C++ assembler is a low-level programming component that allows integration of assembly language instructions within C++ code, providing developers with the ability to optimize performance-critical parts of their applications.
Here's a simple code snippet demonstrating how to use inline assembly in C++:
#include <iostream>
using namespace std;
int main() {
int result;
asm ("movl $42, %0" : "=r" (result)); // This line sets 'result' to 42
cout << "The result is: " << result << endl;
return 0;
}
Understanding Assembly Language
What is Assembly Language?
Assembly language is a low-level programming language that serves as an interface between human-readable code and machine code, which is executed directly by the processor. Unlike high-level programming languages such as C++, assembly language is closely related to computer architecture, providing fine-grained control over hardware. Each assembly language instruction corresponds to a specific machine code instruction, making it a more understandable representation of the operations executed by the CPU.
How Assembly Language Works in C++
In C++, the integration of assembly language allows developers to write performance-critical sections of code in a way that is closer to hardware. When you compile C++ code, it gets transformed into machine code through a process involving several steps – preprocessing, compilation, assembly, and linking. During this process, the compiler can generate assembly code, which can then be transformed into machine code by an assembler. Understanding this flow enables developers to optimize certain parts of their programs effectively by using assembly.

Setting Up Your Environment
Compilers and Assemblers
To start utilizing C++ assembler, you need a suitable compiler that supports assembly language. Popular options include:
- GCC (GNU Compiler Collection): A widely used compiler that supports various programming languages, including C and C++. It integrates seamlessly with assembly code.
- MSVC (Microsoft Visual C++): This compiler is commonly used in Windows environments and offers robust support for assembly language integration.
To begin coding, install your chosen compiler and configure it based on your operating system. Ensure you familiarize yourself with the specific syntax and tools related to assembly language supported by your compiler.
Writing Your First Assembly Code in C++
To demonstrate how to integrate assembly language with C++, let’s write a simple program that calls an assembly function. Here’s a basic example:
extern "C" int add(int a, int b); // Declare the assembly function
int main() {
int result = add(5, 7);
return result;
}
In this code, we're declaring an external assembly function named `add` that takes two integers as parameters. The `extern "C"` linkage specification ensures that the function follows C calling conventions, which is crucial for interoperability.
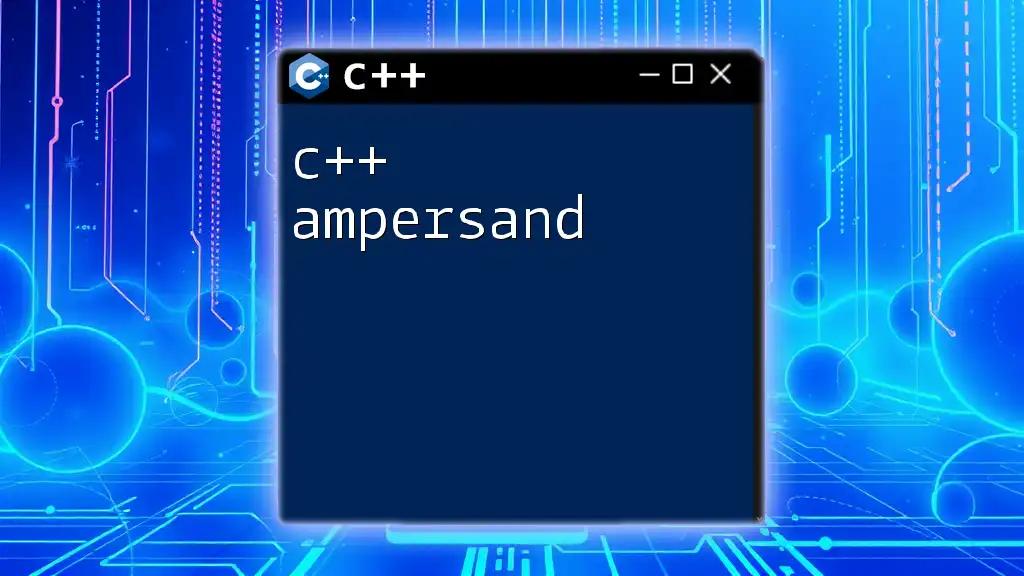
Basics of C++ Assembly Programming
Syntax and Structure
Understanding the syntax and structure of assembly language is paramount when working with C++ assembler. Assembly code consists of instructions, which are specific commands that tell the CPU what to do. These instructions often include:
- Labels: Named placeholders that represent specific addresses in code.
- Operands: Values or registers that the instruction operates on.
For example:
start:
MOV AX, 5 ; Move 5 into register AX
ADD AX, 3 ; Add 3 to the value in AX
JMP start ; Jump back to 'start'
Common Instructions in Assembly
A few instructions are fundamental to grasp when starting with assembly language:
- MOV: Moves data from one location to another.
