duplicates. Examples might include:
- Managing Histograms: Represent the frequency of different scores or events.
- Merging Sorted Sequences: When merging datasets, multisets can handle duplicates while ensuring a sorted output.
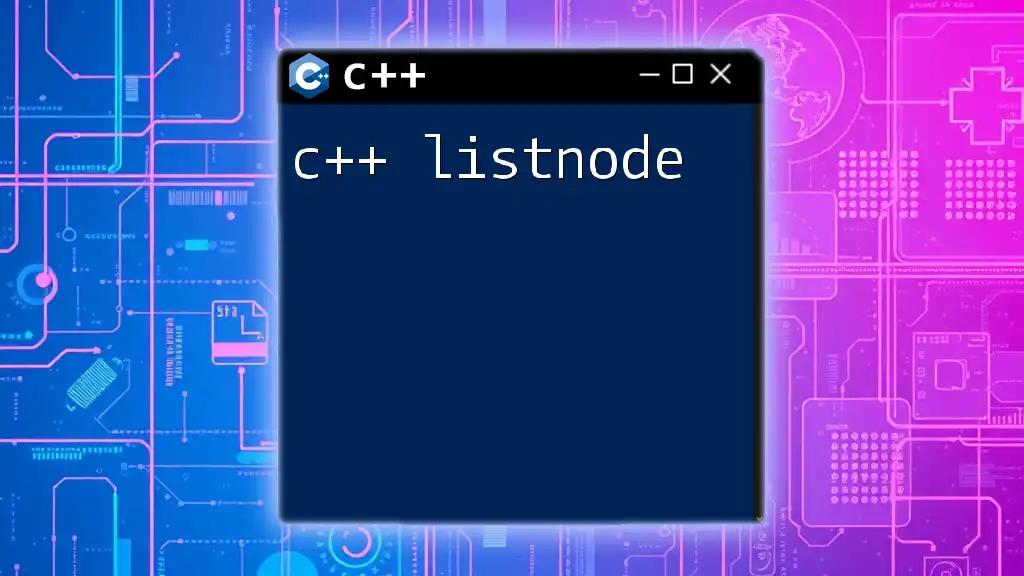
Conclusion
In this guide, we've explored the various aspects of C++ multisets, from their definition and characteristics to their operations and use cases. The power of multisets lies in their flexibility and efficiency when dealing with duplicate elements and maintaining order.
Whether you aim to count occurrences, manage collections of elements, or require sorted datasets without concern for duplicates, the multiset is a highly effective tool in the C++ Standard Library.
For those keen to dive deeper into C++ data structures, numerous resources—such as advanced documentation, tutorials, and books—are available to expand your understanding and application of multisets and related constructs.
FAQs on C++ Multiset
Can I create multisets with types other than integers?
Yes, multisets can store any data type, including user-defined types, as long as a comparison operator is defined for ordering.
What is the difference between `std::set` and `std::multiset`?
The primary difference lies in duplication: a set stores unique elements while a multiset allows duplicates.
How does the performance of a multiset compare to a vector?
When it comes to searching and removing elements, multisets are more efficient due to their underlying tree structure, while vectors might be faster for random access and iteration, though not for search or delete operations.