The `mutable` keyword in C++ lambdas allows you to modify the captured variables by value, enabling changes within the lambda's body without affecting the original variables outside of it.
Here's a code snippet demonstrating the concept:
#include <iostream>
int main() {
int x = 10;
auto lambda = [x]() mutable {
x += 5; // Modifying the captured variable
std::cout << "Inside lambda: " << x << std::endl;
};
lambda();
std::cout << "Outside lambda: " << x << std::endl; // x remains unchanged
return 0;
}
What is a Lambda Function in C++?
Understanding Lambdas
A lambda function in C++ is an anonymous function that allows you to encapsulate a piece of code you can execute inline. This robust feature, introduced in C++11, enhances function objects providing a cleaner, more intuitive syntax. Lambda functions can capture variables from their surrounding scope, making them incredibly versatile and powerful for functional programming.
Basic Syntax of a Lambda
The syntax of a lambda function can be expressed as follows:
[capture_list](parameters) -> return_type {
// function body
}
Key components include:
- Capture List: Specifies which external variables to capture and how to capture them (by value or reference).
- Parameters: Defined just like those in a regular function, allowing you to pass arguments.
- Return Type: Optional; inferred by the compiler if omitted.
- Function Body: The body of the lambda, where the code execution happens.
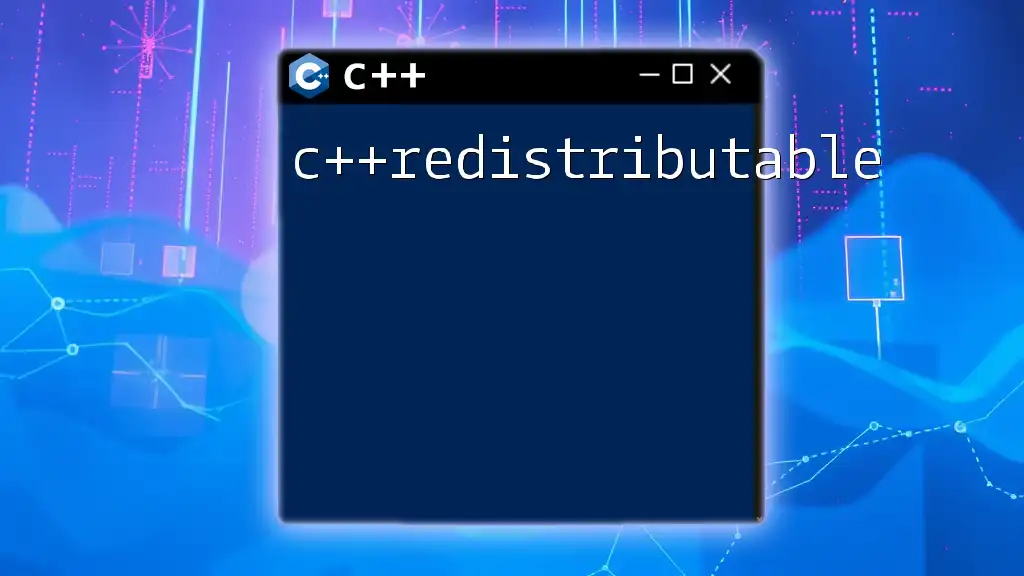
Introduction to Mutable Lambdas
What Does 'Mutable' Mean?
In the context of C++, mutable refers to the ability of a lambda function to modify captured variables when it captures them by value. By default, captured variables are immutable within the lambda's scope, prohibiting modifications. However, when you specify `mutable`, you allow these variables to be modified.
Purpose of Mutable Lambdas
Mutable lambdas are particularly useful in scenarios requiring internal state management. For instance, if you need to update an enclosed variable during each execution of the lambda, you can utilize the `mutable` keyword to achieve this.
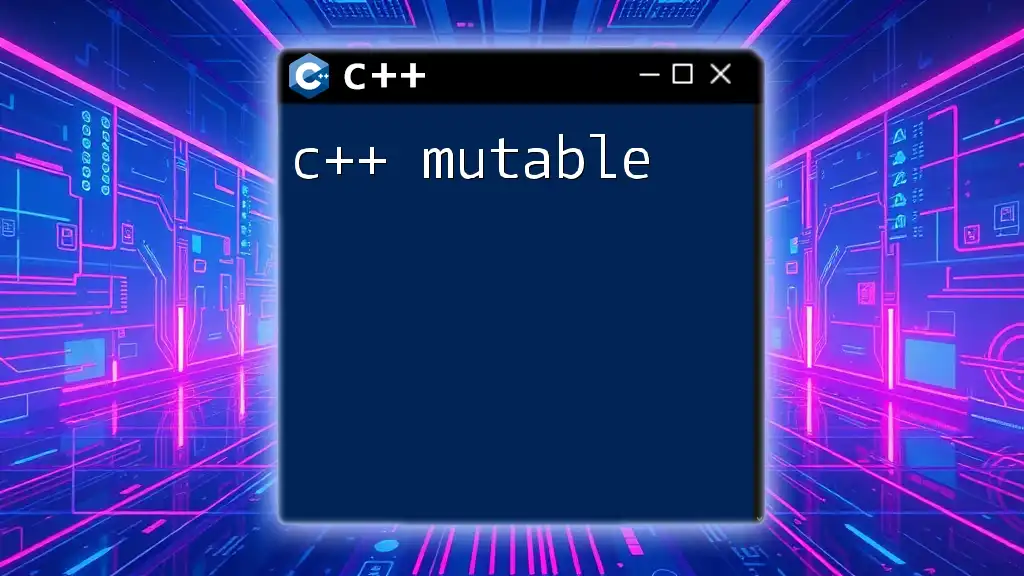
Using Mutable in Lambda Functions
Basic Syntax of a Mutable Lambda
The syntax of a mutable lambda is similar to the standard lambda, with the addition of the `mutable` keyword:
[int x = 10]() mutable {
x += 5;
std::cout << x; // Outputs 15
}
In this example, `x` is modified within the lambda’s body. Without the `mutable` keyword, any attempt to modify `x` would have resulted in a compilation error because it would be considered a constant within the lambda.