- ADD: Adds two values.
- SUB: Subtracts one value from another.
- JMP: Jumps to a different part of the program.
For instance, the following snippet uses the `MOV` and `ADD` instructions:
MOV AX, 5 ; Move the value 5 into the AX register
ADD AX, 3 ; Add 3 to the value in AX, now AX contains 8

Integrating C++ with Assembly
Calling Assembly Functions from C++
To successfully call an assembly function from C++, the assembly routine needs to be defined properly. Let’s expand on our earlier example and implement the `add` function in assembly language:
section .text
global add ; Every function must be declared as global
add:
mov eax, edi ; Move the first argument (a) to EAX
add eax, esi ; Add the second argument (b) to EAX
ret ; Return to the caller
In this code, EAX is a register used to hold the result, and edi and esi are where the function's integer parameters are passed. The `ret` instruction signifies the end of the function, and it returns the result in EAX.
Inline Assembly in C++
Inline assembly allows embedding assembly code within C++ code, providing a way to perform lower-level operations without separate assembly files. This is useful for quick optimizations. An example of using inline assembly is as follows:
__asm__ (
"movl $2, %eax;" // Load 2 into EAX
"addl $3, %eax;" // Add 3 to EAX, resulting in EAX = 5
);
This syntax varies by compiler, but it typically involves using a keyword like `asm` or `asm`.
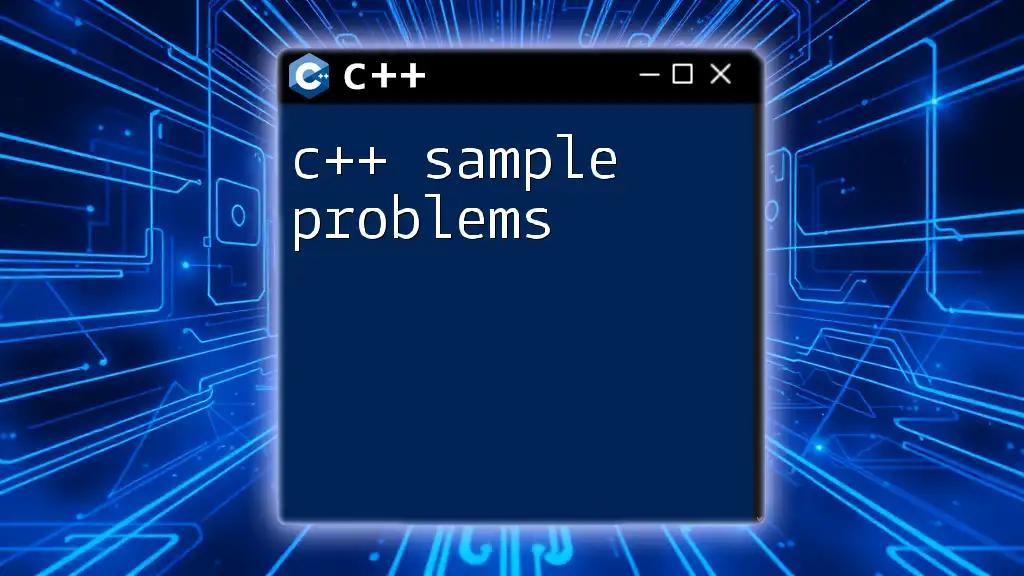
Advanced Topics in C++ Assembly
Using Inline Assembly for Optimization
Using inline assembly can lead to performance improvements in critical parts of your code, such as mathematical calculations or bit manipulation. For operations that are bottlenecks in performance, hand-optimizing the assembly can yield considerable speed gains that are hard to achieve using high-level code.
Debugging and Troubleshooting Assembly Code
When working with assembly language, debugging is crucial because assembly code can be less forgiving than C++. Common issues include:
- Mismatched calling conventions.
- Incorrect parameter usages.
- Memory management problems.
Utilizing a debugger that supports assembly can greatly assist in resolving these issues. Tools like GDB (GNU Debugger) or IDEs with built-in debuggers can show register values and the execution flow, guiding you through troubleshooting processes.
