C++ WebAssembly enables developers to compile C++ code into a binary format that can be executed in web browsers, allowing high-performance applications to run on the web.
Here's a simple example of a C++ program compiled to WebAssembly using Emscripten:
#include <iostream>
extern "C" {
void hello() {
std::cout << "Hello, WebAssembly!" << std::endl;
}
}
To compile this code to WebAssembly using Emscripten, you would run:
emcc example.cpp -o example.js -s EXPORTED_FUNCTIONS='["_hello"]' -s MODULARIZE=1 -s EXPORT_NAME='createModule'
Understanding WebAssembly
What is WebAssembly?
WebAssembly (often abbreviated as wasm) is a binary instruction format designed to be a portable compilation target for high-level programming languages like C++. It allows developers to create applications that run at near-native speed in web browsers. Unlike traditional web technologies such as JavaScript, WebAssembly provides a way to execute code in a safe and performant manner, making it a compelling choice for performance-critical applications.
How WebAssembly Works
WebAssembly utilizes a compilation process that transforms code written in languages such as C++ into a binary format. This binary can be executed directly by modern web browsers while being abstracted away from the underlying architecture. When compiling a C++ program to WebAssembly, the Emscripten compiler generates a `.wasm` file that can be loaded and executed in your web application.
WebAssembly integrates seamlessly with HTML and JavaScript, allowing developers to harness the strengths of both. Using the WebAssembly API, you can import modules and call functions as you would with regular JavaScript.
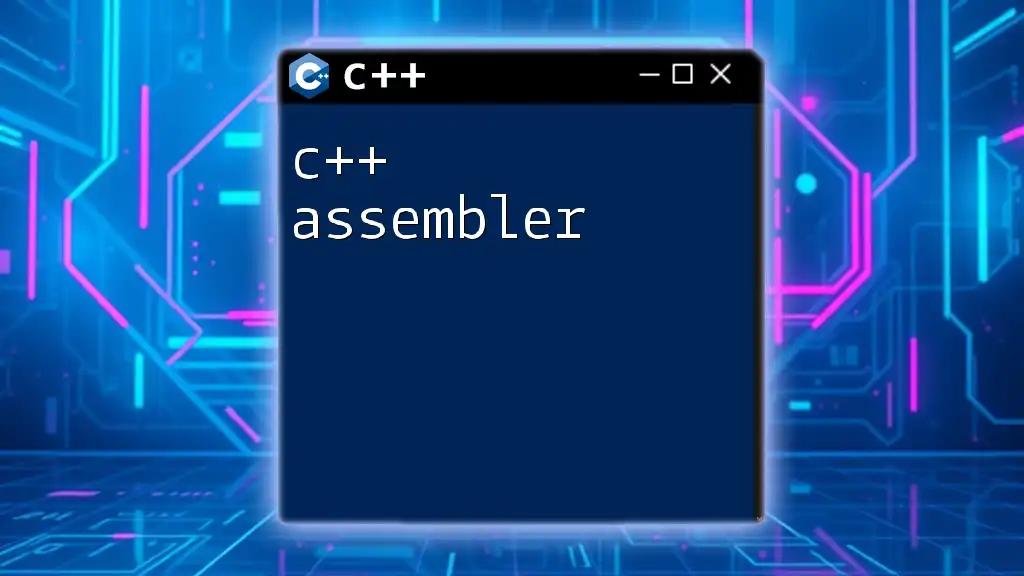
Setting Up the Environment for C++ WebAssembly
Prerequisites
Before you start coding with C++ WebAssembly, you need to ensure that your development environment is set up correctly. Here’s what you will need:
- A C++ compiler (preferably Clang)
- Emscripten SDK, which allows C++ to compile into WebAssembly
- Node.js (optional, but helpful for running local servers)
To install Emscripten, follow the official [Emscripten installation guide](https://emscripten.org/docs/getting_started/downloads.html).
Creating Your First C++ WebAssembly Project
To create your first C++ WebAssembly project, follow these steps:
- Set up your project directory: Create a new folder for your project.
- Create your C++ source file: For example, create a file named `hello.cpp`.
Here’s a simple code snippet for your “Hello, WebAssembly!” example:
#include <iostream>
extern "C" {
void say_hello() {
std::cout << "Hello, WebAssembly!" << std::endl;
}
}
- Compile your code: Use the `emcc` command to compile your .cpp file to WebAssembly:
emcc hello.cpp -o hello.html --exports=say_hello
This command will generate an HTML file and a Wasm file that you can include in your web project.