Capturing by Value vs. Capture by Reference
Capturing by Value
When capturing by value, the lambda creates a copy of the variables from the surrounding scope. This can be useful but, without the `mutable` keyword, prevents any updates to those variables.
int num = 5;
auto lambda1 = [num]() mutable { num++; std::cout << num; }; // num is modified here
Capturing by Reference
In contrast, capturing by reference allows the lambda to modify the original variable directly, which can lead to data races if used in concurrent programming.
int num = 5;
auto lambda2 = [&num]() { num++; std::cout << num; }; // num is modified here
Understanding the difference between capturing by value and by reference is crucial for managing variable lifetimes, affecting memory efficiency and potential side effects.
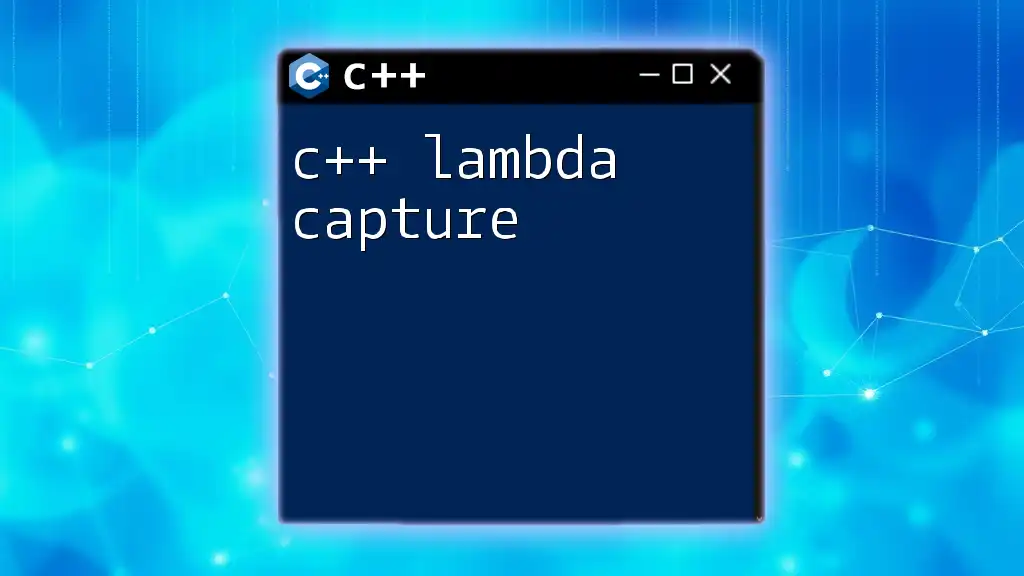
Practical Applications of Mutable Lambdas
Example 1: Using Mutable with Standard Algorithms
One of the most common applications of mutable lambdas is when combined with Standard Library algorithms. For instance, you might want to compute the sum of a collection of numbers.
std::vector<int> vec = {1, 2, 3, 4, 5};
int sum = 0;
std::for_each(vec.begin(), vec.end(), [sum](int x) mutable {
sum += x;
});
std::cout << sum; // Outputs sum of elements
In this code snippet, `sum` is computed as we modify it inside the lambda. The mutable keyword allows you to maintain state across invocations of the lambda while still capturing `sum` by value.
Example 2: Thread-Safe Lambdas
In concurrent programming, mutable lambdas prove useful for managing internal states that may need to be modified in a multi-threaded environment.
#include <thread>
#include <iostream>
void concurrentLambda() {
int count = 0;
auto func = [count]() mutable {
for (int i = 0; i < 10; ++i) {
count++;
std::cout << count << std::endl;
}
};
std::thread t1(func);
std::thread t2(func);
t1.join();
t2.join();
}
When using mutable lambdas within threads, you can separate the state for each thread. However, care must be taken to avoid data races, as each thread will have its own copy of `count`.