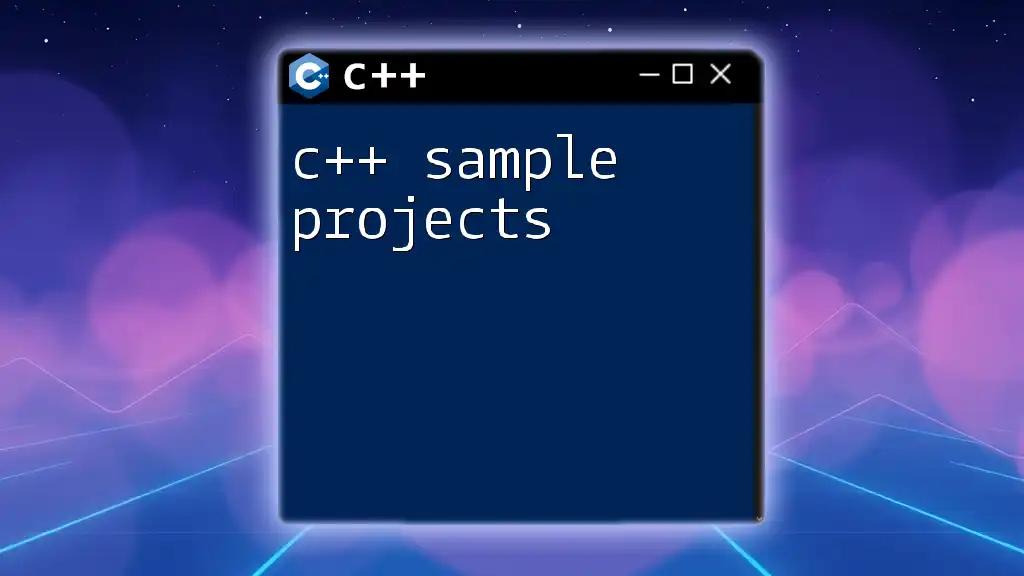
Practical Applications of C++ Assembler
Performance-Critical Applications
Assembly language can deliver substantial performance improvements in applications where speed and resource efficiency are essential. Popular fields utilizing C++ assembler include:
- Game Development: Real-time graphics and physics calculations often require optimized routines to enhance performance.
- Embedded Systems: Direct hardware manipulation is frequently needed, where assembly can interact precisely with the hardware.
- Systems Programming: Writing operating systems or device drivers benefits from the close control assembly provides over system resources.
Interfacing with Hardware
In many contexts, especially in embedded development or systems programming, C++ and assembly are utilized to interact with hardware directly. This is often critical in scenarios such as:
- Reading/writing to hardware registers.
- Implementing interrupt service routines (ISRs).
Such tasks require direct control of CPU operations that high-level languages abstract away, making a solid understanding of C++ assembler essential.

Conclusion
Understanding C++ assembler equips developers with the knowledge to write high-performance code while maintaining fine control over hardware interactions. By integrating assembly programming into C++, you can optimize time-critical sections and create efficient applications tailored to various use cases. Mastery of both C++ and assembly language enables you to leverage the strengths of each, opening the door to effective programming at both high and low levels.
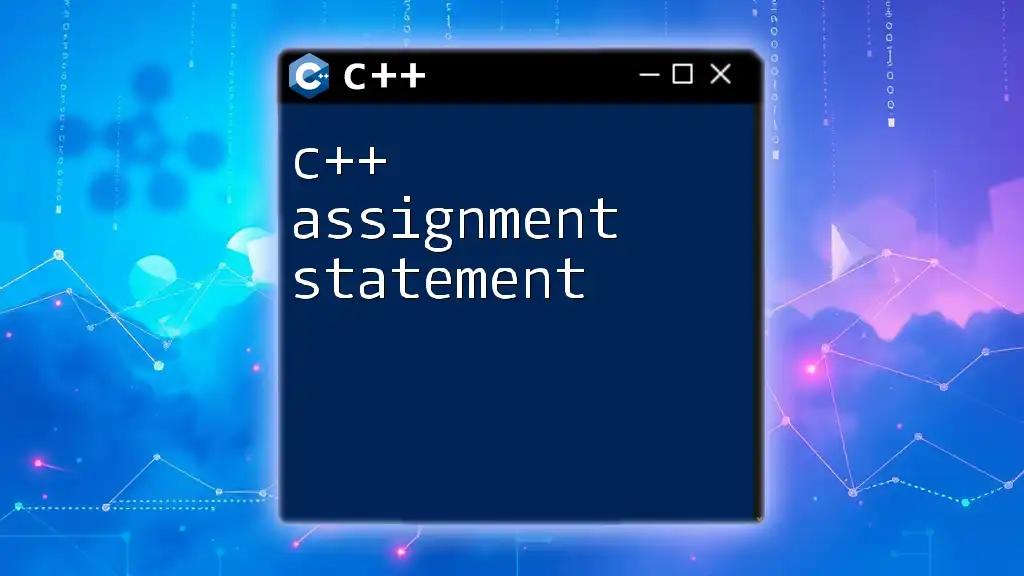
Resources for Further Learning
To further your mastery of C++ assembler, consider exploring these resources:
- Recommended books on C++ and assembly language.
- Online tutorials focused on advanced C++ and assembly programming techniques.
- Joining online communities and forums specializing in C++ and assembly for peer-to-peer learning.
With continued practice and learning, you can harness the full potential of C++ assembler in your development projects.