In C++, `nullptr` is a type-safe null pointer constant that replaces the traditional `NULL` macro, ensuring better type-checking and avoiding ambiguity in pointer assignments.
#include <iostream>
int main() {
int* ptr = nullptr; // Initialize a pointer to nullptr
if (ptr == nullptr) {
std::cout << "Pointer is null!" << std::endl;
}
return 0;
}
What is `nullptr`?
In C++, `nullptr` is a special keyword that represents a null pointer constant. Introduced with C++11, it provides a more type-safe and expressive alternative to previous null pointer representations, such as `NULL` and the literal `0`. The use of `nullptr` eliminates ambiguity and improves code readability, making it clear that a pointer variable intentionally holds no address.
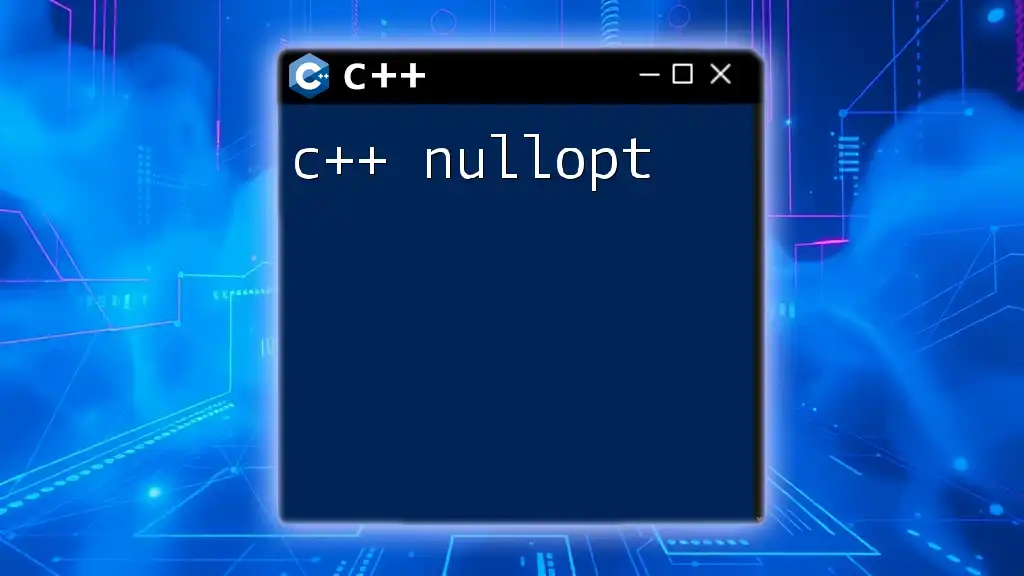
Historical Context
Before the introduction of `nullptr`, programmers primarily used two forms for representing null pointers: `NULL` and `0`.
-
`NULL`: Traditionally defined as zero, `NULL` was simply a macro that often expanded to `0`, but it lacked type safety, leading to potential issues in function overloading and pointer arithmetic.
-
Zero Literal (`0`): Using `0` posed similar concerns, as it could confuse compilers or readers about whether it represented an integer or a null pointer.
With the advent of C++11, `nullptr` replaced these older methods, addressing their shortcomings while improving code clarity.