Compiling C++ to WebAssembly
Using Emscripten
Emscripten is a powerful toolchain that turns C++ code into WebAssembly. It includes various features such as the ability to interact with web APIs and JavaScript code. The primary command for compilation is `emcc`.
Compiling C++ Code
To compile your C++ code to WebAssembly using Emscripten, open a terminal and execute the following command:
emcc hello.cpp -o hello.wasm
This command will generate a WebAssembly binary file named `hello.wasm`, which can then be used in your web application.
Common Compilation Flags
Understanding Emscripten’s compilation flags can significantly enhance your project. Here are some important flags to consider:
- `-o`: Specifies the output file.
- `-s`: Sets specific configurations or optimizations.
- `-std`: Specifies the C++ standard to use (e.g., `-std=c++11`).
Here's an example using flags for optimizations:
emcc hello.cpp -o hello.wasm -O3 -s MODULARIZE=1 -s EXPORT_NAME="createModule"
In this command:
- `-O3` applies optimization for speed.
- `-s MODULARIZE=1` tells Emscripten to output a modularized format.
- `-s EXPORT_NAME` specifies the name of the exported function.

Integrating WebAssembly with JavaScript
Loading WebAssembly in a Web Application
To utilize your compiled WebAssembly module in a web application, you need to load it using JavaScript. Here’s an example of how to do this:
<script>
let instance;
async function loadWasm() {
const response = await fetch('hello.wasm');
const buffer = await response.arrayBuffer();
const module = await WebAssembly.instantiate(buffer);
instance = module.instance;
}
loadWasm();
</script>
Calling C++ Functions from JavaScript
Once your module is loaded, you can call exported C++ functions directly from JavaScript. For example, using the `say_hello` function:
<button onclick="instance.exports.say_hello()">Say Hello</button>
This button will call the `say_hello` function defined in your C++ code, allowing you to showcase interaction between C++ WebAssembly and JavaScript seamlessly.
Exporting JavaScript Functions to WebAssembly
You can also export JavaScript functions to your WebAssembly module. This is useful for interoperability. Use the `EMSCRIPTEN_KEEPALIVE` attribute in your C++ code:
#include <iostream>
extern "C" {
EMSCRIPTEN_KEEPALIVE
void js_function() {
std::cout << "Called from JavaScript!" << std::endl;
}
}
This allows you to call `js_function` from your JavaScript code.
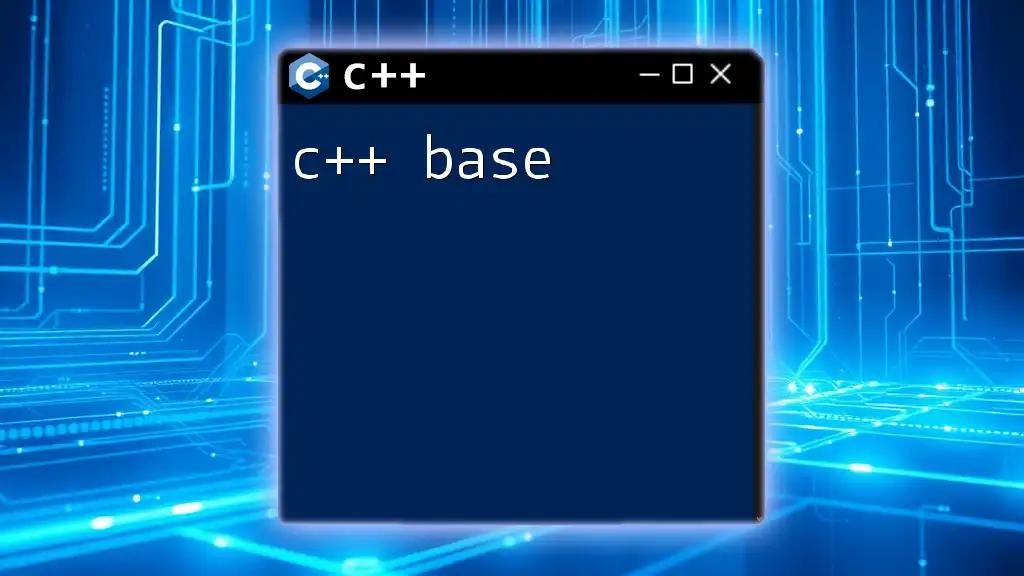
Debugging and Performance Optimization
Debugging WebAssembly
Debugging can be challenging, but Emscripten provides effective tools such as source maps. To enable debugging features, compile your code with the `-g` flag:
emcc hello.cpp -o hello.js -g
This includes debugging information that allows you to leverage browser developer tools to set breakpoints and inspect variables.