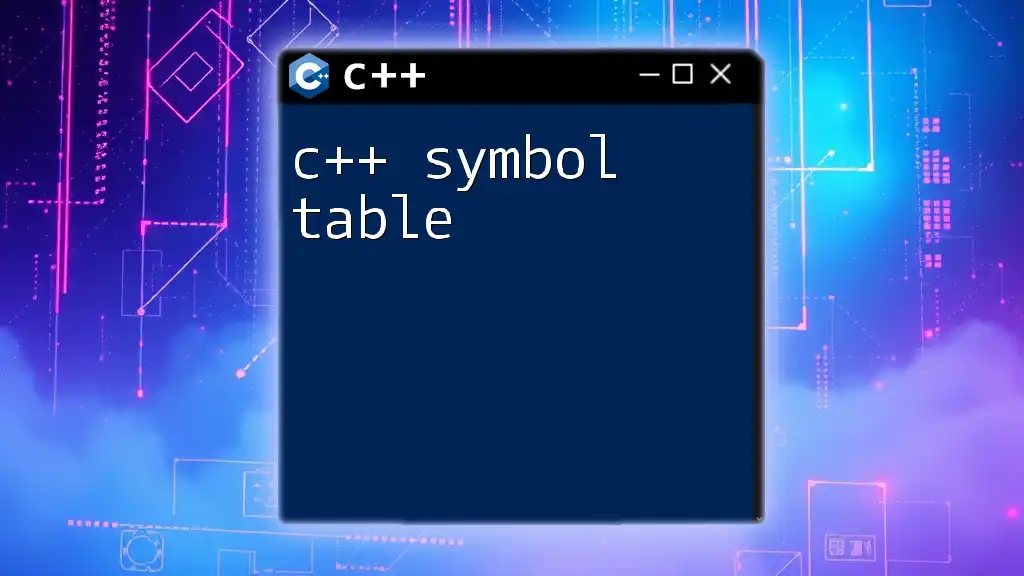
Common Pitfalls and Best Practices
Avoiding Data Races
Data races can occur when different threads modify shared variables simultaneously. To mitigate this, you can:
- Always synchronize access to shared variables using mutexes.
- Prefer capturing by value in lambdas unless reference capturing is explicitly necessary.
Performance Considerations
While mutable lambdas are powerful, they do have performance implications. Modifying state in lambda can lead to overhead due to additional copies. As a best practice, use mutable lambdas judiciously, only when necessary, to optimize performance.
Code Readability and Maintainability
While concise, the use of mutable lambdas can lead to confusion if overused. Write clear and maintainable code by keeping your lambdas simple. If the logic within a lambda becomes complex, consider refactoring the code into a separate named function for better readability.
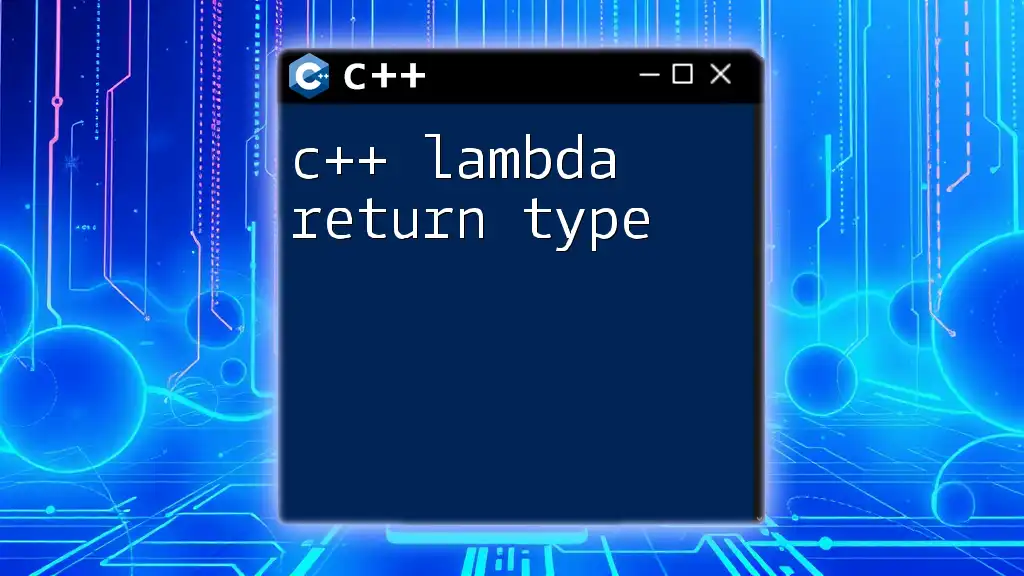
Conclusion
In summary, understanding and effectively utilizing C++ lambda mutable can significantly enhance your programming capabilities. By modifying captured variables, you can create more versatile and powerful functions that maintain their internal state. Experiment with mutable lambdas in your own coding projects, and enjoy the flexibility they bring to your programming toolkit.
Feel free to share your experiences or any questions regarding mutable lambdas in the comments below. If you wish to dive deeper into advanced C++ techniques, consider signing up for our specialized courses.