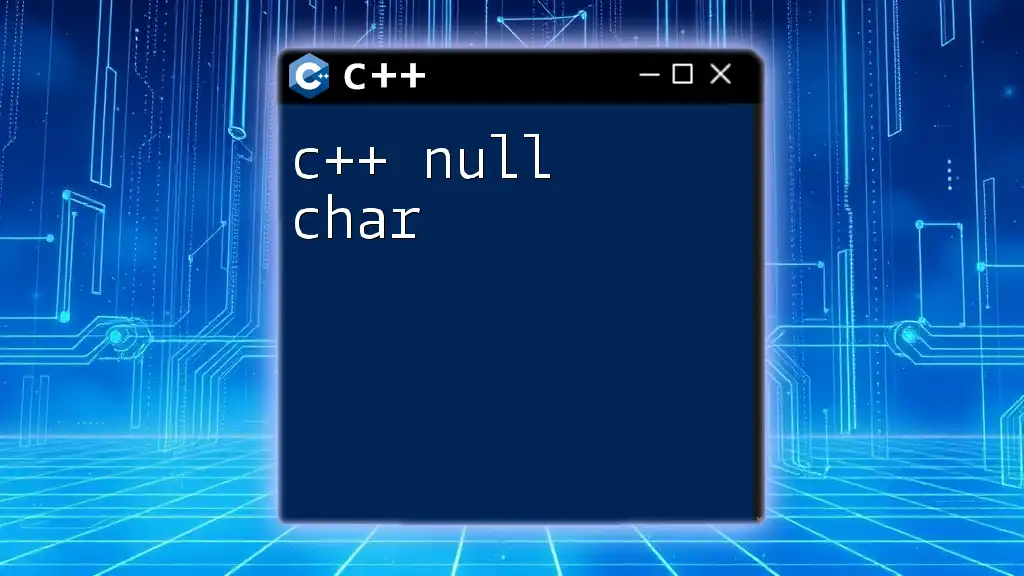
Understanding Pointers in C++
Pointers are a fundamental aspect of C++ that allow programmers to manipulate memory directly. A pointer is a variable that stores the memory address of another variable. However, when a pointer does not point to any valid memory, it should be explicitly marked as null.
The importance of null pointers cannot be overstated; they help avoid dereferencing invalid pointers, which can lead to undefined behavior, application crashes, or memory corruption. Understanding how `nullptr` integrates into C++ programming is crucial for developing robust applications.
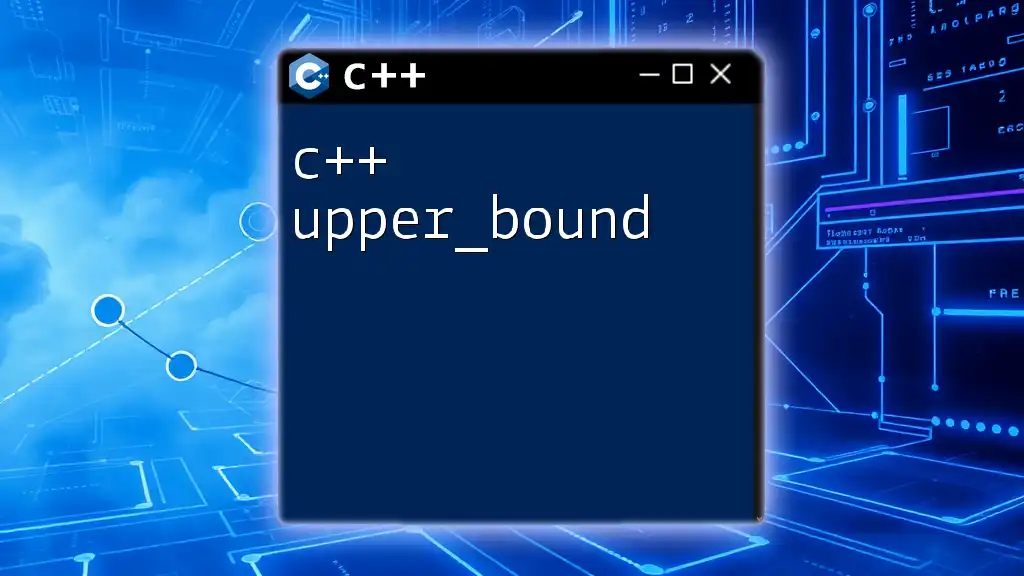
The Syntax of `nullptr`
Declaring and using `nullptr` is straightforward. Here’s how to do it:
int* ptr = nullptr; // Declaring a null pointer
In this example, `ptr` is initialized to `nullptr`, signaling that it does not currently point to any valid memory location. This explicit declaration helps to convey the intended use of the pointer clearly.