Performance Optimization Techniques
For applications that require high performance, consider these optimization techniques:
- Use the `-O3` flag for maximum optimization.
- Minimize WebAssembly size using the `-Os` flag, which optimizes for size rather than speed.
- Profile your application using tools like Chrome DevTools to identify bottlenecks.
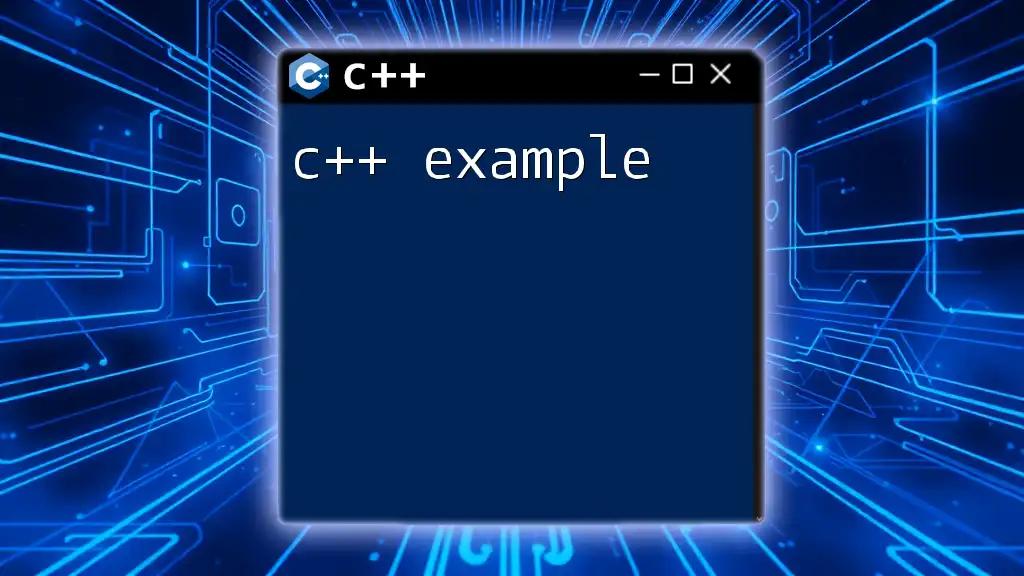
Real-World Applications of C++ WebAssembly
Game Development
C++ and WebAssembly are increasingly popular in game development, enabling developers to create games that run efficiently in web browsers. Titles like Unity and Unreal Engine leverage WebAssembly for web versions of their games, delivering high-performance experiences to users.
Web Applications
WebAssembly is also making its mark in various web applications that require intensive computations, such as image processing or data analysis tools. Examples include popular software like Figma and AutoCAD’s web versions, showcasing how C++ WebAssembly can be applied for real-time, compute-heavy tasks.