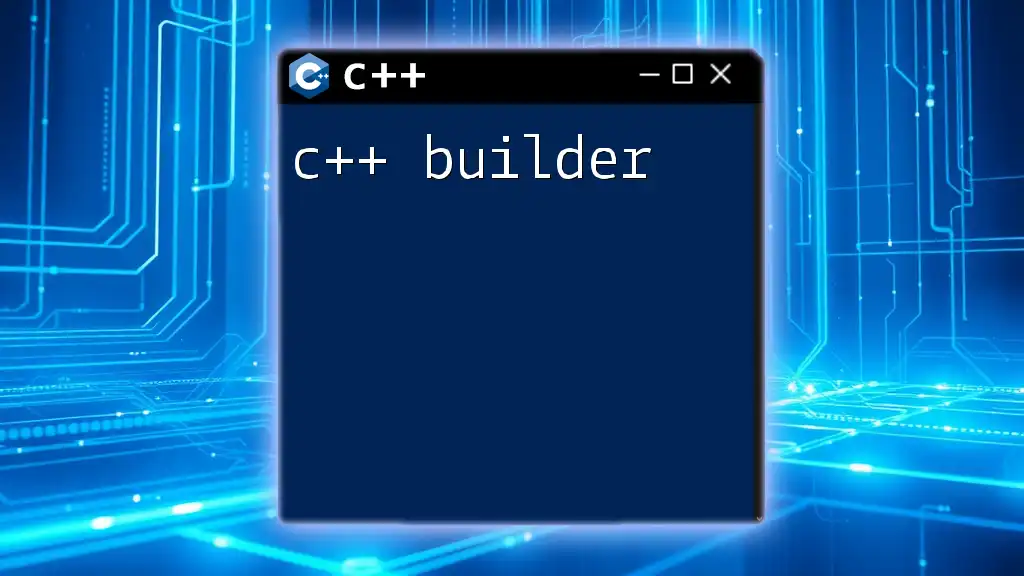
Advantages of Using `nullptr`
Using `nullptr` comes with several advantages:
-
Type Safety Improvements: Unlike `NULL`, which may cause ambiguity between integer types and pointer types, `nullptr` is specifically recognized as a pointer type. This means that you can safely use `nullptr` without worrying about unintended type conversions.
-
Avoiding Ambiguities in Overloaded Functions: When functions are overloaded based on pointer types, using `nullptr` can eliminate confusion. For example:
void process(int* ptr); void process(char* ptr); process(nullptr); // Calls the function with int* type
Here, passing `nullptr` ensures that the compiler handles the call correctly, avoiding potential errors that could arise with `NULL`.
-
Enhanced Code Readability and Maintainability: Code can be easier to read and maintain when you use `nullptr` instead of legacy null representations. Developers can easily understand that a pointer is intentionally null.
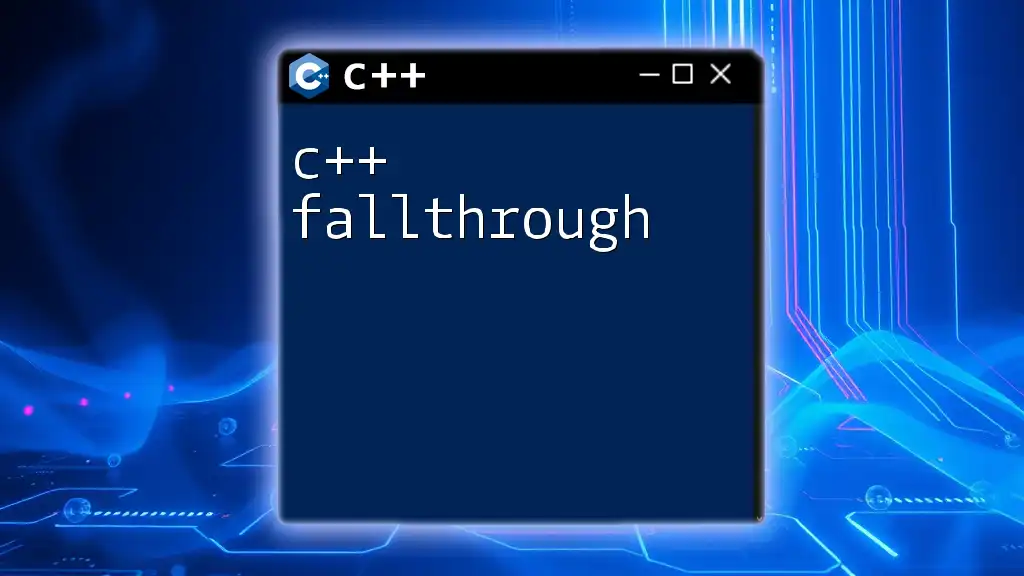
Using `nullptr` in Function Overloading
Function overloading allows for defining multiple functions with the same name but different parameter types. However, using `NULL` or `0` can lead to ambiguity. Using `nullptr` clarifies the function call and makes it type-safe. Here’s an implementation example to illustrate this:
void handleRequest(int* request);
void handleRequest(char* request);
// Using nullptr resolves the ambiguity
handleRequest(nullptr); // Calls handleRequest(int*)
In this case, `nullptr` helps the compiler discern which overloaded function to invoke more effectively.
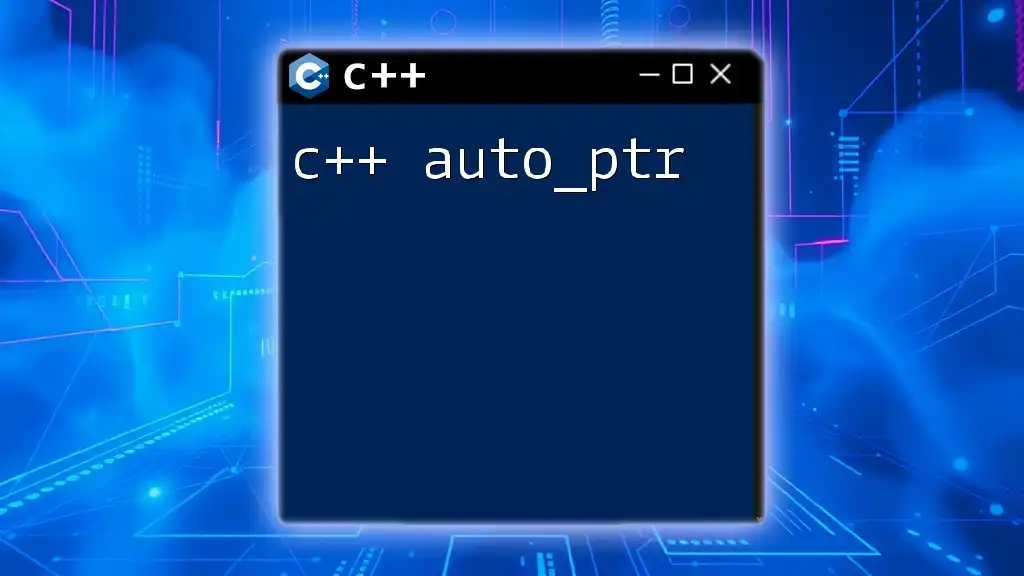
Best Practices for Using `nullptr`
When writing modern C++ code, adhere to the following best practices regarding `nullptr`:
-
Prefer using `nullptr` over `NULL` or `0` for null pointer representation in new code. This practice enhances clarity and decreases the chance of introducing errors.
-
Maintain consistency: Using `nullptr` throughout your codebase can prevent confusion, especially when collaborating with others.
-
Refactor legacy code: If you come across `NULL` or zero literals in existing code, consider updating those to `nullptr` where applicable, to align with modern C++ practices.
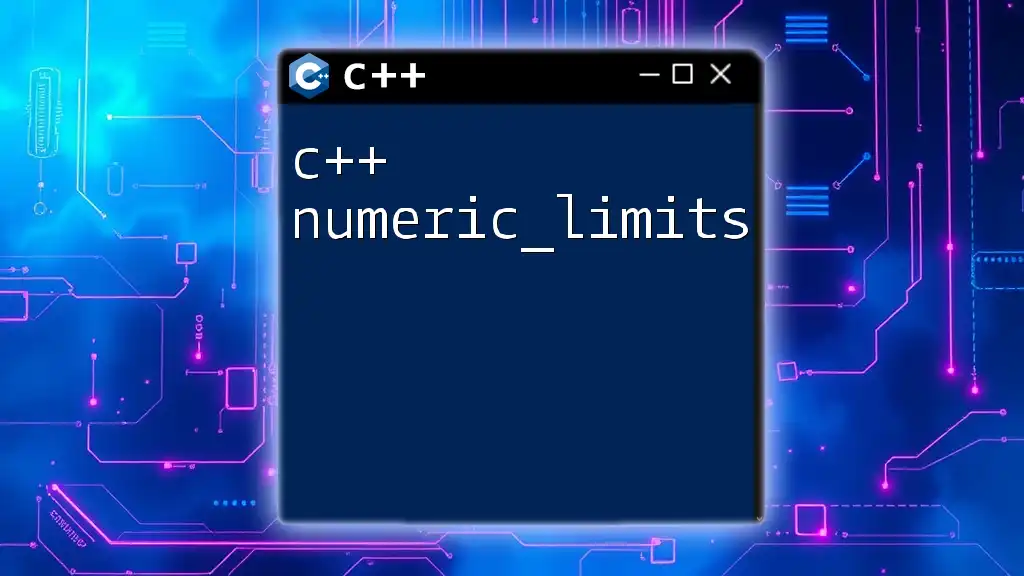
Common Mistakes with `nullptr`
Despite its advantages, some developers may misunderstand `nullptr`. Here are a few common mistakes:
- Misusing `nullptr` in comparisons: It's crucial to compare pointers correctly. Avoid using other null representations like `NULL`. For instance:
int* ptr = nullptr;
// Correct way to check for null
if (ptr == nullptr) {
// logic for handling a null pointer
}
- Assuming `nullptr` can be used interchangeably with integer types: Remember that `nullptr` is specifically intended for pointers. Mistaking it for an integer can lead to confusion and potential errors.