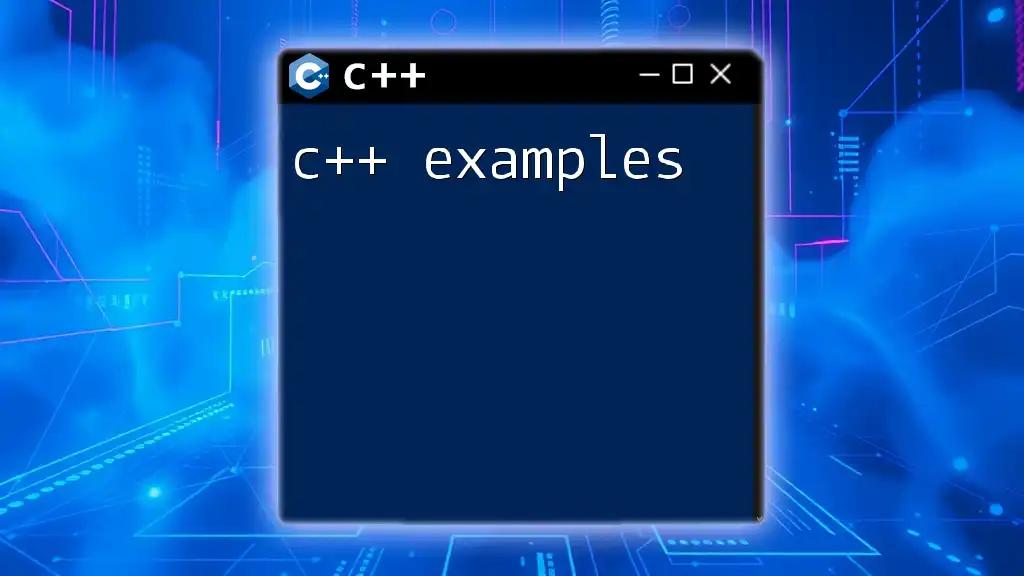
Future of C++ WebAssembly
Emerging Trends and Technologies
The future of C++ WebAssembly looks promising, with community support growing rapidly. Initiatives to improve tooling, integration into development environments, and libraries that facilitate easier use will drive adoption. Advanced features, such as threading and SIMD (Single Instruction, Multiple Data), are also on the horizon.
Resources for Further Learning
To further enhance your understanding of C++ WebAssembly, explore resources like:
- The official Emscripten documentation: [Emscripten Docs](https://emscripten.org/docs/)
- Online courses on platforms such as Udemy and Coursera
- Community forums like Stack Overflow and the Emscripten GitHub repository
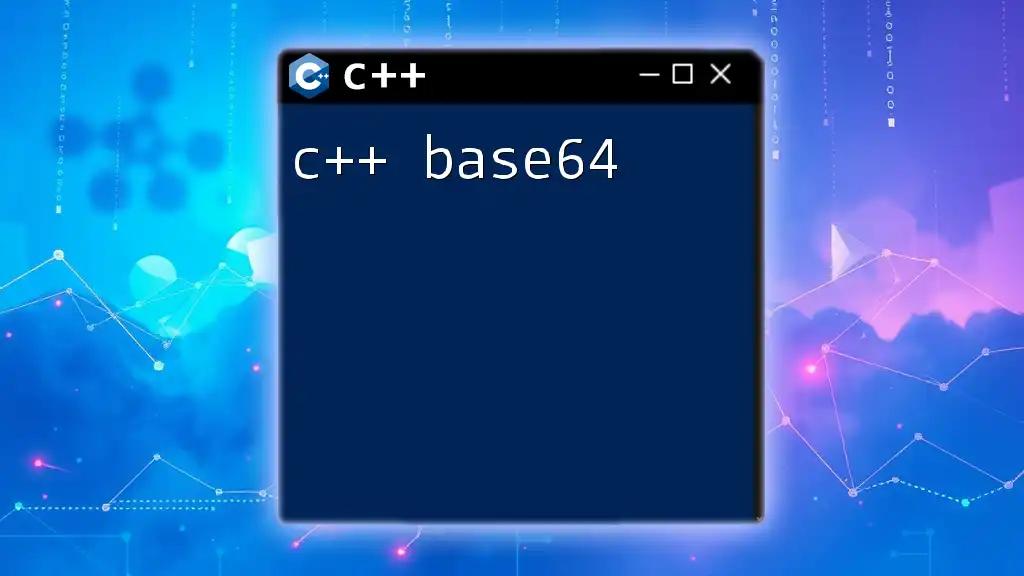
Conclusion
C++ WebAssembly represents a powerful convergence of traditional programming languages with modern web development. By enabling high-performance applications that run in the browser, C++ WebAssembly opens new doors for developers. Experimenting with C++ WebAssembly will allow you to create efficient web applications that leverage existing C++ libraries and skills, bringing the best of native performance to the web.

FAQs about C++ WebAssembly
Common Questions
One common question arises about browser support for WebAssembly. Most modern browsers, including Chrome, Firefox, Safari, and Edge, provide robust support for WebAssembly.
Troubleshooting Tips
If you encounter issues when using C++ WebAssembly, ensure that:
- Your Emscripten toolchain is correctly installed and updated.
- You’re following cross-compatibility practices, especially when dealing with different browsers.
Understanding and leveraging C++ WebAssembly will undoubtedly enhance your web development projects, offering you the performance and capability previously reserved for native applications.