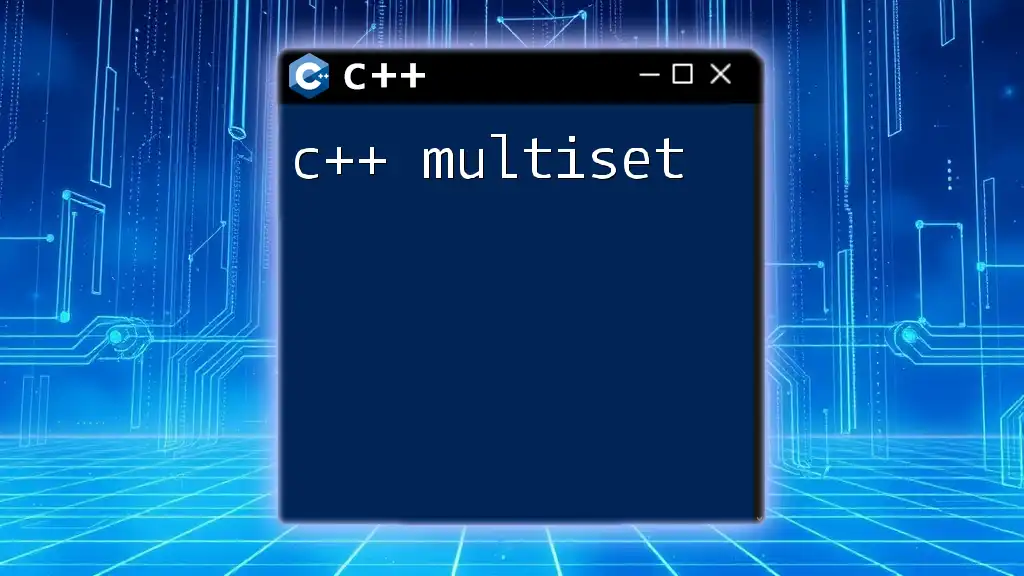
Performance Considerations
In general, the performance impact of using `nullptr` is negligible. It acts as a compile-time constant, and modern compilers optimize its usage well. Comparing it directly to legacy representations reveals no significant performance drawbacks, but its adoption leads to better-structured and safer code.
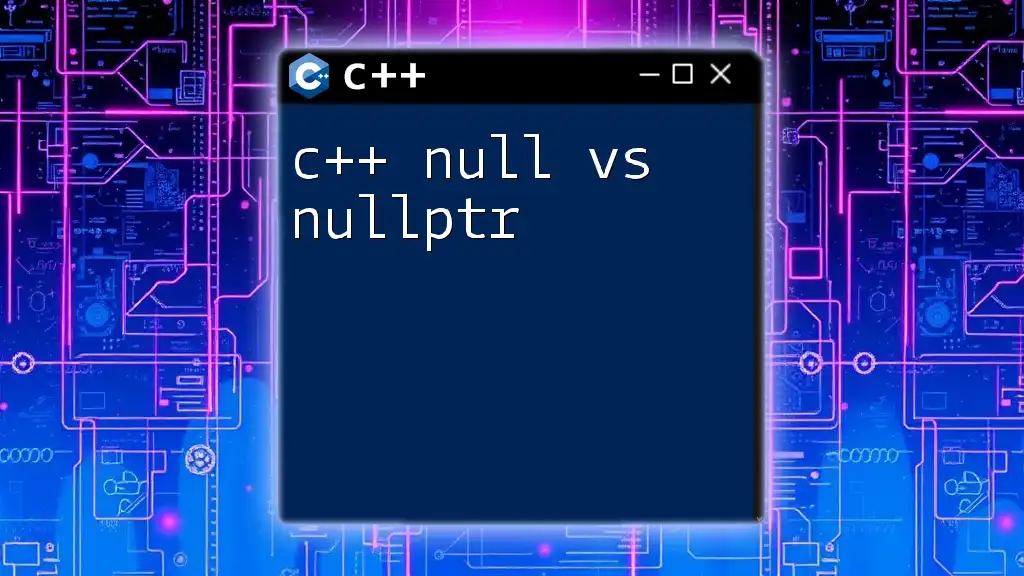
Real-world Applications of `nullptr`
In contemporary software engineering, `nullptr` is widely adopted in various applications and libraries. It has become an integral part of C++ coding standards and conventions, enhancing the overall reliability of codebases. Using `nullptr` correctly can prevent null pointer dereferencing, a common source of bugs in software applications.
For instance, many well-known open-source libraries utilize `nullptr` to ensure cleaner, safer code. Whether implementing complex data structures or handling resource management, C++ developers increasingly rely on `nullptr` for effective memory management.
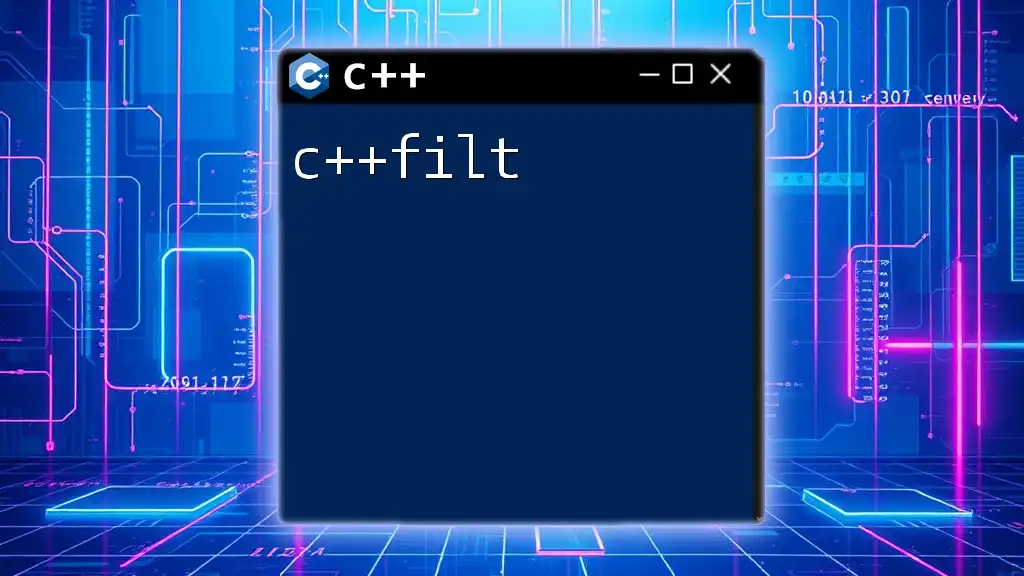
Conclusion
The introduction of `nullptr` in C++ has revolutionized how programmers handle null pointers. By providing a type-safe and clear alternative to legacy null pointer representations, `nullptr` enhances code readability, prevents ambiguities in function overloading, and ultimately leads to fewer bugs in applications.
Embrace `nullptr` in your coding practices, whether you're working on existing code or starting new projects. By doing so, you will contribute to producing widely understood, robust, and maintainable C++ applications.
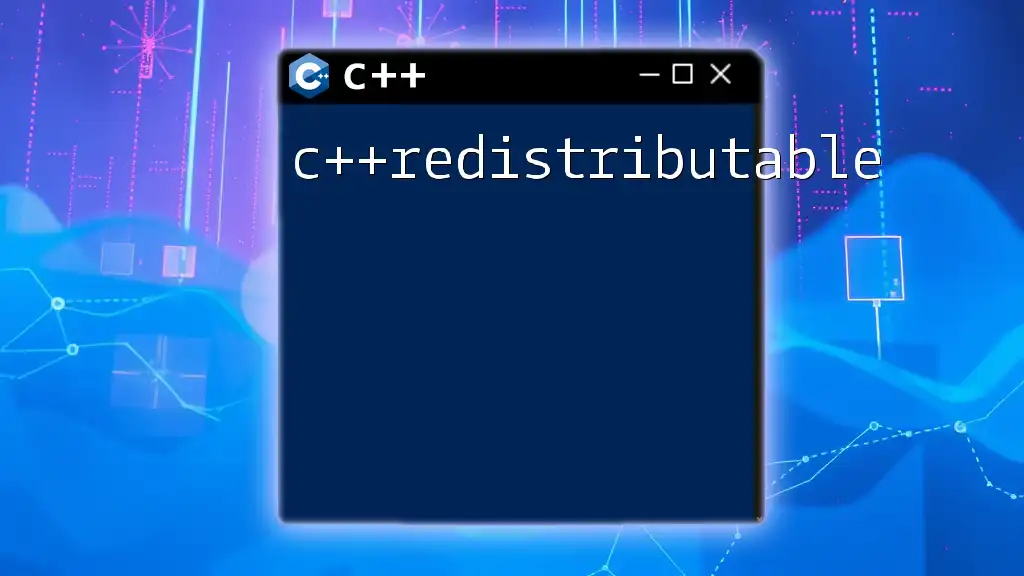
Additional Resources
For those eager to dive deeper, numerous resources are available, including the official C++ documentation, comprehensive programming books focused on C++, and online courses that cover best practices. Always strive for continuous learning to master the nuances of C++ programming and enhance your skills.
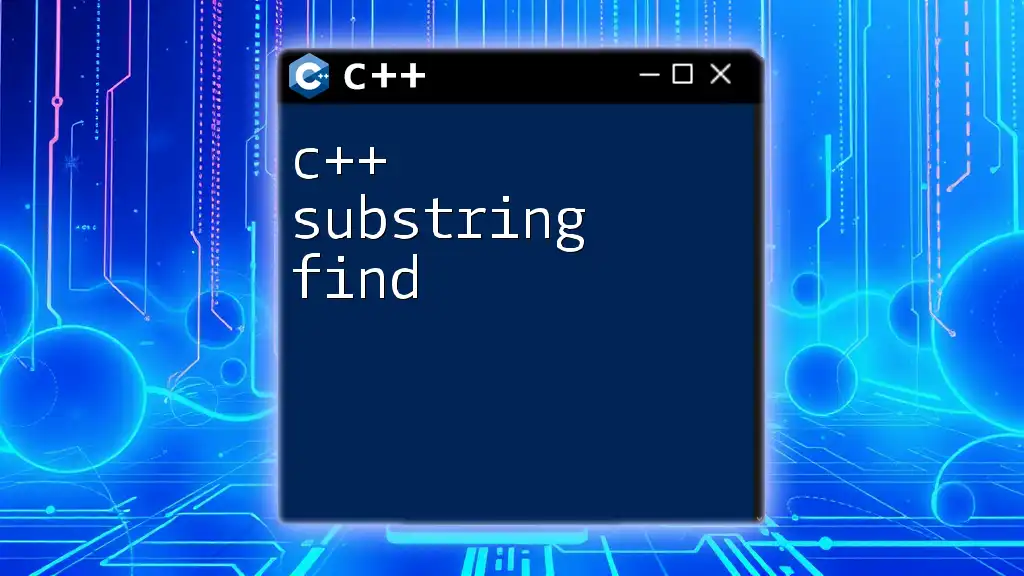
Call to Action
We encourage you to join our community for more insights, tips, and tutorials on C++. If you have questions about using `nullptr` or wish to share your experiences, please reach out—your feedback is invaluable in building a collaborative platform for learning and growth in C++.